AngularJS Input Text
- input [text] is one of the AngularJS input directive in module ng.
- AnguarJS directive input [text] is used to create a standard HTML text input with angular data binding.
- It is inherited by the input elements.
- This directive executes at priority level 0.
Syntax for input [text] directive in AngularJS:
<input type="text"
[name="string"]
[required="string"]
[ng-required="string"]
[ng-minlength="number"]
[ng-maxlength="number"]
[pattern="string"]
[ng-pattern="string"]
[ng-change ="string"]
<ng-trim="boolean"]>
Parameter Values:
Parameter | Type | Description |
---|---|---|
ngModel | String | Defines angular expression to data-bind to. |
name (optional) | String | Name of the form under which the control is available |
required (optional) | String | Denotes the required validation error key if the value is not entered. |
ngRequired (optional) | String | Denotes required attribute and required validation limit to the element when the ngRequired expression evaluates to true. Instead of required use ngRequired for data-bind to the required attribute. |
ngMinlength(optional) | number | States the minlength validation error key if the value is shorter than minlength. |
ngMaxlength(optional) | number | States the maxlength validation error key if the value is longer than maxlength. |
pattern(optional) | string | It is related to ngPattern except that the attribute value is the actual string. It contains the regular expression body that is converted to a regular expression as in the ngPattern directive. |
ngPattern(optional) | String | States pattern validation error key if the ng model value does not match RegExp found by evaluating the Angular expression given in the attribute value. If the expression evaluates to a RegExp object, then this is used directly. If the expression evaluates to a string, then it will be converted to a RegExp after wrapping it in ^ and $ characters. For instance, "abc" will be converted to new RegExp ('^abc$'). |
ngChange(optional) | String | An expression of Angular to be executed when input changes due to user interaction with the input element. |
ngTrim (optional) | boolean | If set to false Angular will not automatically trim the input. Parameter is ignored for input [type=password] controls, which will never trim the input and the default value is true. |
