realloc & free in C
Structure and Linked List with C Programming in Tamil
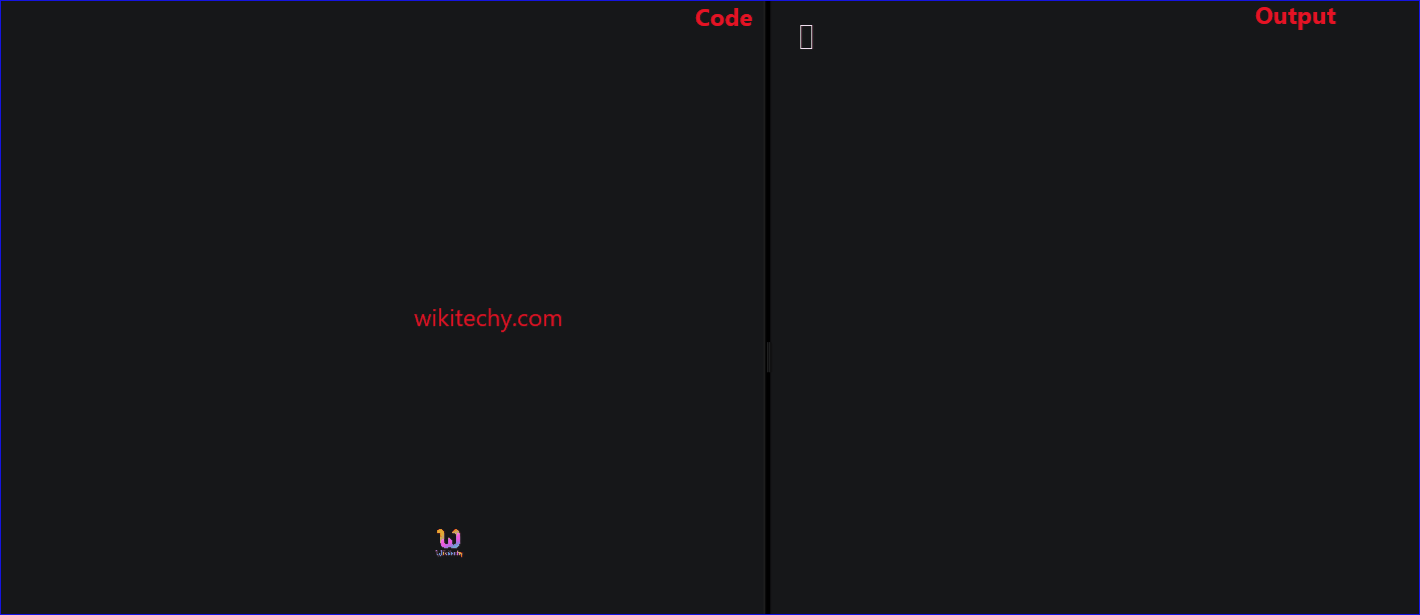
Learn C - C tutorial - realloc - C examples - C programs
C - realloc() & free() Function - Definition and Usage
- In C- Programming the realloc () function is used for modifying the allocated memory size by malloc () and calloc () functions to new size.
- In C-Programming the realloc function is used for extending the allocate memory space.
