java tutorial - Руководство Java Reflection - учебник java - java programming - учиться java - java basics - java for beginners
1- Что такое Java Reflection?
- TODO
2- Неокоторые классы участвующие в примерах
- Вот некоторые из классов, участвующих в примерах данной статьи.
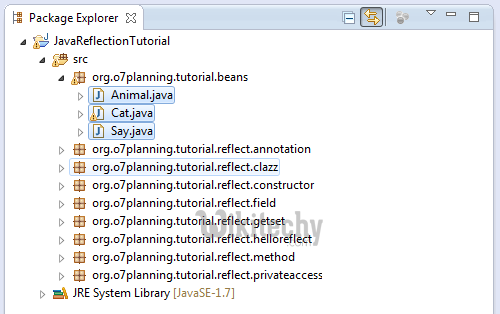
java - джава - учиться java - учебник java -
Обозреватель пакетов Java
- примеры java - java-программы
Animal.java
package com.wikitechy.tutorial.beans;
public abstract class Animal {
public String getLocation() {
return "Earth";
}
public abstract int getNumberOfLegs() ;
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - командаSay.java
package com.wikitechy.tutorial.beans;
public interface Say {
public String say();
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - командаCat.java
package com.wikitechy.tutorial.beans;
public class Cat extends Animal implements Say {
public static final String SAY = "Meo meo";
public static final int NUMBER_OF_LEGS = 4;
// Private field.
private String name;
// Private field
public int age;
public Cat() {
}
public Cat(String name) {
this.name = name;
this.age = 1;
}
public Cat(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return this.name;
}
// Private Method.
private void setName(String name) {
this.name = name;
}
public int getAge() {
return this.age;
}
public void setAge(int age) {
this.age = age;
}
/**
* Implements from interface Say.
*/
@Override
public String say() {
return SAY;
}
/**
* Implements from Animal.
*/
@Override
public int getNumberOfLegs() {
return NUMBER_OF_LEGS;
}
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - команда3- Начнем с простого примера
- Вот простой пример извлечения списка публичных методов класса, включая методы, унаследованные от родительского класса, и интерфейсы.
ListMethod.java
package com.wikitechy.tutorial.reflect.helloreflect;
import java.lang.reflect.Method;
public class ListMethod {
// Protected method
protected void info() {
}
public static void testMethod1() {
}
public void testMethod2() {
}
public static void main(String[] args) {
// Get a list of public methods of this class
// Include methods inherited from the parent class, or interface.
// Lấy ra danh sách các method public của class này
// Bao gồm các các method thừa kế từ class cha, hoặc các interface.
Method[] methods = ListMethod.class.getMethods();
for (Method method : methods) {
System.out.println("Method " + method.getName());
}
}
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - командаЗапуск примера:
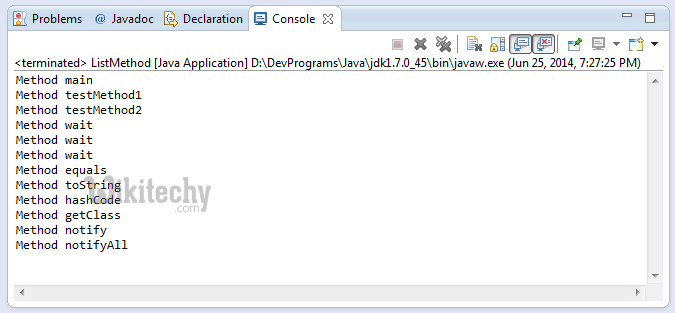
java - джава - учиться java - учебник java -
Список метод
- примеры java - java-программы
4- Class
- Некоторые важные методы в Reflection, относящиеся к классу.
- Пример выписывает основные сведения о классе, такие как имя класса, пакет (package), модификатор (modifier), ..
ShowClassInfo.java
package com.wikitechy.tutorial.reflect.clazz;
import java.lang.reflect.Modifier;
public final class ShowClassInfo {
public static void main(String[] args) {
// Get Class object represent ShowClassInfo class.
Class<ShowClassInfo> aClass = ShowClassInfo.class;
// Print out class name, including the package name.
System.out.println("Class Name= " + aClass.getName());
// Print out simple class name (without package name).
