AngularJS <form> Directive
- AngularJS alters the default behavior of the <form> element
- Form Directive in AngularJS is used to create a FormController.
- The form is specified with the name attribute, then the form controller available on the current scope.
- In AngularJS forms are nested which means the outer form is valid when all the sub forms are said to be valid.
- Browser does not allow the nesting <form> elements.
- Angular delivers the ng-form directive, which acts similarly to the form but the form can be nested.
- Nested form is useful, for example: if the authority of the sub group control is needs to be determined the form.
- If the action attribute is not specified the forms in AngularJS prevented the default action when submitting the form to the server.
- While submitting the form there are two ways to specify the type of the javascript method they are:
- ng-submit – ngsubmit directive on the form element.
- ng-click - ngclick directive on the input field of type submit.
- To preventing the double execution of the handler the user can use only one directive either ng-submit or ng-click.
Syntax for form directive in AngularJs:
<form name="formname"></form>
Parameter Values:
Value | Description |
---|---|
name(optional) | Name of the form. Denotes the form controller will be published into related scope, under this name. |
Properties of the form directive in AngularJS application:
- AngularJS properties defines a current state of the form.
- The current value of each state denotes a Boolean value (i.e true or false)
Properties | Description |
---|---|
$pristine | Denotes no fields has not been modified. |
$dirty | One or more fields has been changed. |
$invalid | Content in the form is not valid. |
$valid | Content in the form is valid. |
$submitted | Content in the form is submitted. |
CSS Class Properties of the form directive in AngularJS application:
- AngularJS classes used to style forms according to their state and the classes are removed means if the current state value is false.
Properties | Description |
---|---|
ng-pristine | Denotes no fields has not been modified. |
ng-dirty | One or more fields has been changed. |
ng-invalid | Content in the form is not valid. |
ng-valid | Content in the form is valid. |
ng-valid-key | One key for each validation. (Example: more than one thing to be validated.) ng-valid-required |
ng-invalid-key | Example: ng-invalid-required |
Sample coding for form directive in AngularJs:
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
</head>
<body>
<p>Enter any name in input field:</p>
<form name="myForm">
<input name="ngInput" ng-model="ngInput" required>
</form>
<p>The forms's valid state is:</p>
<h1>{{myForm.$valid}}</h1>
</body>
</html>
Form directive in AngularJs:
- Form name is given as “myForm”
<form name="myForm">
<input name="ngInput" ng-model="ngInput" required>
</form>
Code Explanation for form directive in AngularJS:
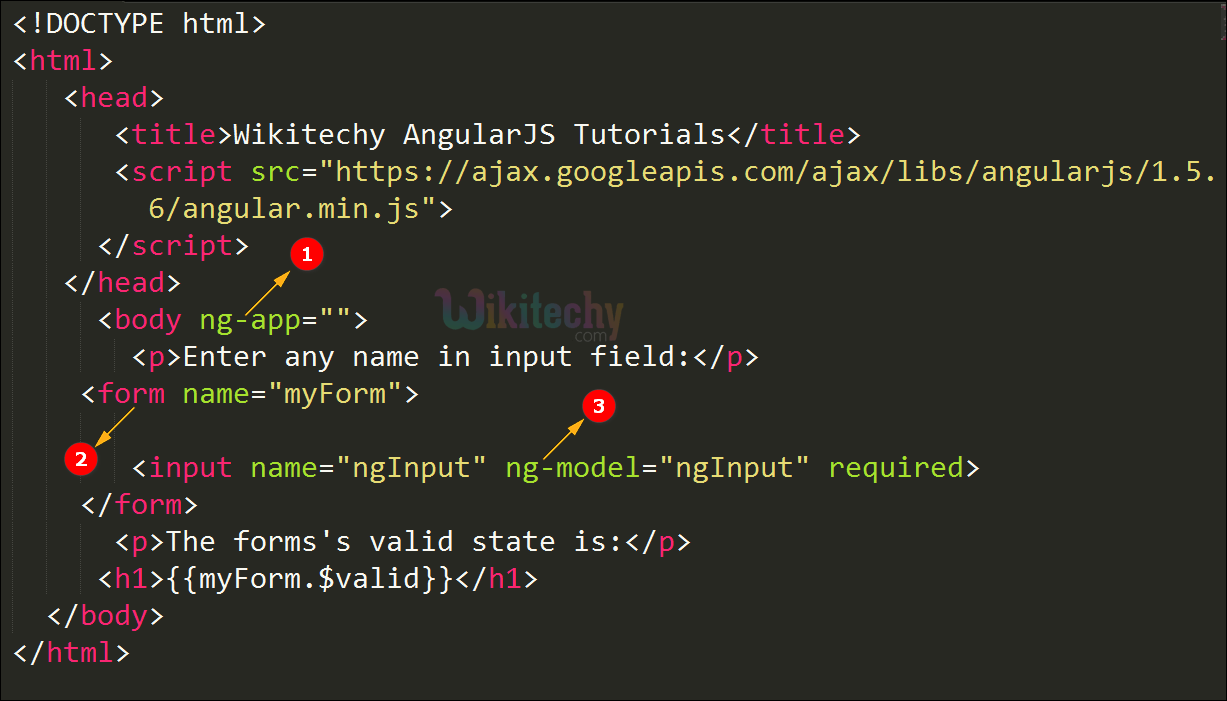
- The ng-app specifies the root element (e.g. <body> or <html> or <div> tags) to define AngularJS application.
