Swap Function in C - C Program Swap Numbers in Cyclic Order Using Call by Reference
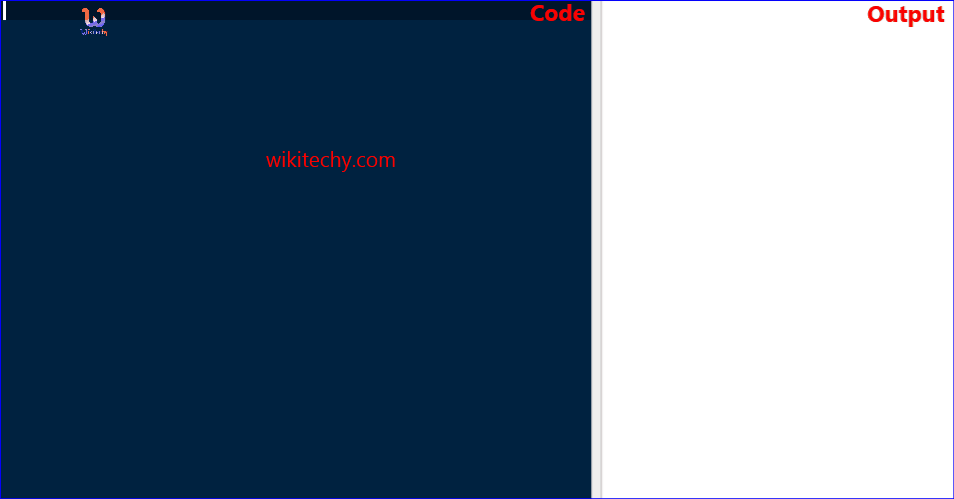
Learn C - C tutorial - c program swap numbers in cyclic order using call by reference - C examples - C programs
C Program Swap Numbers in Cyclic Order Using Call by Reference
- Call by reference is a method for passing data into the functions.
- The call by reference method of passing arguments to a function copies the address of an argument into the formal parameter.
- To exchange the values of the two integer variables swap() function is used.
Sample Code
#include<stdio.h>
void cyclicSwap(int *a,int *b,int *c);
int main()
{
int a=5,b=8,c=3;
printf("a, b and c:5,8,3\n");
printf("Value before swapping:\n");
printf("a = %d \nb = %d \nc = %d\n",a,b,c);
cyclicSwap(&a, &b, &c);
printf("Value after swapping:\n");
printf("a = %d \nb = %d \nc = %d",a, b, c);
return 0;
}
void cyclicSwap(int *a,int *b,int *c)
{
int temp;
temp = *b;
*b = *a;
*a = *c;
*c = temp;
}
Output
a, b and c:5,8,3
Value before swapping:
a = 5
b = 8
c = 3
Value after swapping:
a = 3
b = 5
c = 8