react - ReactJS Component Life Cycle - react js - reactjs
Lifecycle Methods
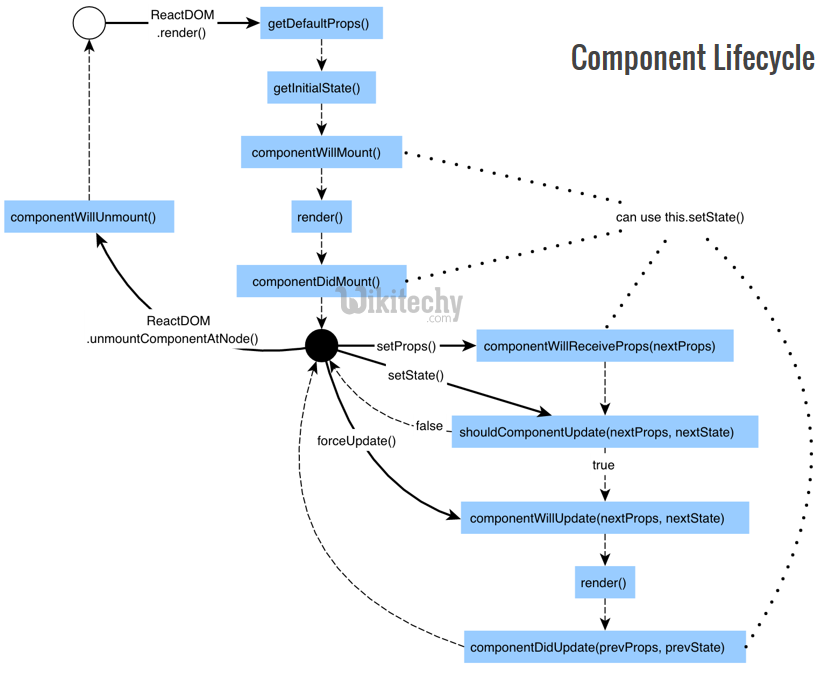
- The component Life cycle has different Phases:
- Mounting
- Updating
- Unmounting
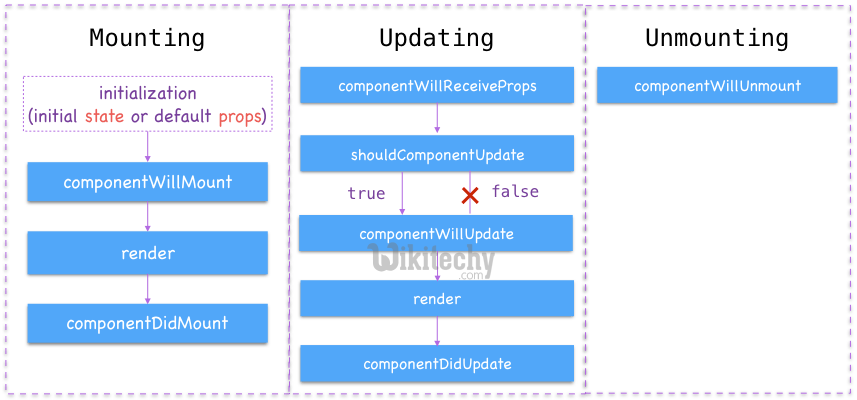
Mounting
- Mounting means putting elements into the DOM.
- React has four built-in methods that gets called, in this order, when mounting a component:
- constructor()
- getDerivedStateFromProps()
- render()
- componentDidMount()
constructor()
- constructor() method is called before anything else, when the component is initiated, and it is place to set up the original state and other initial values.
- The constructor() method is called with the props, as arguments, and you must always start by calling the super(props) before anything else, this will initiate the parent's constructor method and allows the component to receive methods from its parent (React.Component).
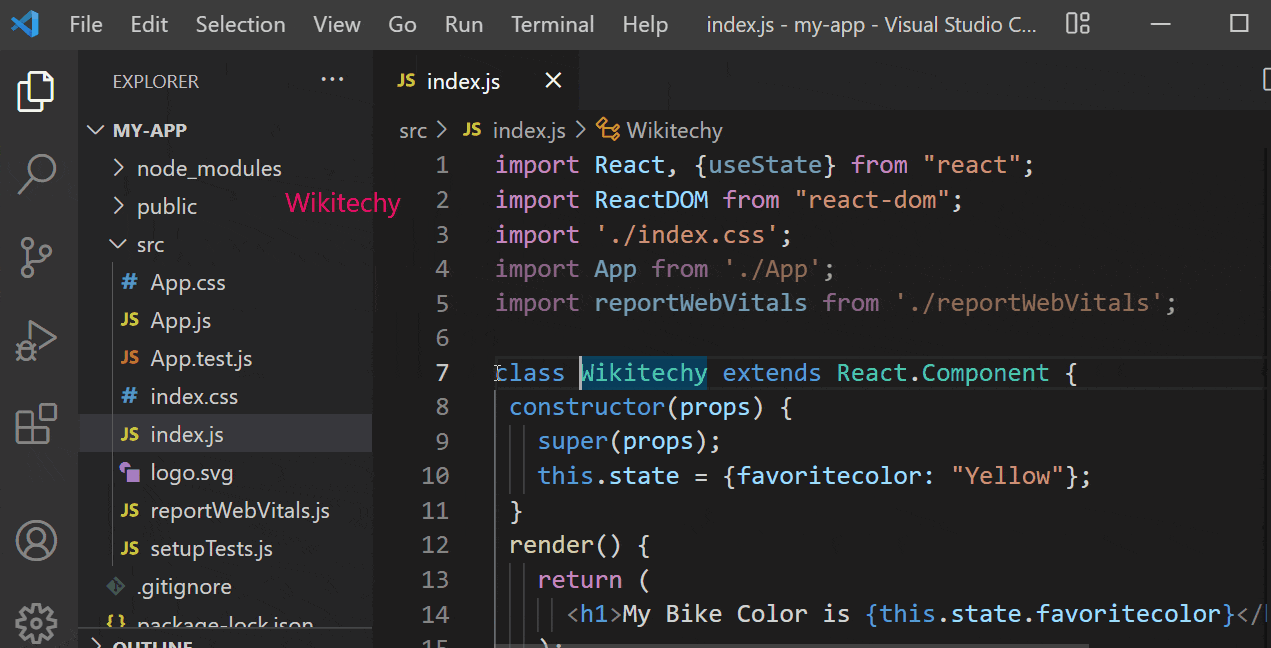
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Wikitechy extends React.Component {
constructor(props) {
super(props);
this.state = {favoritecolor: "Yellow"};
}
render() {
return (
<h1> My Bike Color is {this.state.favoritecolor}</h1>
);
}
}
ReactDOM.render(<Wikitechy />, document.getElementById('root'));
Output
My Bike Color is Yellow
getDerivedStateFromProps()
- The getDerivedStateFromProps() method is called right before rendering the element(s) in the DOM.
