fopen C
File Operations in Tamil
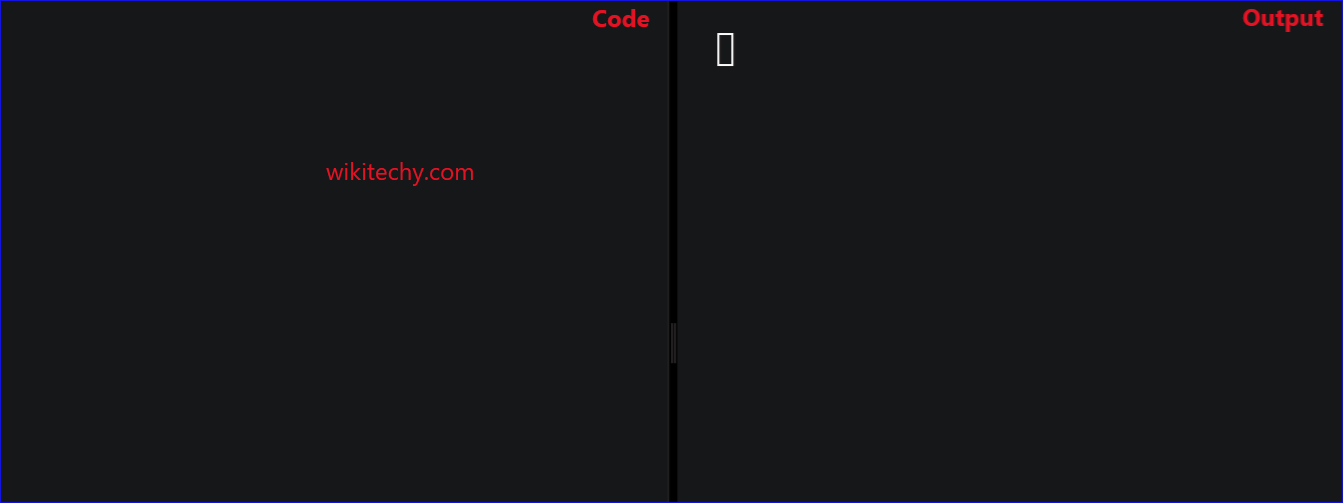
Learn C - C tutorial - Fopen c - C examples - C programs
C - fopen() - Definition and Usage
- In C – Programming, the fopen function is used to create a new file (or) open the exiting file.
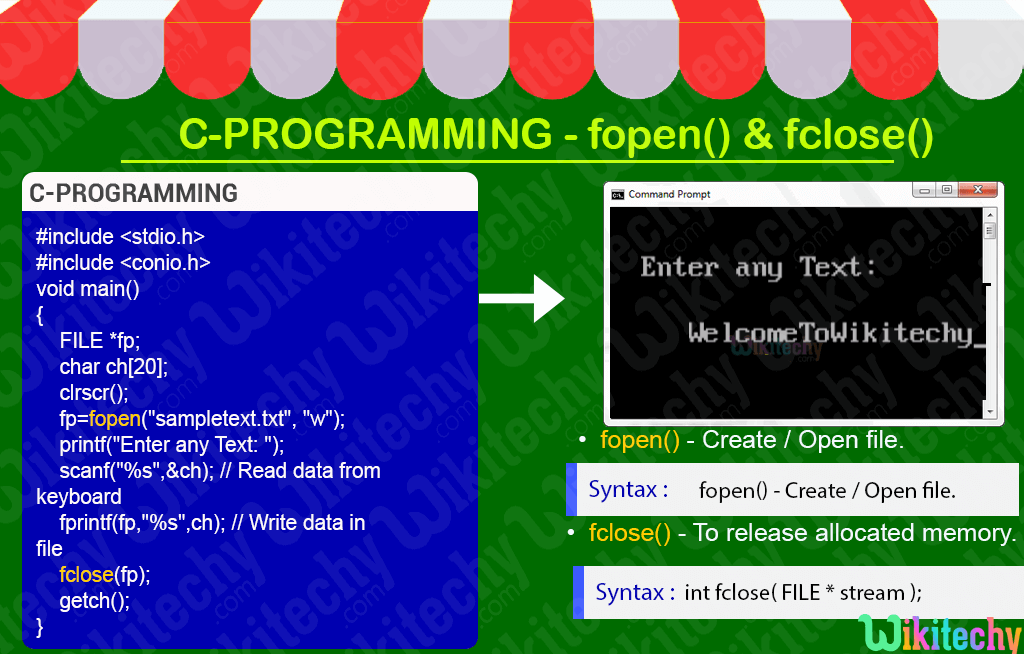
C Syntax
FILE *fopen( const char * filePath, const char * mode );
- In this syntax the file path defines the file location. .