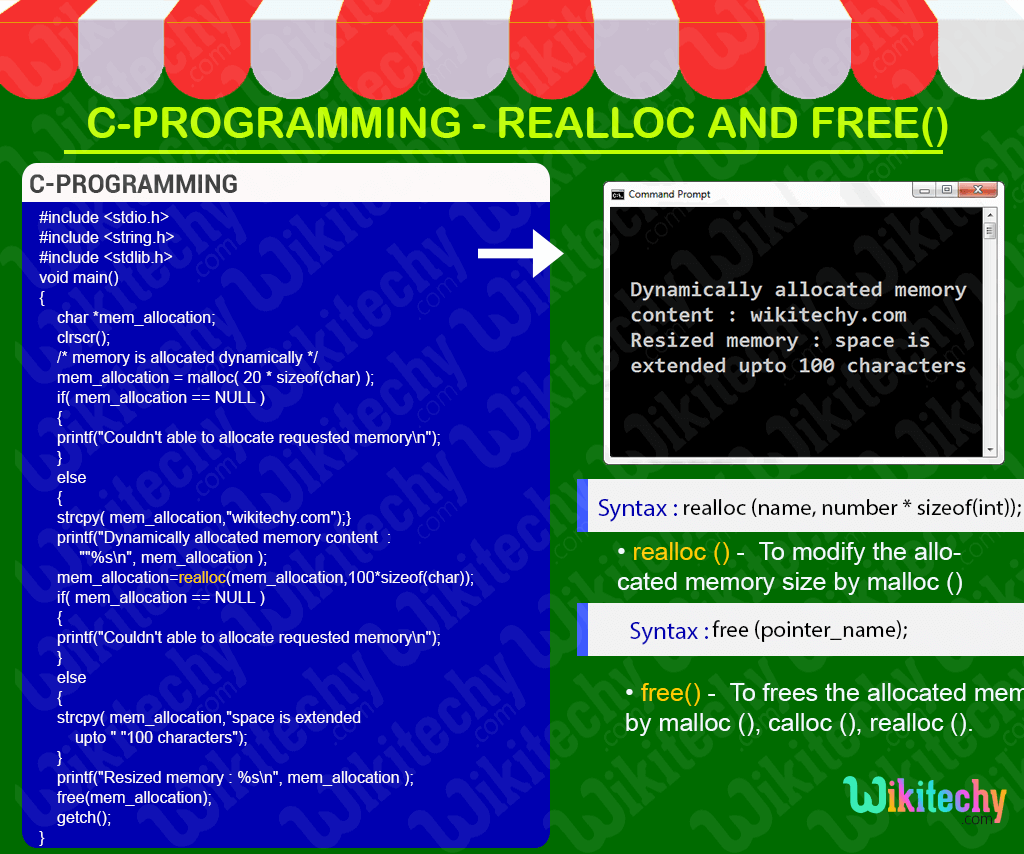
C Syntax
realloc (pointer_name, number * sizeof(int));
or
ptr=realloc(ptr, new-size)
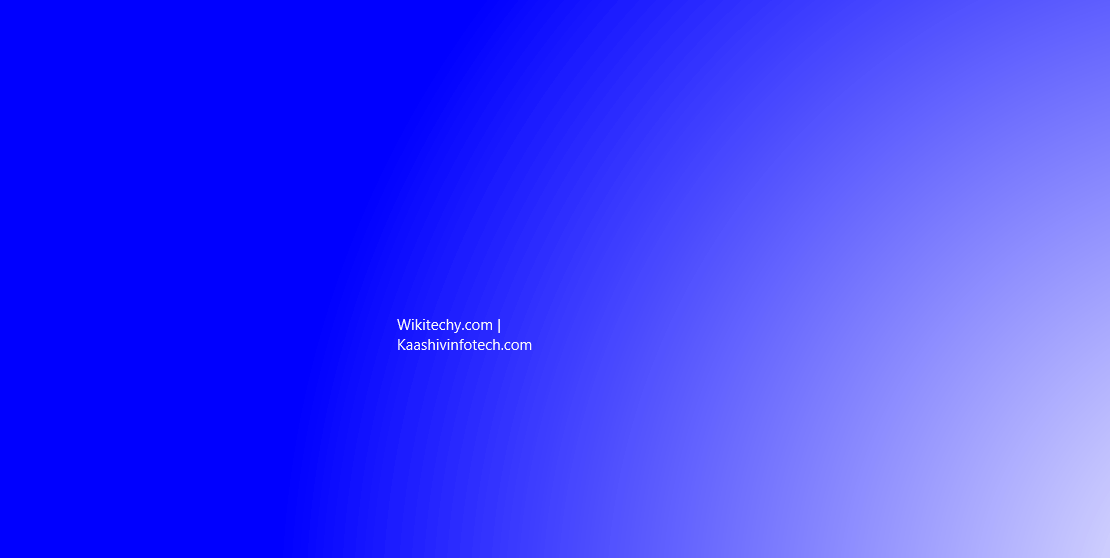
Free() Function :
- In C-Programming the free function is used to frees the allocated memory by malloc (), calloc (), realloc () functions and returns the memory to the system.