- This is the natural place to set the state object based on the initial props.
- It takes state as an argument, and returns an object with changes to the state.
- The example below starts with the favorite color being "green", but the getDerivedStateFromProps() method updates the favorite color based on the favcol attribute:
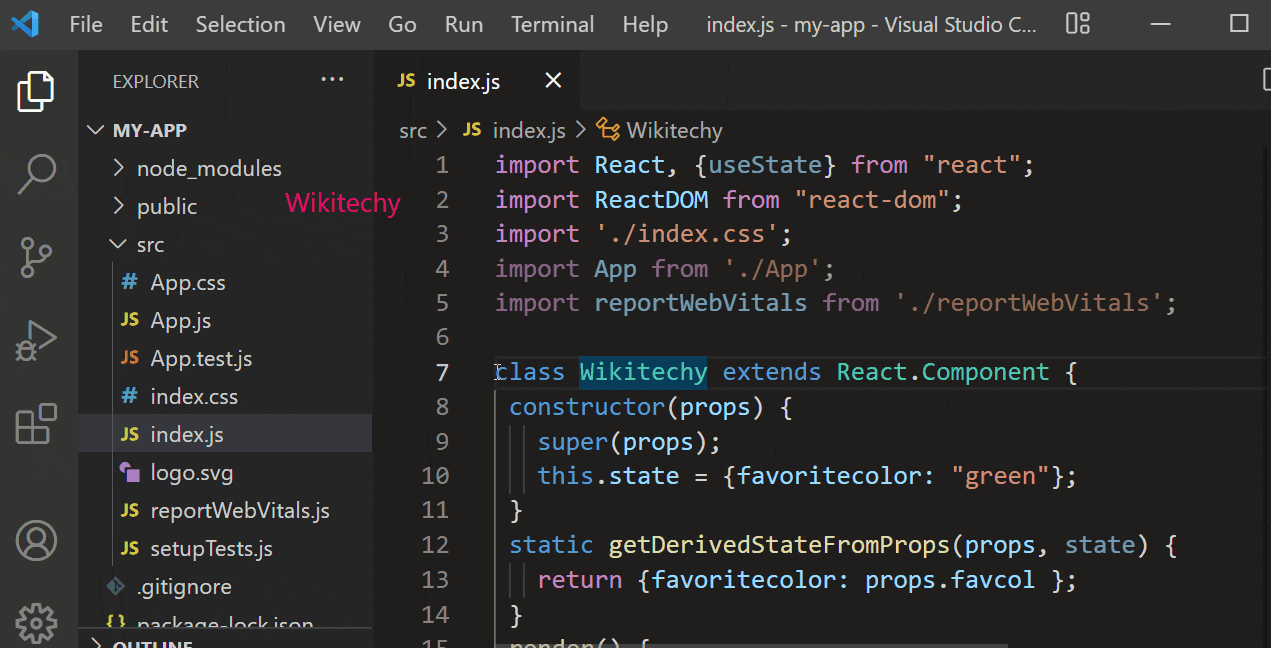
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Wikitechy extends React.Component {
constructor(props) {
super(props);
this.state = {favoritecolor: "green"};
}
static getDerivedStateFromProps(props, state) {
return {favoritecolor: props.favcol };
}
render() {
return (
<h1>My Car Color is {this.state.favoritecolor}</h1>
);
}
}
ReactDOM.render(<Wikitechy favcol="blue"/>, document.getElementById('root'));
Output
My Car Color is blue
render()
- The render() method is required, and is the method that really outputs the HTML to the DOM.