- Form is used to specify the HTML form in AngularJS.
- ng-model binds the value from the HTML control to application data.
Sample Output for form directive in AngularJS:
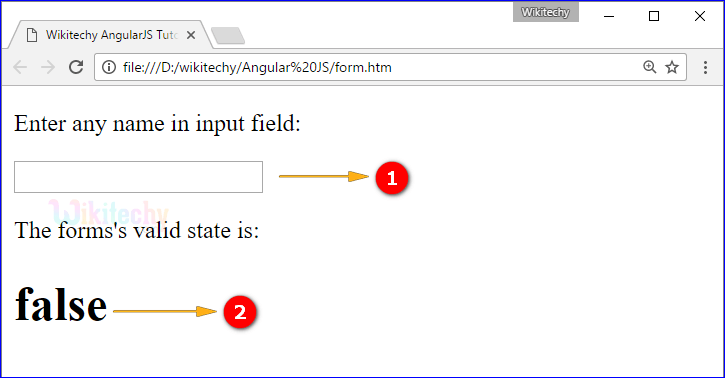
- The empty input field is displayed in the output.
- The form valid state is displayed as false because of the empty input field.
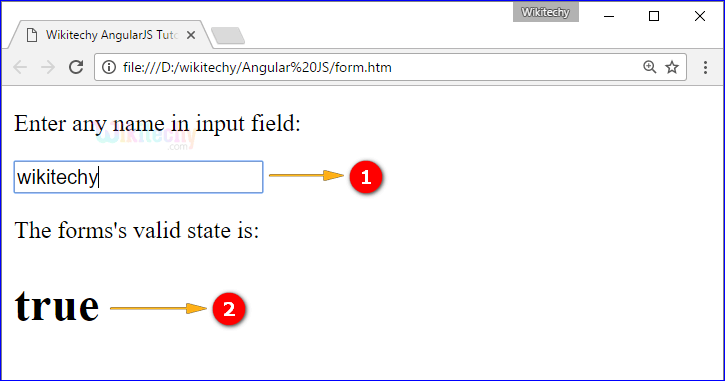
- We can type any name in the input field (here wikitechy is the name which is displayed in the output).
- The form valid state is displayed as True because of name in input field.
Sample coding for form directive with CSS Class in AngularJs:
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
</head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"></script>
<style>
form.ng-pristine {
background-color:blue;
}
form.ng-dirty {
background-color:green;
}
</style>
<body ng-app="">
<form name="myForm">
