AngularJS Input Week
- input [week] is one of the AngularJS input directive in module ng.
- AnguarJS directive input [week] is used to create an HTML input with week-of-the-year validation and transformation to Date.
- The input text must be entered in the format ISO-8601 ( i.e. yyyy-W##)
- In browsers does not yet support the HTML5 time input, instead text element will be used in a valid ISO-8601 local time format.
- The data model must be date object.
- The timezones is used to read/write the Date instance in the model by using ngModelOptions.
- This directive executes at priority level 0.
For ex: 2016-W05.
Syntax for input [week] directive in AngularJS:
<input type="week"
ng-model="string"
[name="string"]
[min="string"]
[max="string"]
[ng-min=" "]
[ng-max=" "]
[required ="string"]
[ng-required ="string"]
[ng-change ="string”]>
Parameter Values:
Parameters | Type | Description |
---|---|---|
ngModel | String | Defines angular expression to data-bind to. |
name (optional) | String | Name of the form under which the control is available |
min (optional) | String | To set the min validation error key if the value entered is shorter than min. (e.g “{{ minWeek | date :’yyyy-Www’ }}”) |
max (optional) | String | To set the max validation error key if the value entered is greater than max. (e.g “{{ maxWeek| date : ’yyyy-Www’ }}”) |
ngMin (optional) | date, string | To set the min validation constraint to the Date/ISO week string the ngMin expression calculates to. Reminds that it does not set the min attribute. |
ngMax(optional) | date, string | To set the max validation constraint to the Date/ISO week string the ngMax expression calculates to. Reminds that it does not set the max attribute. |
required (optional) | string | Denotes the required validation error key if the value is not entered. |
ngRequired(optional) | String | Sets the required attribute and required validation constraint to the element when the ngRequired expression sets to true. Instead of required use ngRequired when we want data-bind to the required attribute. |
ngChange(optional) | String | An expression of Angular to be executed when input changes due to user interaction with the input element. |
Sample coding for input [week] directive in AngularJS
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
</head>
<body>
<form ng-app="myApp" name="Form" ng-controller="WeekCtrl">
<h3>input[week] directive example in AngularJS</h3>
Pick a date in 2016:
<input type="week" name="input" ng-model="value"
placeholder="YYYY-W##" min="2015-53" max="2016-W52" required />
<span ng-show="Form.input.$error.required">
Required!</span>
<span ng-show="Form.input.$error.week">
Not a valid Week!</span>
<p>Date = {{value | date: "yyyy-Www"}}</p>
<p>Form.input.$valid = {{Form.input.$valid}}</p>
<p>Form.input.$error = {{Form.input.$error}}</p>
<p>Form.$valid = {{ Form.$valid }}</p>
<p>Form.$error.required = {{ !!Form.$error.required }}</p>
</form>
<script>
var app = angular.module("myApp", []);
app.controller('WeekCtrl', function($scope) {
}]);
</script>
</body>
</html>
Code Explanation input [week] directive in AngularJS:
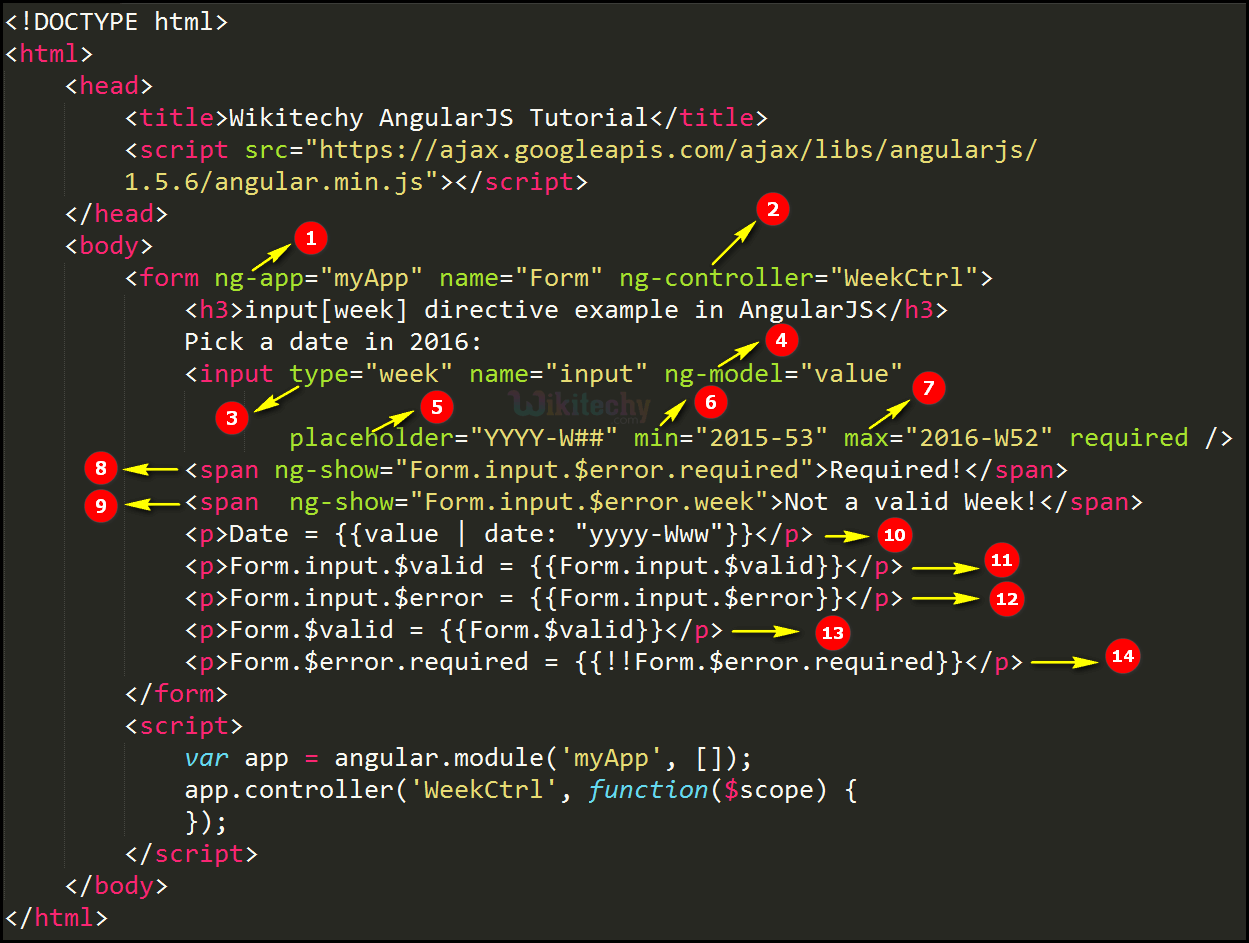
- The ng-app specifies the root element (“myapp”) to define AngularJS application.