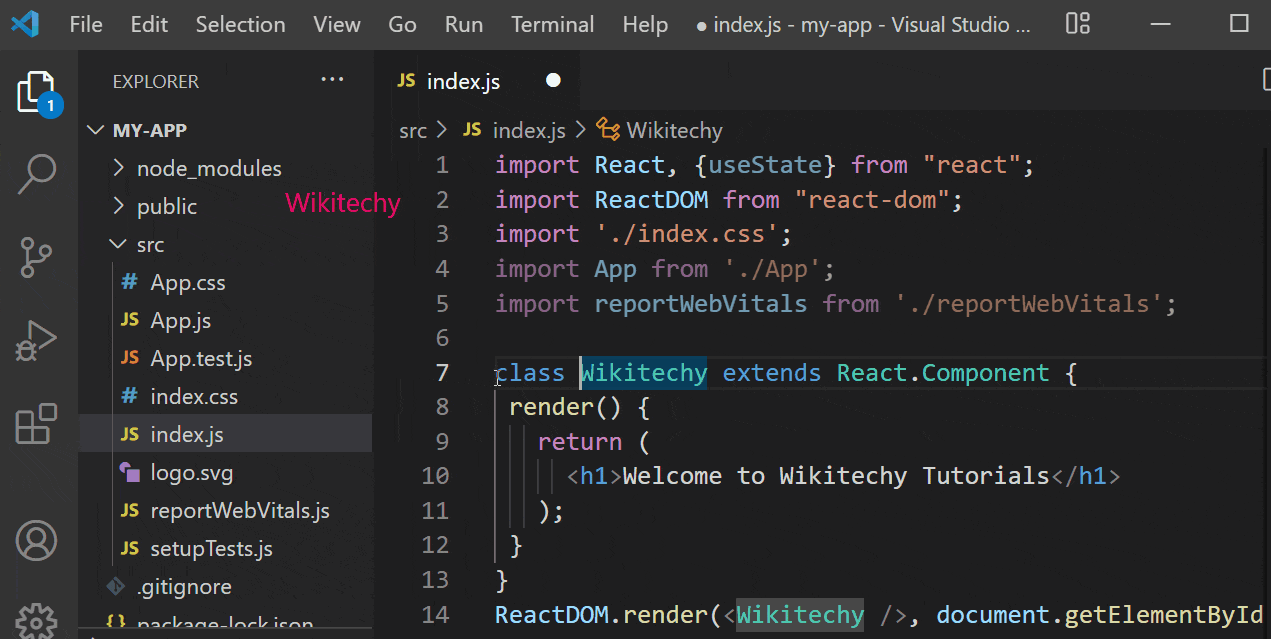
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Wikitechy extends React.Component {
render() {
return (
<h1>Welcome to Wikitechy Tutorials</h1>
);
}
}
ReactDOM.render(<Wikitechy />, document.getElementById('root'));
Output
Welcome to Wikitechy Tutorials
componentDidMount
- The componentDidMount() method is called after the component is rendered.
- This is where you run statements that requires that the component is already placed in the DOM.
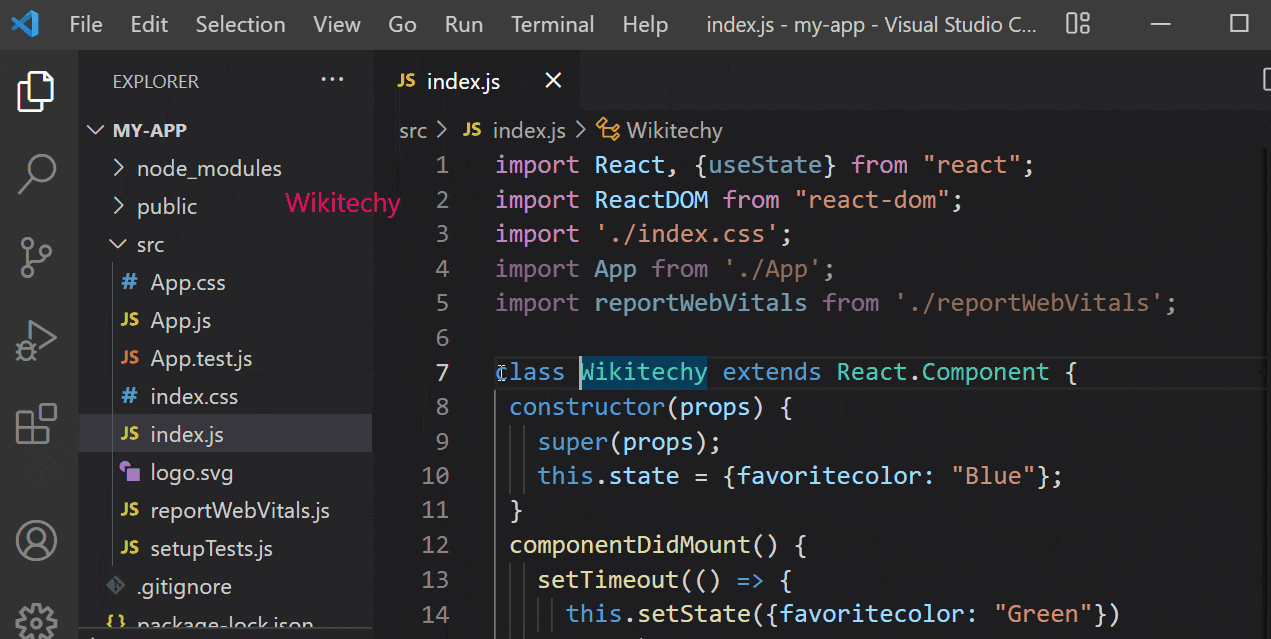
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Wikitechy extends React.Component {
constructor(props) {
super(props);
this.state = {favoritecolor: "Blue"};
}
componentDidMount() {
setTimeout(() => {
this.setState({favoritecolor: "Green"})
}, 1000)
}
render() {
return (
<h1>My Drees Color is {this.state.favoritecolor}</h1>
);
}
}
ReactDOM.render(<Wikitechy />, document.getElementById('root'));
Output
My Drees Color is Green
Updating
- The next stage in the lifecycle is when a component is updated.
- A component is updated whenever there is a change in the component's state or props.
- React has built-in methods that gets called, in this order, when a component is updated:
- getDerivedStateFromProps()
- shouldComponentUpdate()
- render()
- getSnapshotBeforeUpdate()
- componentDidUpdate()
- The render() method is required and will always be called, the others are optional and will be called if you define them.
getDerivedStateFromProps
- getDerivedStateFromProps is the first method that is called when a component gets updated.
- This is still the place to set the state object based on the initial props.
- The example below has a button that changes the course to java, but since the getDerivedStateFromProps() method is called, which updates the state with the course from the course attribute, the course is still rendered as react.
