php tutorial - PHP Sanitize and Validate Input Fields via Filters - php programming - learn php - php code - php script
In this tutorial, I’ll explain you to sanitize and validate form fields using PHP filter function filter_var(). This function requires a variable value to filter and name of the filter to use as an argument. It returns filtered data on success and FALSE on failure.
Why exactly do we need to sanitize and validate form fields..?? As you build a site using PHP, gaining unauthorized access to your site’s data can cause it to be hacked. Invalid submitted data not only lead to security problems, but it can also break your webpage. Whenever data or information flows over internet, it becomes one of the important aspects of information security.
Lets take a look on how to remove illegal characters and validate data. You can start doing this in PHP with validating and sanitizing data on your site. Why sanitize and not just validate? It’s possible that the user accidentally typed in a wrong character or maybe it was a bad copy and paste. By sanitizing the data, any illegal character from the data is removed. Just because the data is sanitized doesn’t ensure that it is properly formatted. And so validation is done which determines if the data is in proper form.
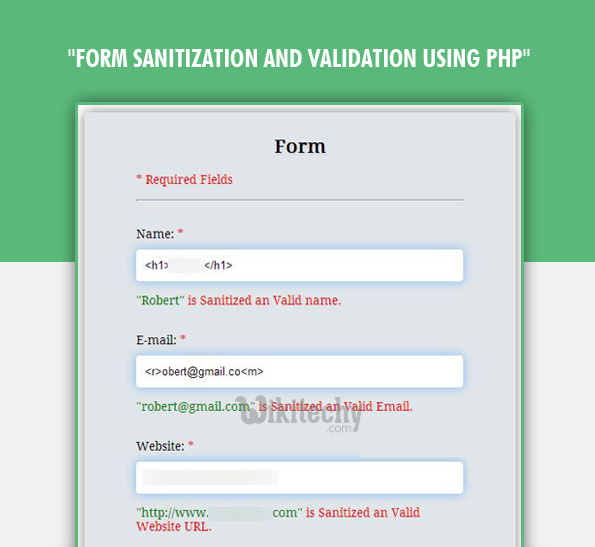
Learn php - php tutorial - field-validation - php examples - php programs
PHP File: sanitization.php
In this php script filter_var() filter function is used for sanitization and validation. Here we have an HTML form with three input fields namely: name, email and website. As user fills all information and clicks on submit button all input fields will be sanitized and validated using filters.
<?php
// Initializing Error Variables To Null.
$nameError ="";
$emailError ="";
$websiteError ="";
// This code block will execute when form is submitted
if(isset($_POST['submit'])){
/*--------------------------------------------------------------
Fetch name value from URL and Sanitize it
--------------------------------------------------------------*/
if($_POST['name'] != ""){
// Sanitizing name value of type string
$_POST['name'] = filter_var($_POST['name'], FILTER_SANITIZE_STRING);
$nameError = "<span class=\"valid\">\"".$_POST['name']."\" </span>is Sanitized an Valid name.";
if ($_POST['name'] == ""){
$nameError = "<span class=\"invalid\">Please enter a valid name.</span>";
}
}
else {
$nameError = "<span class=\"invalid\">Please enter your name.</span>";
}
/*------------------------------------------------------------------
Fetch email value from URL, Sanitize and Validate it
--------------------------------------------------------------------*/
if($_POST['email'] != ""){
//sanitizing email
$_POST['email'] = filter_var($_POST['email'], FILTER_SANITIZE_EMAIL);
//After sanitization Validation is performed
$_POST['email'] = filter_var($_POST['email'], FILTER_VALIDATE_EMAIL);
$emailError = "<span class=\"valid\">\"".$_POST['email']."\" </span>is Sanitized an Valid Email.";
if($_POST['email'] == ""){
$emailError = "<span class=\"invalid\">Please enter a valid email.</span>";
}
}
else {
$emailError = "<span class=\"invalid\">Please enter your email.</span>";
}
/*---------------------------------------------------------------------------
Fetch website value from URL, Sanitize and Validate it
----------------------------------------------------------------------------*/
if($_POST['website'] != ""){
//sanitizing URL
$_POST['website'] = filter_var($_POST['website'], FILTER_SANITIZE_URL);
//After sanitization Validation is performed
$_POST['website'] = filter_var($_POST['website'], FILTER_VALIDATE_URL);
$websiteError = "<span class=\"valid\">\"".$_POST['website']."\" </span>is Sanitized an Valid Website URL.";
if ($_POST['website'] == ""){
$websiteError = "<span class=\"invalid\">Please enter a valid website start with http:// </span>";
}
}
else {
$websiteError = "<span class=\"invalid\">Please enter your website URL.</span>";
}
}
?>
<!DOCTYPE html>
<html>
<head>
<title>Form Sanitization and Validation Using PHP - Demo Preview</title>
<meta content="noindex, nofollow" name="robots">
<link href="style.css" rel="stylesheet">
</head>
<body>
<div class="maindiv">
<div class="form_div">
<div class="title">
<h2>Form Sanitization and Validation Using PHP</h2>
</div>
<form action="sanitization.php" method="post">
<h2>Form</h2>
<p>* Required Fields</p>
Name: <span class="invalid">*</span>
<input class="input" name="name" type="text" value="">
<p><?php echo $nameError;?></p>
E-mail: <span class="invalid">*</span>
<input class="input" name="email" type="text" value="">
<p><?php echo $emailError;?></p>
Website: <span class="invalid">*</span>
<input class="input" name="website" type="text" value="">
<p><?php echo $websiteError;?></p><input class="submit" name="submit" type="submit" value="Submit">
</form>
</div>
</div> <!-- HTML Ends Here -->
</body>
</html>
click below button to copy the code. php tutorial - team
CSS File: style.css
For Styling HTML Elements.
@import "http://fonts.googleapis.com/css?family=Droid+Serif";
/* Above line is to import google font style */
.maindiv {
margin:0 auto;
width:980px;
height:500px;
background:#fff;
padding-top:20px;
font-size:14px
}
.title {
width:500px;
height:70px;
text-shadow:2px 2px 2px #cfcfcf;
font-size:16px;
text-align:center;
font-family:'Droid Serif',serif
}
.form_div {
width:70%;
float:left
}
p {
color:red
}
hr {
margin-top:15px;
margin-bottom:30px
}
form {
margin-top:50px;
width:380px;
border-radius:5px;
box-shadow:0 0 20px gray;
padding:10px 60px 40px;
background-color:#e0e5e9;
font-family:'Droid Serif',serif
}
form h2 {
text-align:center;
text-shadow:2px 2px 2px #cfcfcf
}
.input {
width:97%;
border-radius:4px;
box-shadow:0 0 16px 1px #8FC2F5;
margin-top:10px;
height:35px;
padding-left:10px;
border:none
}
.submit {
color:#fff;
border-radius:3px;
background:#1F8DD6;
margin-top:35px;
border:none;
width:100%;
height:40px;
box-shadow:0 0 1px 2px #123456;
font-size:16px
}
.radio {
width:15px;
height:15px;
border-radius:1px;
margin-top:10px;
padding:5px;
border:none;
margin-bottom:20px
}
.valid {
color:green
}
.invalid {
color:red
}