Sample coding for input[text] directive in AngularJS
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
</head>
<body>
<form ng-app="myApp" name="Form" ng-controller="TextCtrl">
<h3>input[text] directive example in AngularJS</h3>
Single word:
<input type="text" name="input" ng-model="text"
ng-pattern="/^[a-zA-Z]+$/" ng-trim="false" required >
<span ng-show="Form.input.$error.required"><br/ ><br/ >
Required!</span>
<span ng-show="Form.input.$error.pattern"><br/ ><br/ >
Single Word only!</span>
<p>text = {{ text }}</p>
<p>Form.input.$valid = {{ Form.input.$valid }}</p>
<p>Form.input.$error = {{ Form.input.$error }}</p>
<p>Form.$valid = {{ Form.$valid }}</p>
<p>Form.$error.required = {{ !!Form.$error.required }}</p>
</form>
<script>
var app = angular.module("myApp", []);
app.controller('TextCtrl', function($scope) {
}]);
</script>
</body>
</html>
Code Explanation input [range] directive in AngularJS:
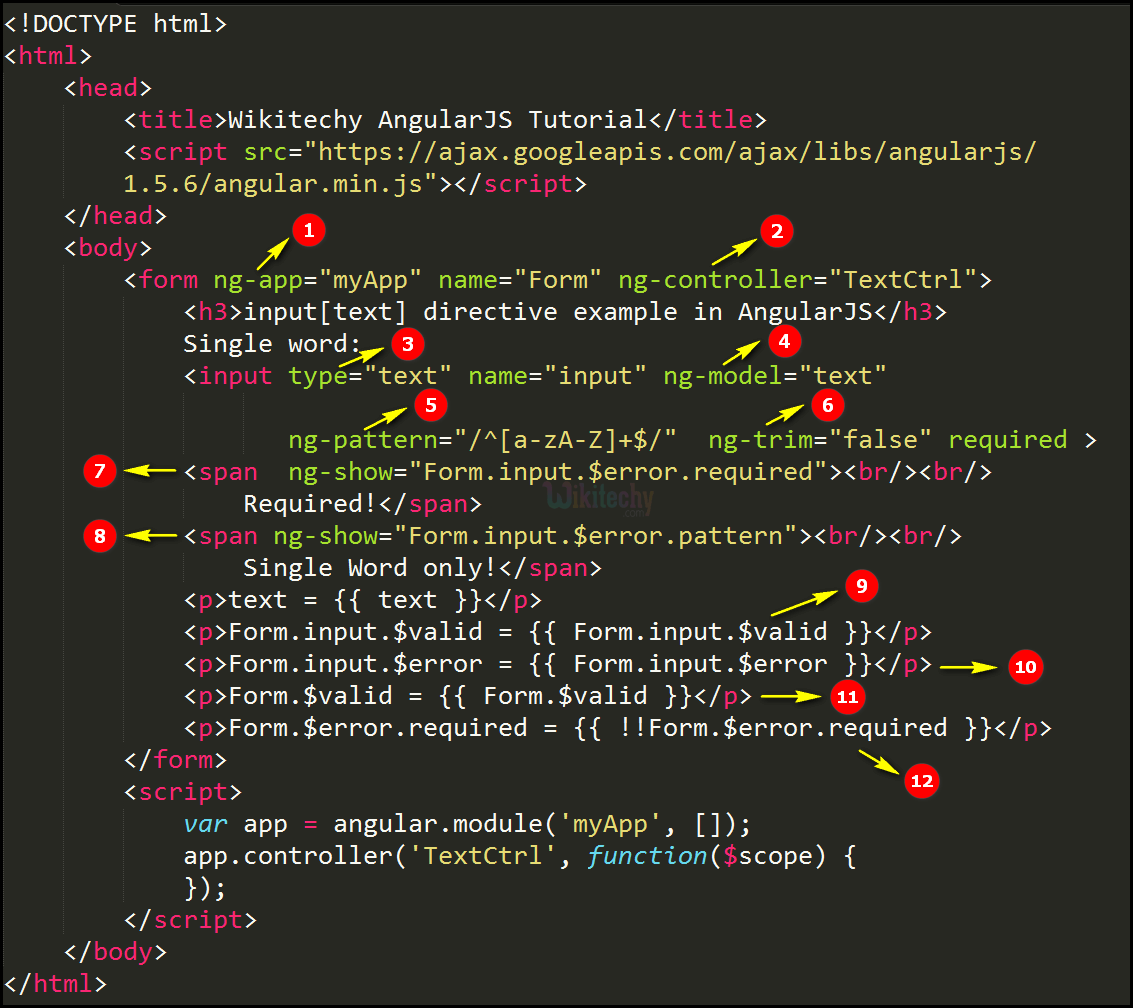
- The ng-app specifies the root element (“myapp”) to define AngularJS application.
- ng-controller specifies the application controller in AngularJS the controller value is given as “TextCtrl”.
- “text” is declare the type value of the <input> tag.
- The ng-model bind an input field value to AngularJS application variable (“text”).
- ng-pattern is used to specify pattern model (like “/^[a-zA-Z]+$/”)
- ng-trim=”false” means it is not automatically trim in the input.
- ng-show directive is used to hides HTML elements. If the required directive shows an error the content is displayed like “Required”
- ng-show directive is used to hides HTML elements. If the required directive shows an error the content is displayed like “Single Word Only”.
- Form.input.$valid to checks the valid text or not. If the text in the input field then the output will be displays as true otherwise false.
- Form.input.$error to check whether the valid text or not .If the text specified in error it through the exception (Like “required”=”true) otherwise it is an empty curly braces ( { } ).
- Form.$valid is used to check whether the form is valid or not and output will be displayed in <p> tag.
- Form.error.$required is used to text is required or not. If the text is required and output will be displayed as false otherwise true.