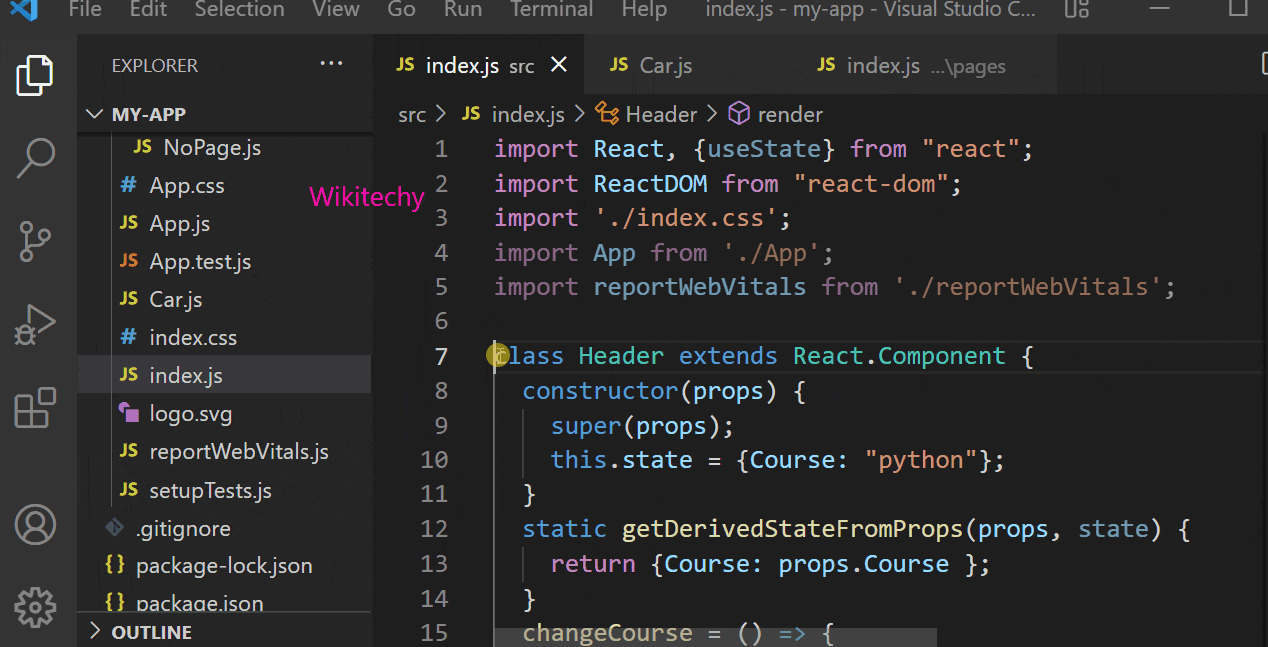
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Header extends React.Component {
constructor(props) {
super(props);
this.state = {Course: "python"};
}
static getDerivedStateFromProps(props, state) {
return {Course: props.Course };
}
changeCourse = () => {
this.setState({Course: "java"});
}
render() {
return (
<div>
<h1> Best Learning Course {this.state.Course}</h1>
<button type="button" onClick={this.changeCourse}>Change Course</button>
</div>
);
}
}
ReactDOM.render(<Header Course="react"/>, document.getElementById('root'));
Output
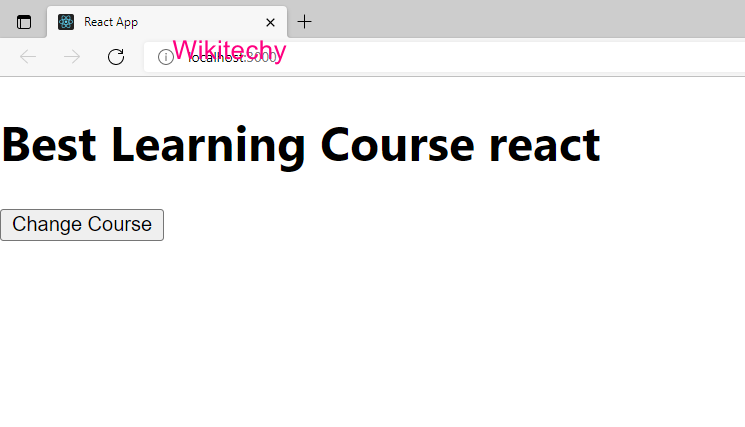
shouldComponentUpdate
- shouldComponentUpdate() method you can return a Boolean value that requires whether React should continue with the rendering or not.
- The default value is true.
The example below shows what happens when the shouldComponentUpdate() method returns false:
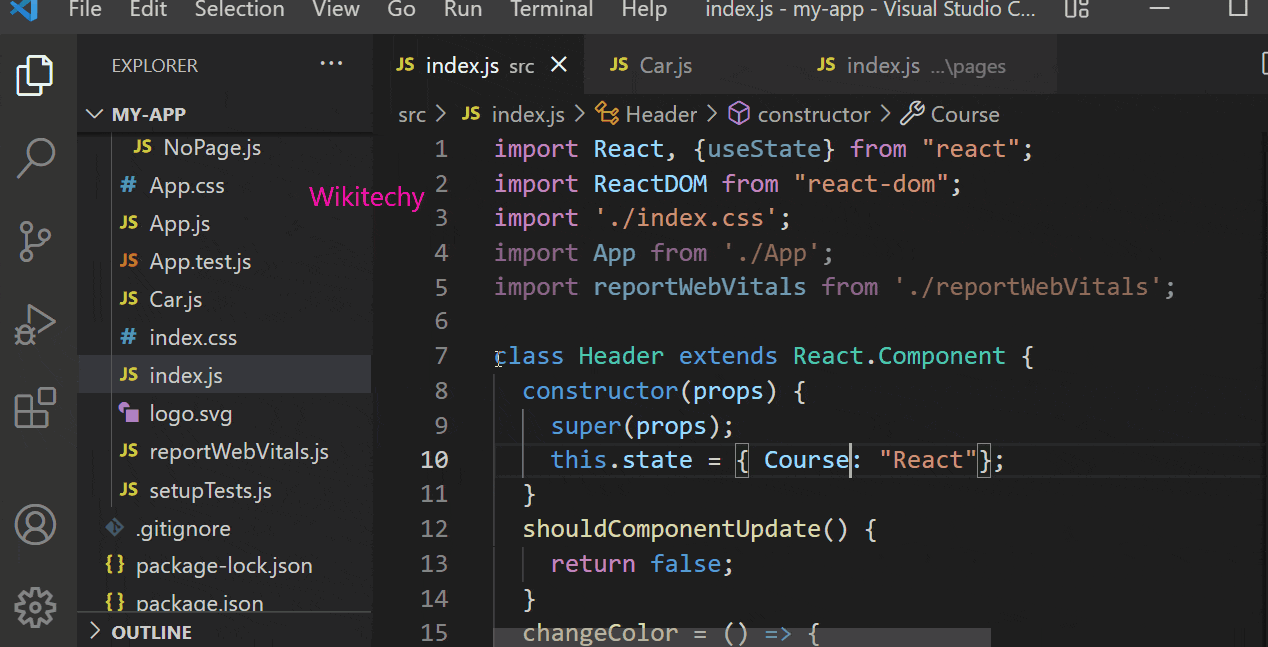
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Header extends React.Component {
constructor(props) {
super(props);
this.state = { Course: "React"};
}
shouldComponentUpdate() {
return false;
}
changeColor = () => {
this.setState({ Course: "HTML"});
}
render() {
return (
<div>
<h1>Best Learning Course is {this.state. Course}</h1>
<button type="button" onClick={this.changeCourse}>Change Course</button>
</div>
);
}
}
ReactDOM.render(<Header />, document.getElementById('root'));
Output
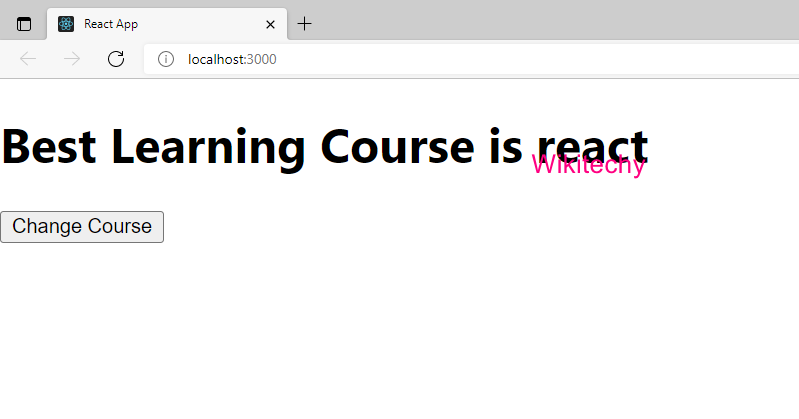
Here the same example as above, but this time the shouldComponentUpdate() method returns true instead:
