AngularJS ngswitch
- The ng-switch directive in AngularJS identifies a condition that is used to show/hide child elements depending on an expression.
- HTML elements only with in the ng-switch but without ng-switch-when or ng-switch-default directives will be maintained at the location as specified in the pattern.
- If the expression gets a match, then the child elements with the ng-switch-when directive will be displayed. Otherwise the child element will be removed.
- If the matching expression is not found in the elements, then the ng-switch-default attribute will be displayed.
- It is supported by all HTML elements.
Syntax for ng-switch Directive in AngularJS:
<element ng-switch="expression">
<element ng-switch-when="value"></element>
<element ng-switch-when="value"></element>
<element ng-switch-when="value"></element>
<element ng-switch-default="value"></element>
</element>
Parameter Values:
Parameter | Type | Description |
---|---|---|
ng-switch | * | States the expression to match against ng-switch-when. Ng-switch-when: If the elments gets match then the ng-switch-when directive will be displayed. If the match elements appears multiple times, all the elements will be displayed using ngswitchWhenSeparator attribute. Ng-switch-default: If no other elments gets match, then the default case will be displayed. |
Sample code for ng-switch Directive in AngularJS:
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"></script>
</head>
<body ng-app="">
<h3>ng-switch Directive in AngularJS Tutorial</h3>
Tutorials list:
<select ng-model="topic">
<option value="html">HTML
<option value="css">CSS
<option value="php">PHP
</select>
<div ng-switch="topic">
<div ng-switch-when="html">
<h1>HTML</h1>
<p><b>HTML</b> is a standard markup language for
creating web pages</p>
</div>
<div ng-switch-when="css">
<h1>CSS</h1>
<p><b>CSS</b> is used to design style for a web page.</p>
</div>
<div ng-switch-when="php">
<h1>PHP</h1>
<p><b>Hypertext Preprocessor</b> is used to
create dynamic Web pages.</p>
</div>
<div ng-switch-default>
<h1>Switch</h1>
<p>Select topic from the dropdown, to switch the content.</p>
</div>
</div>
</body>
</html>
Use ng-switch Directive in AngularJS:
<div ng-switch="topic">
<div ng-switch-when="html">
<h1>HTML</h1>
<p><b>HTML</b> is a standard markup language for creating web pages</p>
</div>
<div ng-switch-when="css">
<h1>CSS</h1>
<p><b>CSS</b> is used to design style for a web page.</p>
</div>
<div ng-switch-when="php">
<h1>PHP</h1>
<p><b>Hypertext Preprocessor</b> is used to create dynamic Web pages.</p>
</div>
<div ng-switch-default>
<h1>Switch</h1>
<p>Select topic from the dropdown, to switch the content.</p>
</div>
</div>
- Ng-switch specifies a condition that will be used to show/hide child elements.
Use ng-switch-when Directive in AngularJS:
<div ng-switch-when="html">
<h1>HTML</h1>
<p><b>HTML</b> is a standard markup language for creating web pages</p>
</div>
<div ng-switch-when="css">
<h1>CSS</h1>
<p><b>CSS</b> is used to design style for a web page.</p>
</div>
<div ng-switch-when="php">
<h1>PHP</h1>
<p><b>Hypertext Preprocessor</b> is used to create dynamic Web pages.</p>
</div>
- If the expression gets a match, then the child elements with the ng-switch-when directive will be displayed.
Use ng-switch-default Directive in AngularJS:
<div ng-switch-default>
<h1>Switch</h1>
<p>Select topic from the dropdown, to switch the content.</p>
</div>
- If the matching expression is not found in the elements, then the ng-switch-default attribute will be displayed.
