AngularJS ngrequired
- The ng-required directive in AngularJS used to sets the required attribute of a form field (Like input or textarea).
- It specifies the required attribute of an element.
- The form field will be required if the expression inside the ng-required attribute returns true.
- It mainly used for input, textarea and select controls, but it can also use for custom controls
- The ng-required directive is necessary to move the value between true and false.
- In HTML, we cannot set the required attribute value to false (The presence of required attribute makes the element value is required)
- This directive executes at priority level 0.
Syntax for ng-required directive in AngularJS:
<input ng-required=”expression” ></input>
Parameter Values:
Value | Description |
---|---|
expression | Denotes an expression will set the required attribute if it returns the true value. |
Sample coding for ng-required directive in AngularJS:
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"></script>
</head>
<body>
<form ng-app="myApp" name="form" ng-controller="requiredCtrl">
<h3>ng-required Directive in AngularJS Tutorial</h3>
Toggle required:
<input type="checkbox" ng-model="required"><br>
Enter the text:
<input type="text" ng-model="model" name="input"
ng-required="required" /><br>
<p>form.input.$error.required = {{ form.input.$error.required }}</p>
<p>model = {{model}}</p>
</form>
<script>
var app=angular.module('myApp', []);
app. controller('requiredCtrl', ['$scope', function($scope) {
$scope. required = true;
}]);
</script>
</body>
</html>
Code Explanation for ng-required directive in AngularJS:
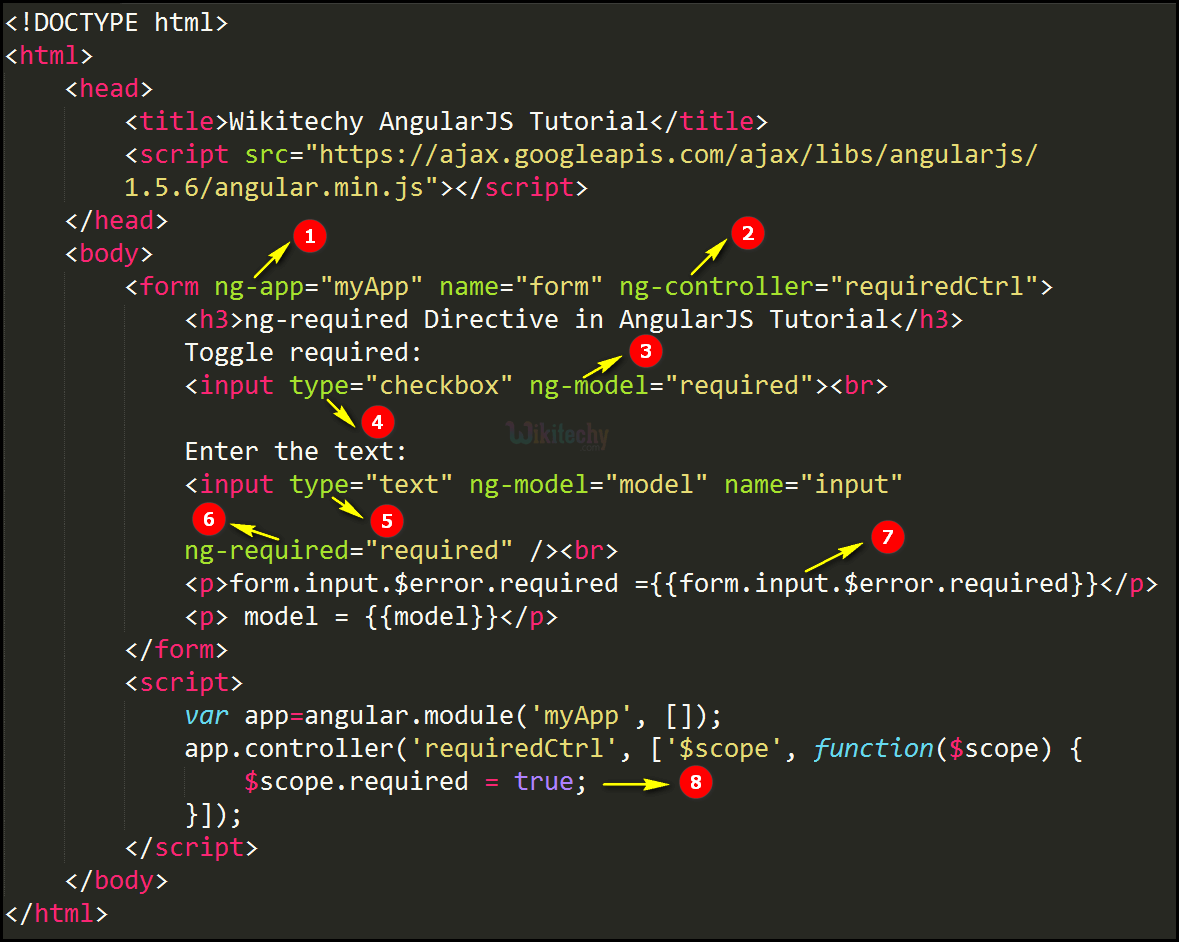
- The ng-app specifies the root element (“myApp”) to define AngularJS application.
- The ng-controller specifies the application controller in AngularJS the controller value is given as “requiredCtrl”.
- The ng-model bind an input field value to AngularJS application variable (“required”).
- The “checkbox” is declare the type value of the <input> tag.
- The “text” is declare the type value of the <input> tag.
- The ng-required directive sets the required attribute of an input field. Here sets the ng-required directive to “required”.
- Form.input.$error.required is used to text is required or not. If the text is required and the output will be displays true.
- The value is given as true in the scope object.
Sample Output for ng-required directive in AngularJS:
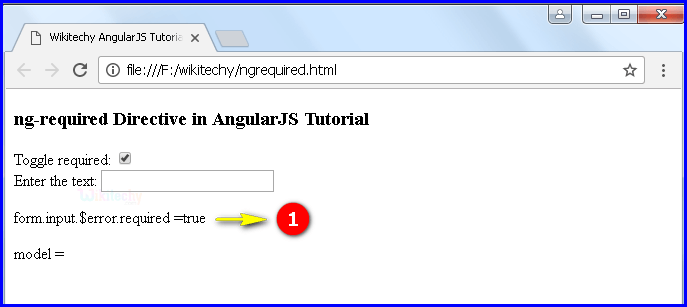
- The output displays the default value of true because the text is required in the input field.
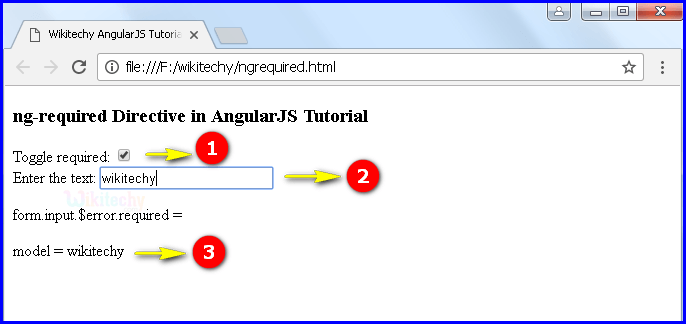
- The output shows the user click the checkbox
- If the user type text in the input field as “wikitechy”.
- The output displays the model as “wikitechy” because the required attribute is false.