- Ng-controller specifies the application controller in AngularJS the controller value is given as “WeekCtrl”.
- “week” is declare the type value of the <input> tag.
- The ng-model bind an input field value to AngularJS application variable (“value”).
- Placeholder is used to declare the time format ( Like”YYYY-W##”).
- min parameter is used to declare the start week value(2015-53)
- max parameter is used to declare the end week value(2016-52)
- ng-show directive is used to hides HTML elements. If the required directive shows an error the content is displayed like “Required”
- ng-show directive is used to hides HTML elements. If the required directive shows an error the content is displayed like “Not Valid week!”.
- Here the date filter formats a date into specified format (YYYY-Www) and the output will be updated in the <p> tag.
- Form.input.$valid to checks the correct week format or not. If the user scroll week and year in the input field then the output will be displays as true otherwise false.
- Form.input.$error to check whether the valid week format or not .If the url specified in error it throw an exception (Like “required”=”true) otherwise it is an empty curly braces ( { } ).
- Form.$valid is used to check whether the form is valid or not and output will be displayed in <p> tag.
- Form.$error.required is used to check whether week is required or not. If the week is required and output will be displays as false otherwise true.
Sample Output input[week] directive in AngularJS:
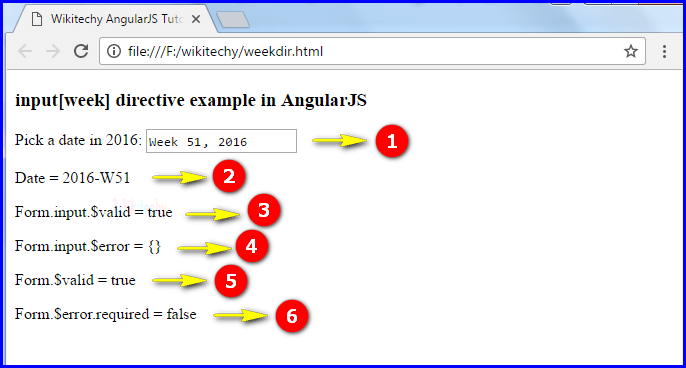
- If the user scroll the week and year in the input field.
- The output displays as Date=2016-W51.
- The output displays true because it is consider as a week.
- The output displays empty curly braces it means does not thrown any error.
- The output displays true it is valid date.
- If the text box does not empty so the output displays as false.
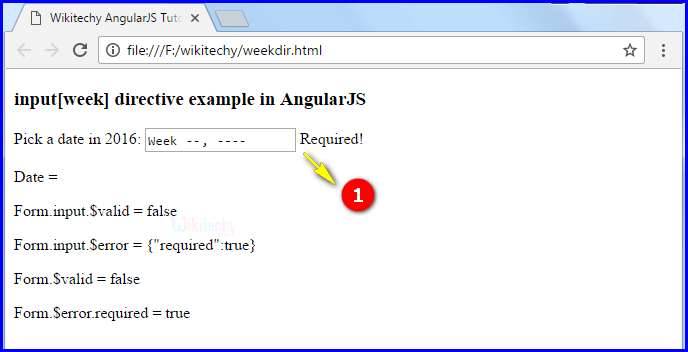
- If the user does not scroll any value in the input field and the output shows an error “Required”.
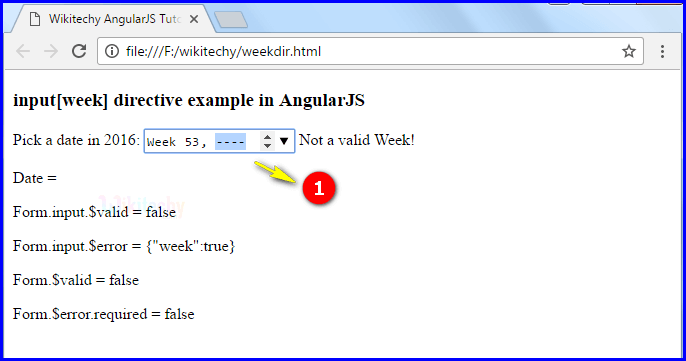
- If the user does not type year in the input field and the output displays as Not a valid Week!.