java tutorial - How to connect access database in java - java programming - learn java - java basics - java for beginners
Connect access database in java
- There are two methods we are using to connect java application with the access database.
- Without DSN (Data Source Name)
- With DSN
- Java used with Oracle, mysql, or DB2 database.
Sample Code
Connect Java Application with access without DSN
- Below example, describe to connect the java program with the access database.
- Step 1: First your create a database "db.mdb" on d:sample folder
- Step 2: Create employee table as below -
- Table Name - wikitechy_employee
- emp_id (pK) Text,
- emp_name Text,
- emp_city Text,
- emp_salary number
- and insert a record emp_id-"w01278", emp_name-"Shym Andrews", emp_city- "US", emp_salary-75000
Sample Code
package pkg1;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.logging.Logger;
public class App1 {
public Connection setupConnection()
{
try
{
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
Connection con = DriverManager.getConnection("jdbc:odbc:Driver=
{Microsoft Access Driver (*.mdb)};Dbq=d:sampledb1.mdb");
return con;
catch (Exception e) {
e.printStackTrace();
}
}
public void dispEmp(){
try
{
Connection con=setupConnection();
Statement st=con.createStatement();
ResultSet res=st.executeQuery("select * from wikitechy_employee");
while(res.next())
{
System.out.println(res.getString(1)+" "+res.getString(2)+" "+res.getString(3)+" "+res.getString(4));con.close();
}
catch (Exception e) {
System.out.println("error in fetching data");
}
}
public static void main(String[] args)
{
new App1().dispEmp();
}
}
click below button to copy the code. By - java tutorial - team
Connect Java Application with access with DSN
- To connect a Java application with Access database using JDBC-ODBC Bridge (type-1) Driver. We need to follow the below steps are,
- Go to control panel
- Go to Administrative tools
- Select Data Source (ODBC)
- Add new DSN name, select add
- Select Access driver from list, then click on finish
- Give a DSN name, click ok
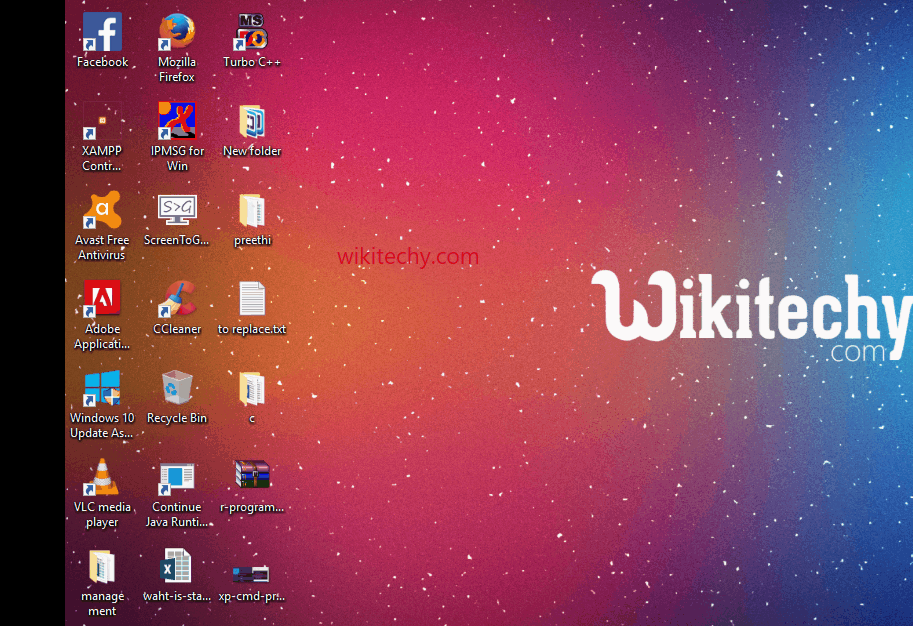
Learn Java - Java tutorial - Create DSN Name - Java examples - Java programs
Sample Code
import java.sql.*;
class Test
{
public static void main(String []args)
{
try{
Class.forName("sun.jdbc.odbc.JdbcOdbcDriver");
Connection con = DriverManager.getConnection("jdbc:odbc:Test", "", "");
Statement s=con.createStatement(); //creating statement
ResultSet rs=s.executeQuery("select * from wikitechy_employee"); //executing statement
while(rs.next()){
System.out.println(rs.getInt(1)+" "+rs.getString(2));
}
con.close(); //closing connection
}
catch(Exception e)
{
e.printStackTrace();
}
}
}