Bubble Sort in C - Bubble Sort using C Program
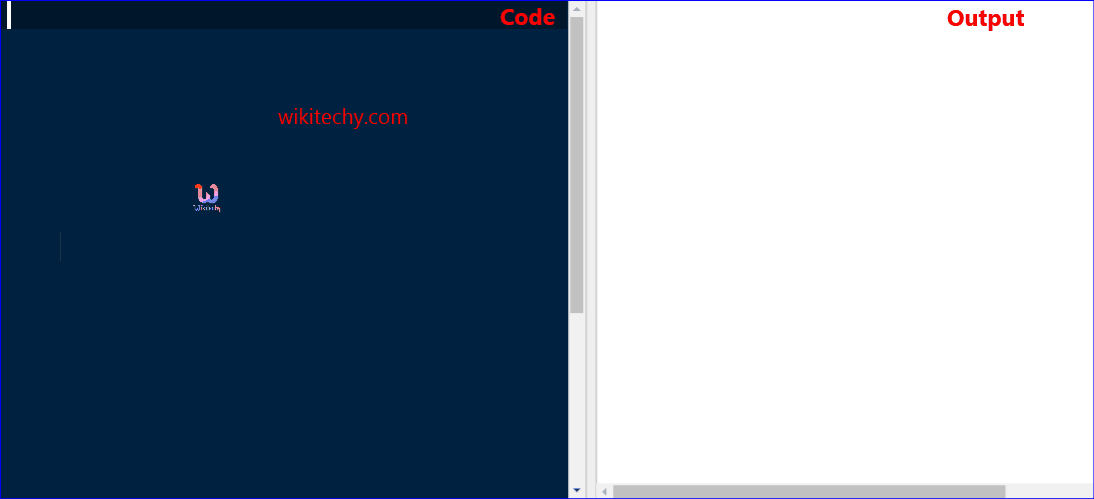
Learn C - C tutorial - bubble sort using c program - C examples - C programs
Bubble Sort using C Program
- Comparing each pair of adjacent elements and swaps them if they are out of order.This process is said to be bubble sort algorithm.
- To apply the Bubble Sort we follow the below steps.
- Compare first two elements and exchange them if they are out of order.
- Decrease one element and compare second and third elements. Exchange if necessary. Continue until end of array.
- Repeat process and exchanging as necessary.
- Repeat until a pass is made with no exchanges.
Sample Code
#include <stdio.h>
void swap(int *xp, int *yp)
{
int temp = *xp;
*xp = *yp;
*yp = temp;
}
void bubbleSort(int arr[], int n)
{
int i, j;
for (i = 0; i < n-1; i++)
for (j = 0; j < n-i-1; j++)
if (arr[j] > arr[j+1])
swap(& arr[j], & arr[j+1]);
}
void printArray(int arr[], int size)
{
int i;
for (i=0; i < size; i++)
printf("%d ", arr[i]);
printf("n");
}
int main()
{
int arr[] = {64, 34, 25, 12, 22, 11, 90};
int n = sizeof(arr)/sizeof(arr[0]);
bubbleSort(arr, n);
printf("Sorted array: \n");
printArray(arr, n);
return 0;
}
Output
Sorted array:
11 12 22 25 34 64 90 n