- Whereas, the second argument defines the access mode of the file.
Fopen function access mode :
- r-Opens an existing text file.
- w-Opens a text file for writing, if file doesn’t exist then a new file is created.
- a-Opens a text file for appending (writing at the end of existing file) and create the file if it does not exist.
- r+-Opens a text file for reading and writing.
- w+-Open for reading and writing and create the file if it does not exist. If the file exists, then make it blank.
- a+-Opens for reading and appending and also to create the file if it does not exist. The reading will start from the beginning but writing can only be appended.
Fclose function :
- In C-Programming the fclose function is used to release the memory space allocated by the fopen() function.
C Syntax
int fclose( FILE * stream );
Sample - C Code
#include <stdio.h>
#include <conio.h>
void main()
{
FILE *fp;
char ch[20];
clrscr();
fp=fopen("sampletext.txt", "w");
printf("Enter any Text: ");
scanf("%s",&ch); // Read data from keyboard
fprintf(fp,"%s",ch); // Write data in file
fclose(fp);
getch();
}
C Code - Explanation
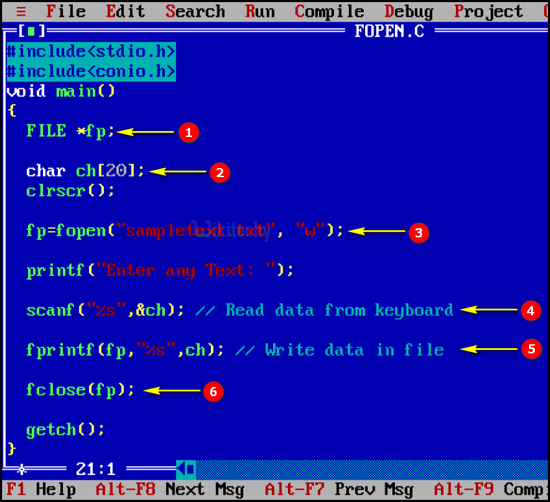
- Here in this statement we create FILE *fp; which is the file pointer.
- Here in this statement we create a character array.
- In this statement we open the file using fopen function and the file will be assigned for the variable “fp” .
- In this statement we get the input using scanf statement.
- In this, fprintf statement is used to write the data from the file.
- After the completion of file manipulation, the file will be closed using fclose function.
Sample Output - Programming Examples
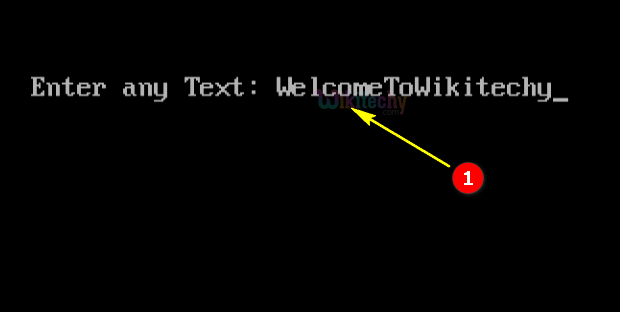
- Here in this output we enter a text for “WelcomeToWikitechy”, where the statement will be stored in sampletext.txt as shown below whose file location will be in the bin folder.
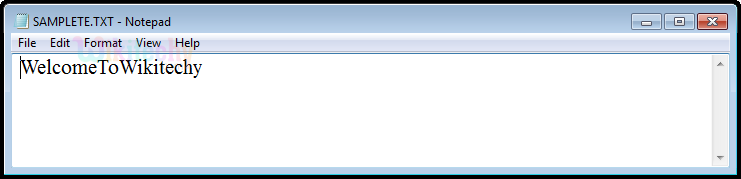
C - fread - Definition and Usage
- It is the function used to read the binary data from the file and store into the object. It reads “n” items of “size” bytes each through the file pointer and assigns to the members of struct object whose address is being sent to the function.
- Fileread accepts the
- Address of object,
- Size of object,
- Number object to be read and
- File pointer as arguments.
- Returns the number of items fetched from the file, returns -1 if any thing goes wrong in reading.