System.out.println("Simple Class Name= " + aClass.getSimpleName());
// Package info
Package pkg = aClass.getPackage();
System.out.println("Package Name = " + pkg.getName());
// Modifier
int modifiers = aClass.getModifiers();
boolean isPublic = Modifier.isPublic(modifiers);
boolean isInterface = Modifier.isInterface(modifiers);
boolean isAbstract = Modifier.isAbstract(modifiers);
boolean isFinal = Modifier.isFinal(modifiers);
// true
System.out.println("Is Public? " + isPublic);
// true
System.out.println("Is Final? " + isFinal);
// false
System.out.println("Is Interface? " + isInterface);
// false
System.out.println("Is Abstract? " + isAbstract);
}
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - командаЗапуск примера:
- Пример для записи информации класса Cat, такого как имя класса, и интерфейсов, которые реализует этот класс.
ShowClassCatInfo.java
package com.wikitechy.tutorial.reflect.clazz;
import com.wikitechy.tutorial.beans.Cat;
public class ShowClassCatInfo {
public static void main(String[] args) {
// The Class object represent Cat class
Class<Cat> aClass = Cat.class;
// Class name
System.out.println("Simple Class Name = " + aClass.getSimpleName());
// Get the Class object represent parent of Cat class
Class<?> aSuperClass = aClass.getSuperclass();
System.out.println("Simple Class Name of Super class = "
+ aSuperClass.getSimpleName());
// Determines the interfaces implemented by the class
// or interface represented by this object.
Class<?>[] itfClasses = aClass.getInterfaces();
for (Class<?> itfClass : itfClasses) {
System.out.println("Interface: " + itfClass.getSimpleName());
}
}
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - командаЗапуск примера:
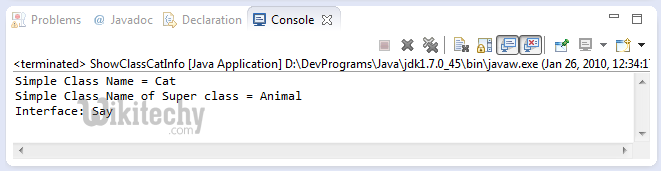
java - джава - учиться java - учебник java -
шоу класса java
- примеры java - java-программы
- Пример получения информации о конструкторе, методе, поле класса (только публичные), включая публичные методы, общедоступное поле, унаследованное от родительского класса, интерфейсы.
ShowMemberInfo.java
package com.wikitechy.tutorial.reflect.clazz;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import com.wikitechy.tutorial.beans.Cat;
public class ShowMemberInfo {
public static void main(String[] args) {
// Get object represent Cat class.
Class<Cat> aClass = Cat.class;
// Get Constructor array of Cat.
Constructor<?>[] constructors = aClass.getConstructors();
System.out.println(" ==== CONSTRUCTORs: ===== ");
for (Constructor<?> constructor : constructors) {
System.out.println("Constructor: " + constructor.getName());
}
// Get a list of public method of Cat
// Include the methods inherited from the parent class and the interfaces
Method[] methods = aClass.getMethods();
System.out.println(" ==== METHODs: ====== ");
for (Method method : methods) {
System.out.println("Method: " + method.getName());
}
// Get the list of the public fields
// Include the fields inherited from the parent class, and the interfaces
Field[] fields = aClass.getFields();
System.out.println(" ==== FIELDs: ====== ");
for (Field field : fields) {
System.out.println("Field: " + field.getName());
}
}
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - командаРезультаты:
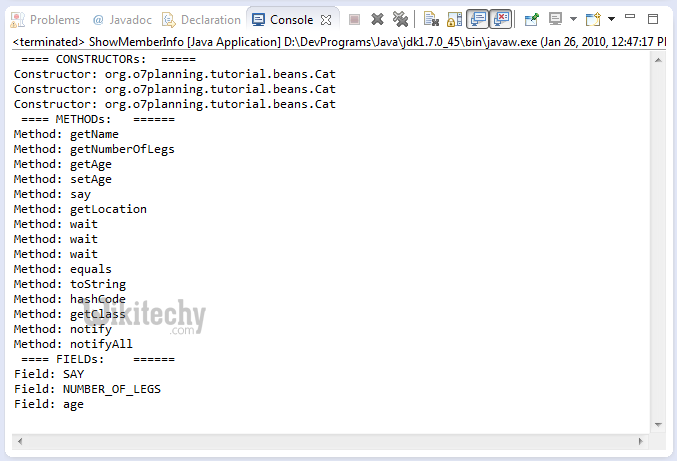
java - джава - учиться java - учебник java -
член шоу java
- примеры java - java-программы
5- Constructor
- Пример получения конструктора (Constructor) с указанными параметрами. И записать информацию об этом конструкторе (constructor).
