Inheritance In C++ | Public, Protected and Private Inheritance in C++ Programming - Learn C++ - C++ Tutorial - C++ programming
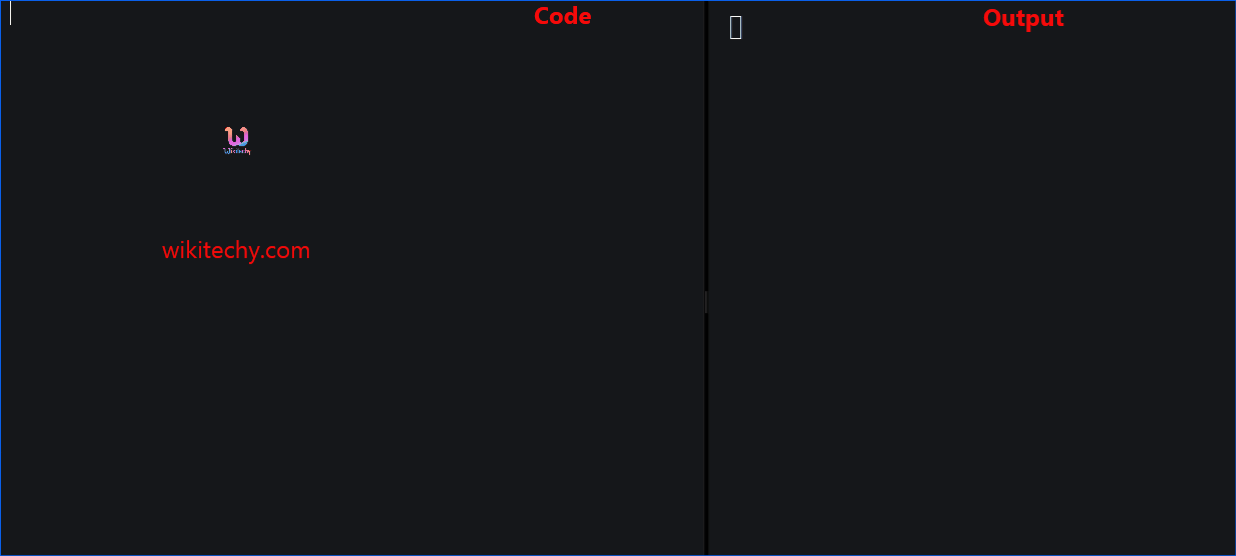
Learn c++ - c++ tutorial - inheritance in c++ - c++ examples - c++ programs

- You can declare a derived class from a base class with different access control, i.e., public inheritance , protected inheritance or private inheritance.
#include <iostream>
using namespace std;
class base
{
.... ... ....
};
class derived : access_specifier base
{
.... ... ....
};
- Note: Either public, protected or private keyword is used in place of access_specifierterm used in the above code.
Example of public, protected and private inheritance in C++
class base
{
public:
int x;
protected:
int y;
private:
int z;
};
class publicDerived: public base
{
// x is public
// y is protected
// z is not accessible from publicDerived
};
class protectedDerived: protected base
{
// x is protected
// y is protected
// z is not accessible from protectedDerived
};
class privateDerived: private base
{
// x is private
// y is private
// z is not accessible from privateDerived
}
- In the above example, we observe the following things:
- base has three member variables: x, y and z. which are public, protected andprivate member respectively.
- publicDerived inherits variables x and y as public and protected. z is not inherited as it is a private member variable of base.
- protectedDerived inherits variables x and y. Both variables become protected. z is not inherited.
- If we derive a class derived FromProtectedDerived from protected
- Derived, variables x and y are also inherited to the derived class.
- privateDerived inherits variables x and y. Both variables become private. z is not inherited. If we derive a class derivedFromPrivateDerived
- from private Derived, variables x and y are not inherited because they are private variables of privateDerived.
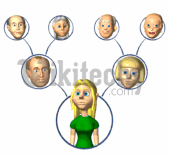
learn c++ tutorials - inheritance in c++ Example
Accessibility in Public Inheritance
Accessibility | private variables | protected variables | public variables |
---|---|---|---|
Accessible from own class? | yes | yes | yes |
Accessible from derived class? | no | yes | yes |
Accessible from 2nd derived class? | no | yes | yes |
Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
Accessibility in Protected Inheritance
Accessibility | private variables | protected variables | public variables |
---|---|---|---|
Accessible from own class? | yes | yes | yes(inherited as protected variables) |
Accessible from derived class? | no | yes | yes |
Accessible from 2nd derived class? | no | yes | yes |
Accessibility in Private Inheritance
Accessibility | private variables | protected variables | public variables |
---|---|---|---|
Accessible from own class? | yes | yes | yes |
Accessible from derived class? | no | yes(inherited as private variables) | yes(inherited as private variables) |
Accessible from 2nd derived class? | no | yes | yes |
C++ Function Overriding
- In this article, you will learn about function overriding. Also, you will learn how can assess the overridden function of the base class in C++ programming

- It allows software developers to derive a new class from the existing class. The derived class inherits features of the base class (existing class).
- Suppose, both base class and derived class have a member function with same name and arguments (number and type of arguments).
- If you create an object of the derived class and call the member function which exists in both classes (base and derived), the member function of the derived class is invoked and the function of the base class is ignored.
- This feature in C++ is known as function overriding.
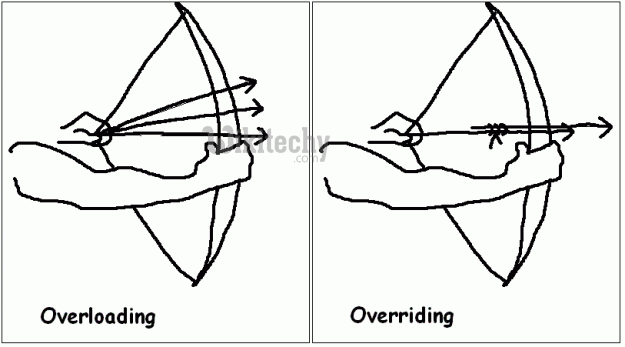
learn c++ tutorials - overloading vs overriding in c++ Example

Learn C++ , C++ Tutorial , C++ programming - C++ Language -Cplusplus
How to access the overridden function in the base class from the derived class?
- To access the overridden function of the base class from the derived class, scope resolution operator :: is used. For example,
- If you want to access getData() function of the base class, you can use the following statement in the derived class.
Base::getData();