Code Explanation for ng-switch Directive in AngularJS:
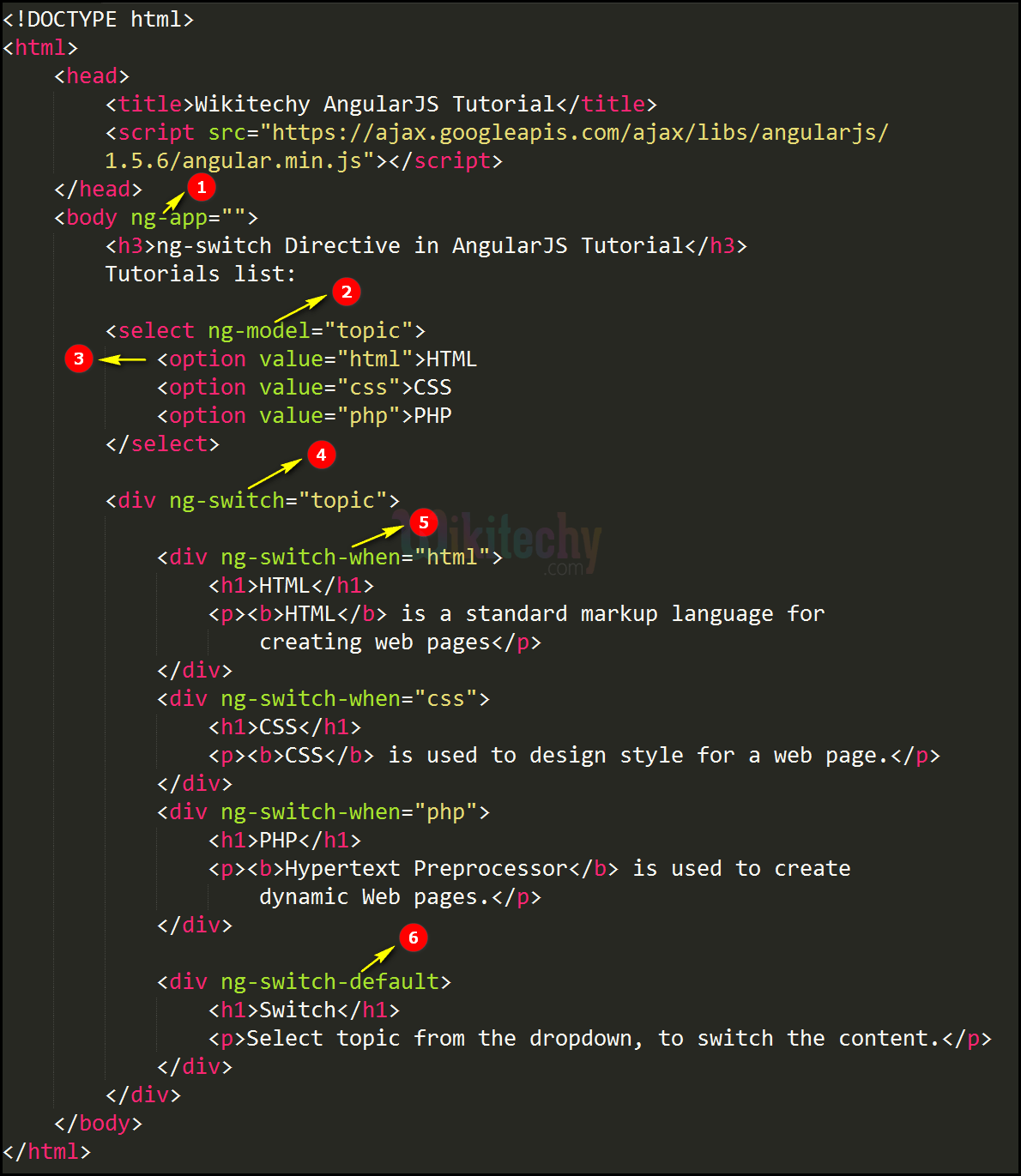
- The ng-app specifies the root element (<div>) to define AngularJS application.
- The ng-model bind an input field value to AngularJS application variable (“topic”).
- <option> tag is used to described an option in a select list such as HTML, CSS and PHP.
- The child elements of the HTML, CSS and PHP gets match with in the ng-switch directive (ng-switch=”topic”) then the ng-switch-when directive will be displayed.
- If the child elements are not in the option list, then the default value of ng-switch-default directive will be displayed.
Sample Output for ng-switch Directive in AngularJS:
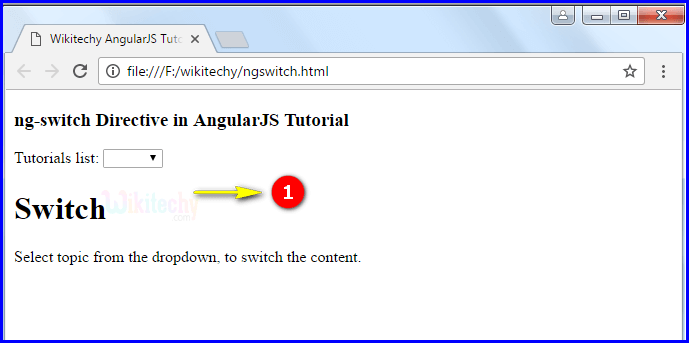
- When the page is loads the default value of switch option will be displayed.
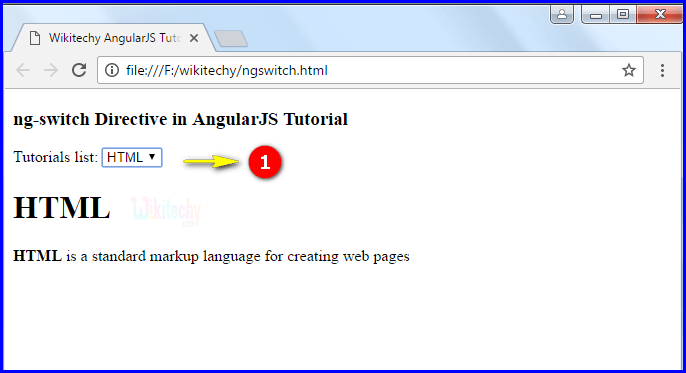
- If the user selects the HTML option which shows the child element HTML content will be displayed.