Headless Selenium Browsers - Selenium Testing Tutorial
Headless Browser
- A web browser without user interface is known as Headless browser.
- Webpages are actually accessed in headless browser but without the GUI which is hidden from the browser.
- It is similar to other browsers the only difference is we cannot see anything on the screen.
- Here program runs on the background and anything cannot be seen on the screen.
- Like other browsers it clicks on links, navigating pages, downloading the documents etc are performed by headless browsers.
- In a normal browser each step of the program execution will be presented with a GUI representation but in a headless browser all the steps are carried out sequentially and correctly and we can keep track of it with the help of command prompt.
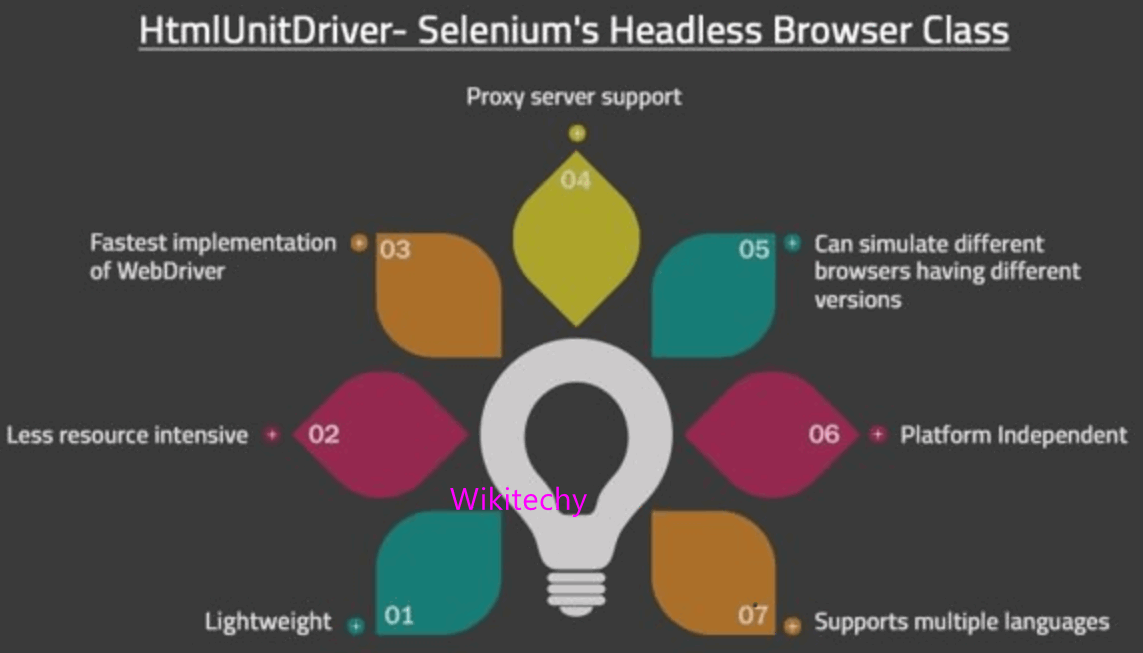
Advantages Of Headless Browser
- When machine has no GUI headless browsers are used and is executed via command line interface.
- This browser can be used when we dont need to view anything and purpose is to ensure that all the tests are getting executed line by line.
- UI based browsers consume lot of memory, hence when running parallel test, headless browsers can be used.
- Performing regression testing with continous integration and when cross browser testing is finished, we can used headless browser testing.
- If we want to execute multiple browsers on a single machine or run test cases just for creation of data, we use headless browser.
- Headless browsers are really faster when compared with real browsers, hence can be used for faster execution.
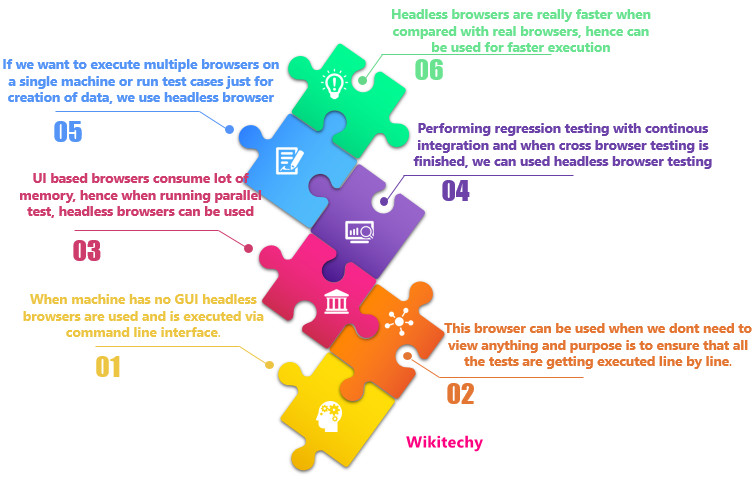
Disadvantages Of Headless Browser
- Though headless browsers are very fast, there are some disadvantges also.
