php tutorial - PayPal Integration in PHP Using PDO - php programming - learn php - php code - php script
In our previous blog, we have already discussed, how can we use PayPal button for collecting payments
online. This time we came up with slight changes in that tutorial.
Here, we will be going to see how we can Integrate PayPal payment gateway using PHP and PDO (PHP Data Objects). Using PDO you can Integrate your script to any Database which supports PDO.
The complete motive of this tutorial is to showcase an online product selling website that we call as a ECommerce website, using which one can sell his product using a secure payment mode, i.e. PayPal.
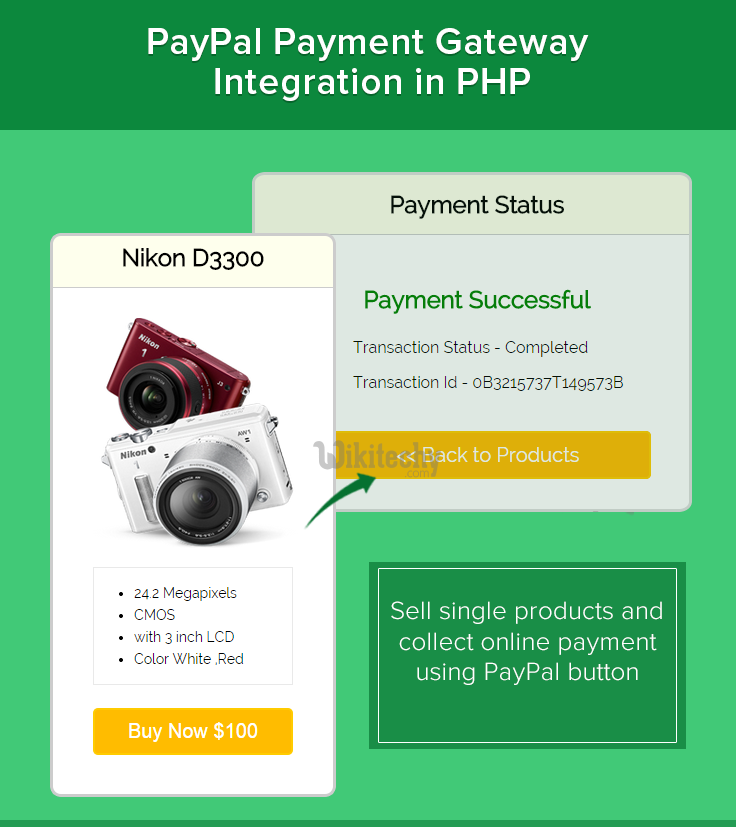
Learn php - php tutorial - pdo - php examples - php programs
Note : The installation documentation is given in the download file.
PayPal offers sandbox account for development and testing purpose. Here we are using Sandbox for demo. so if you would like to test our demo you can use your PayPal sandbox credentials.
If you want to run script to your live projects just change the $paypal_url on process.php page
//Paypal url for live
$paypal_url = 'https://www.paypal.com/cgi-bin/webscr';
//PayPal url for testing
$paypal_url = 'https://www.sandbox.paypal.com/cgi-bin/webscr';
click below button to copy the code. php tutorial - team
Steps to follow :
Here in the download code provided above, there is file naming paypal.sql in which sql queries are written for creating database, table and different columns in it.
You just need to import it in your phpmyadmin it will create the required tables.
After doing it successfully, to run the script you need to call payments.php file.
Below are the details of the code used in the tutorial :
MY-SQL Code
CREATE TABLE IF NOT EXISTS `tbl_product_detail` (
`product_id` int(11) NOT NULL AUTO_INCREMENT,
`item_number` varchar(255) NOT NULL,
`product_name` varchar(255) NOT NULL,
`product_img` varchar(255) NOT NULL,
`product_price` int(11) NOT NULL,
`product_currency` varchar(255) NOT NULL,
`product_dec` text NOT NULL,
PRIMARY KEY (`product_id`)
)
click below button to copy the code. php tutorial - team
Connection.php
This is file used for database connectivity. It is used for making connection variable by PDO(PHP Data Objects).
<?php
function connection_open() {
// Database credential
$servername = "localhost";
$username = "root";
$password = "";
//Data base name
$dbname = "paypal";
try {
global $conn;
// Open the connection using PDO.
$conn = new PDO("mysql:host=$servername;dbname=$dbname", $username, $password);
// set the PDO error mode to exception
$conn->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
} catch (PDOException $e) {
// echo $sql . "<br>" . $e->getMessage();
die();
}
}
function connection_close() {
global $conn;
$conn = null;
}
?>
click below button to copy the code. php tutorial - team
Function.php
This file contain all required function for database operation.
