C - Primitive Data types
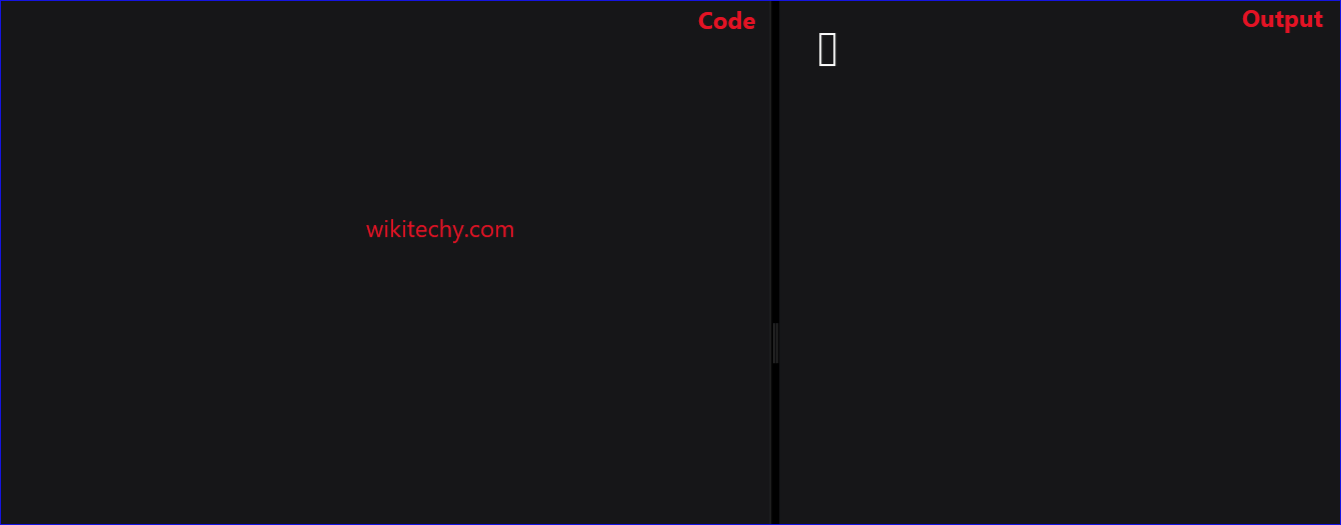
Learn C - C tutorial - Primitive data types - C examples - C programs
C - Primitive Data types - Definition and Usage
- The primitive data types in c language are the inbuilt data types provided by the c language itself .
- Thus, all C compilers provide support for these data types. The Primitive Datatypes are as follows:
- Integer Data Type (int)
- Float data Type (float)
- Double Data Type (double)
- Character Data Type (char)
- Void Data Type (void)
Read Also
Preprocessor directives in C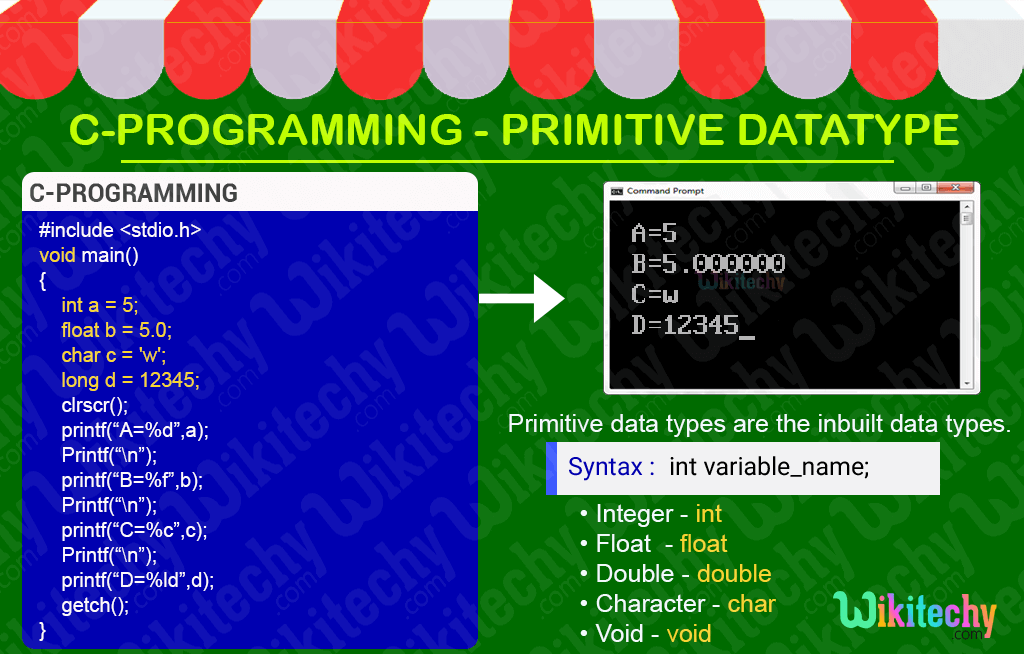
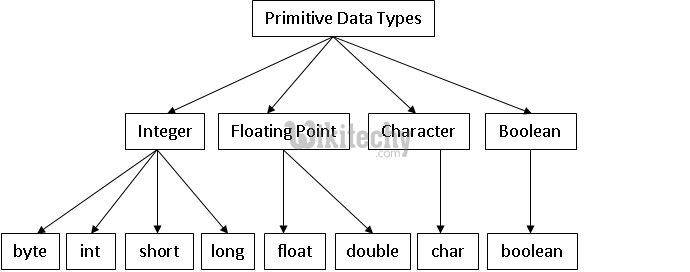
Integer Data Type (int) :
- Integer data type allows a variable to store numeric values (7,34,678,32…).
