C program remove spaces or blanks from a string, For example consider the string
"c programming"
There are two spaces in this string, so our program will print a string
“c programming”. It will remove spaces when they occur more than one time consecutively in string anywhere.
[ad type=”banner”]
C programming code
[pastacode lang=”c” manual=”%23include%20%3Cstdio.h%3E%0A%20%0Aint%20main()%0A%7B%0A%20%20%20char%20text%5B1000%5D%2C%20blank%5B1000%5D%3B%0A%20%20%20int%20c%20%3D%200%2C%20d%20%3D%200%3B%0A%20%0A%20%20%20printf(%22Enter%20some%20text%5Cn%22)%3B%0A%20%20%20gets(text)%3B%0A%20%0A%20%20%20while%20(text%5Bc%5D%20!%3D%20’%5C0′)%20%7B%0A%20%20%20%20%20%20if%20(text%5Bc%5D%20%3D%3D%20’%20′)%20%7B%0A%20%20%20%20%20%20%20%20%20int%20temp%20%3D%20c%20%2B%201%3B%0A%20%20%20%20%20%20%20%20%20if%20(text%5Btemp%5D%20!%3D%20’%5C0′)%20%7B%0A%20%20%20%20%20%20%20%20%20%20%20%20while%20(text%5Btemp%5D%20%3D%3D%20’%20’%20%26%26%20text%5Btemp%5D%20!%3D%20’%5C0′)%20%7B%0A%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20if%20(text%5Btemp%5D%20%3D%3D%20’%20′)%20%7B%0A%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20c%2B%2B%3B%0A%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%7D%20%20%0A%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20temp%2B%2B%3B%0A%20%20%20%20%20%20%20%20%20%20%20%20%7D%0A%20%20%20%20%20%20%20%20%20%7D%0A%20%20%20%20%20%20%7D%0A%20%20%20%20%20%20blank%5Bd%5D%20%3D%20text%5Bc%5D%3B%0A%20%20%20%20%20%20c%2B%2B%3B%0A%20%20%20%20%20%20d%2B%2B%3B%0A%20%20%20%7D%0A%20%0A%20%20%20blank%5Bd%5D%20%3D%20’%5C0’%3B%0A%20%0A%20%20%20printf(%22Text%20after%20removing%20blanks%5Cn%25s%5Cn%22%2C%20blank)%3B%0A%20%0A%20%20%20return%200%3B%0A%7D” message=”” highlight=”” provider=”manual”/]
If you want you can copy blank into text string so that original string is modified.
Output of program:
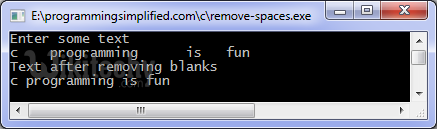
C programming code using pointers
[ad type=”banner”]
[pastacode lang=”c” manual=”%23include%20%3Cstdio.h%3E%0A%23include%20%3Cstring.h%3E%0A%23include%20%3Cstdlib.h%3E%0A%23define%20SPACE%20’%20’%0A%20%0Achar%20*process(char*)%3B%0A%20%0Aint%20main()%0A%7B%0A%20%20%20char%20text%5B1000%5D%2C%20*r%3B%0A%20%0A%20%20%20printf(%22Enter%20a%20string%5Cn%22)%3B%0A%20%20%20gets(text)%3B%0A%20%0A%20%20%20r%20%3D%20process(text)%3B%0A%20%0A%20%20%20printf(%22%5C%22%25s%5C%22%5Cn%22%2C%20r)%3B%0A%20%0A%20%20%20free(r)%3B%20%20%20%20%20%0A%20%0A%20%20%20return%200%3B%0A%7D%0A%20%0Achar%20*process(char%20*text)%20%7B%0A%20%20%20int%20length%2C%20c%2C%20d%3B%0A%20%20%20char%20*start%3B%0A%20%0A%20%20%20c%20%3D%20d%20%3D%200%3B%0A%20%0A%20%20%20length%20%3D%20strlen(text)%3B%0A%20%0A%20%20%20start%20%3D%20(char*)malloc(length%2B1)%3B%0A%20%0A%20%20%20if%20(start%20%3D%3D%20NULL)%0A%20%20%20%20%20%20exit(EXIT_FAILURE)%3B%0A%20%0A%20%20%20while%20(*(text%2Bc)%20!%3D%20’%5C0′)%20%7B%0A%20%20%20%20%20%20if%20(*(text%2Bc)%20%3D%3D%20’%20′)%20%7B%0A%20%20%20%20%20%20%20%20%20int%20temp%20%3D%20c%20%2B%201%3B%0A%20%20%20%20%20%20%20%20%20if%20(*(text%2Btemp)%20!%3D%20’%5C0′)%20%7B%0A%20%20%20%20%20%20%20%20%20%20%20%20while%20(*(text%2Btemp)%20%3D%3D%20’%20’%20%26%26%20*(text%2Btemp)%20!%3D%20’%5C0′)%20%7B%0A%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20if%20(*(text%2Btemp)%20%3D%3D%20’%20′)%20%7B%0A%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20c%2B%2B%3B%0A%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20%7D%20%20%0A%20%20%20%20%20%20%20%20%20%20%20%20%20%20%20temp%2B%2B%3B%0A%20%20%20%20%20%20%20%20%20%20%20%20%7D%0A%20%20%20%20%20%20%20%20%20%7D%0A%20%20%20%20%20%20%7D%0A%20%20%20%20%20%20*(start%2Bd)%20%3D%20*(text%2Bc)%3B%0A%20%20%20%20%20%20c%2B%2B%3B%0A%20%20%20%20%20%20d%2B%2B%3B%0A%20%20%20%7D%0A%20%20%20*(start%2Bd)%3D%20’%5C0’%3B%0A%20%0A%20%20%20return%20start%3B%0A%7D” message=”” highlight=”” provider=”manual”/]
Super concept
it is really amazing!