php tutorial - PHP - PHP Variables - PHP Tutorial - php programming - learn php - php code - php script
PHP Variables
- In PHP the variable is used for storing the value as text (or) number.
- The advantage for defining the variable is that we can able to reuse the variable throughout your code.
- A variable start with the “$” dollar symbol, followed by the name of the variable.
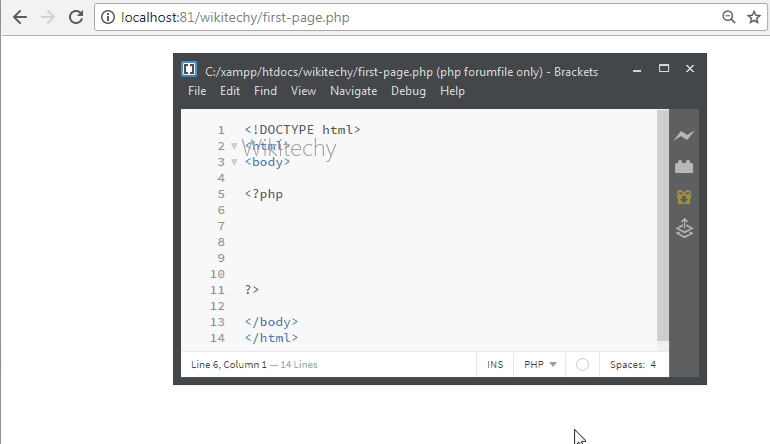
Learn PHP - PHP tutorial - Variables - PHP examples - PHP programs
php scripts Syntax :
$variable_name = Value;
click below button to copy the code. php tutorial - team
Conditions for Defining Variable Name :
- Generally, a variable name starts with letter or the underscore character and the variable name cannot start with number.
- A variable name can only contain alpha-numeric characters and underscores (A-z , 0-9 , and _ ).
Features of Variable :
- In php we no need to define the datatype of the variable, because it will automatically define the datatype based on the value which we are specifying.
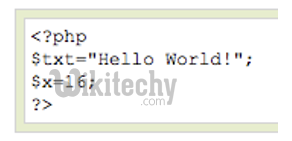
- In PHP, a variable does not need to be declared before adding a value to it.
- In the example above, you see that you do not have to tell PHP which data type the variable is.
- PHP automatically converts the variable to the correct data type, depending on its value.
