Functions in C
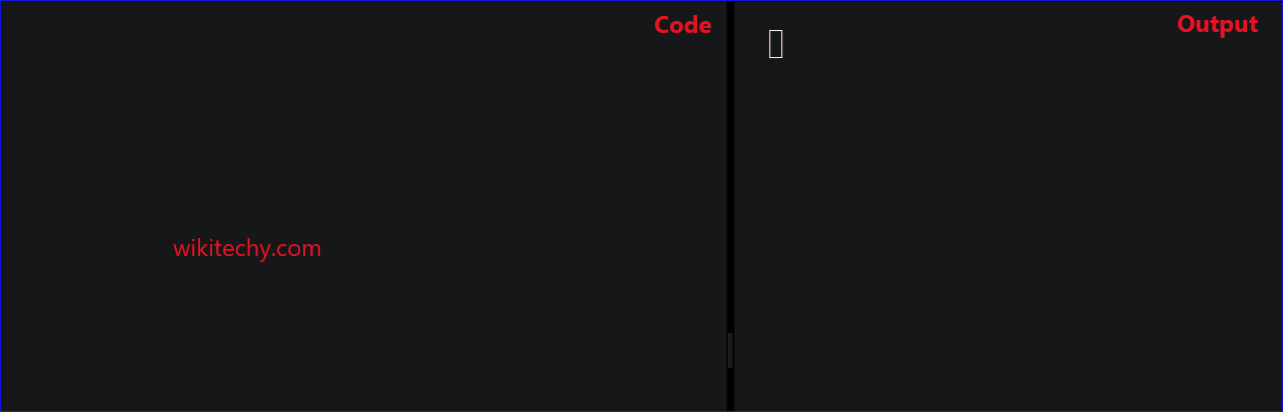
Learn C - C tutorial - Functions in c - C examples - C programs
Functions in C - Definition and Usage
- A function is a group of statements that together perform a task.
- Every C program has at least one function, which is main() , and all the most trivial programs can define additional functions.
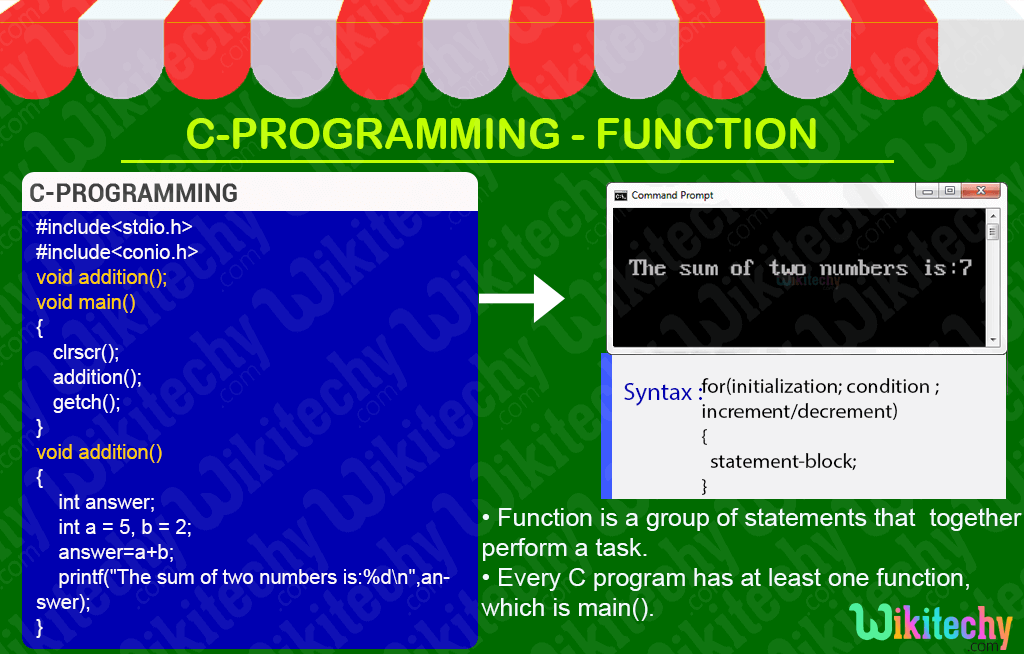
Benefits of using function in C :
- Functions provides reusable code.
- In large programs of C, debugging and editing tasks is easy with the use of functions.
- The program can be modularized into smaller parts.
Types of functions in C :
There are two types of functions in C
- Built in(Library) Functions
- User Defined Functions
Built in(Library) Functions :
- These functions are provided by the system and stored in C library.
- Example: scanf(), printf(), strcmp, strcpy, strlen, strlwr,
User Defined Functions :
- These functions are defined by the user at the time of writing the program.