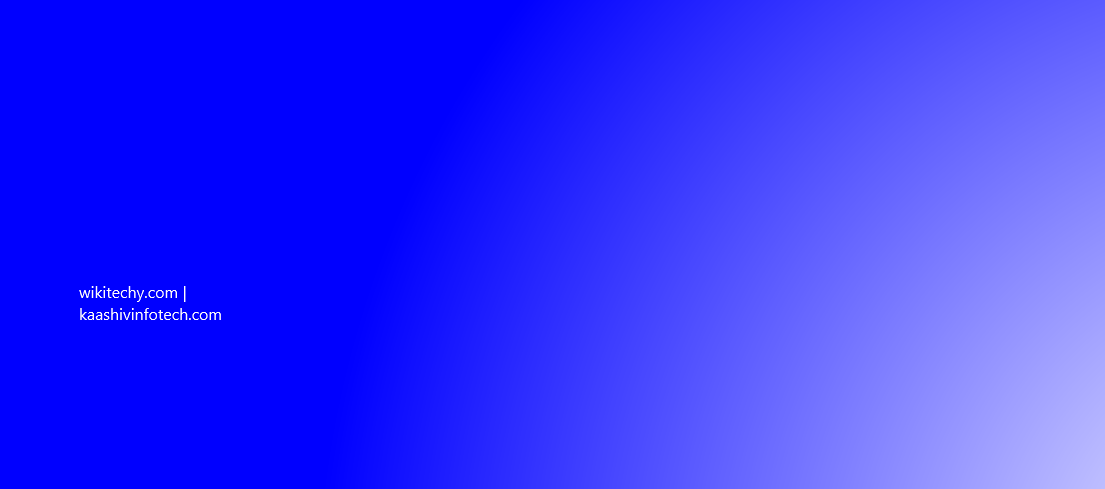
C Syntax
free (pointer_name);
Example 1
Sample - C Code
#include <stdio.h>
#include <conio.h>
void main()
{
char *mem_allocation;
clrscr();
/* memory is allocated dynamically */
mem_allocation = malloc( 20 * sizeof(char) );
if( mem_allocation == NULL )
{
printf("Couldn't able to allocate requested memory\n");
}
else
{
strcpy( mem_allocation,"wikitechy.com");}
printf("Dynamically allocated memory content :
""%s\n", mem_allocation );
mem_allocation=realloc(mem_allocation,100*sizeof(char));
if( mem_allocation == NULL )
{
printf("Couldn't able to allocate requested memory\n");
}
else
{
strcpy( mem_allocation,"space is extended
upto " "100 characters");
}
printf("Resized memory : %s\n", mem_allocation );
free(mem_allocation);
getch();
}
C Code - Explanation
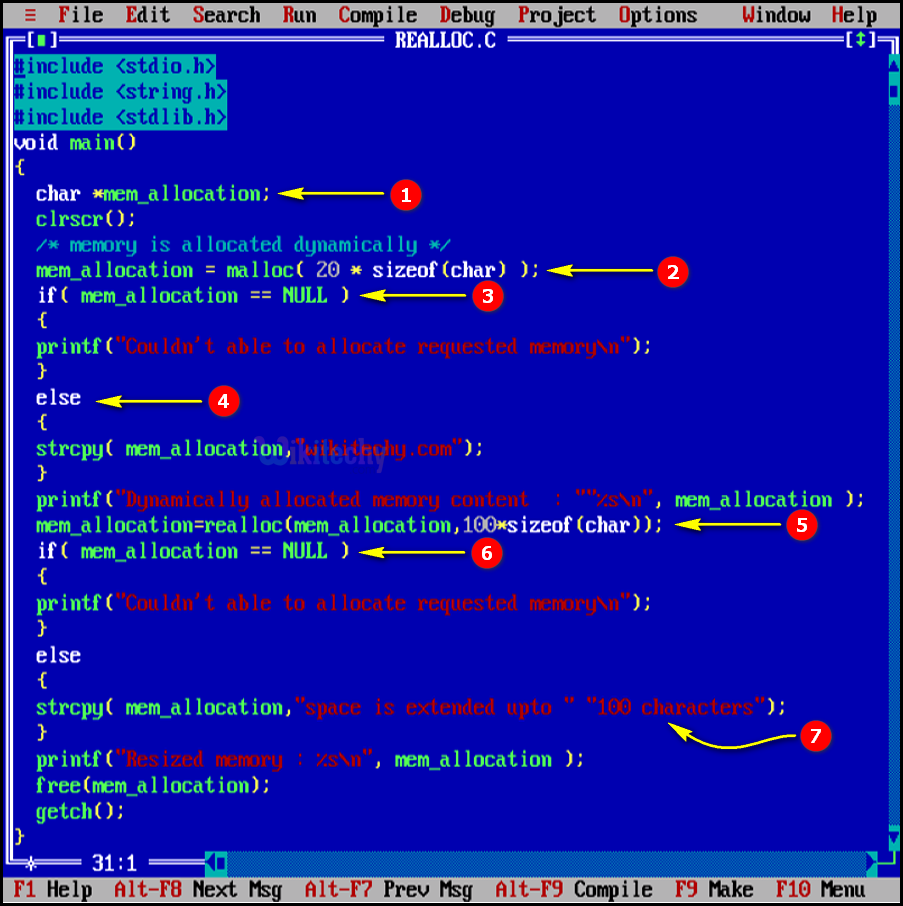
- In this statement we assign the address of the “q” variable to “ptr” .