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Header extends React.Component {
constructor(props) {
super(props);
this.state = { Course: "React"};
}
shouldComponentUpdate() {
return true;
}
changeColor = () => {
this.setState({ Course: "HTML"});
}
render() {
return (
<div>
<h1>Best Learning Course is {this.state. Course}</h1>
<button type="button" onClick={this.changeCourse}>Change Course</button>
</div>
);
}
}
ReactDOM.render(<Header />, document.getElementById('root'));
Output
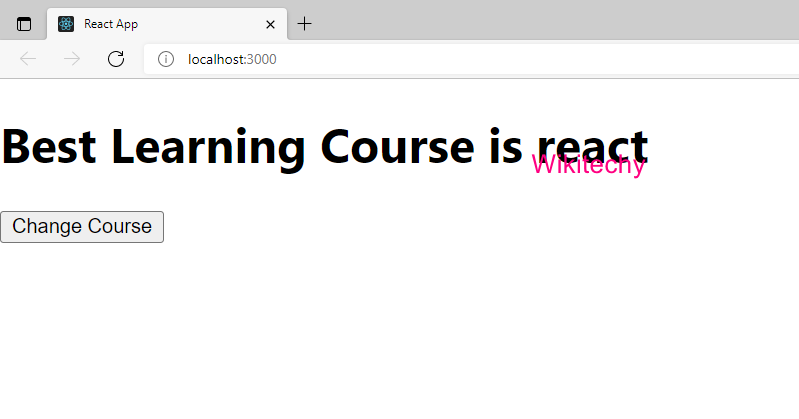
render
- The render() method is of course called when a component gets updated, it has to re-render the HTML to the DOM, with the new changes.
- The example below has a button that changes the course to python:
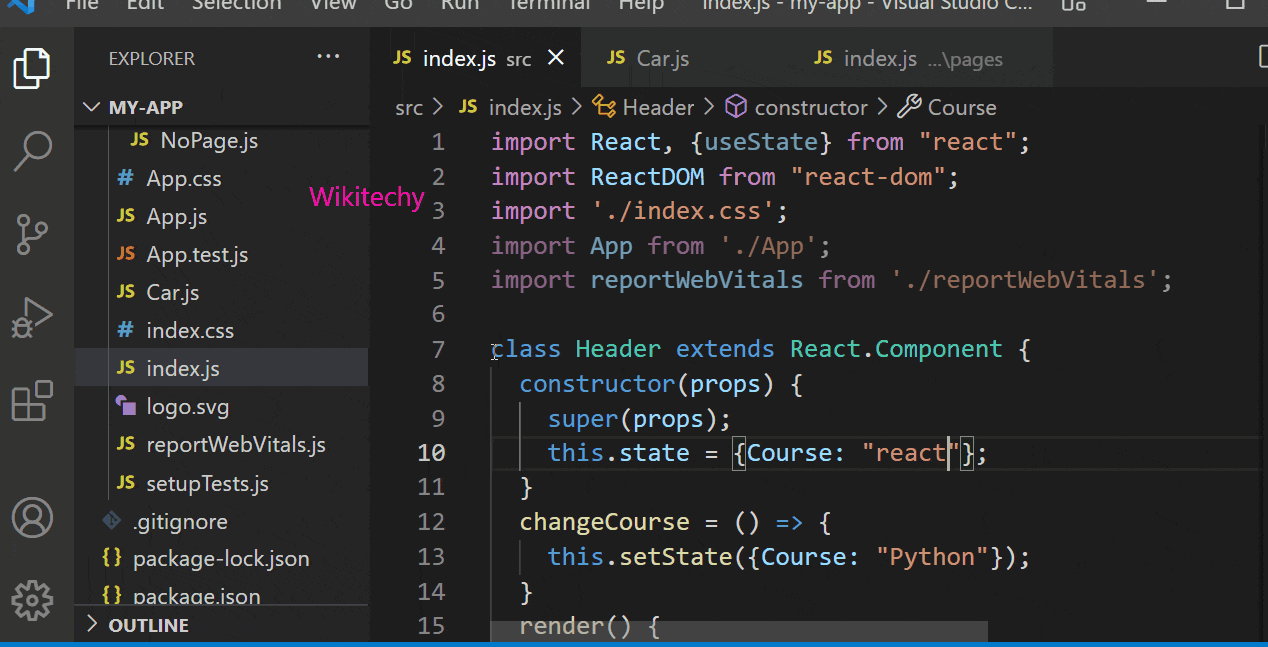
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Header extends React.Component {
constructor(props) {
super(props);
this.state = {Course: "react"};
}
changeCourse = () => {
this.setState({Course: "Python"});
}
render() {
return (
<div>
<h1>Best Learning Course is {this.state.Course}</h1>
<button type="button" onClick={this.changeCourse}>Change Course</button>
</div>
);
}
}
ReactDOM.render(<Header />, document.getElementById('root'));
Output
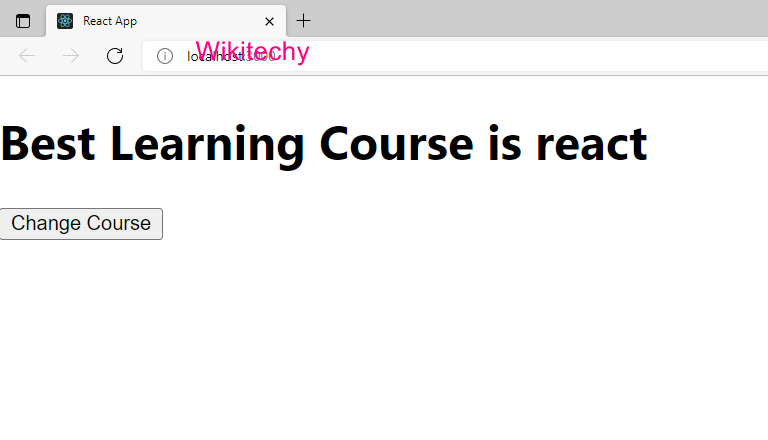
If you are clicking the Change Course button, the react course will changed to python course.
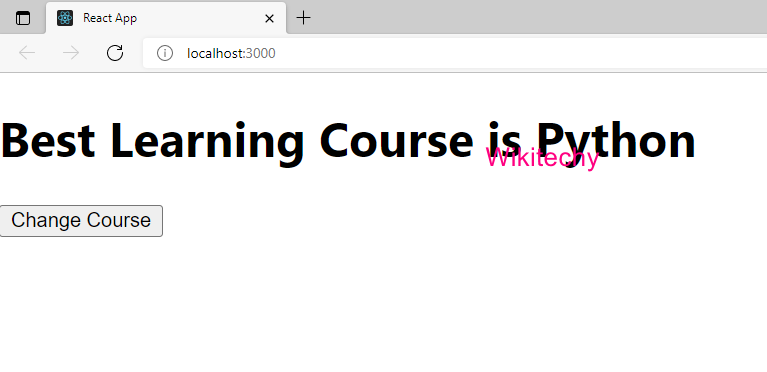
getSnapshotBeforeUpdate