ConstructorExample.java
package com.wikitechy.tutorial.reflect.constructor;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import com.wikitechy.tutorial.beans.Cat;
public class ConstructorExample {
public static void main(String[] args) throws NoSuchMethodException,
SecurityException, InstantiationException, IllegalAccessException,
IllegalArgumentException, InvocationTargetException {
// Get Class object represent Cat class.
Class<Cat> aClass = Cat.class;
// Get the Constructor object of the public constructor
// that matches the specified parameterTypes
Constructor<?> constructor = aClass.getConstructor(String.class,
int.class);
// Get parameter array of this constructor.
Class<?>[] paramClasses = constructor.getParameterTypes();
for (Class<?> paramClass : paramClasses) {
System.out.println("Param: " + paramClass.getSimpleName());
}
// Initialize the object in the usual way
Cat tom = new Cat("Tom", 3);
System.out
.println("Cat 1: " + tom.getName() + ", age =" + tom.getAge());
// Using Java reflection to create object
Cat tom2 = (Cat) constructor.newInstance("Tom", 2);
System.out.println("Cat 2: " + tom.getName() + ", age ="
+ tom2.getAge());
}
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - командаРезультаты запуска класса:
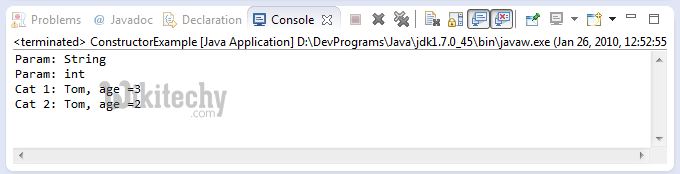
java - джава - учиться java - учебник java -
Пример конструктора
- примеры java - java-программы
6- Field
- В следующем примере извлекается поле ( field.....) с указанным именем.
FieldExample.java
package com.wikitechy.tutorial.reflect.field;
import java.lang.reflect.Field;
import com.wikitechy.tutorial.beans.Cat;
public class FieldExample {
public static void main(String[] args) throws NoSuchFieldException,
SecurityException, IllegalArgumentException, IllegalAccessException {
// Get Class object represent Cat class
Class<Cat> aClass = Cat.class;
// Get Field object represent field 'NUMBER_OF_LEGS'.
Field field = aClass.getField("NUMBER_OF_LEGS");
Class<?> fieldType = field.getType();
System.out.println("Field type: " + fieldType.getSimpleName());
Field ageField = aClass.getField("age");
Cat tom = new Cat("Tom", 5);
// Returns the value of the field represented by this Field,
// on the specified object.
Integer age = (Integer) ageField.get(tom);
System.out.println("Age = " + age);
// Sets the field represented by this Field object on
// the specified object argument to the specified new value.
ageField.set(tom, 7);
System.out.println("New Age = "+ tom.getAge());
}
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - командаРезультаты запуска класса:
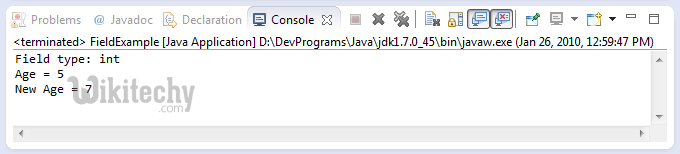
java - джава - учиться java - учебник java -
Пример поля
- примеры java - java-программы
7- Method
- Пример получения метода с указанным именем и указанными параметрами. Запишите информацию об этом методе, такую как вид возвращаемого значения, список параметров, ...
