Ruby on Rails - Anatomy of Ruby Variables - ruby on rails tutorial - rails guides - rails tutorial - ruby rails
What is Variables in Ruby on Rails?
- Variables are identifiers.
- They are storage points that hold known or unknown quantity of information referred to as a value.
- The variable's name should denote the information the variable contains.
- The operations on variables vary for different programming languages and the value they hold.
- In Ruby, for instance, if variable switches to store a boolean instead of an array, the possible operations would vary.
- Still, on variables, we can liken them to Buckets where information can be kept.
- The name of the variables can be seen as name tags placed in the bucket.
- When referring to one of the buckets, we use the name of the bucket, not the data stored in the bucket.
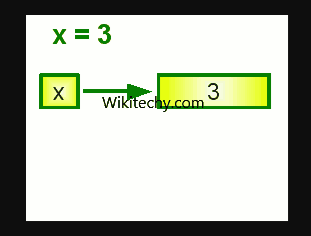
learn ruby on rails tutorial - ruby variables process - ruby on rails example
Scopes of Variables
- There are generally, two scopes of variables. They are:
- Local Variables
- Global Variables
1. Local Variables
- These are variables that are assigned within a method.
- They can be used only within the method in which they have been declared.
def counter
count = 0
while count <= 10
p count
count += 1
end
end
count #=> 0 1 2 3 4 5 6 7 8 9 10
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
- The example above is a Ruby method I named counter.
- A local variable count was declared in this method and it was used to print 0..10.
- However, if you call countoutside this method, it would throw an undefined variable or method error.
- It is important to note that arguments passed into a method or function, are also treated as local variables.
- For example, the code snippet below works exactly as the one above.
def counter(count)
while count <= 10
p count
count += 1
end
end
counter(0); #=>0 1 2 3 4 5 6 7 8 9 10
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
2. Global Variables
- These are variables that can be used by two or more methods.
- These variables are declared outside the methods and they can be manipulated within methods.
- Global variables in Ruby are denoted with the $ symbol.
- The example below shows how global variables are declared and used in Ruby.
$count = 0
def counter
while $count <= 10
p $count
$count += 1
end
end
counter #=>0 1 2 3 4 5 6 7 8 9 10
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
- · You will notice that count was declared outside the method, and it was incremented within the method.
- Also, we didn't need to pass count as an argument when calling the method.
Variables in Ruby
- Based on these two scopes of variables, Ruby has different types of variables. They are:
- Instance Variables
- Class Variables
- Class Instance Variables
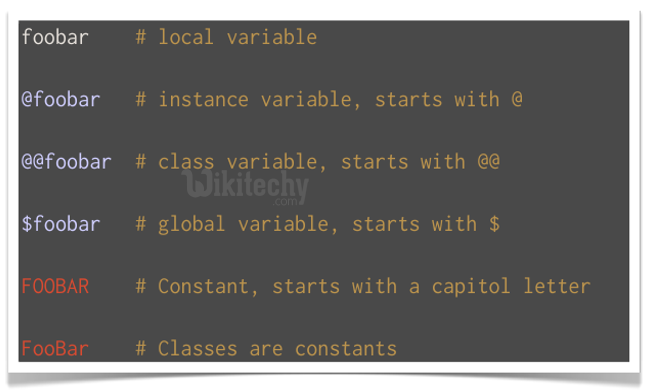
ruby on rails tutorial tags - ruby , rail , ruby on rails , rail forum , ruby on rails tutorial , ruby tutorial , rails guides , rails tutorial , learn ruby
1. Instance Variables
- Instance Variables are global variables that are ONLY used in Instance Methods within a class.
- Instance variables in Ruby start with a single @ sign.
- Any variable declared starting with a single @ sign can be manipulated within instance methods in Ruby.
- The example below shows an instance variable than has been used in an instance method.
class User
def initialize
@name = "John"
end
def greet
"Hello #{@name}"
end
end
User.new.greet #=> “Hello John”
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
- The above example has instance variable @name which holds a name John.
- The instance method greet adds Hello to the value of @name.
- This shows that instance variables act as global variables within a class.
2. Class Variables
- Class variables work exactly like instance variables.
- These variables are denoted with a double @ sign, i.e @@.
- These variables are used in both Instance Methods and Class Methods within a class.
- They can be used anywhere within the class or its children.
- They are the closest to the regular global variable in Ruby.
- For example, if we were to rewrite the above example as a class method, the method greet will be written as self.greet.
- Class methods, however, can be called directly on the class they belong to.
- The example below shows how a class variable has been used in a class method.
class User
@@name = “John”
def self.greet
"Hello #{@@name}"
end
end
User.greet #=> "Hello John"
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
3. Class Instance Variables
- These variables are a combination of Instance variables and class variables.
- This means they are declared in a class method like instance variables, but they can be used in any other class methods within the same class and other classes that inherit from that class.
- The example below shows how class instance variables are used.
class Person
def self.username(name)
@name = name
end
def self.greet
"Hello #{@name}"
end
end
Person.username("John") #=> "John"
Person.greet #=> "Hello John"
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
- In the snippet above, name method was used to get the person's name and it was assigned to @name variable.
- This variable, however, can be used in any other class method within that class.
- One important thing to know is that these kind of variables will not be available in classes that inherit from the class in which they are declared.
class Person
@name = "John"
def self.greet
"Hello #{@name}"
end
end
Person.greet #=> "Hello John"
class Student < Person
end
Student.greet #=> "Hello "
class Student < Person
@name = "James"
end
Student.greet #=> "Hello James"
Clicking "Copy Code" button will copy the code into the clipboard - memory. Please paste(Ctrl+V) it in your destination. The code will get pasted. Happy coding from Wikitechy - ruby on rails tutorial - rails guides - ruby rails - rubyonrails - learn ruby on rails - team
- From the example above, @name was assigned to "John" in class Person and calling Person.greet returned "Hello John"
- But that was not the case in class Student. class Student inherits everything from class Person except the value of @name.
- In other to use this variable in this new class, the variable has to be declared again.
What makes these variables different from each other?
- The major differences between these variables wrap around the scope at which they are to be used and the syntax for assigning them.
- The difference between an instance variable and a class instance variable is the scope at which they are used.
- Instance Variables are scoped to Instance Methods while Class Instance Variable are scoped to Class Methods.
- The difference between a Class Instance Variable and a Class Variable is the syntax of assigning them and their behavior could also count.
- Class instance Variables are assigned with a single @ symbol while Class Variables are assigned with double @ symbol, i.e @@
Conclusion
- Variables generally work almost the same way across all computer programming languages.
- What differentiates them is the way they are used, how they are been assigned, and the syntax used to declare them.