AngularJS <textarea> Directive
- AngularJS alter the default behavior of the <textarea> element, when the ng-model attribute is present.
- HTML textarea element is control with AngularJS data-binding which means they are part of the ng-model and it can be referred and updated both in the AngularJS functions and in the DOM.
- The databinding and validation properties of this element are same as the input element.
- A textarea provides the validation, when the required attribute value is empty the $valid state shows in false condition.
- AngularJS textarea directive provides the input state control which is holds the current state of all the input elements.
- Textarea represents the value of each state is Boolean value and it either true or false.
Syntax for textarea directive in AngularJS:
<textarea ng-model="name"></textarea>
Properties of the textarea directive in AngularJS application:
Properties | Description |
---|---|
$pristine | Denotes no fields has not been modified. |
$dirty | One or more fields has been changed. |
$invalid | Content in the form is not valid. |
$valid | Content in the form is valid. |
$touched | Denotes that the field has been touched |
$untouched | Denotes that the field has been not touched |
CSS Class Properties of the textarea directive in AngularJS application:
Properties | Description |
---|---|
ng-pristine | Denotes no fields has not been modified. |
ng-dirty | One or more fields has been changed. |
ng-invalid | Content in the form is not valid. |
ng-valid | Content in the form is valid. |
ng-valid-key | One key for each validation. (Example: more than one thing to be validated.) ng-valid-required |
ng-invalid-key | Example: ng-invalid-required |
ng-invalid-key | Example: ng-invalid-required |
$touched | Denotes that the field has been touched |
$untouched | Denotes that the field has been not touched |
Sample coding for textarea directive in AngularJS:
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
<style>