- A variable name must start with a letter or an underscore "_" -- not a number
- A variable name can only contain alpha-numeric characters, underscores (a-z, A-Z, 0-9, and _ )
- A variable name should not contain spaces. If a variable name is more than one word, it should be separated with an underscore ($my_string) or with capitalization ($myString)
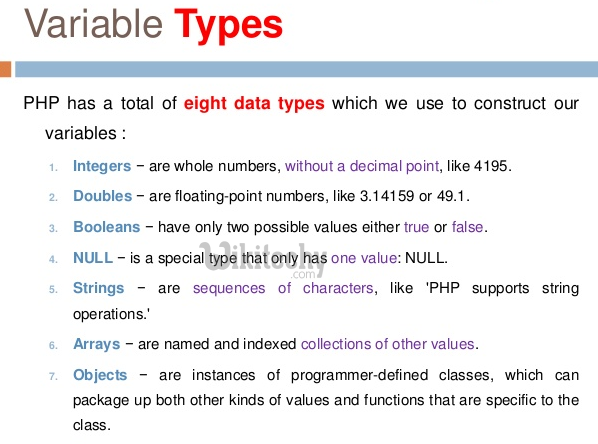
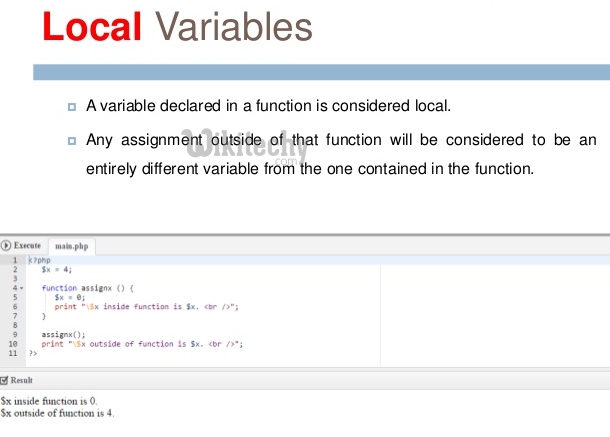
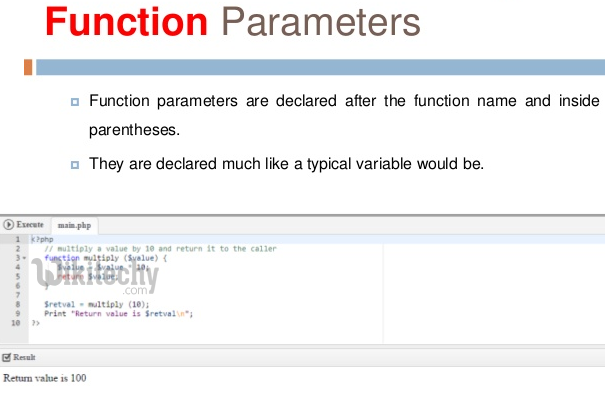
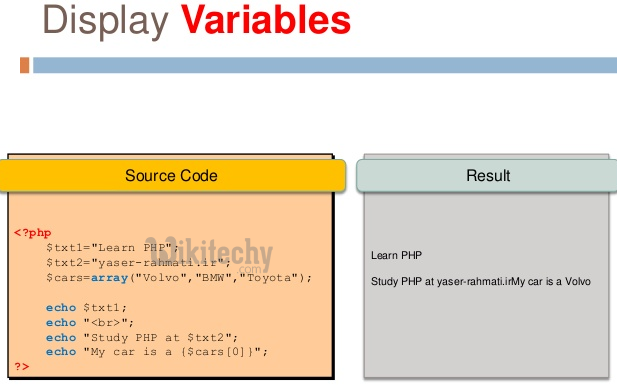
PHP Concatenation
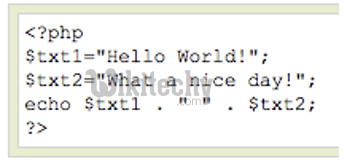

<!DOCTYPE html>
<html>
<body>
<?php
$samp1 = "Welcome Wikitechy PHP Tutorial Point";
$samp2 = 100;
$samp3 = 10.5;
echo $samp1;
echo "<br>";
echo $samp2;
echo "<br>";
echo $samp3;
?>
</body>
</html>
click below button to copy the code. php tutorial - team
php programmer Code Explanation :
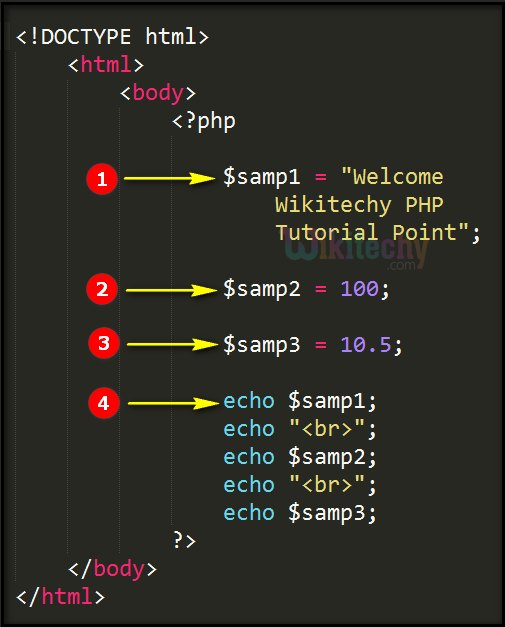
- In this Example of Php code, “samp1” (variable name) starts with “$” dollar sign. Here the variable “samp1” holds the string value inside the double quotes “Welcome Wikitechy PHP Tutorial point” which must be ended up with the semicolon ( ; ) .
- Here the variable “samp2” holds the integer data type with its value as 100 which must be ended up with the semicolon ( ; ) .
- In this step, the variable “samp3” holds the float data type value as 10.5 which must be ended up with the semicolon ( ; ) .