- “int” keyword is used to refer integer data type.
- The storage size of int data type is 2 or 4 or 8 bytes.
C Syntax
int variable_name;
Float data Type (float) :
- Float data type declares a variable that can store numbers containing a decimal number (0.7 , 0.46, etc.) .
- “float” keyword is used to refer float data type.
C Syntax
float variable_name;
Character Data Type (char) :
- Character variables declared as char data type can only store one single character.
- “char” keyword is used to refer character data type.
C Syntax
char variable_name;
Read Also
Non Primitive data Types.C Syntax
void variable_name;
Sample - C Code
#include <stdio.h>
void main()
{
int a = 5;
float b = 5.0;
char c = 'w';
long d = 12345;
clrscr();
printf(“A=%d”,a);
Printf(“\n”);
printf(“B=%f”,b);
Printf(“\n”);
printf(“C=%c”,c);
Printf(“\n”);
printf(“D=%ld”,d);
getch();
}
C Code - Explanation :
- Here in the c programming, void main() is declared as a parent function of all the functions in C. If we don't need any return value, we use return type 'void'.
- In C programming, int a = 5 is specified as positive integer data type.
- In C programming, float b = 5.0 is specified as positive float data type.
- In C programming, char c = 'w' is specified as char data type.
- In C programming, long d = 12345 is specified as long positive integer data type.
- Here, printf() function is used to print the “integer, float, character, double values” onto the output screen where its format specifier is used to display the value of the variables.
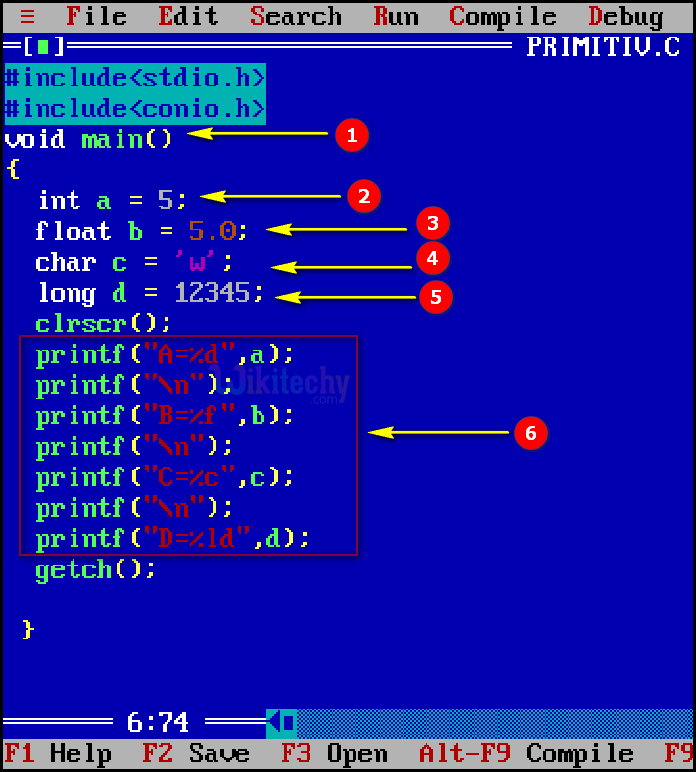
Sample Output - Programming Examples
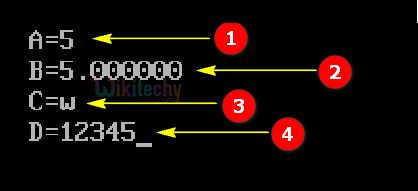
- Here in this output A=5 specifies the integer data type.
- Here in this output B=5.000000 specifies the float data type.
- Here in this output C=w specifies the character data type.
- Here in this output D=12345 specifies the long character data type.