textarea.ng-invalid {
background-color:yellow;
}
textarea.ng-valid {
background-color:pink;
}
</style>
</head>
<body ng-app="">
<h3>textarea Directive in AngularJS Tutorial</h3>
<p>Type any content inside the textarea</p>
<textarea ng-model="myTextarea" required > </textarea>
<p>The content of the textarea is:</p>
<h1>{{myTextarea}}</h1>
</body>
</html>
Textarea directive in AngularJS:
<textarea ng-model="myTextarea" required ></textarea>
Using CSS properties for Textarea directive in AngularJS:
<style>
textarea.ng-invalid {
background-color:yellow;
}
textarea.ng-valid {
background-color:pink;
}
</style>
Code Explanation for textarea directive in AngularJS:
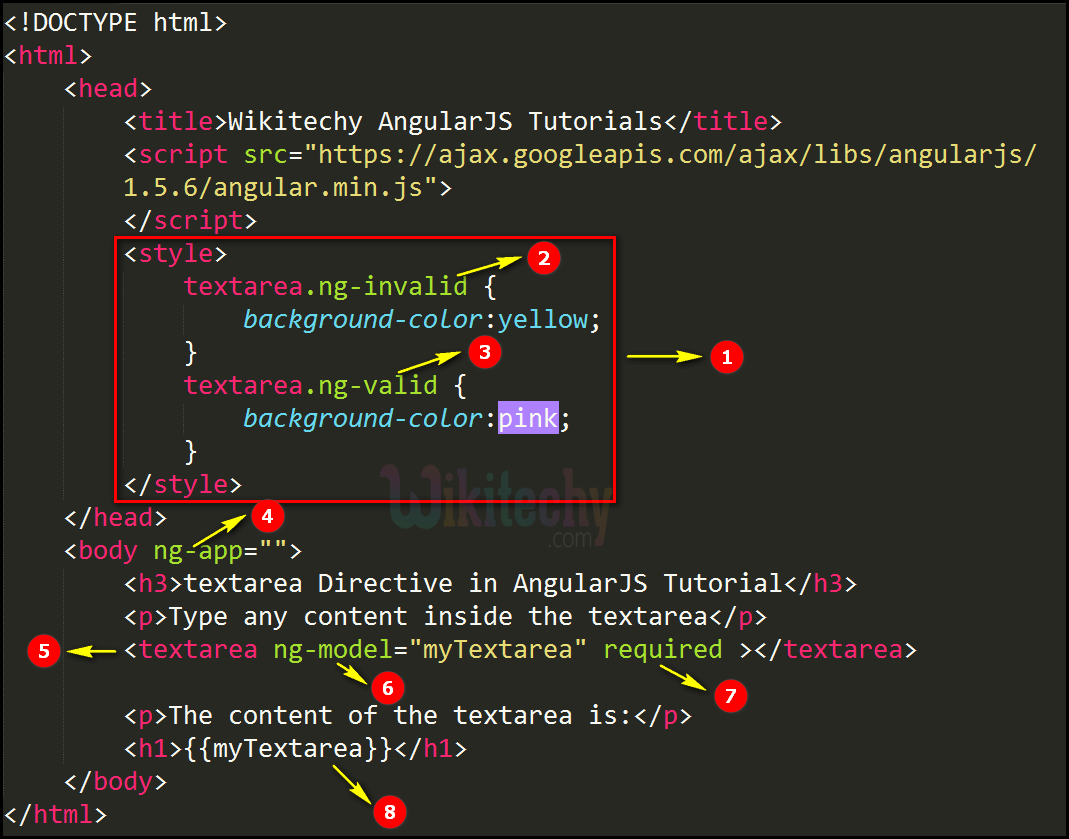
- <style> tag is used to set the background color of the textarea elements.
- The CSS classes for the textarea.ng-invalid class is used to declare the background color is yellow. (Here the textarea background color is yellow which means the textarea is notvalid.)
- textarea.ng-valid class is define a background color is pink.(here the texarea is pink which means the textarea is a valid.
- The ng-app specifies the root element (<div>) to define AngularJS application.
- <textarea> tag is used to get multiline text in the input field
- The ng-model bind an input field value to AngularJS application variable (“myTextarea”).
- The required is used to display the content in the textarea field.
- The expression {{ myTextarea }} is bind the value of mytextarea variable.
Sample Output for textarea directive in AngularJS:
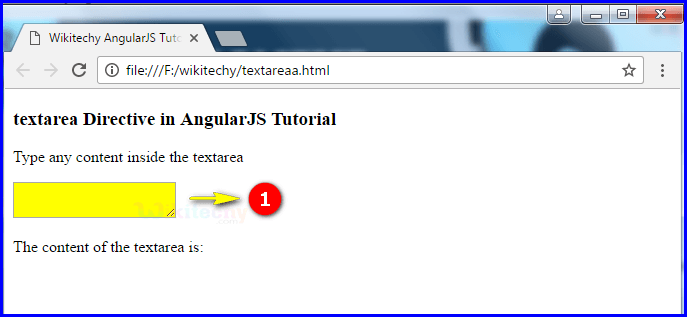
- The textarea is displayed on the page load and the output displays the yellow color which means the textarea is not valid.
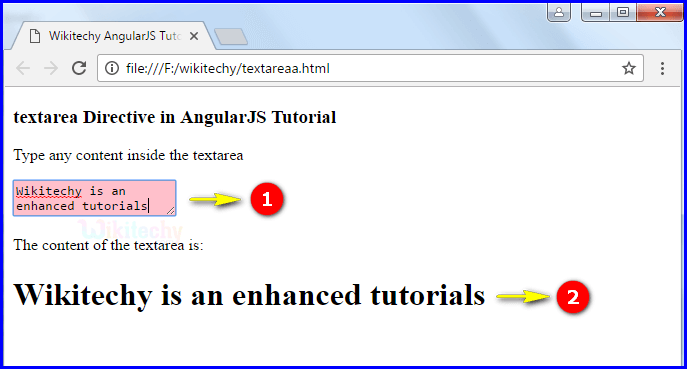
- If the user enters the text in the input field and the color will be changes to pink which means textarea field is a valid state using CSS property.
- The content inside in the textarea is displayed below the textarea box as a heading.