- getSnapshotBeforeUpdate() method you have access to the props and state before the update, meaning that even after the update, you can check what the values were before the update.
- If the getSnapshotBeforeUpdate() method is present, you must include the componentDidUpdate() method, otherwise you will get an error.
- The example below might seem complex, but all it does is this:
- When the component is mounting it is rendered with the course "react".
- When the component has been mounted, a timer changes the state, and after one second, the course becomes "python".
- This action triggers the update phase, and since this component has a getSnapshotBeforeUpdate() method, this method is executed, and writes a message to the empty DIV1 element.
- Then the componentDidUpdate() method is executed and writes a message in the empty DIV2 element:
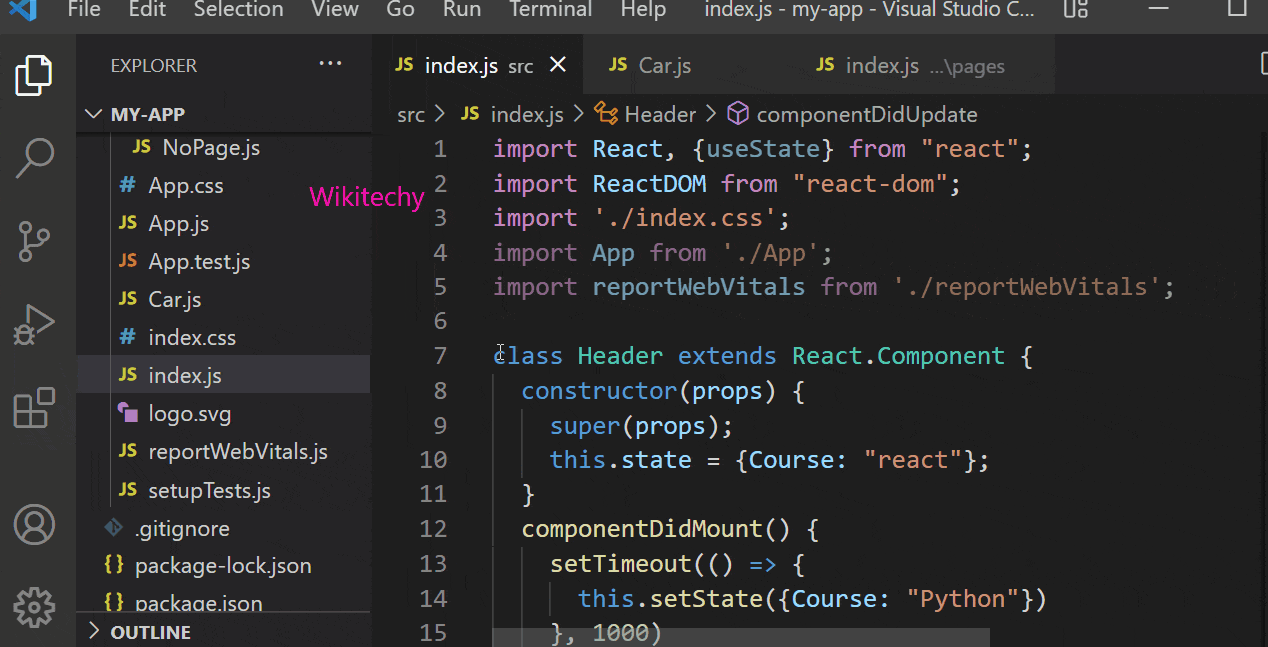
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Header extends React.Component {
constructor(props) {
super(props);
this.state = {favoritecolor: "react"};
}
componentDidMount() {
setTimeout(() => {
this.setState({favoritecolor: "Python"})
}, 1000)
}
getSnapshotBeforeUpdate(prevProps, prevState) {
document.getElementById("div1").innerHTML =
"Before the update, the course was " + prevState.favoritecolor;
}
componentDidUpdate() {
document.getElementById("div2").innerHTML =
"Now the updated Course is " + this.state.favoritecolor;
}
render() {
return (
<div>
<h1>My Best Cousre is {this.state.favoritecolor}</h1>
<div id="div1"></div>
<div id="div2"></div>
</div>
);
}
}
ReactDOM.render( <Header />, document.getElementById('root'));
Output