- echo $samp1 - echo statement is similar to print statement, which will display the value by calling the particular variable. Here we have 3 echo statements displaying the contents in the browser as shown below.
php development Sample Output :
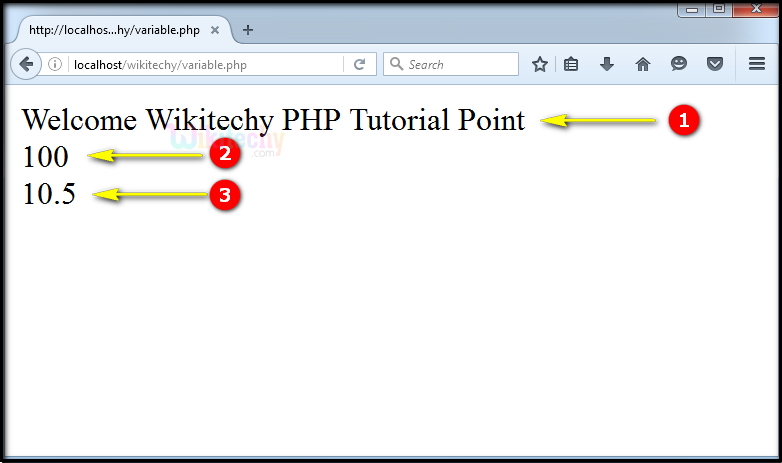
- Here the echo statement “Welcome Wikitechy PHP Tutorial Point” is shown in the browser window.
- Here the echo statement “100” which is the integer datatype is shown in the browser window.
- Here the echo statement “10.5” which is the float datatype is shown in the browser window.
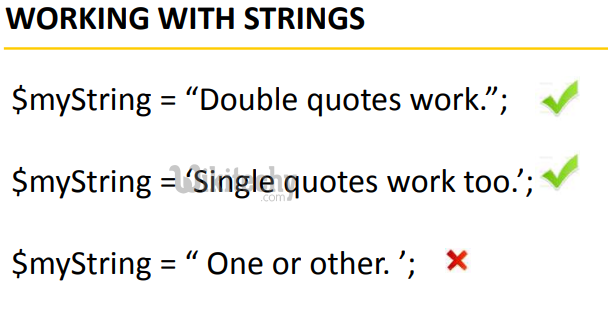
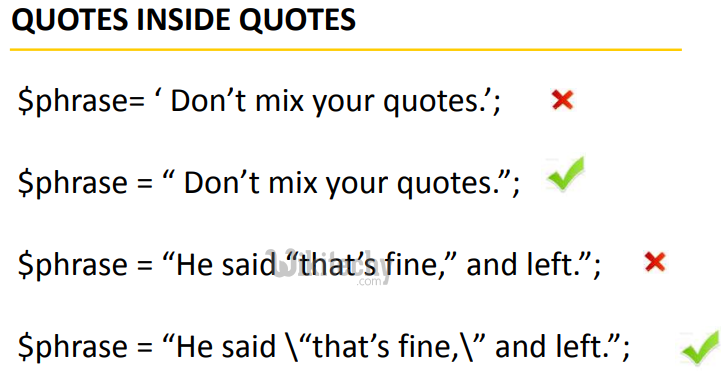
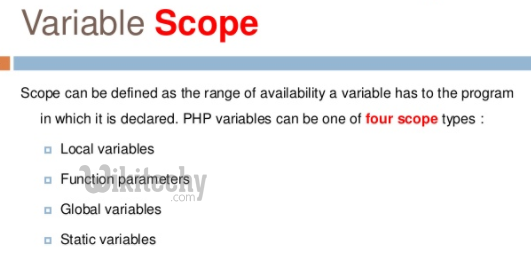
Global and Local Scope
<?php
$x = 5; // global scope
function myTest() {
// using x inside this function will generate an error
echo "<p>Variable x inside function is: $x</p>";
}
myTest();
echo "<p>Variable x outside function is: $x</p>";
?>
click below button to copy the code. php tutorial - team
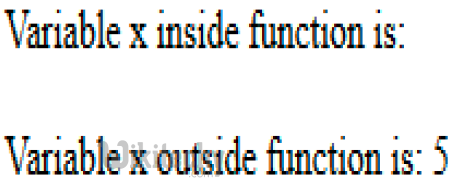
PHP The global Keyword
<?php
$x = 5;
$y = 10;
function myTest() {
global $x, $y;
$y = $x + $y;
}
myTest();
echo $y; // outputs 15
?>
click below button to copy the code. php tutorial - team
Output: 15
PHP The static Keyword
<?php
function myTest() { Output
static $x = 0;
echo $x;
$x++;
}
myTest();
myTest();
myTest();
?>