AngularJS ngbindhtml
- The ng-bind-html directive evaluate the expression and insert the HTML element in a secure way.
- Resulting HTML content will be sanitized using the $sanitize service.
- We should ensure that $sanitize is available, for example, by including ngSanitize in your module's dependencies.
- It binds the innerHTML of an HTML element to an application data, and always removes dangerous code from HTML string.
- ng-bind-html directive supported by all HTML elements.
- To use ngSanitize function in our code, we need to include “angular-sanitize.js” module in your application.
- We can bypass sanitization for values which is safe. To do, bind to an explicitly trusted value through $sce.trustAsHtml.
- Bypassing the trusted value is called Strict Contextual Escaping (SCE).
Syntax for ng-bind-html directive in AngularJS:
<element ng-bind-html="expression"></element>
Parameter Values:
Value | Description |
---|---|
expression | Denotes a variable, or an expression to evaluate. |
Sample coding for ng-bind-html directive in AngularJS:
Tryit<!DOCTYPE html>
<html>
<head>
<title>Wikitechy AngularJS Tutorials</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular.min.js"> </script>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.6/
angular-sanitize.js"> </script>
</head>
<body>
<div ng-app="myApp" ng-controller="ngbindCtrl">
<h3>ng-bind-html Directive example in AngularJS</h3>
<p ng-bind="value"></p>
<p ng-bind-html="value"></p>
</div>
<script>
var app = angular.module("myApp", ['ngSanitize']);
app.controller("ngbindCtrl", function($scope) {
$scope.value = "<b>Welcome to</b>" +
"<a href= http://www.wikitechy.com/>Wikitechy.com</a>";
}]);
</script>
</body>
</html>
Code Explanation for ng-bind-html directive in AngularJS:
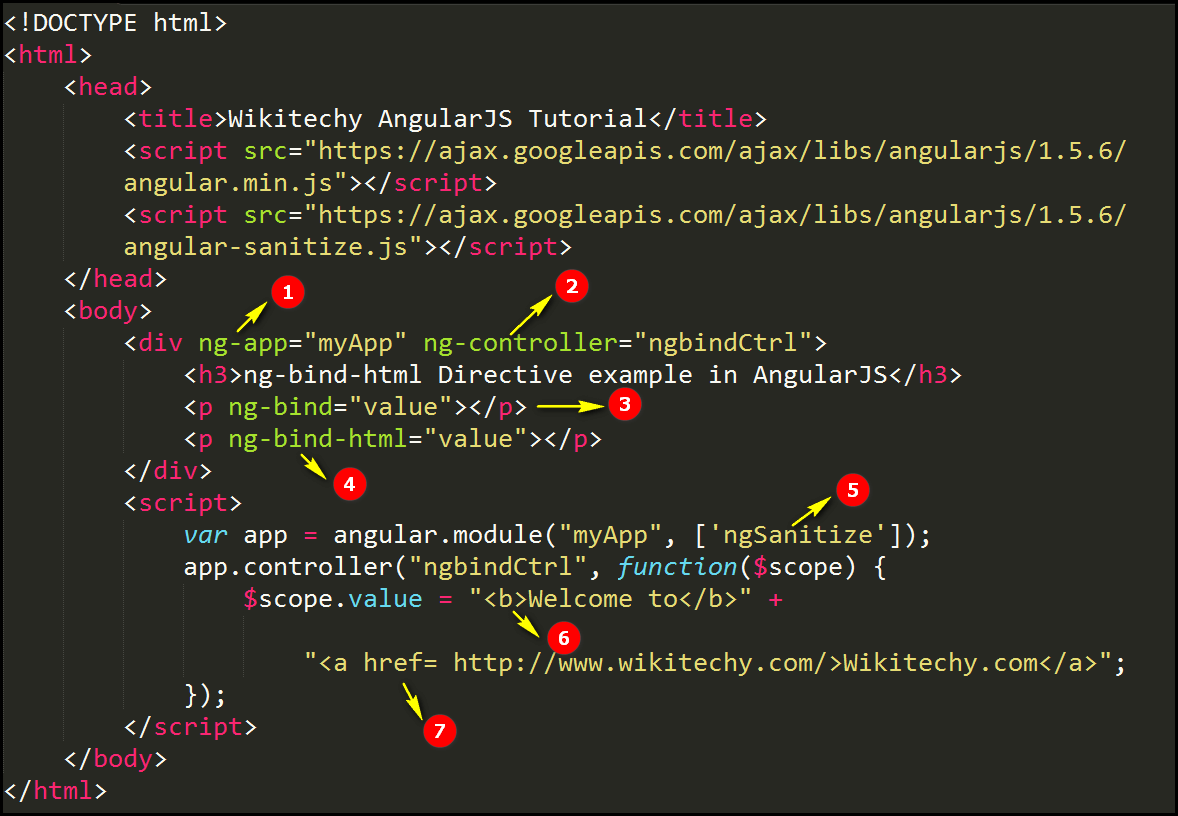
- The ng-app specifies the root element (“myapp”) to define AngularJS application.
