AngularJS Input Radio
- input [radio] is one of the AngularJS input directive in module ng.
- AnguarJS directive input [radio] is used to create an HTML radio button.
- radio button is a graphical element that allows the user to choose only one option.
- This directive executes at priority level 0.
Syntax for input [radio] directive in AngularJs:
<input type="radio"
ng-model="string"
value="string"
[name="string"]
[ng-change ="string"]
ng-value="string">
Parameter Values:
Parameter | Type | Description |
---|---|---|
ngModel | Defines angular expression to data-bind to. | |
value | To set the value to which the ngModel expression should be set when selected.value parameter only supports string values. | |
name (optional) | Name of the form under which the control is available | |
ngChange (optional) | An expression of Angular to be executed when input changes due to user interaction with the input element. | |
ngValue | In Angular expression to which ngModel will be set when the radio button is selected. Use ngModel instead of value attribute. |
Sample coding for input[radio] directive in AngularJS
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
</head>
<body>
<form ng-app="myApp" ng-controller="radioCtrl">
<h3>input[radio] directive example in AngularJS</h3>
<input type="radio" ng-model="choice" value="true">
Choice A :
<input type="radio" ng-model="choice" ng-value="specialValue" >
Choice B:
<input type="radio" ng-model="choice" value="false" >
Choice C:
<p>Value = {{choice }} </p>
</form>
<script>
var app = angular.module("myApp", []);
app.controller('radioCtrl', ['$scope', function($scope) {
$scope.specialValue = {
"id": "123",
"value": "true",
};
}]);
</script>
</body>
</html>
Code Explanation input[radio] directive in AngularJS:
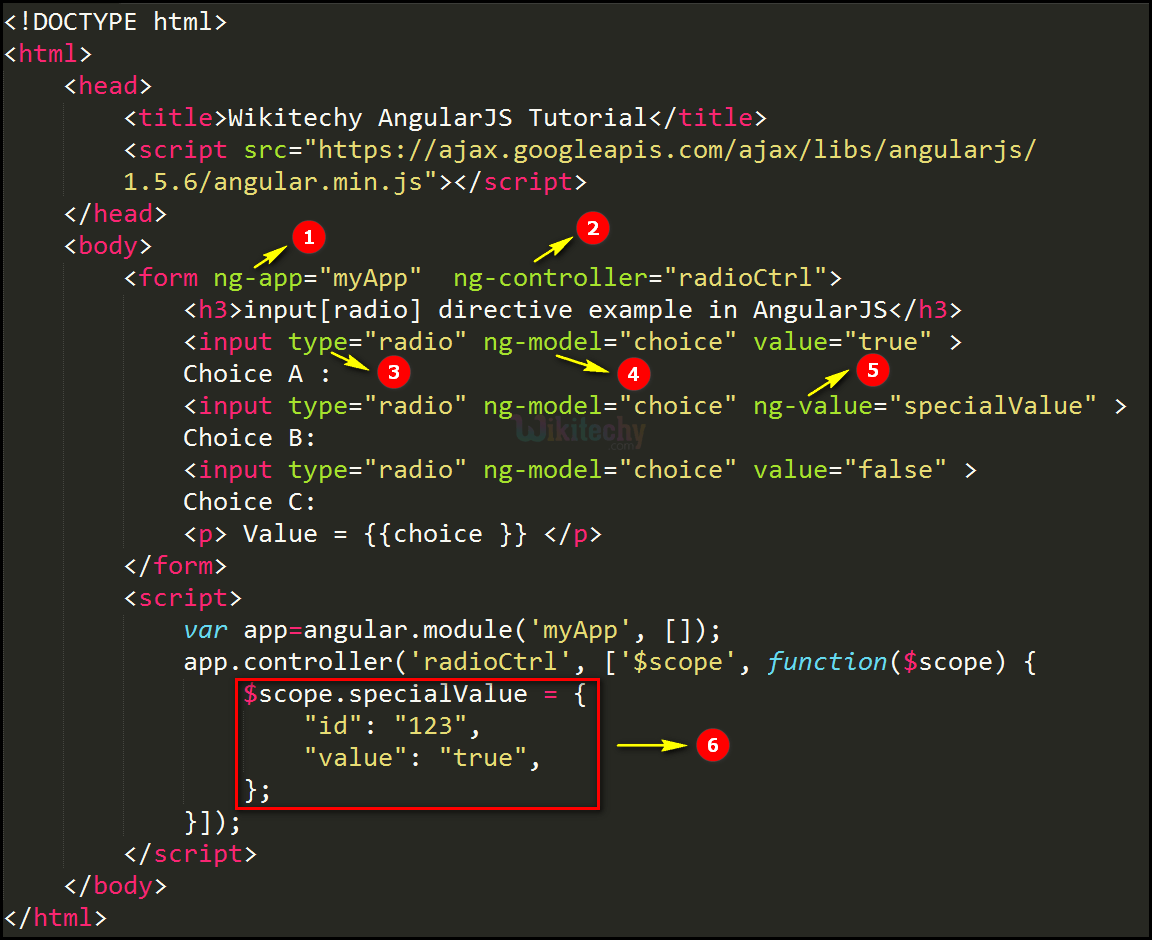
- The ng-app specifies the root element (“myapp”) to define AngularJS application.
