golang tutorial - Structures In Golang | Golang Structures - golang - go programming language - google go - go language - go program - google language
Structures In Golang
- Structure is a collection of variables of different types under a single name. For example: You want to store some information about a person:
- his/her name,
- citizenship number and
- salary.
- You can easily create different variables name, citNo, salary to store these information separately.
- In the future, you would want to store information about multiple persons. Now, you'd need to create different variables for each information per person: name1, cityNo1, salary1, name2, citNo2, salary2
- A better approach will be to have a collection of all related information under a single name Person, and use it for every person. Now, the code looks much cleaner, readable and efficient as well.
- This collection of all related information under a single name Person is a structure.
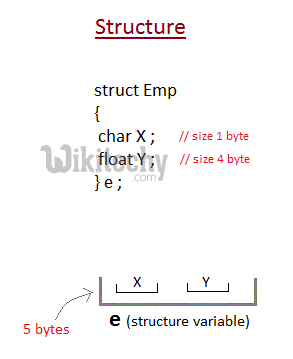
A sample example golang structure
Example structure program in C
struct person
{
char name[50];
int cityNo;
float salary;
};
int main()
{
struct person person1, person2;
return 0;
}
click below button to copy the code. By - golang tutorial - team
- Person1 and person2 are individual structure objects created for the main structure person.
Another way of creating a structure variable is:
struct person
{
char name[50];
int cityNo;
float salary;
} person1, person2;
click below button to copy the code. By - golang tutorial - team
Defining a Structure in go language programming :
- To define a structure, you must use type and struct statements.
- The struct statement defines a new data type, with more than one member for your program.
- type statement binds a name with the type which is struct in our case.
Format of the structure :
type struct_variable_type struct {
member definition;
member definition;
...
member definition;
}
click below button to copy the code. By - golang tutorial - team
- After defining the structure type, it can be used to declare variables of that type using the below syntax.
variable_name := structure_variable_type {value1, value2...value-n}
click below button to copy the code. By - golang tutorial - team
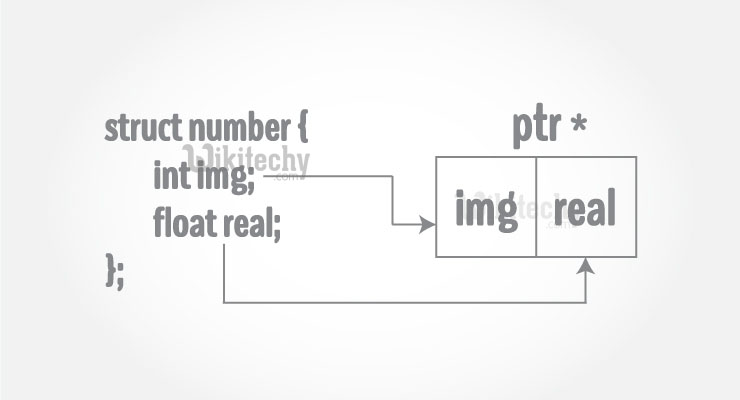
A sample example golang structure
Accessing Structure Members
- To access any member of a structure, we use the member access operator (.).
- Member access operator is coded as a period between the structure variable name and the structure member that we wish to access. (person1.age , person1.name)
- Use struct keyword to define variables of structure type.
Example to explain usage of structure:
package main
import "fmt"
type Persons_struct struct {
BookNamestring
Name string
LearningDetails string
Person__id int
}
func main() {
var Person_1 Persons_struct /* Declare Person_1 of type Person_ */
var Person_2 Persons_struct /* Declare Person_2 of type Person_ */
/* Person_ 1 specification */
Person_1.BookName= "Go Programming"
Person_1.Name = "Mahesh Kumar"
Person_1.LearningDetails = "Go Programming Tutorial"
Person_1.Person__id = 112
/* Person_ 2 specification */
Person_2.BookName= "Telecom Billing"
Person_2.Name = "Zara Ali"
Person_2.LearningDetails = "Telecom Billing Tutorial"
Person_2.Person__id = 113
/* print Person_1 info */
fmt.Printf( "Person_ 1 BookName: %s\n", Person_1.title)
fmt.Printf( "Person_ 1 Name : %s\n", Person_1.Name)
fmt.Printf( "Person_ 1 LearningDetails : %s\n", Person_1.LearningDetails)
fmt.Printf( "Person_ 1 Person__id : %d\n", Person_1.Person__id)
/* print Person_2 info */
fmt.Printf( "Person_ 2 BookName: %s\n", Person_2.title)
fmt.Printf( "Person_ 2 Name : %s\n", Person_2.Name)
fmt.Printf( "Person_ 2 LearningDetails : %s\n", Person_2.LearningDetails)
fmt.Printf( "Person_ 2 Person__id : %d\n", Person_2.Person__id)
}
click below button to copy the code. By - golang tutorial - team
golang , gopro , google go , golang tutorial , google language , go language , go programming language
Output for the above go program
Person_ 1 BookName: Go Programming
Person_ 1 Name : Mahesh Kumar
Person_ 1 LearningDetails : Go Programming Tutorial
Person_ 1 Person__id : 112
Person_ 2 BookName: Telecom Billing
Person_ 2 Name : Zara Ali
Person_ 2 LearningDetails : Telecom Billing Tutorial
Person_ 2 Person__id : 113
Structures as Function Arguments
- Structure can be passed as a function argument in very similar way as you pass variable or pointer.
