[Solved-5 Solutions] ASP.NET MVC 404 Error Handling
Error Description:
ASP.NET MVC 404 Error Handling
Solution 1:
- 404 Errors are a little trickier to handle in ASP.NET MVC. We will walk through the following steps to nail all kinds of 404 errors in our application
- Step 1: To start off with, we will update our Web.config to route 404 errors to a different view called ‘FailWhale’

Learn dotnet - dotnet tutorial - dotnet failwhale - dotnet examples - dotnet programs
- Step 2: Add an empty View in the Shared folder and call it FailWhale.cshtml
- Step 3: Add an empty Controller called ErrorController and update it with an action method as follows
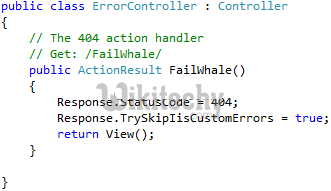
Learn dotnet - dotnet tutorial - dotnet seja - dotnet examples - dotnet programs
We are updating the Response.StatusCode to 404 so that robots scanning the URL get the 404.
- We are setting the TrySkipIisCustomErrors = true so that IIS doesn’t try to hijack the 404 and show it’s own error page. Without this, when remote users try to navigate to an invalid URL they will see the IIS 404 error page instead of your custom FailWhale page.
- Finally we are returning the default “FailWhale” view.
- Step 4: Update the Routes (RouteConfig.cs in MVC4) to send the 404 error view to the Error Controller
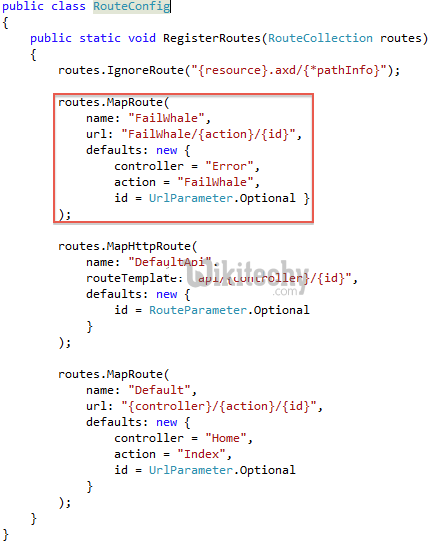
Learn dotnet - dotnet tutorial - dotnet reet - dotnet examples - dotnet programs
Logging the 404 error
- The 404 error is not available in the above ErrorController because ASP.NET has already handled the error, bubbled it up to the routing framework that is using the web.config setting to route the request to the ‘redirected page’.
- Essentially it is a 302 redirection. To log the error, we have to handle the global Application_Error event and update the Global.asax as follows
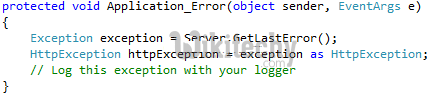
Learn dotnet - dotnet tutorial - dotnet application error - dotnet examples - dotnet programs
Solution 2:
- Put this as the last route in Global.asax
routes.MapRoute("Error", "{*url}", new { controller = "Error", action = "Error" });
click below button to copy the code. By - .Net tutorial - team
- This will trap anything that doesn't conform to the MVC model of /Controller/Action/ID
- It will send it to the ErrorController's Error Action which will direct to the 404 View.
- If we enter a valid Controller with a non-existent Action then handle the HandleUnknownAction in our controller. What we have done is inherit all controllers of a base controller that handles this method and then redirects to a Index view. Of course we have to be confident all controllers have a index view!
Solution 3:
- Yet another solution.
- Add ErrorControllers or static page to with 404 error information.
- Modify web.config (in case of controller).
<customErrors mode="On" >
<error statusCode="404" redirect="~/Errors/Error404" />
</customErrors>
click below button to copy the code. By - .Net tutorial - team
- Or in case of static page
<customErrors mode="On" >
<error statusCode="404" redirect="~/Static404.html" />
</customErrors>
click below button to copy the code. By - .Net tutorial - team
- This will handle both missed routes and missed actions.
Solution 4:
- The response from Marco is the BEST solution. Of course, we have extended the solution and created a full error management system that manages everything.
Code:
protected void Application_EndRequest()
{
if (Context.Response.StatusCode == 404)
{
var exception = Server.GetLastError();
var httpException = exception as HttpException;
Response.Clear();
Server.ClearError();
var routeData = new RouteData();
routeData.Values["controller"] = "ErrorManager";
routeData.Values["action"] = "Fire404Error";
routeData.Values["exception"] = exception;
Response.StatusCode = 500;
if (httpException != null)
{
Response.StatusCode = httpException.GetHttpCode();
switch (Response.StatusCode)
{
case 404:
routeData.Values["action"] = "Fire404Error";
break;
}
}
// Avoid IIS7 getting in the middle
Response.TrySkipIisCustomErrors = true;
IController errormanagerController = new ErrorManagerController();
HttpContextWrapper wrapper = new HttpContextWrapper(Context);
var rc = new RequestContext(wrapper, routeData);
errormanagerController.Execute(rc);
}
}
and inside ErrorManagerController :
public void Fire404Error(HttpException exception)
{
//you can place any other error handling code here
throw new PageNotFoundException("page or resource");
}
click below button to copy the code. By - .Net tutorial - team
- Now if we throwing a Custom Exception that we have created. And that Controller is inheriting from a custom Controller Based class that we have created. The Custom Base Controller was created to override error handling. Here is custom Base Controller class:
public class MyBasePageController : Controller
{
protected override void OnException(ExceptionContext filterContext)
{
filterContext.GetType();
filterContext.ExceptionHandled = true;
this.View("ErrorManager", filterContext).ExecuteResult(this.ControllerContext);
base.OnException(filterContext);
}
}
click below button to copy the code. By - .Net tutorial - team
- The "ErrorManager" in the above code is just a view that is using a Model based on ExceptionContext.
Solution 5:
- Create the following pages in the root of our application:
- 404.html - for IIS
- 404.aspx - for ASP.NET
- 500.html - for IIS
- 500.aspx - for ASP.NET
- Ensure we set the appropriate response status code within the ASPX pages.
- Ditch the MVC HandleErrorAttribute global filter and configure ASP.NET's custom errors as below:
<customErrors mode="RemoteOnly" redirectMode="ResponseRewrite" defaultRedirect="~/500.aspx">
<error statusCode="404" redirect="~/404.aspx"/>
<error statusCode="500" redirect="~/500.aspx"/>
</customErrors>
click below button to copy the code. By - .Net tutorial - team
- Configure IIS's custom errors as below:
<httpErrors errorMode="DetailedLocalOnly">
<remove statusCode="404"/>
<error statusCode="404" path="404.html" responseMode="File"/>
<remove statusCode="500"/>
<error statusCode="500" path="500.html" responseMode="File"/>
</httpErrors>