java tutorial - String concatenation in Java - java programming - learn java - java basics - java for beginners
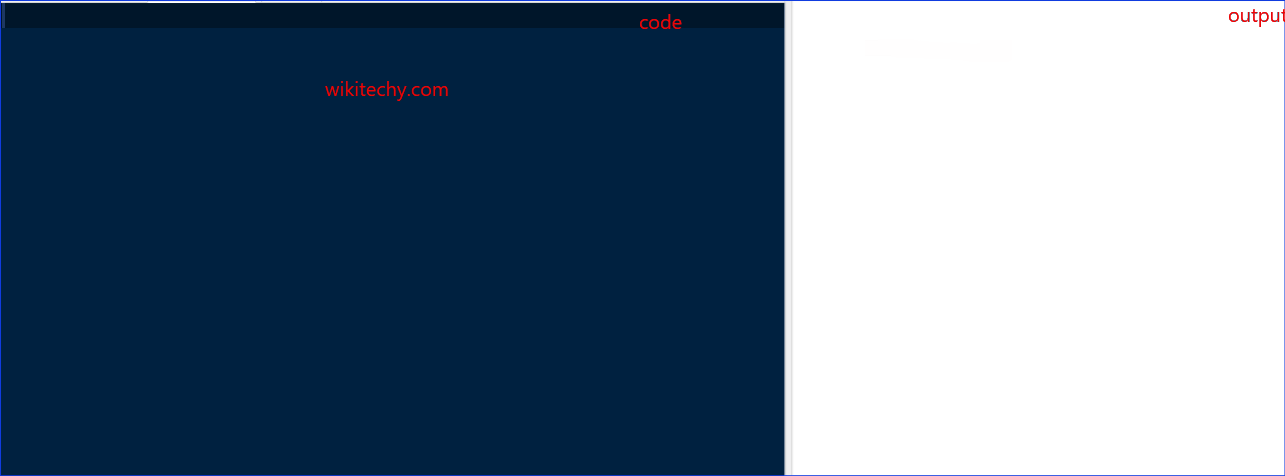
Learn java - java tutorial - How to perform string-concatenation - java examples - java programs
String concatenation:
- It is an operation of joining the character strings back-to-back.
- In this example, we will see how to concatenate Strings in Java.
- The String concatenation in Java can be done in numerous ways.
- Using + operator - while use + operator
string_1 + string_2
statement, then it will executed as, new StringBuffer().append(string_1 ).append(string_2) within it. - + operator is not recommended for huge number of String concatenation as the performance is not good.
- Using String.concat() method – concatenates the specified string to the end of this string.
- Using StringBuffer.append method - Appends the specified StringBuffer to this sequence.
sample code:
public class kasshiv_infotech
{
public static void main(String args[])
{
String string_1 = "Kaashiv";
String string_2 = "Infotech";
String string_3 = string_1 + string_2;
System.out.println("+ operator : " + string_3);
String string_4 = string_1.concat(string_2);
System.out.println("String concat method : " + string_4);
String string_5 = new StringBuffer().append(string_1).append(string_2).toString();
System.out.println("StringBuffer append method : " + string_5);
}
}
Output:
+ operator : KaashivInfotech
String concat method : KaashivInfotech
StringBuffer append method : KaashivInfotech