- Ng-controller specifies the application controller in AngularJS the controller value is given as “radioCtrl”.
- “radio” is declare the type value of the <input> tag.
- The ng-model bind an input field value to AngularJS application variable (“choice”).
- ng-value is used of value of an input element. Here “specialValue” is declare the ng-value of an <input> tag.
- The value is given as { “id”=”123”, “value”=”true” } in the scope object.
Sample Output input[radio] directive in AngularJS:
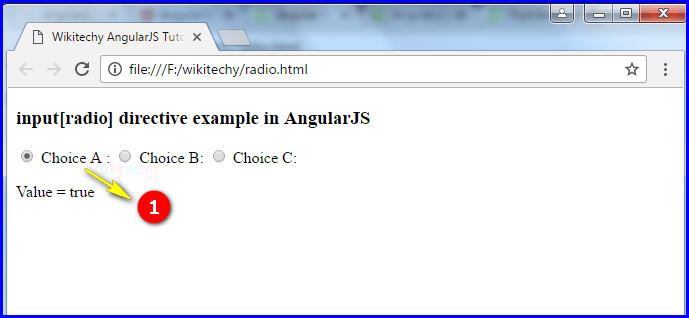
- If the user click the choice A button and the output displays value as “true”. since Value=”true” is given in the <input> tag.
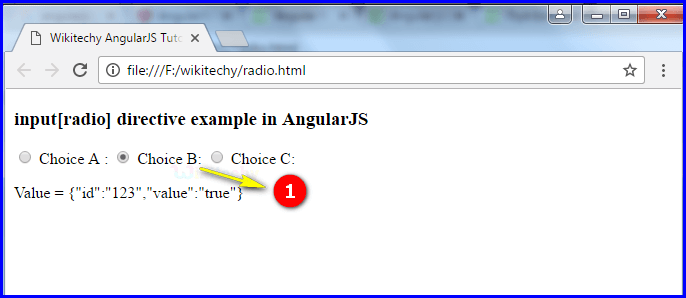
- If user click the choice B and the output will be displays as Value ={“id”:”123”,”value”:”true”} because here we declare the ng-value as a “specialValue”.
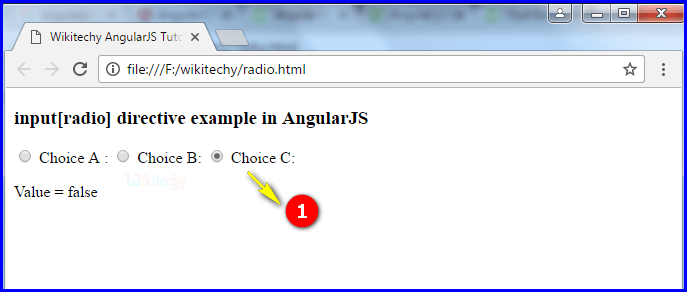
- If the user click the choice C button and the output displays value as “false” since Value=”false” is given in the <input> tag.