java tutorial - Java Math.pow() Method - java programming - learn java- java basics - java for beginners
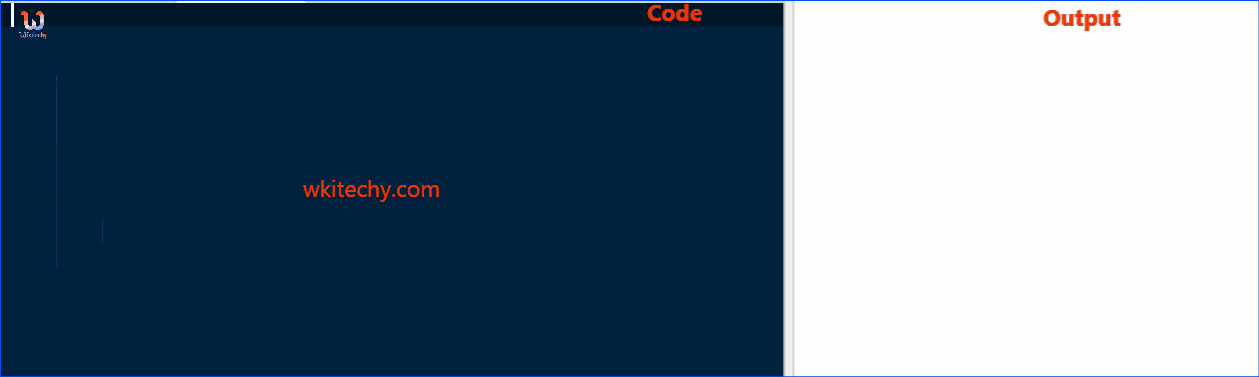
Learn Java - Java tutorial - Java numbers pow - Java examples - Java programs
Description
The method Math.pow() - raises the value of the first argument to the power of the second argument, thereby allowing a quick raising to the power of any values
Syntax
double pow(double base, double exponent)
click below button to copy the code. By - java tutorial - team
Options
Detailed information about the parameters:
- base is any primitive data type.
- exponent is any primitive data type.
Return value:
- In Java, Math.pow() returns a double value of the first argument, raised to the power of the second argument.
Example 1: squaring and cube
- To raise any number to a square using the Math.pow() method, use the value 2 for the second argument, and 3 for the cube, and so on. Note that "% .0f" is used to display the integer value, since the method returns a double value.
public class Test {
public static void main (String args []) {
// Squaring number 3
int a1 = 3;
int b1 = 2;
System.out.printf ("The number 3 in the square is equal to% .0f \ n", Math.pow (a1, b1));
// Squaring the number 5
int a2 = 5;
int b2 = 2;
System.out.println ("The number 5 in the square equals" + Math.pow (a2, b2));
// Cube the number 2
int a3 = 2;
int b3 = 3;
System.out.printf ("The number 2 in the cube is% .0f \ n", Math.pow (a3, b3));
// Cube the number 3
int a4 = 3;
int b4 = 3;
System.out.println ("The number 3 in the cube is equal to" + Math.pow (a4, b4));
}
}
click below button to copy the code. By - java tutorial - team
Output
The number 3 in the square is 9
The number 5 is squared equal to 25.0
The number 2 in the cube is equal to 8
The number 3 in the cube is 27.0
Example 2: raising a number to a fractional power
public class Test {
public static void main (String args []) {
double x1 = 10.635;
double y1 = 3.76;
System.out.printf ("The value of% .3f in the degree of% .2f is equal to% .3f \ n", x1, y1, Math.pow (x1, y1));
System.out.printf ("pow (% .3f,% .3f) =% .3f \ n \ n", x1, y1, Math.pow (x1, y1));
int x2 = 2;
double y2 = 3.76;
System.out.printf ("The value of 2 in the degree of% .2f is equal to% .3f \ n", y2, Math.pow (x2, y2));
System.out.printf ("pow (2,% .3f) =% .3f", y2, Math.pow (x2, y2));
}
}
click below button to copy the code. By - java tutorial - team
Output
The value of 10.635 in the power of 3.76 is 7253.256
pow (10,635, 3,760) = 7,253,256
The value of 2 in the power of 3.76 is 13.548
pow (2, 3,760) = 13,548
Example 3: raising a number to a negative power
public class Test {
public static void main (String args []) {
// Raise a number to a negative fractional power
double x = 7.525;
double y = -1.49;
System.out.printf ("The value of% .3f in the degree of% .2f is equal to% .3f \ n", x, y, Math.pow (x, y));
System.out.printf ("pow (% .3f,% .3f) =% .3f \ n \ n", x, y, Math.pow (x, y));