Parts of Function in C Programming :
There are three types of functions such as
- Function Prototype (function declaration)
- Function Definition
- Function Call
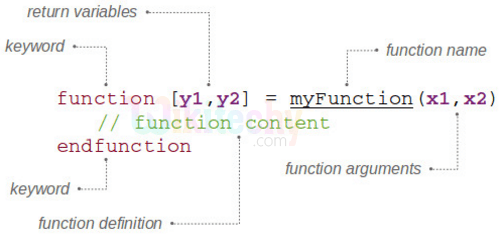
Function Prototype :
- A function prototype is a function declaration that specifies the data types of its arguments in the parameter list.
- Prototypes are syntactically distinguished from the old style of function declaration.
C Syntax
datatype functionName (Parameter List)
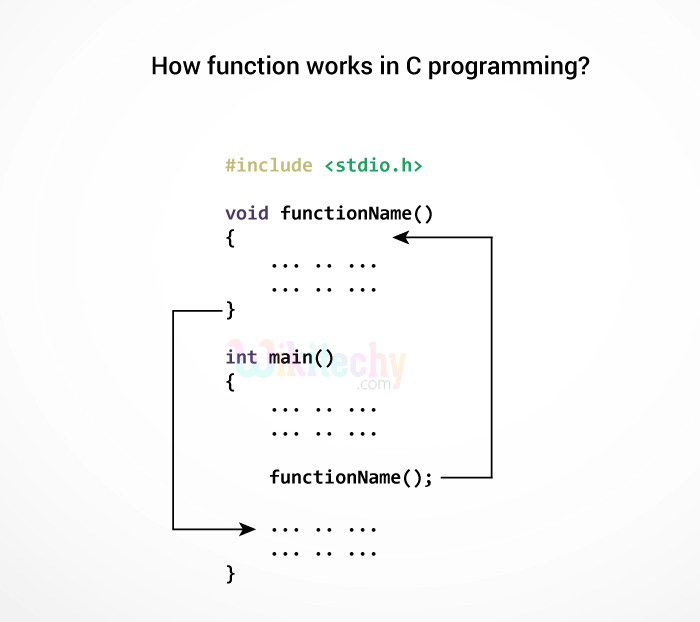
Function Definition :
- A function definition specifies the name of the function, the types and number of parameters it expects to receive, and its return type.
- A function definition also includes a function body with the declarations of its local variables, and the statements that determine what the function does.
C Syntax
returnType functionName(Function arguments)
{
//body of the function
}
Function Call :
- A function call in C , tells the compiler about a function's name, return type, and parameters , in which it defines the actual body of the function.
- Apart from this in C , the standard library provides numerous built-in functions that our program can call.
C Syntax
function_name ( arguments list );
Sample - C Code
#include<stdio.h>
#include<conio.h>
void addition();
void main()
{
clrscr();
addition();
getch();
}
void addition()
{
int answer;
int a = 5, b = 2;
answer=a+b;
printf("The sum of two numbers is:%d\n",answer);
}
C Code - Explanation:
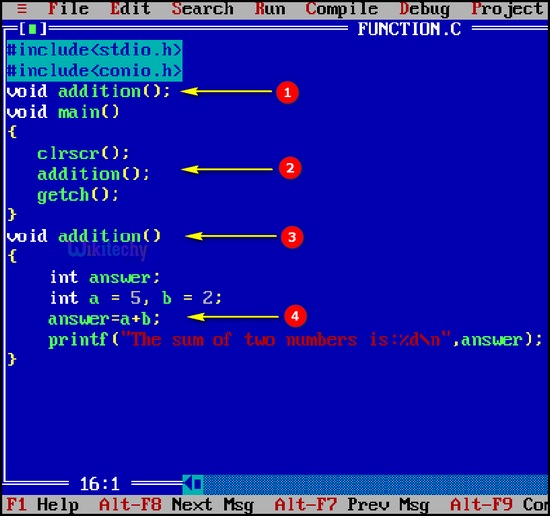
- In this Example, void addition(); is specified as a function declaration .
- Here, addition is specified as a function call that is considered as the local variable definition.
- Here, void addition() is said to be the calling function to get an addition values of a, b.
- In this example, int a = 5, b = 2 addition function returning the addition of two numbers 5 & 2 in the output statement stored in in the variable “answer”.
Sample Output - Programming Examples
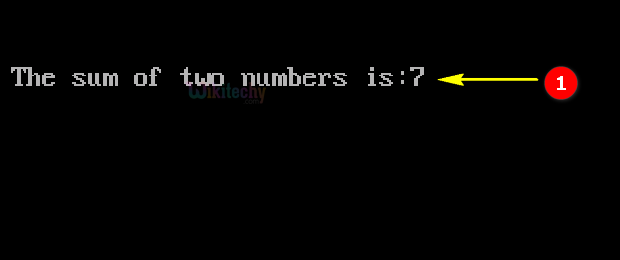
- Here in this output “The sum of two numbers:“ is printed where its sum of 5 & 2 =7 is shown here.