- Since headless browsers are very fast, it is difficult to debug the issues.
- Real browser testing includes executing the test cases in the presence of GUI.
- Since these tests are performed in front of the user, user can interact with the team and where ever changes and correction are required. We cannot use headless browser in this case.
- Screenshots for reporting errors cannot be done using headless browsers since they don`t have GUI.
- Using headless browser is a challenging task where lot of browser debugging is needed.
Examples Of Headless Browsers
- Html Unit Browsers
- Firefox
- Chrome
- PhantomJS
- Zombie.js
- TrifleJS
- SlimerJS
- Splash
- SimpleBrowser
- NodeJS
Headless Testing With Selenium
- Selenium is a free, open-source testing tool. It is a quite well-known and efficient automation tool for performing automation tests.
- Selenium allows us to write test scripts in various languages like Java, Python, C#, Ruby, Perl, Scala, etc. by supporting numerous browsers like Firefox, Chrome, Internet Explorer, Opera, Safari, etc. and is capable to run on Windows, Linux, and macOS.
- Selenium Webdriver provides good support to dynamic web pages, where various web elements change without the page itself being reloaded.
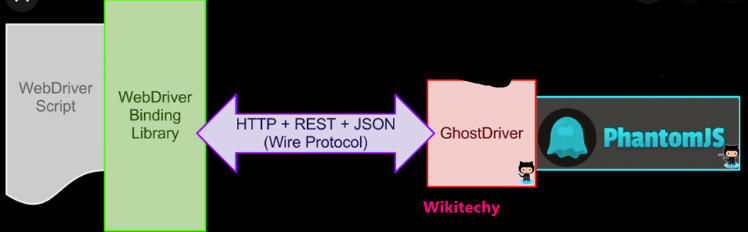
Headless Chrome And Firefox
- Both firefox and chrome browser support headless automation testing which can be implemented in the code in firefox and chrome without using GUI.
Headless Firefox Example
- For the firefox browser support for headless browser starts from version 56 and is available for windows, linux, and MacOs.
- We need to download the geckodriver.exe file of the latest version of Firefox and ensure that the version we will be using is greater than the minimum supported version. Firefox runs in headless mode via the headless() method.
Let’s see the code for Firefox Browser in Headless mode:
package headless_testing; import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; public class HeadlessFirefox { public static void main(String[] args) { // TODO Auto-generated method stub System.setProperty("webdriver.gecko.driver"," E://Selenium/latest firefox exe /geckodriver.exe"); FirefoxOptions options = new FirefoxOptions(); options.setHeadless(true); WebDriver driver = new FirefoxDriver(options); driver.get("www.google.com/"); System.out.println("Executing Firefox Driver in Headless mode..\n"); System.out.println(">> Page Title : "+driver.getTitle()); System.out.println(">> Page URL : "+driver.getCurrentUrl()); } }
- On executing the above code for Firefox Browser in Headless mode, the title of the Page and its URL are displayed. The code is executed in Headless mode and can be tracked on the Console.
Output

Read Also
Selenium Training in Chennai , Selenium Placement Training institutes in Chennai , best selenium training institute in chennaiHeadless Chrome Example
- For chrome headless browser version is supported from versions 60 onwards and is available for windows, linux, and MacOs.
- We need to download the latest .exe file for the latest version of chrome browser.
Below given is the syntax for using chrome as headless browser.
ChromeOptions options = new ChromeOptions(); options.addArguments(“--headless”); OR options.setHeadless(true);
Let’s see the code for Chrome Browser in Headless mode:
package headless_testing; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; public class HeadlessChrome { public static void main(String[] args) { // TODO Auto-generated method stub System.setProperty("webdriver.chrome.driver","E://Selenium/latest chrome exe /chromedriver.exe"); ChromeOptions options = new ChromeOptions(); options.addArguments("--headless"); WebDriver driver = new ChromeDriver(options); driver.get("www.google.com/"); System.out.println("Executing Chrome Driver in Headless mode..\n"); System.out.println(">> Page Title : "+driver.getTitle()); System.out.println(">> Page URL : "+driver.getCurrentUrl()); } }
- On executing the above code for the Chrome Browser in Headless mode, the title of the Page and its URL are displayed. The code is executed and the execution can be tracked on the Console.
