String Compare in C
Arrays and Strings in Tamil
String Compare in C
- The strcmp() function takes two strings and return an integer.
- The strcmp() compares two strings character by character.
- If the first character of two strings are equal, next character of two strings are compared.
- This continues until the corresponding characters of two strings are different or a null character '\0' is reached.
- It is defined in string.h header file.
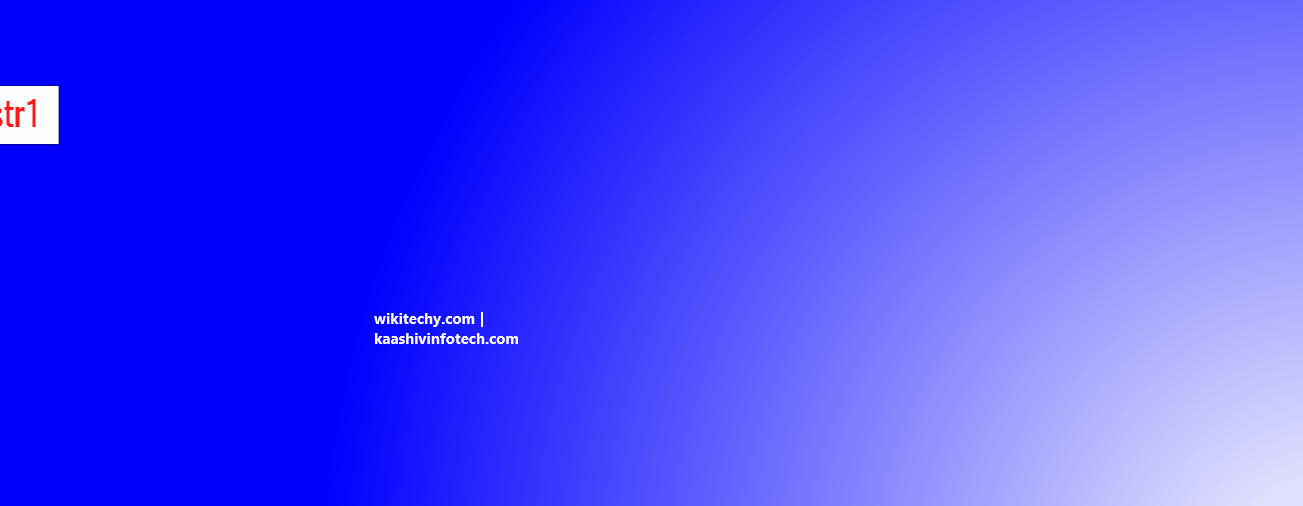
Sample Code
#include<stdio.h>
#include<string.h>
void main()
{
char str1[20],str2[20];
printf("Enter 1st string: ");
gets(str1);
printf("Enter 2nd string: ");
gets(str2);
if((strcmp(str1,str2)==0))
printf("Strings are equal\n");
else
printf("Strings are not equal\n");
getch();
}
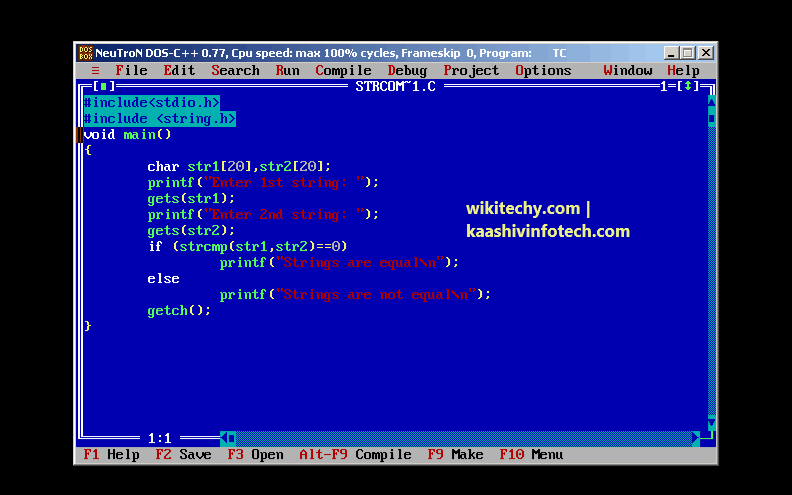
Learn C - C tutorial - String Compare - C examples - C programs
Output
Enter 1st string : hi this is venkat
Enter 2nd string : hi this is venkat
Strings are equal
Enter 1st string : kaashiv
Enter 2nd string : wikitechy
Strings are not equal
Read Also
Compare Two Strings in JavaString Compare Without Using Strcmp()
Sample Code
#include<stdio.h>
void main()
{
char string1[50],string2[50];
int i,temp = 0;
printf("Enter the string1 value: ");
scanf("%s",string1);
printf(" Enter the String2 value: ");
scanf("%s",string2);
for(i=0; string1[i]!='\0'; i++)
{
if(string1[i] == string2[i])
temp = 1;
else
temp = 0;
}
if(temp == 1)
printf("Strings are equal.\n");
else
printf("Strings are not equal.\n");
getch();
}
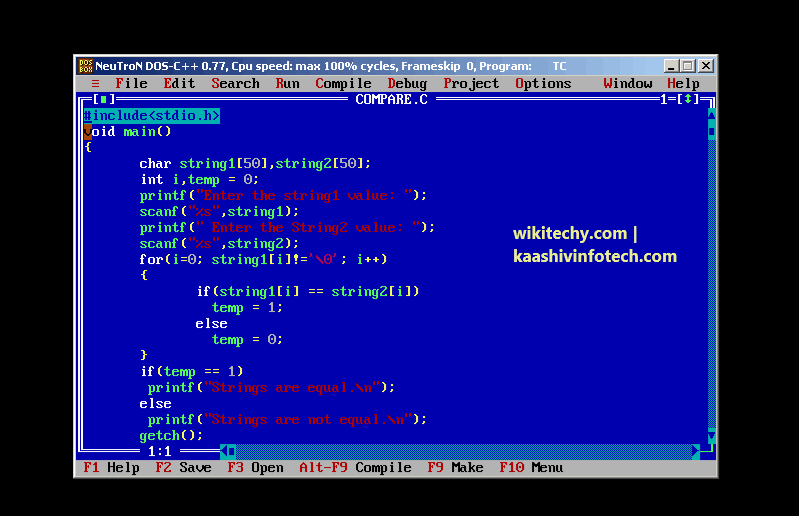
Learn C - C tutorial - String Compare Without Using Strcmp - C examples - C programs
Output
Enter the string1 value: wikitechy
Enter the string2 value: wikitechy
Strings are equal
Enter the string1 value: Kaashiv
Enter the string2 value: wikitechy
Strings are not equal