C++ Global Variable - Global Variables - Learn C++ - C++ Tutorial - C++ programming
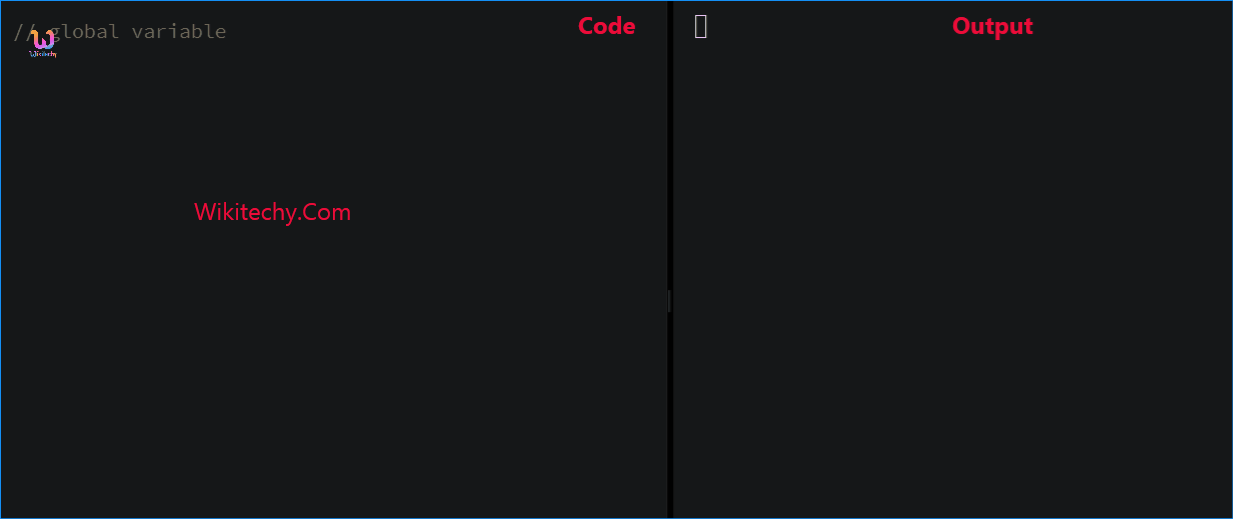
Learn c++ - c++ tutorial - c++ global variables - c++ examples - c++ programs
Defining global variables:
- Variables declared outside of a block are called global variables.
- Global variables have static duration, which means they are created when the program starts and are destroyed when it ends.
- Global variables have file scope (also informally called “global scope” or “global namespace scope”), which means they are visible until the end of the file in which they are declared.
- By convention, global variables are declared at the top of a file, below the includes, but above any code.
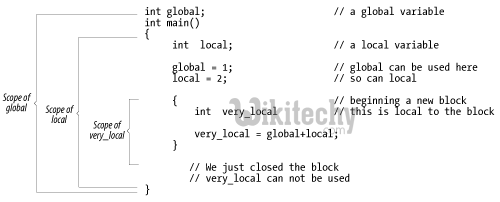
learn c++ tutorials - global variables in c++ and local variable in c++ Example
#include <iostream>
using namespace std;
// Global variable declaration:
int g;
int main () {
// Local variable declaration:
int a, b;
// actual initialization
a = 10;
b = 20;
g = a + b;
cout << g;
return 0;
}
Output:
30
- Similar to how variables in an inner block with the same name as a variable in an outer block hides the variable in the outer block, local variables with the same name as a global variable hide the global variable inside the block that the local variable is declared in.
- However, the global scope operator (::) can be used to tell the compiler you mean the global version instead of the local version.
#include <iostream>
int value(5); // global variable
int main()
{
int value = 7; // hides the global variable value
value++; // increments local value, not global value
::value--; // decrements global value, not local value
std::cout << "global value: " << ::value << "\n";
std::cout << "local value: " << value << "\n";
return 0;
} // local value is destroyed
Output:
global value: 4
local value: 8
Linkage:
- In general, linkage will refer to the visibility of symbols to the linker when processing files. Linkage can be either internal or external.
External Linkage:
- When a symbol (variable or function) has external linkage, that means that that symbol is visible to the linker from other files, i.e. it is “globally” visible and can be shared between translation units
int x; // is the same as
extern int x{}; // which will both likely cause linker errors.
extern int x; // while this only declares the integer, which is ok.
Internal Linkage:
- When a symbol has internal linkage, it will only be visible within the current translation unit. Do not confuse the term visible here with access rights like private.
- Visibility here means that the linker will only be able to use this symbol when processing the translation unit in which the symbol was declared, and not later (as with symbols with external linkage)
Example:
- Here an example:
header.hpp:
static int variable = 42;
file1.hpp:
void function1();
file2.hpp:
void function2();
file1.cpp:
#include "header.hpp"
void function1() { variable = 10; }
file2.cpp:
#include "header.hpp"
void function2() { variable = 123; }
main.cpp:
#include "header.hpp"
#include "file1.hpp"
#include "file2.hpp"
#include <iostream>
auto main() -> int
{
function1();
function2();
std::cout << variable << std::endl;
}
- Because variable has internal linkage, each translation unit that includes header.hpp gets its own unique copy of variable. Here, there are three translation units:
- file1.cpp
- file2.cpp
- main.cpp
- When function1 is called, file1.cpp’s copy of variable is set to 10. When function2 is called, file2.cpp’s copy of variable is set to 123. However, the value printed out in main.cpp is variable, unchanged: 42.
Internal and external linkage via the static and extern keywords:
- In addition to scope and duration, variables have a third property: linkage.
- A variable’s linkage determines whether multiple instances of an identifier refer to the same variable or not.
- A variable with internal linkage is called an internal variable (or static variable).
- Variables with internal linkage can be used anywhere within the file they are defined in, but cannot be referenced outside the file they exist in.
- A variable with external linkage is called an external variable.
- Variables with external linkage can be used both in the file they are defined in, as well as in other files.