My Course is Python Before the update, the course was react Now the updated course is python
componentDidUpdate
- The componentDidUpdate method is called after the component is updated in the DOM.
- The example below might seem complex, but all it does is this:
- When the component is mounting it is rendered with the course "react".
- When the component has been mounted, a timer changes the state, and the course becomes "java".
- This action triggers the update phase, and since this component has a componentDidUpdate method, this method is executed and writes a message in the empty DIV element:
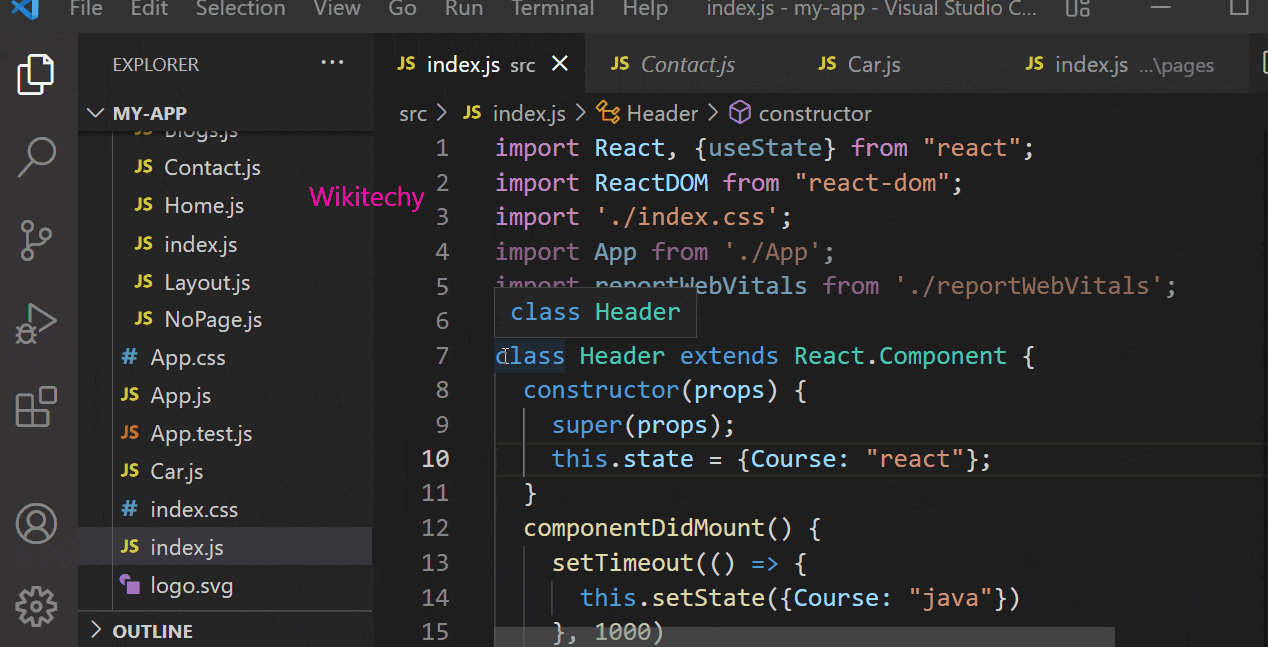
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Header extends React.Component {
constructor(props) {
super(props);
this.state = {Course: "react"};
}
componentDidMount() {
setTimeout(() => {
this.setState({Course: "java"})
}, 1000)
}
componentDidUpdate() {
document.getElementById("mydiv").innerHTML =
"Now The updated Course is " + this.state.Course;
}
render() {
return (
<div>
<h1>My Best Course is {this.state.Course}</h1>
<div id="mydiv"></div>
</div>
);
}
}
ReactDOM.render(<Header />, document.getElementById('root'));
Output
My Best Course is java Now The updated course is java
Unmounting
- when a component is removed from the DOM, or unmounting as React likes to call it.
- React has only one built-in method that gets called when a component is unmounted:
- componentWillUnmount()
componentWillUnmount
- The componentWillUnmount method is called when the component is removed from the DOM.