<?php
//This function is used for fetching all the records from the table
function Select_All_Records($table_name) {
global $conn;
$sql = "select * from $table_name";
try {
$stmt = $conn->query($sql);
return $stmt;
} catch (PDOException $e) {
print $e->getMessage();
}
}
//This function is used for fetching record with one Filter.
function Select_Record_By_One_Filter($data,$table_name){
global $conn;
$key = array_keys($data);
$value = array_values($data);
$sql = "select * from $table_name where $key[0] = '$value[0]'";
try {
$stmt = $conn->query($sql);
return $stmt;
} catch (PDOException $e) {
print $e->getMessage();
}
}
?>
click below button to copy the code. php tutorial - team
Payments.php
This is the main file in which the products details are being displayed with buy now button.
<?php
include('include/function.php');
include('include/connection.php');
echo connection_open();
?>
<html>
<head>
<title>paypal_simple_integration</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<div id = "main">
<h1>PayPal Payment Gateway Integration in PHP</h1>
<?php
//Select_All_Records function is used for fetching all the records from the table
$query = Select_All_Records('tbl_product_detail');
$query->setFetchMode(PDO::FETCH_ASSOC);
while ($result = $query->fetch()) {
?>
<div id = "login">
<h2><?php echo $result['product_name']; ?></h2>
<hr/>
<form action = "process.php" method = "post">
<input type = "hidden" value = "<?php echo $result['item_number']; ?>" name = "product_id">
<img id = "product_img" src = "<?php echo $result['product_img']; ?>"/><br><br>
<div id = "product_content">
<ul><?php $description = explode('#@#', $result['product_dec']);
foreach ($description as $value) { ?>
<li>
<?php echo $value; ?>
</li>
<?php } ?>
</ul>
</div>
<input type = "submit" value = " Buy Now $ <?php echo $result['product_price']; ?> " name = "submit"/><br />
<span></span>
</form>
</div>
<?php } ?>
</div>
</body>
</html>
<?php
//connection_close() is used for closing the connection .
connection_close();
?>
click below button to copy the code. php tutorial - team
Process.php
This file contain code to process payment to paypal.
<?php
include('include/function.php');
include('include/connection.php');
if (isset($_POST['submit'])) {
echo connection_open();
if (isset($_POST['product_id'])) {
$product_id = base64_decode($_POST['product_id']);
$data = array(
'product_id' => $product_id
);
$query = Select_Record_By_One_Filter($data, 'tbl_product_detail');
$query->setFetchMode(PDO::FETCH_ASSOC);
$result = $query->fetch();
//Put sandbox url for testing.
//$paypal_url = 'https://www.sandbox.paypal.com/cgi-bin/webscr';
//Paypal url for live
$paypal_url = 'https://www.paypal.com/cgi-bin/webscr';
//Here we can used seller email id.
$merchant_email = 'your paypal seller email id';
//here we can put cancle url when payment is not completed.
$cancel_return = "http://localhost/paypal_simple_integration/payments.php";
//here we can put cancle url when payment is Successful.
$success_return = "http://localhost/paypal_simple_integration/success.php";
$product_name = $result['product_name'];
$product_price = $result['product_price'];
$product_currency = $result['product_currency'];
echo connection_close();
}
?>
<div style="margin-left: 38%"><img src="ajax-loader.gif"/><img src="processing_animation.gif"/></div>
<form name="myform" action="<?php echo $paypal_url; ?>" method="post" target="_top">
<input type="hidden" name="cmd" value="_xclick">
<input type="hidden" name="cancel_return" value="<?php echo $cancel_return ?>">
<input type="hidden" name="return" value="<?php echo $success_return; ?>">
<input type="hidden" name="business" value="<?php echo $merchant_email; ?>">
<input type="hidden" name="lc" value="C2">
<input type="hidden" name="item_name" value="<?php echo $product_name; ?>">
<input type="hidden" name="item_number" value="<?php echo $_POST['product_id']; ?>">
<input type="hidden" name="amount" value="<?php echo $product_price; ?>">
<input type="hidden" name="currency_code" value="<?php echo $product_currency; ?>">
<input type="hidden" name="button_subtype" value="services">
<input type="hidden" name="no_note" value="0">
</form>
<script type="text/javascript">
document.myform.submit();
</script>
<?php } ?>
click below button to copy the code. php tutorial - team
Success.php
PayPal call this file when payment gets successfully completed and provide $_REQUEST array which contains product id, PayPal transaction ID, PayPal received amount value, PayPal received currency type and PayPal product status which is displayed in this page.
