MongoDB Filter Query - Node JS tutorial
How to Filter Query of MongoDB in Node.js ?
- When selecting records from a table, we can filter the result by using a query object.
- The first argument of the find() method is a query object, and is used to limit the search.
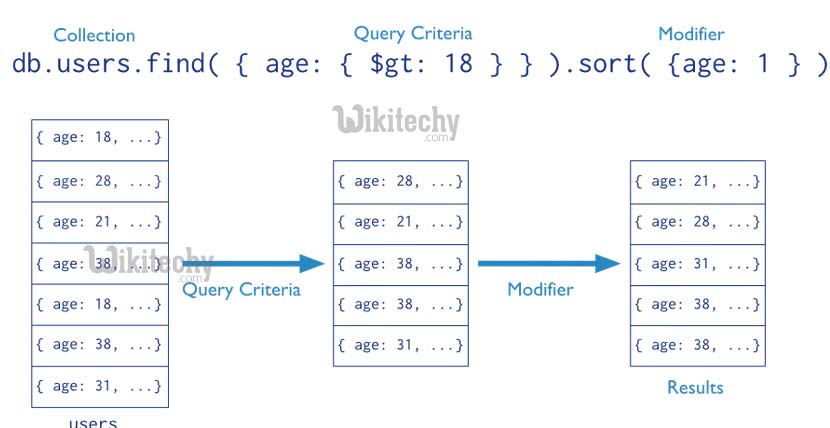
Learn Node js - node js Tutorial - MongoDB Find Query Sort - node - Node js Examples
Mongodb Find Query Explanation - In depth:
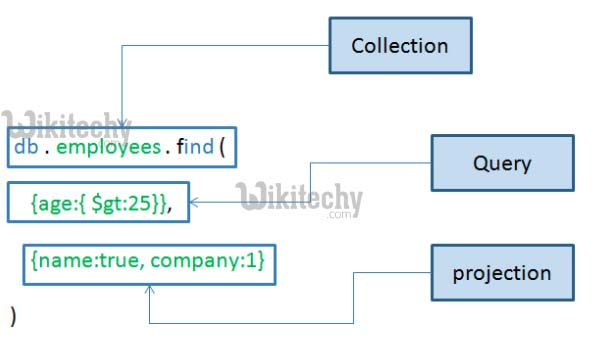
Learn Node js - node js Tutorial - MongoDB Find Query - node - Node js Examples
- db is a variable which refers to current database being used. employees is the collection on which find query is being fired just like FROM employees in SQL
- {age:{$gt: 25}} is the query criteria. Just like WHERE age > 25 clause in SQL
- {name: true, company:1} is the projection part of query. This tells the query to retrieve name and company of documents. It means that name and company fields should be selected and retrieved by the query
- But in the results you’ve seen _id field also selected, where did it come from ? By default mongodb find query always selects _id. So if we want to exclude it, then projection should be {_id:0}.
- This mongodb find query is equivalent to SELECT _id, name, company FROM Employee WHERE age > 25.
Read Also
Mongodb Data Types.Example
- Filter the records to retrieve the specific employee whose address is "Delhi"
- Create a js file named "query1.js", having the following code:
query1.js
var http = require('http');
var MongoClient = require('mongodb').MongoClient;
var url = "mongodb://localhost:27017/MongoDatabase";
MongoClient.connect(url, function(err, db) {
if (err) throw err;
var query = { address: "Delhi" };
db.collection("employees").find(query).toArray(function(err, result) {
if (err) throw err;
console.log(result);
db.close();
});
});
Open the command terminal and run the following command: Node query1.js
Output:

Learn Node js - node js Tutorial - filtering records in filter query - node - Node js Examples
Node.js MongoDB Filter With Regular Expression
- We can also use regular expression to find exactly what we need to search.
- Regular expressions can be used only to query strings.
Example
- Retrieve the record from the collection where address start with letter "L".Create a js file named "query2".
query2.js
var http = require('http');
var MongoClient = require('mongodb').MongoClient;
var url = "mongodb://localhost:27017/MongoDatabase";
MongoClient.connect(url, function(err, db) {
if (err) throw err;
var query = { address: /^L/ };
db.collection("employees").find(query).toArray(function(err, result) {
if (err) throw err;
console.log(result);
db.close();
});
});
Read Also
mongoDB - Create Table CollectionOpen the command terminal and run the following command: Node query2.js
Output:

Learn Node js - node js Tutorial - regular expressions in node.js filter query - node - Node js Examples