- ng-controller specifies the application controller in AngularJS the controller value is given as “ngbindCtrl”.
- The ng-bind is used to binds the content of the HTML document. Here AnguarJS get the value from the scope object and does not displays the content in html tag format.
- The ng-bind-html is used to binds the inner HTML of an HTML element. AngularJS get the value from the scope object and the output will be displayed in the HTML tag format (Like bold text).
- “ngSanitize” functions is used for removing dangerous tokens from the HTML.
- The text (Welcome to) inside the <b> tag and the output will be displayed in bold text.
- “href” is used for define the link page(like Wikitechy.com)
Sample Output for ng-bind-html directive in AngularJS:
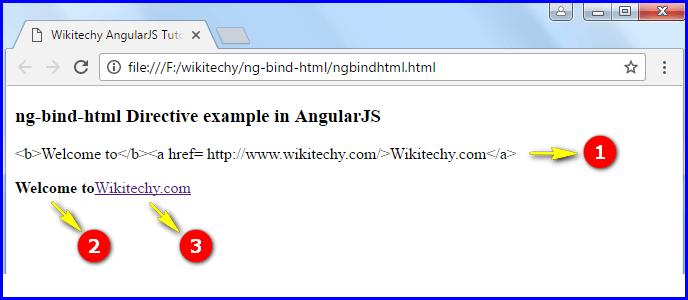
- The output shows the content which does not change the HTML tag format.
- The output displays the content “Welcome to” is the bold text.
- The output shows the wikitechy.com has been hyperlinked which will navigate wikitechy.com website
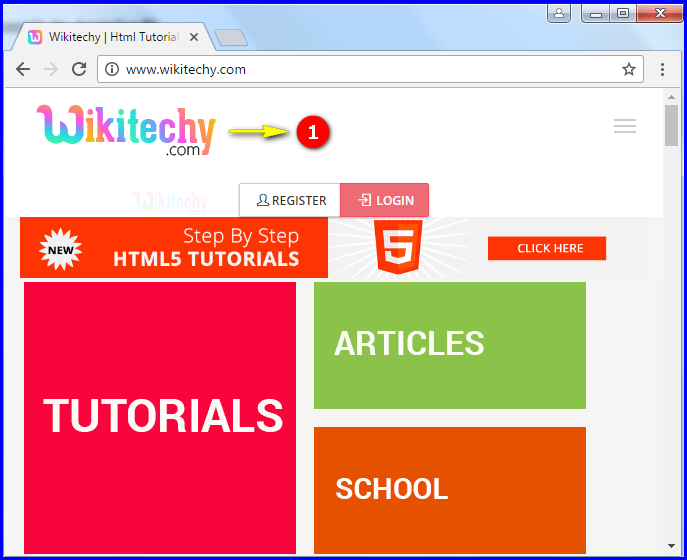
- If user click the wikitechy.com that navigate to the www.wikitechy.com website