<p>Enter any name in input field:</p>
<input name="ngName" ng-model="ngName" required>
</form>
</body>
</html>
Code Explanation for form directive with CSS Class in AngularJs:
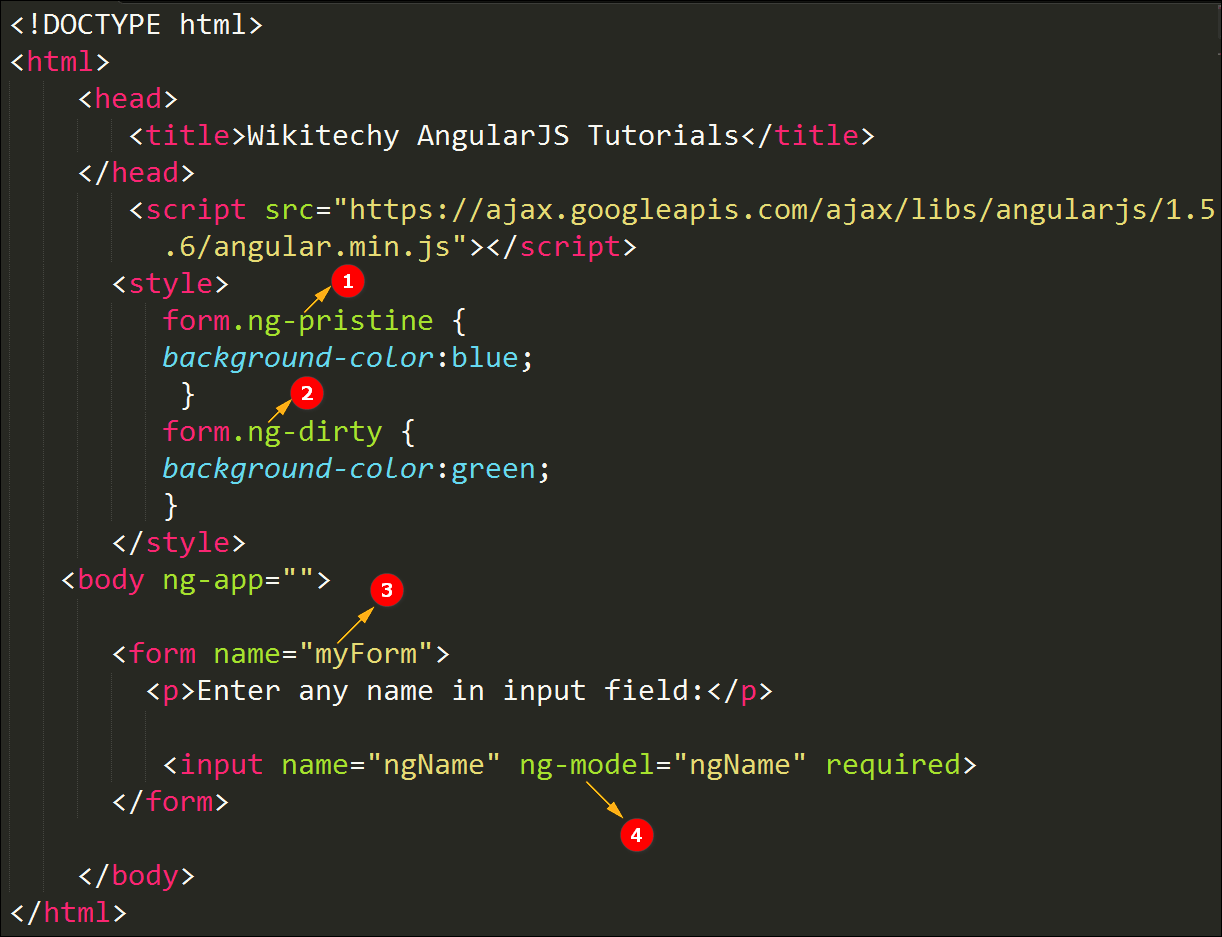
- ng-pristine is given for the model when the control has not been changed from the first compilation.
- ng-dirty is used to remove the ng-pristine class and sets into dirty state when the control has been changed from first compilation.
- myForm is given as form name of the Angular application.
- ng-model binds the value from the HTML control to application data.
Sample Output for form directive with CSS Class in AngularJs:
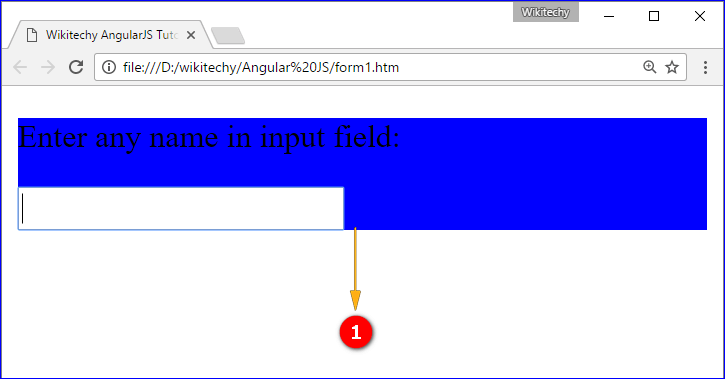
- The background color is displayed as blue in the output with empty input field.
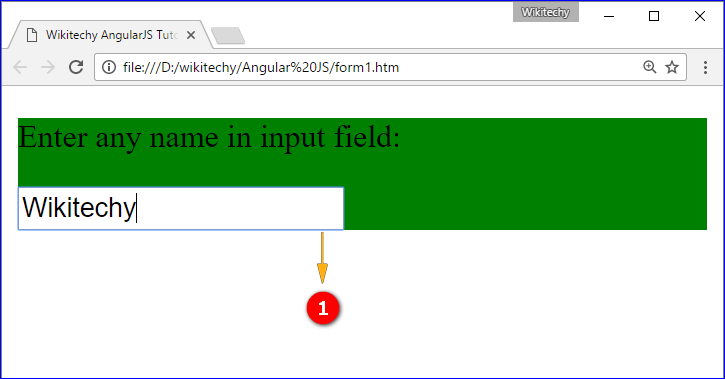
- The background color is displayed as green in the output when we type name in input field.