package main
import "fmt"
type Persons_struct struct {
BookNamestring
Name string
LearningDetails string
Person_id int
}
func main() {
var Person_1 Persons_struct /* Declare Person_1 of type Person_ */
var Person_2 Persons_struct /* Declare Person_2 of type Person_ */
/* Person_ 1 specification */
Person_1.BookName= "Go Programming"
Person_1.Name = "Venkat"
Person_1.LearningDetails = "Go Programming Tutorial"
Person_1.Person_id = 112
/* Person_ 2 specification */
Person_2.BookName= "Go Lang Programming"
Person_2.Name = "Arun"
Person_2.LearningDetails = " Go Lang Programming Tutorial"
Person_2.Person_id = 113
/* print Person_1 info */
printPerson_(Person_1)
/* print Person_2 info */
printPerson_(Person_2)
}
func printPerson_( Person_ Persons_struct ) {
fmt.Printf( "Person_BookName: %s\n", Person_.title);
fmt.Printf( "Person_Name : %s\n", Person_.Name);
fmt.Printf( "Person_LearningDetails : %s\n", Person_.LearningDetails);
fmt.Printf( "Person_Person__id : %d\n", Person_.Person__id);
}
click below button to copy the code. By - golang tutorial - team
golang , gopro , google go , golang tutorial , google language , go language , go programming language
Output for the above go program
Person_BookName: Go Programming
Person_Name: Venkat
Person_LearningDetails : Go Programming Tutorial
Person_Person_id : 112
Person_BookName: Go Lang Programming
Person_Name: Arun
Person_LearningDetails : Go Lang Programming Tutorial
Person_Person_id : 113
Pointers to Structures
- pointers to structures declaration is very similar way as you define pointer to any other variable as below,
var struct_pointer *Persons_struct
click below button to copy the code. By - golang tutorial - team
- Store the address of a structure variable in the above defined pointer variable.
- To get the address of a structure variable, place the & operator before the structure's name as below,
struct_pointer = &Person_1;
click below button to copy the code. By - golang tutorial - team
- To access the members of a structure using a pointer to that structure, you must use the "." operator as follows:
struct_pointer.title;
click below button to copy the code. By - golang tutorial - team
Program using structure pointer
package main
import "fmt"
type Persons_struct struct {
BookName string
Name string
LearningDetails string
Person_id int
}
func main() {
var Person_1 Persons_struct /* Declare Person_1 of type Person_ */
var Person_2 Persons_struct /* Declare Person_2 of type Person_ */
/* Person_ 1 specification */
Person_1.BookName= "Go Programming"
Person_1.Name = "Venkat"
Person_1.LearningDetails = "Go Programming Tutorial"
Person_1.Person_id = 112
/* Person_ 2 specification */
Person_2.BookName= " Go Lang Programming "
Person_2.Name = "Arun"
Person_2.LearningDetails = " Go Lang Programming Tutorial"
Person_2.Person_id = 113
/* print Person_1 info */
printPerson_(&Person_1)
/* print Person_2 info */
printPerson_(&Person_2)
}
func printPerson_( Person_ *Persons_struct ) {
fmt.Printf( "Person_BookName : %s\n", Person_.title);
fmt.Printf( "Person_Name : %s\n", Person_.Name);
fmt.Printf( "Person_LearningDetails : %s\n", Person_.LearningDetails);
fmt.Printf( "Person_Person__id : %d\n", Person_.Person__id);
}
click below button to copy the code. By - golang tutorial - team
golang , gopro , google go , golang tutorial , google language , go language , go programming language
Output for the above go program
Person_BookName: Go Programming
Person_Name : Venkat
Person_LearningDetails : Go Programming Tutorial
Person_Person_id : 112
Person_BookName: Go Lang Programming
Person_Name : Arun
Person_LearningDetails : Go Lang Programming Tutorial
Person_Person_id : 113