Sample Output input[text] directive in AngularJS:
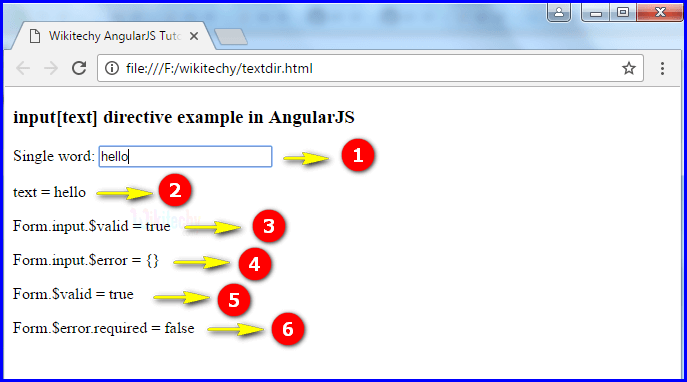
- If the user type any text in the input field.
- The output will be displays as text=hello
- The output displays true because it is consider as a text.
- The output displays empty curly braces it means does not thrown any error.
- The output displays true it is valid text.
- If the text box does not empty so the output displays as false.
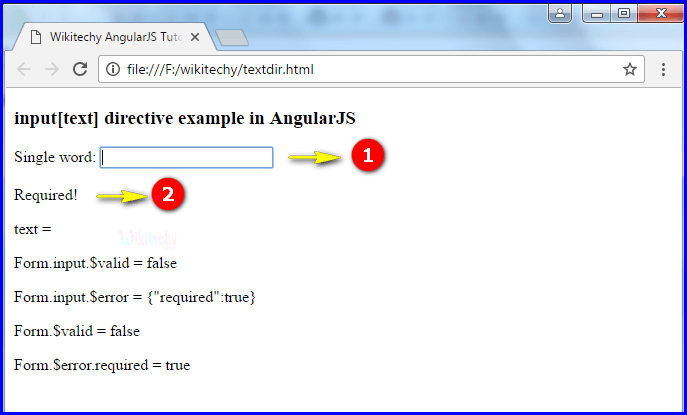
- If the user does not type any text in the input field.
- The output shows an error “Required”.
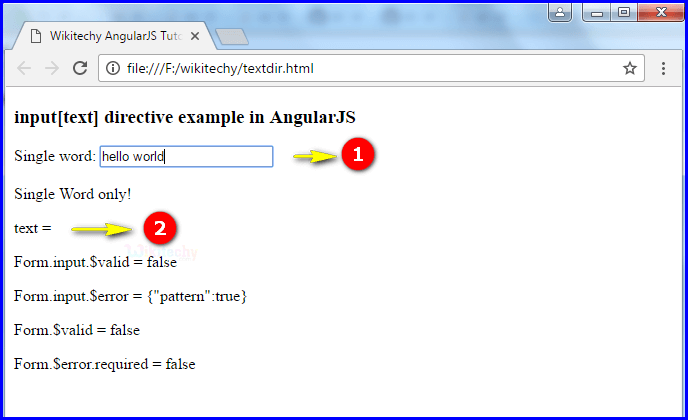
- If the user type double word in the input field.
- The output displays as empty (text=)
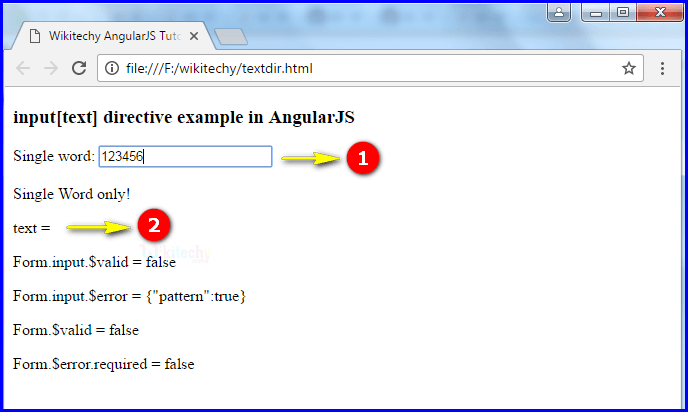
- If the user type any number in the input field.
- The output displays as empty (text=) because the pattern is (a-z).