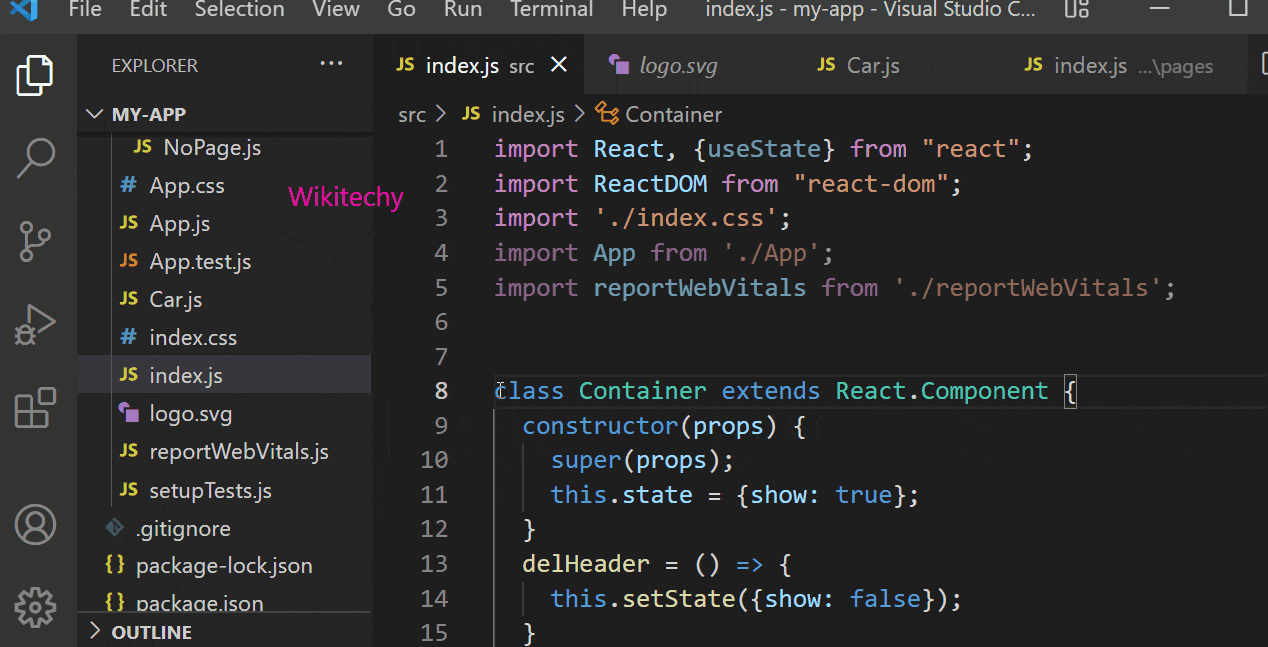
Sample Code
import React, {useState} from "react";
import ReactDOM from "react-dom";
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
class Container extends React.Component {
constructor(props) {
super(props);
this.state = {show: true};
}
delHeader = () => {
this.setState({show: false});
}
render() {
let header;
if (this.state.show) {
header = <Child />;
};
return (
<div>
{header}
<button type="button" onClick={this.delHeader}>Delete Header</button>
</div>
);
}
}
class Child extends React.Component {
componentWillUnmount() {
alert("Welcome to Wikitechy Header is unmounted.");
}
render() {
return (
<h1>Welcome to Wikitechy Tutorial</h1>
);
}
}
ReactDOM.render(<Container />, document.getElementById('root'));
Output
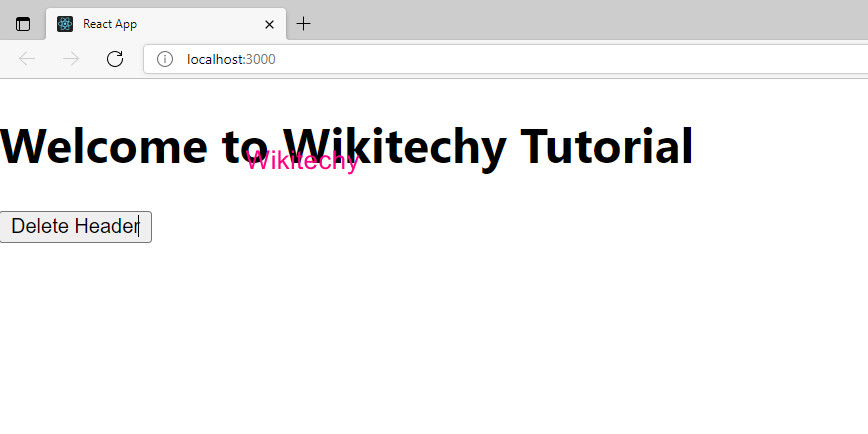
If you are clicking the Delete header button to display an alert box.
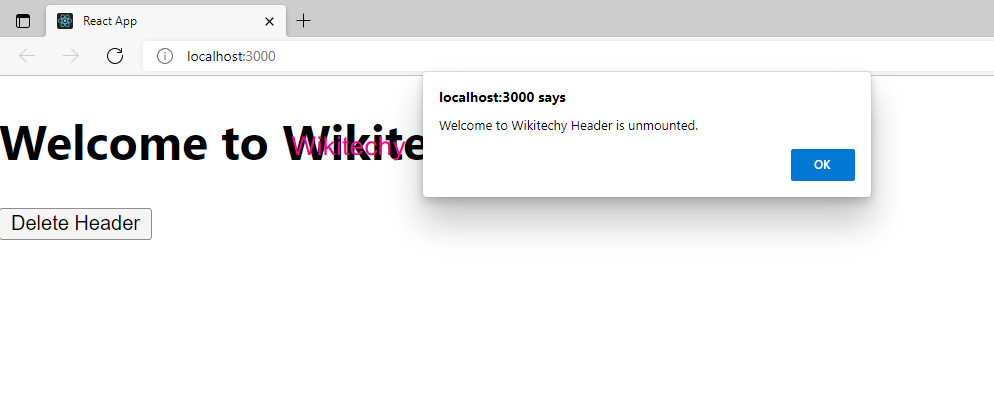