MethodExample.java
package com.wikitechy.tutorial.reflect.method;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import com.wikitechy.tutorial.beans.Cat;
public class MethodExample {
public static void main(String[] args) throws NoSuchMethodException,
SecurityException, IllegalAccessException,
IllegalArgumentException, InvocationTargetException {
// Class object represent Cat class
Class<Cat> aClass = Cat.class;
// Method object represent getAge() method.
Method getAgeMethod = aClass.getMethod("getAge");
// return type of method.
Class<?> returnType= getAgeMethod.getReturnType();
System.out.println("Return type of getAge: "+ returnType.getSimpleName());
Cat tom = new Cat("Tom", 7);
// Call method 'getAge' way Reflect
// This is equivalent to calling: tom.getAge()
int age = (int) getAgeMethod.invoke(tom);
System.out.println("Age = " + age);
// Method object represent setAge(int) method of Cat class.
Method setAgeMethod = aClass.getMethod("setAge", int.class);
// Call method setAge(int) way Reflect
// This is equivalent to calling: tom.setAge(5)
setAgeMethod.invoke(tom, 5);
System.out.println("New Age = " + tom.getAge());
}
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - командаРезультаты запуска класса:
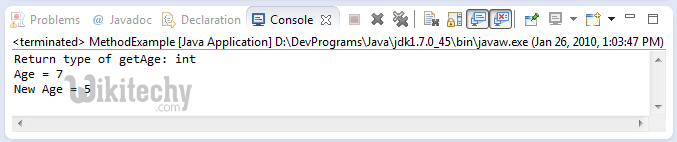
java - джава - учиться java - учебник java -
Пример метода
- примеры java - java-программы
8- Методы getter и setter
- В следующем примере перечислены методы public setter и public getter класса.
GetSetExample.java
package com.wikitechy.tutorial.reflect.getset;
import java.lang.reflect.Method;
import com.wikitechy.tutorial.beans.Cat;
public class GetSetExample {
// Method is getter if names start with get, and no parameters.
public static boolean isGetter(Method method) {
if (!method.getName().startsWith("get")) {
return false;
}
if (method.getParameterTypes().length != 0) {
return false;
}
if (void.class.equals(method.getReturnType())) {
return false;
}
return true;
}
// Method is setter if names start with set, and only one parameter.
public static boolean isSetter(Method method) {
if (!method.getName().startsWith("set")) {
return false;
}
if (method.getParameterTypes().length != 1) {
return false;
}
return true;
}
public static void main(String[] args) {
// Class object represet Cat class
Class<Cat> aClass = Cat.class;
// public methods
Method[] methods = aClass.getMethods();
for (Method method : methods) {
boolean isSetter = isSetter(method);
boolean isGetter = isGetter(method);
System.out.println("Method: " + method.getName());
System.out.println(" - Is Setter? " + isSetter);
System.out.println(" - Is Getter? " + isGetter);
}
}
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - командаРезультаты запуска программы:
Method: getName
- Is Setter? false
- Is Getter? true
Method: getNumberOfLegs
- Is Setter? false
- Is Getter? true
Method: getAge
- Is Setter? false
- Is Getter? true
Method: setAge
- Is Setter? true
- Is Getter? false
Method: say
- Is Setter? false
- Is Getter? false
Method: getLocation
- Is Setter? false
- Is Getter? true
Method: wait
- Is Setter? false
- Is Getter? false
Method: wait
- Is Setter? false
- Is Getter? false
Method: wait
- Is Setter? false
- Is Getter? false
Method: equals
- Is Setter? false
- Is Getter? false
Method: toString
- Is Setter? false
- Is Getter? false
Method: hashCode
- Is Setter? false
- Is Getter? false
Method: getClass
- Is Setter? false
- Is Getter? true
Method: notify
- Is Setter? false
- Is Getter? false
Method: notifyAll
- Is Setter? false
- Is Getter? false
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - команда9- Доступ в приватный метод (private method), поле (field)
AccessPrivateFieldExample.java
package com.wikitechy.tutorial.reflect.privateaccess;
import java.lang.reflect.Field;
import com.wikitechy.tutorial.beans.Cat;
public class AccessPrivateFieldExample {
public static void main(String[] args) throws IllegalArgumentException,
IllegalAccessException, NoSuchFieldException, SecurityException {
// Class object represent Cat class
Class<Cat> aClass = Cat.class;
// Class.getField(String) get public field only.
