php tutorial - PHP Constants - php programming - learn php - php code - php script
- A constant is an identifier (identifier is the name that identifies labels, identity and so on). In general, the constant identifiers will always be in uppercase.
- In terms of the constant, its value cannot change during the execution of the script.
- 2 types of Constants are available:
- Valid Constants
- Invalid Constants
If a constant name starts with a letter or underscore, followed by any number of letters, numbers, or underscores then it is called as Valid Constants if not then it will be said to be Invalid Constant.
Syntax
<?php
// Syntax for Valid constant names
define("WIKI", "something");
define("WIKI7", "something else");
define("WIKI_TECHY", "something more");
// Syntax for Invalid constant names
define("7WIKI", "something");
?>
<?php
define(name, value, case-insensitive)
?>
- name: Here the name variable specifies the name of the constant
- value: Here the value variable specifies the value of the constant.
- case-insensitive: This specifies whether the constant name should be case-insensitive or not .
- Constants are defined using define() function, which accepts two arguments:
- Name of the constant,
- And its value.
- Below is an example of defining a constant in a script shown below in the sample code.
Sample Code 1
<!DOCTYPE html>
<html>
<head>
<title>Datatype-Constant</title>
</head>
<body>
<?php
define("wikitechy", 25);
echo wikitechy;
echo constant("wikitechy");
?>
</body>
</html>
php examples Code Explanation :
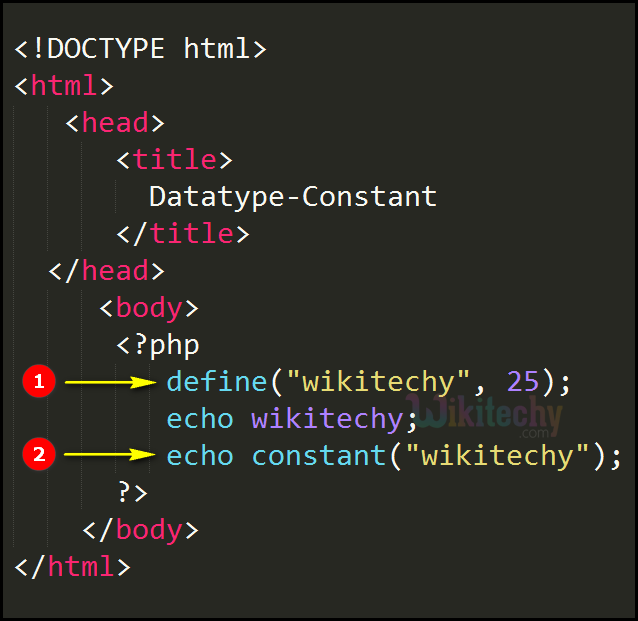
- Here in this example of Php, define(“wikitechy”,25) it specifies the define() function that defines a constant with its name and value “25”.
- echo constant(“wikitechy”) specifies the echo constant value is 25.
php tutorials Sample Output :
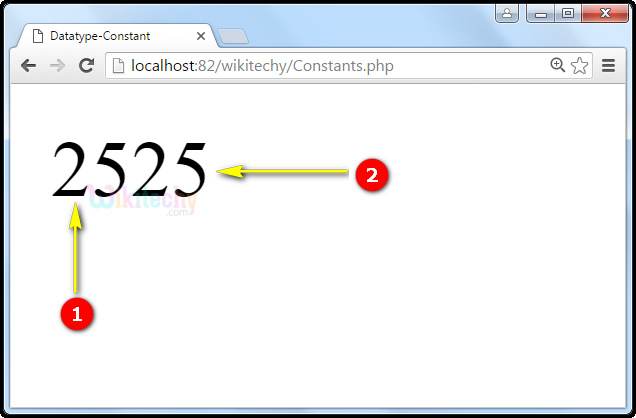
- Output value “25” indicates the define() function which has a value(25) for the term wikitechy.
- Output value “25” specifies the echo constant(“wikitechy”) whose value was already called as 25.
Sample Code 2
- Create a constant with a case-sensitive name:
<?php
define("GREETING", "Welcome to Wikitechy.com!");
echo GREETING;
?>
Output
Welcome to Wikitechy.com!
Sample Code 3
- Create a constant with a case-insensitive name
<?php
define("GREETING", "Welcome to Wikitechy.com!", true);
echo greeting;
?>
Output
Welcome to Wikitechy.com!
PHP Constant Arrays
- In PHP7, you can create an Array constant using the define() function.
Sample Code
<?php
define("cars", ["Honda","Swift","Vento"]);
echo cars[1];
?>
Output
Swift