AngularJS ngMaxlength Directive
- The ng-maxlength directive in AngularJS used to restrict an input field with some limitation for the input fields.
- This directive defines the maximum number of characters allowed inside the input field.
- The ng-maxlength directive will not stop the users from typing more than the restricted number of characters, but the form will be invalid (i.e. form. $valid= false ).
- It is mainly used for text-based input controls but also applied for custom text-based controls.
Syntax for ng-maxlength directive in AngularJS:
<input type="text" ng-maxlength=”number”></input>
Parameter value for ng-maxlength directive in AngularJS:
Value | Description |
---|---|
number | Denotes the maximum number of characters legal for the input field. |
Sample coding for ng-maxlength directive in AngularJS:
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorial</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
</head>
<body>
<form ng-app="" name="form" ng-init="maxlength=5" >
<h3>ng-maxlength Directive example in AngularJS Tutorial</h3>
Set a maxlength <input type="number" ng-model="maxlength" /><br/><br/>
Enter the text or Number:<input type="text" ng-model="value"
name="input" ng-maxlength="maxlength" /><br/><br/>
<p>Form.input.$valid = {{form.input.$valid}}</p>
<p>Model = {{value}}</p>
</form>
</body>
</html>
Using ng-maxlength directive in AngularJS
<input type="text" ng-model="value" name="input" ng-maxlength="maxlength" /><br/><br/>
- Here ng-maxlength directive specifies the maximum number of characters allowed inside the input field.
Code Explanation for ng-maxlength directive in AngularJS:
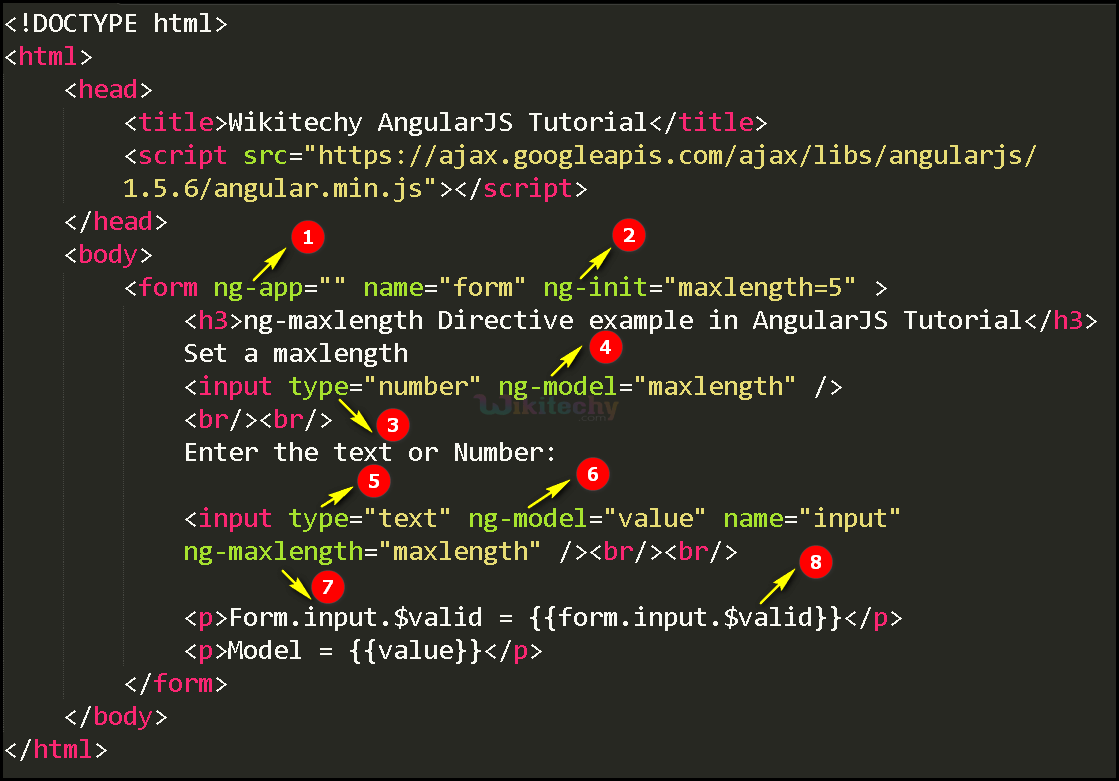
- The ng-app specifies the root element (e.g. <body> or <html> or <div> tags) to define AngularJS application.