// Use Class.getDeclaredField(String):
// Get the Field object of field declared in class.
Field private_nameField = aClass.getDeclaredField("name");
// Allows for access to private field.
// Avoid IllegalAccessException
private_nameField.setAccessible(true);
Cat tom = new Cat("Tom");
String fieldValue = (String) private_nameField.get(tom);
System.out.println("Value field name = " + fieldValue);
// Set new valud for 'name' field.
private_nameField.set(tom, "Tom Cat");
System.out.println("New name = " + tom.getName());
}
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - командаРезультаты запуска класса:
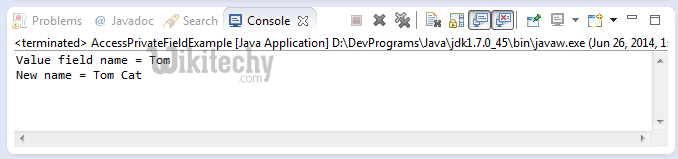
java - джава - учиться java - учебник java -
частный доступ
- примеры java - java-программы
- Следующий пример - доступ к приватному (private) методу.
AccessPrivateMethodExample.java
package com.wikitechy.tutorial.reflect.privateaccess;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import com.wikitechy.tutorial.beans.Cat;
public class AccessPrivateMethodExample {
public static void main(String[] args) throws NoSuchMethodException,
SecurityException, IllegalAccessException,
IllegalArgumentException, InvocationTargetException {
// Class object represent Cat class.
Class<Cat> aClass = Cat.class;
// Class.getMethod(String) get public method only.
// Use Class.getDeclaredMethod(String):
// Get the Method object of method declared in class.
Method private_setNameMethod = aClass.getDeclaredMethod("setName",
String.class);
// Allows for access to private method.
// Avoid IllegalAccessException
private_setNameMethod.setAccessible(true);
Cat tom = new Cat("Tom");
// Call private method
private_setNameMethod.invoke(tom, "Tom Cat");
System.out.println("New name = " + tom.getName());
}
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - командаРезультаты:

java - джава - учиться java - учебник java -
закрытый метод доступа
- примеры java - java-программы
10- Annotation
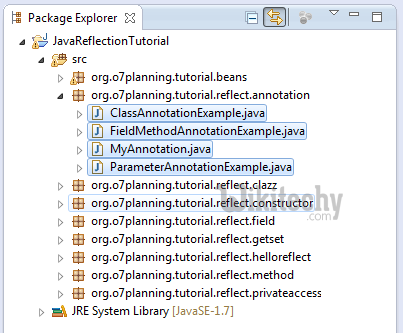
java - джава - учиться java - учебник java -
reflection
- примеры java - java-программы
MyAnnotation.java
package com.wikitechy.tutorial.reflect.annotation;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
// Annotation can be used at runtime.
@Retention(RetentionPolicy.RUNTIME)
// Use for class, interface, method, field, parameter.
@Target({ ElementType.TYPE, ElementType.METHOD, ElementType.FIELD,
ElementType.PARAMETER })
public @interface MyAnnotation {
String name();
String value() default "";
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - командаПример Annotation (Аннотации) с классом:
package com.wikitechy.tutorial.reflect.annotation;
import java.lang.annotation.Annotation;
@MyAnnotation(name = "Table", value = "Employee")
public class ClassAnnotationExample {
public static void main(String[] args) {
Class<?> aClass = ClassAnnotationExample.class;
// Get array of the Annotation of class
Annotation[] annotations = aClass.getAnnotations();
for (Annotation ann : annotations) {
System.out.println("Annotation: " + ann.annotationType().getSimpleName());
}
// Or More specific
Annotation ann = aClass.getAnnotation(MyAnnotation.class);
MyAnnotation myAnn = (MyAnnotation) ann;
System.out.println("Name = " + myAnn.name());
System.out.println("Value = " + myAnn.value());
}
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - командаРезультаты:
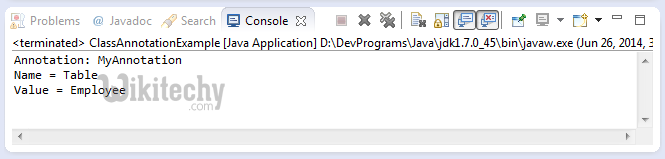
java - джава - учиться java - учебник java -
класс заметки
- примеры java - java-программы
FieldMethodAnnotationExample.java
package com.wikitechy.tutorial.reflect.annotation;
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
public class FieldMethodAnnotationExample {
@MyAnnotation(name = "My Field")
private int myField;
@MyAnnotation(name = "My Method", value = "My Method Value")
protected void myMethod(String str) {
}
public static void main(String[] args) throws NoSuchFieldException,
SecurityException, NoSuchMethodException {
Class<?> aClass = FieldMethodAnnotationExample.class;
//
System.out.println(" == FIELD == ");
Field field = aClass.getDeclaredField("myField");
// Get array of Annotation of field
Annotation[] fieldAnns = field.getAnnotations();
for (Annotation methodAnn : fieldAnns) {
System.out.println("Annotation: "
+ methodAnn.annotationType().getSimpleName());
}
// Or more specific
Annotation fieldAnn = field.getAnnotation(MyAnnotation.class);
MyAnnotation myAnn1 = (MyAnnotation) fieldAnn;
System.out.println("Name = " + myAnn1.name());
System.out.println("Value = " + myAnn1.value());
// Similar for method ...