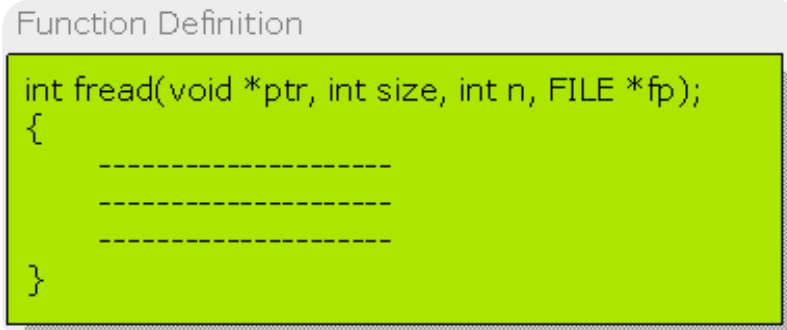
Learn C - C tutorial - C fread - C examples - C programs
C Syntax
fread(OutputBuffer, 1,10,fp);
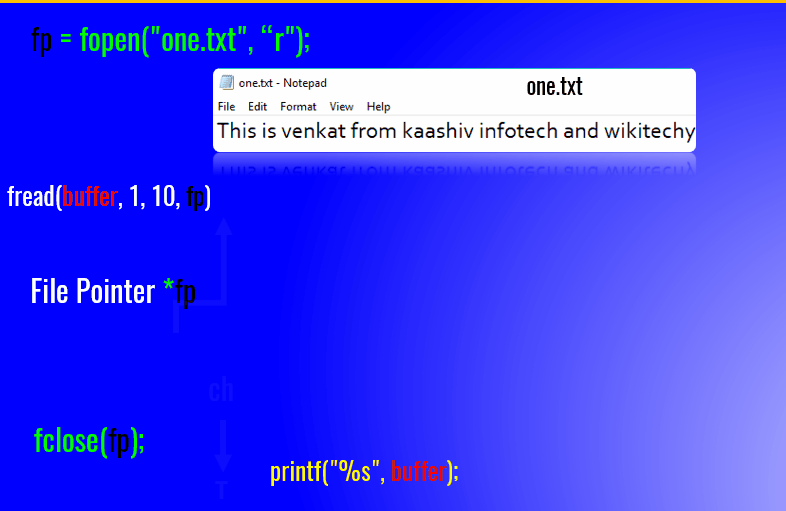
Sample - C Code
#include <stdio.h>
#include <conio.h>
int main()
{
FILE *f;
char buffer[11];
if (f = fopen("one.txt", "r"))
{
fread(buffer, 1, 10, f);
buffer[10] = 0;
fclose(f);
printf("First 10 characters in file:\n %s \n", buffer);
}
getch();
return 0;
}
Sample Output
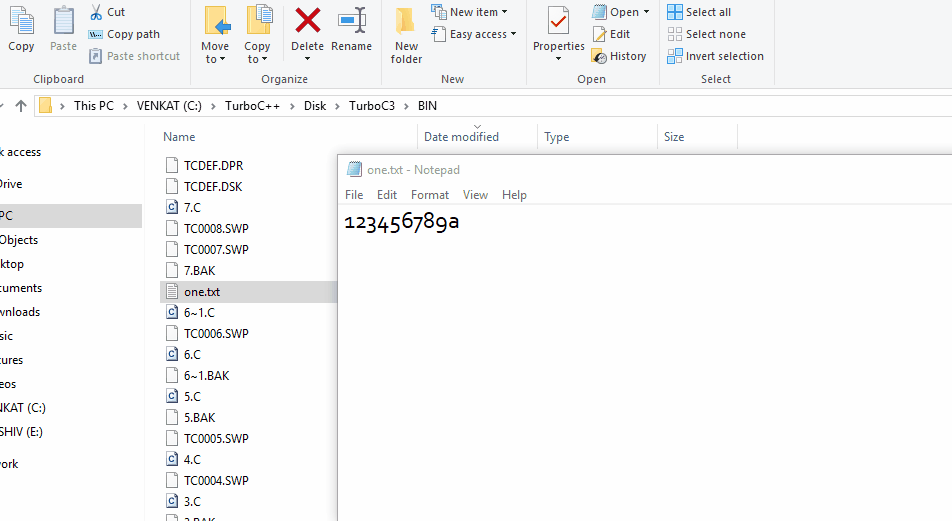
Learn C - C tutorial - C fread - C examples - C programs