- The ng-init is used to initialize the values for an application.(here the value 5 is mentioned for “maxlength”)
- “number” is declare the type value of the <input> tag.
- The ng-model bind an input field value to AngularJS application variable (“maxlength”).
- The “text” is declare the type value of the <input> tag.
- The ng-model bind an input field value to AngularJS application variable (“value”).
- The ng-maxlength directive used for specifies the maximum number of characters allowed in the input field. Here “maxlength” is declare the ng-maxlength value of the <input> tag.
- Form.input.$valid to checks the valid maxlength format or not. If the specified maxlength is correct and the output displays true otherwise false.
Sample Output for ng-maxlength directive in AngularJS:
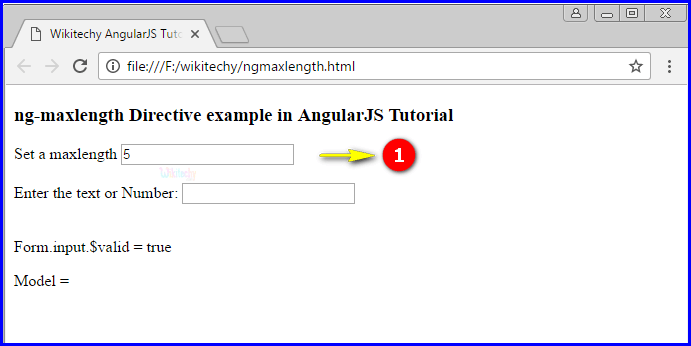
- The output displays the default value of maxlength as “5”.
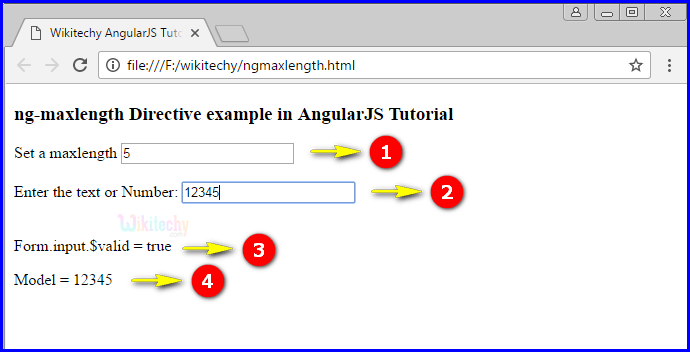
- The output displays the default value of maxlength as “5”.
- If the user type the number in the inputfield.
- The output displays the true because the maxlength value is 5.
- The output displays the model as “12345”.
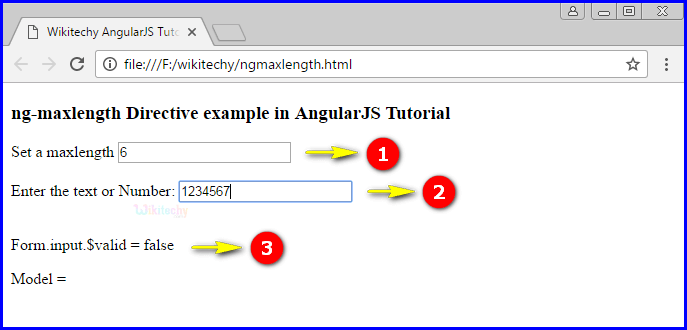
- If the user type the maxlength value is 6.
- If the user type the number in the inputfield.
- The output displays the false because the maxlength value is 6 but the user type the number in the input field as “1234567”.
Tips and Notes
- The ngMaxlength does not set the maxlength attribute.
- The ngMaxlength directive must be an expression, while the maxlength attribute value must be interpolated.