C++ Flow Control | Control flow introduction in c++ - Learn C++ , C++ Tutorial , C++ programming
What is Flow Control in C++?
- C++ provides control flow statements (also called flow control statements), which allow the programmer to change the CPU’s path through the program.
- There are quite a few different types of control flow statements, so we will cover them briefly here, and then in more detail throughout the rest of the chapter.

Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
Halt:
- The most basic control flow statement is the halt, which tells the program to quit running immediately.
- In C++, a halt can be accomplished through use of the exit() function that is defined in the cstdlib header.
- The exit function takes an integer parameter that is returned to the operating system as an exit code, much like the return value of main().
Jumps:
- The next most basic flow control statement is the jump.
- A jump unconditionally causes the CPU to jump to another statement.
- The goto, break, and continue keywords all cause different types of jump
- Function calls also cause jump-like behavior.
- When a function call is executed, the CPU jumps to the top of the function being called.
- When the called function ends, execution returns to the statement after the function call.
Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
Conditional branches:
- A conditional branch is a statement that causes the program to change the path of execution based on the value of an expression.
- The most basic conditional branch is an if statement, which you have seen in previous examples.
- Consider the following program:
- The switch keyword also provides a mechanism for doing conditional branching. We will cover if statements and switch statements in more detail in an upcoming section.
Loops:
- A loop causes the program to repeatedly execute a series of statements until a given condition is false.
- C++ provides 3 types of loops: while, do while, and for loops. C++11 added support for a new kind of loop called a for each loop.
- We will discuss loops at length toward the end of this chapter, except for the for each loop, which we’ll discuss a little later.
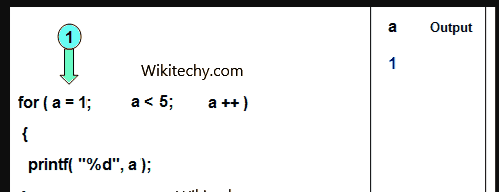
learn c++ tutorials - loops-for loop Example
Exceptions:
- Exceptions offer a mechanism for handling errors that occur in a function.
- If an error occurs in a function that the function cannot handle, the function can trigger an exception.
- This causes the CPU to jump to the nearest block of code that handles exceptions of that type.
- Exception handling is a fairly advanced feature of C++, and is the only type of control flow statement that we won’t be discussing in this section.
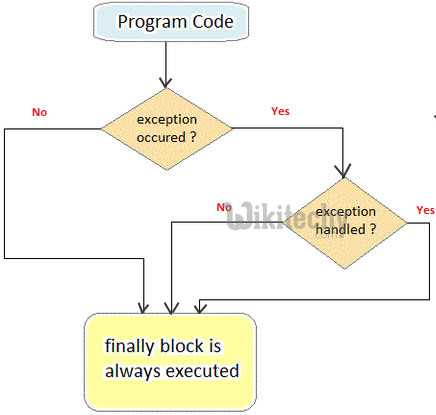
learn c++ tutorials - exception handling in c++ Example
Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
Conclusion:
- Using program flow statements, you can affect the path the CPU takes through the program and control under what conditions it will terminate.
- Prior to this point, the number of things you could have a program do was fairly limited.
- Being able to control the flow of your program makes any number of interesting things possible, such as displaying a menu.
- the user makes a valid choice, printing every number between x and y, or determining the factors of a number.
- Once you understand program flow, the things you can do with a C++ program really open up. No longer will you be restricted to toy programs and academic exercises.