<?php
include('include/function.php');
include('include/connection.php');
echo connection_open();
?>
<html>
<head>
<title>paypal_simple_integration</title>
<link rel="stylesheet" type="text/css" href="style.css">
</head>
<body>
<?php
if (!empty($_REQUEST)) {
$product_no = $_REQUEST['item_number']; // Product ID
$product_transaction = $_REQUEST['tx']; // Paypal transaction ID
$product_price = $_REQUEST['amt']; // Paypal received amount value
$product_currency = $_REQUEST['cc']; // Paypal received currency type
$product_status = $_REQUEST['st']; // Paypal product status
$product_no = base64_decode($product_no);
$data = array(
'product_id' => $product_no
);
$query = Select_Record_By_One_Filter($data, 'tbl_product_detail');
$query->setFetchMode(PDO::FETCH_ASSOC);
$result = $query->fetch();
echo connection_close();
}
?>
<div id="main">
<h1>PayPal Payment Gateway Integration in PHP</h1>
<div id="return">
<h2>Payment Status </h2>
<hr/>
<?php
//Rechecking the product price and currency details
if ($product_price == $result['product_price'] && $product_currency == $result['product_currency']) {
echo "<h3 id='success'>Payment Successful</h3>";
echo "<P>Transaction Status - " . $product_status . "</P>";
echo "<P>Transaction Id - " . $product_transaction . "</P>";
echo "<div class='back_btn'><a href='payments.php' id= 'btn'><< Back to Products</a></div>";
} else {
echo "<h3 id='fail'>Payment Failed</h3>";
echo "<P>Transaction Status - Unompleted</P>";
echo "<P>Transaction Id - " . $product_transaction . "</P>";
echo "<div class='back_btn'><a href='payments.php' id= 'btn'><< Back to Products</a></div>";
}
?>
</div>
<div id="wiki">
<a href=https://www.wikitechy.com/app><img src="wikitechy.jpg" alt="Online Form Builder"/></a>
</div>
</div>
</body>
</html>
click below button to copy the code. php tutorial - team
Style.css
Includes basic styling of HTML elements.
@import url(http://fonts.googleapis.com/css?family=Raleway);
#main{
width: 950PX;
margin: 50PX auto;
font-family:raleway;
}
span{
color:red;
}
h1{
margin-left: 14%;
}
h2{
background-color: #FEFFED;
text-align:center;
border-radius: 10px 10px 0 0;
margin: -10px -40px;
padding: 15px;
}
hr{
border:0;
border-bottom:1px solid #ccc;
margin: 10px -40px;
margin-bottom: 30px;
}
#login{
width: 200px;
float: left;
border-radius: 10px;
font-family:raleway;
border: 2px solid #ccc;
padding: 10px 40px 11px;
margin: 16PX;
}
input[type=text],input[type=password]{
width:99.5%;
padding: 10px;
margin-top: 8px;
border: 1px solid #ccc;
padding-left: 5px;
font-size: 16px;
font-family:raleway;
}
input[type=submit]{
width: 100%;
background-color:#FFBC00;
color: white;
border: 2px solid #FFCB00;
padding: 10px;
font-size:20px;
cursor:pointer;
border-radius: 5px;
margin-bottom: 15px;
}
#profile{
padding:50px;
border:1px dashed grey;
font-size:20px;
background-color:#DCE6F7;
}
#logout{
float:right;
padding:5px;
border:dashed 1px gray;
}
a{
text-decoration:none;
color: cornflowerblue;
}
i{
color: cornflowerblue;
}
ul{
line-height: 22px;
}
#product_img{
width: 210px;
height: 230px;
}
#product_content{
width: 198px;
height: 116px;
margin-bottom: 23px;
font-size: 14px;
border: 1px solid rgba(128, 128, 128, 0.17);
}
#return{
width: 492px;
height: 350px;
float: left;
border-radius: 10px;
font-family: raleway;
border: 2px solid #ccc;
padding: 10px 40px 11px;
margin: 16PX;
}
#return h3#success{
text-align: center;
font-size: 24px;
margin-top: 50px;
color: green;
}
#return h3#fail{
text-align: center;
font-size: 24px;
margin-top: 50px;
color: red;
}
#btn{
width: 100%;
background-color: #FFBC00;
color: white;
border: 2px solid #FFCB00;
padding: 10px 70px;
font-size: 20px;
cursor: pointer;
border-radius: 5px;
margin-bottom: 15px;
margin: 0 auto;
}
#return .back_btn{
margin-top: 51px;
margin-left: 19%;
}
#return P{
margin-left: 122px;
}