Flask HTTP methods
Flask HTTP methods
HTTP(Hyper Text Transfer Protocol) is used to data transfer in the world wide web. Flask framework provides various HTTP methods for data communication.
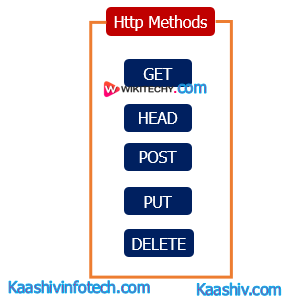
HTTP Methods
Method | Description |
---|---|
GET | It is used to send data in the unencrypted form to the server. |
HEAD | It is similar to the GET but used without the response body. |
POST | It is used to send the form data to the server. |
PUT | It is used to replace all the current representation of the target resource with the uploaded content. |
DELETE | It is used to delete all the current representation of the target resource specified in the URL. |
POST Method
To handle the POST requests at the server, first create a form to get some data at the client side from the user, and we will try to access this data on the server by using the POST request.
login.html
<html>
<body>
<form action = "http://localhost:5000/login" method = "post">
<table>
<tr><td>Name</td>
<td><input type ="text" name ="uname"></td></tr>
<tr><td>Password</td>
<td><input type ="password" name ="pass"></td></tr>
<tr><td><input type = "submit"></td></tr>
</table>
</form>
</body>
</html>
post_ex.py
from flask import *
app = Flask(__name__)
@app.route('/login',methods = ['POST'])
def login():
uname=request.form['uname']
passwrd=request.form['pass']
if uname=="wikitechy" and passwrd=="1234":
return "hello, %s" %uname
if __name__ == '__main__':
app.run(debug = True)
Output
- Now, open the HTML file, login.html on the web browser as shown in the following result.
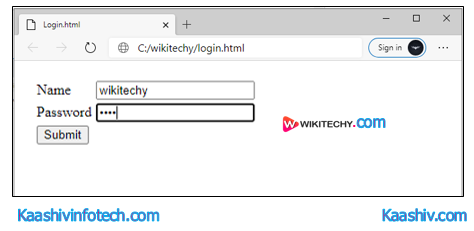
HTTP Login
- Now give the required input and click Submit, we will get the following result.
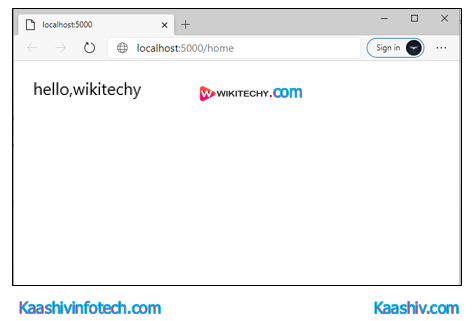
Login Form Output
GET Method
In GET Method, there are some changes in the data retrieval syntax on the server side.
login.html
<html>
<body>
<form action = "http://localhost:5000/login" method = "get">
<table>
<tr><td>Name</td>
<td><input type ="text" name ="uname"></td></tr>
<tr><td>Password</td>
<td><input type ="password" name ="pass"></td></tr>
<tr><td><input type = "submit"></td></tr>
</table>
</form>
</body>
</html>
Get_ex.py
from flask import *
app = Flask(__name__)
@app.route('/login',methods = ['GET'])
def login():
uname=request.args.get('uname')
passwrd=request.args.get('pass')
if uname=="wikitechy" and passwrd=="1234":
return "hello,%s" %uname
if __name__ == '__main__':
app.run(debug = True)
Output
- Now, open the HTML file, login.html on the web browser as shown in the following result.
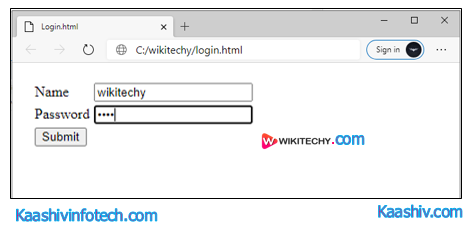
Login Form
- Now, click the submit button.
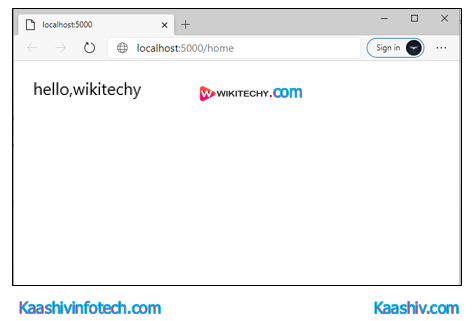
Login Form Output
As we can check the result. The data sent using the get() method is retrieved on the development server.
The data is obtained by using this code.
uname = request.args.get('uname')
.
Here, the args is a dictionary object which contains the list of pairs of form parameter and its respective value.
In the above image, Additionaly we can check the URL which contains the data sent with the request to the server. This is the main difference between the GET requests and the POST requests as the data sent to the server is not shown in the URL on the browser in the POST requests.
If you want to learn about Python Course , you can refer the following links Python Training in Chennai , Machine Learning Training in Chennai , Data Science Training in Chennai , Artificial Intelligence Training in Chennai