- In this printf statement we print the address of the variable “q” .
- In this printf statement we print the value of the variable “q” .
- In this statement the memory space will be allocated where the content will be assigned for the certain variable.
- In this statement we reallocate the memory space where we extend the memory space using realloc function.
- In this statement we check the memory space is empty are not.
- In this printf statement we print the extended memory space.
Sample Output - Programming Examples
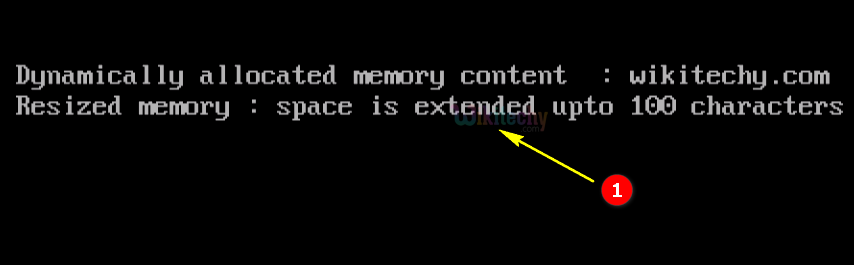
- In this statement we declare the pointer variable “mem_allocation” as character datatype.
- In this statement we allocate the memory space dynamically using calloc function.
- In this condition we check the memory space is empty or not..
Example 2
Sample Code
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void main ()
{
char *str;
/* Initial memory allocation */
str = (char *) malloc(15);
strcpy(str, "wikitechy");
printf("String = %s, Address = %u\n", str, str);
/* Reallocating memory */
str = (char *) realloc(str, 25);
strcat(str, ".com");
printf("String = %s, Address = %u\n", str, str);
/* Deallocate allocated memory */
free(str);
getch();
}
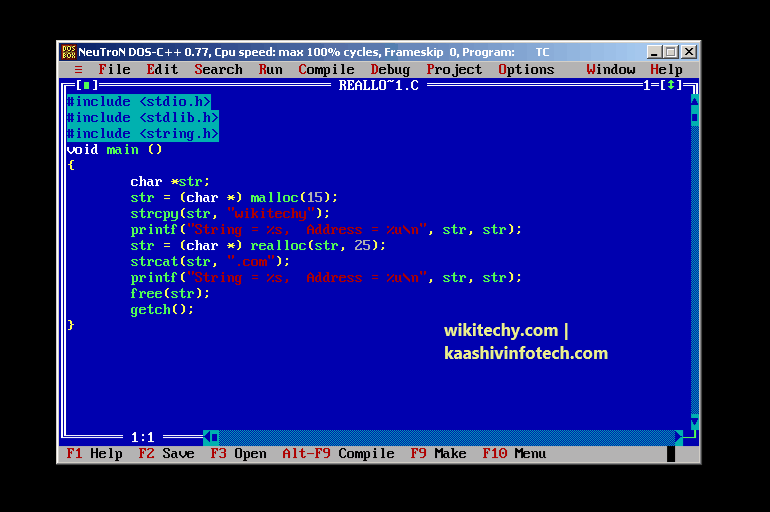
Output
String = wikitechy, Address = 1508
String = wikitechy.com, Address = 1528