// Raising a number to a negative degree
int a = 2;
int b = -2;
System.out.printf ("The value of 2 to the power of -2 is% .2f \ n", Math.pow (a, b));
System.out.printf ("pow (2, -2) =% .2f", Math.pow (a, b));
}
}
click below button to copy the code. By - java tutorial - team
Output
The value of 7.525 to the power of -1.49 is 0.049
pow (7.525, -1.490) = 0.049
The value 2 in the power of -2 is 0.25
pow(2, -2) = 0,25
Example 4: the erection of a negative number of degrees
- Note that when we raise a negative number to a fractional power, we do not get the result (NaN).
public class Test{
public static void main (String args []) {
// Raise a negative fractional number to the power of
double x1 = -7.525;
double y1 = 1.49;
System.out.printf ("The value of% .3f in the degree of% .2f is equal to% .3f \ n", x1, y1, Math.pow (x1, y1));
System.out.printf ("pow (% .3f,% .3f) =% .3f \ n \ n", x1, y1, Math.pow (x1, y1));
double x2 = -7.525;
double y2 = 2;
System.out.printf ("The value of% .3f in the degree of% .0f is equal to% .3f \ n", x2, y2, Math.pow (x2, y2));
System.out.printf ("pow (% .3f,% .0f) =% .3f \ n \ n", x2, y2, Math.pow (x2, y2));
double x3 = -7.525;
double y3 = 3;
System.out.printf ("The value of% .3f in the degree of% .0f is equal to% .3f \ n", x3, y3, Math.pow (x3, y3));
System.out.printf ("pow (% .3f,% .0f) =% .3f \ n \ n", x3, y3, Math.pow (x3, y3));
// Raising a negative number to the power of
int a1 = -2;
int b1 = 2;
System.out.printf ("The value of -2 in power 2 is% .0f \ n", Math.pow (a1, b1));
System.out.printf ("pow (-2, 2) =% .0f \ n \ n", Math.pow (a1, b1));
int a2 = -2;
int b2 = 3;
System.out.printf ("The value of -2 to the power of 3 is% .0f \ n", Math.pow (a2, b2));
System.out.printf ("pow (-2, 3) =% .0f", Math.pow (a2, b2));
}
}
click below button to copy the code. By - java tutorial - team
Output
The value of -7.525 to the power of 1.49 is NaN
pow (-7.525, 1.490) = NaN
The value of -7.525 in power 2 is 56.626
pow (-7.525, 2) = 56.626
The value of -7.525 in the power of 3 is -426,108
pow (-7.525, 3) = -426.108
The value of -2 in power 2 is 4
pow (-2, 2) = 4
The value of -2 to the power of 3 is -8
pow(-2, 3) = -8
Example 5: erecting each number in a square and displaying it on the screen
public class Test{
public static void main(String args[]){
int a = 1; // Start number
int b = 10; // Finite number
// Raise each number in square and output to the screen
for (int i = a; i <= b; i++){
System.out.printf("Value" + i + "in the square is%.0f \n", Math.pow(i, 2));
}
}
}
click below button to copy the code. By - java tutorial - team
Output
The value 1 in the square is 1
The value of 2 in the square is 4
The value of 3 in the square is 9
The value of 4 in the square is 16
The value of 5 in the square is 25
The value of 6 in the square is 36
The value of 7 in the square is 49
The value of 8 in the square is 64
The value of 9 in the square is 81
The value of 10 in the square is 100