System.out.println(" == METHOD == ");
Method method = aClass.getDeclaredMethod("myMethod", String.class);
// Get array of Annotation of method
Annotation[] methodAnns = method.getAnnotations();
for (Annotation methodAnn : methodAnns) {
System.out.println("Annotation: "
+ methodAnn.annotationType().getSimpleName());
}
// For more specific
Annotation methodAnn = method.getAnnotation(MyAnnotation.class);
MyAnnotation myAnn2 = (MyAnnotation) methodAnn;
System.out.println("Name = " + myAnn2.name());
System.out.println("Value = " + myAnn2.value());
}
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - командаРезультаты запуска:
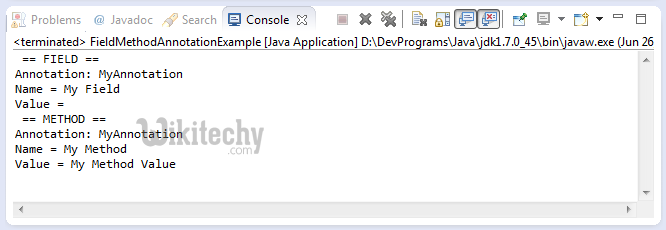
java - джава - учиться java - учебник java -
Аннотация поле метод
- примеры java - java-программы
- Пример Annotation с параметром метода:
package com.wikitechy.tutorial.reflect.annotation;
import java.lang.annotation.Annotation;
import java.lang.reflect.Method;
public class ParameterAnnotationExample {
// For example, a method with annotations in parameters.
protected void doSomething(int jobType,
@MyAnnotation(name = "Table", value = "Employee") String info) {
}
public static void main(String[] args) throws NoSuchMethodException,
SecurityException {
Class<?> aClass = ParameterAnnotationExample.class;
// Get Method object of doSomething(int,String) method.
Method method = aClass.getDeclaredMethod("doSomething", int.class,
String.class);
// Get parameters list of method
Class<?>[] parameterTypes = method.getParameterTypes();
for (Class<?> parameterType : parameterTypes) {
System.out.println("Parametete Type: "
+ parameterType.getSimpleName());
}
System.out.println(" ---- ");
// Returns an array of arrays of Annotations that
// represent the annotations on the formal parameters
Annotation[][] annotationss = method.getParameterAnnotations();
// Get Annotation list of parameter index 1.
Annotation[] annotations = annotationss[1];
for (Annotation ann : annotations) {
System.out.println("Annotation: "
+ ann.annotationType().getSimpleName());
}
}
}
нажмите кнопку ниже, чтобы скопировать код. - от - java tutorials - команда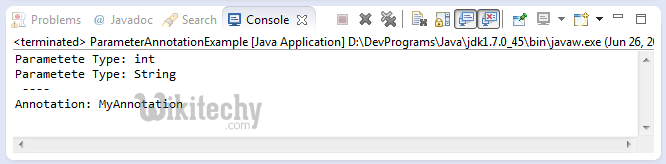