Headless HtmlUnitDriver
- HtmlUnitDriver is a Headless web browser written in Java.
- As the name suggests, headless driver is based on Htmlunit. There is a built in headless browser in selenium which is called as HtmlUnitDriver. It is one of the most lightweight and fastet browser.
- Implementation of html unit driver can be done by downloading the html unit jar files from selenium official website.
HtmlUnitDriver In Headless Mode
- Just like all the other browsers, for the HtmlUnitDriver as well, we need to create an object for the class to run the code in headless mode.
package headless_testing; import org.openqa.selenium.WebDriver; import org.openqa.selenium.htmlunit.HtmlUnitDriver; public class HtmUnitDriver { public static void main(String[] args) { // TODO Auto-generated method stub WebDriver driver = new HtmlUnitDriver(); driver.get("https://www.google.com/"); System.out.println("Executing HtmlUnitDriver in Headless mode..\n"); System.out.println(">> Page Title : "+ driver.getTitle()); System.out.println(">> Page URL : "+ driver.getCurrentUrl()); } }
- Thus on executing the above code for HtmlUnitDriver in Headless mode, the Output received displays the Title of the Page and its URL. The Output is received through the Console where all the functions performed in the program can be viewed in a stepwise manner.
Output
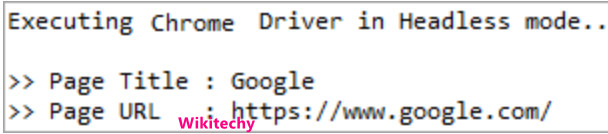
Features/Advantages Of HtmlUnitDriver
- Supports http and https protocols
- Provides support for java script
- Multitasking is possible, which means we can run multiple tests.
- Supports cookies and proxy servers.
- Speed and performance of test scripts can be improved as this is the fastest browser.
- HtmlUnitDriver is platform-independent.
- As it is Headless by default, it supports Headless Testing.
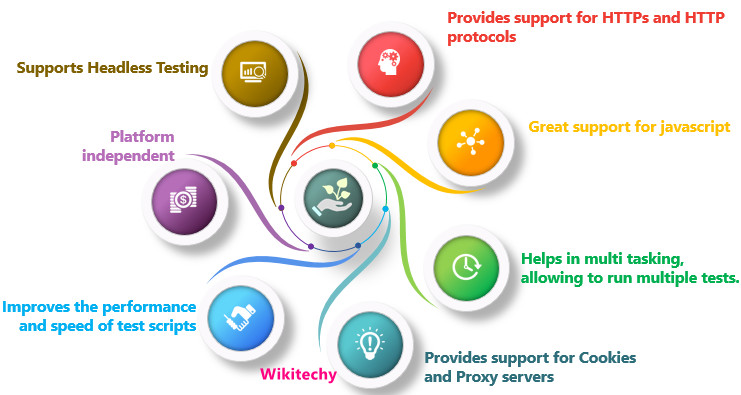
Disadvantages Of HtmlUnitDriver
- We cannot use html unit driver for complex websites.
- When compared with the real browser testing, debugging the script will be very difficult using the html unit driver.
- We cannot take screen shots using htmlunit driver.
- Headless Browsers emulate other browsers.
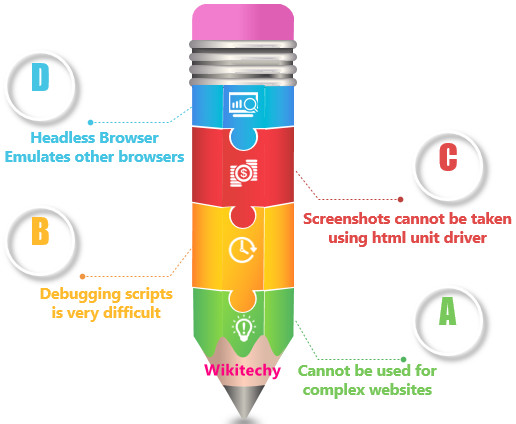