Android tutorial - datepicker in android - android app development - android studio - android development tutorial
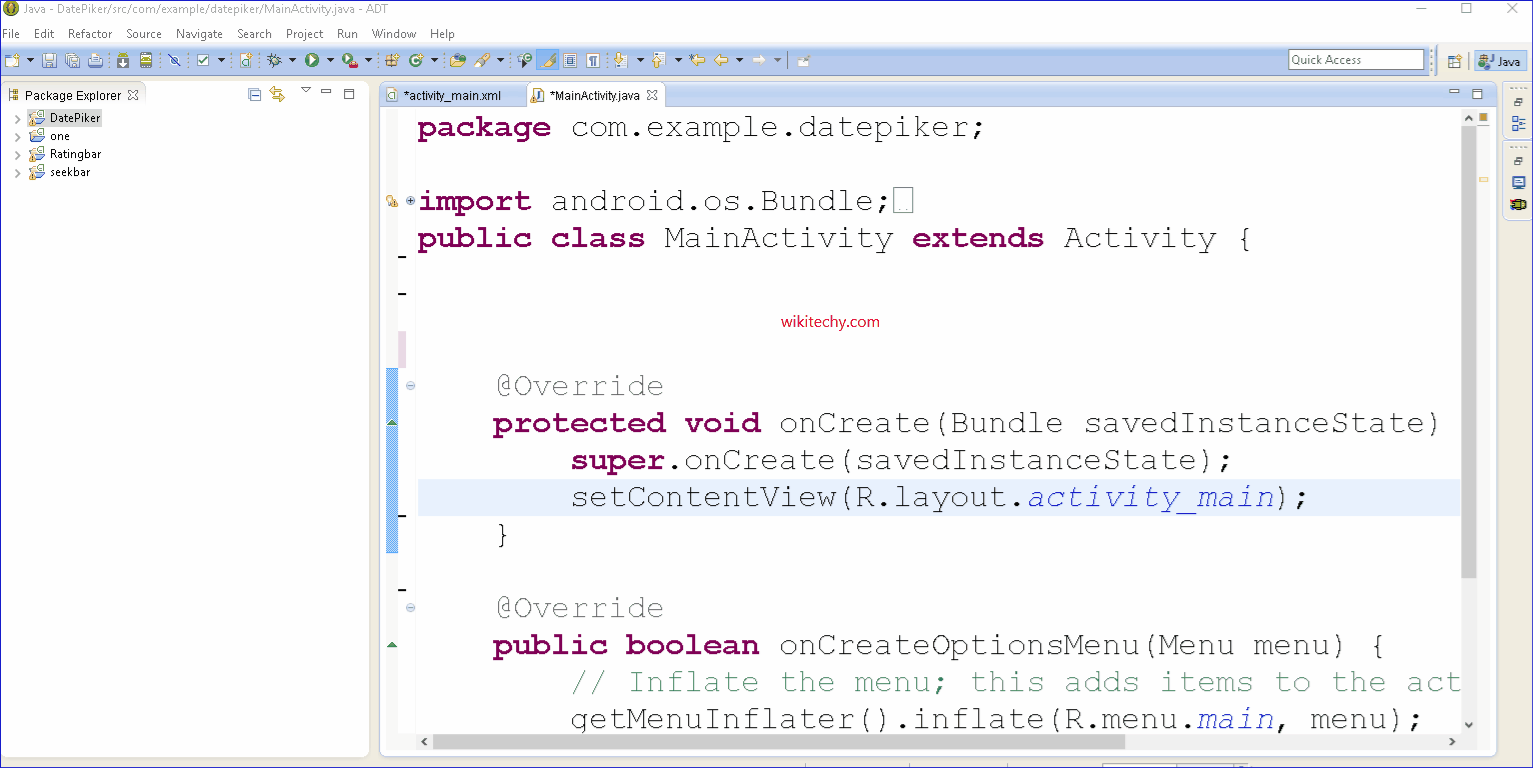
Learn android - android tutorial - Datepicker in android - android examples - android programs
Android DatePicker:
- Android Date Picker allows you to select the date consisting of day, month and year in your custom user interface.
- For this functionality android provides DatePicker and DatePickerDialog components.
- In Android, DatePicker is a widget used to select a date.
- It allows to select date by day, month and year in your custom UI (user interface).
- If we need to show this view as a dialog then we have to use a DatePickerDialog class. For selecting time Android also provides timepicker to select time.
- A date picker, popup calendar, date and time picker, or time picker is a graphical user interface widget which allows the user to select a date from a calendar and/or time from a time range.
- The typical practice is to provide a text box field which,
- when clicked upon to enter a date,
- pops up a calendar next to or below the field,
- allowing the user to populate the field with an appropriate date,
- provides a text box with an icon of a calendar such that when the icon is clicked on, the calendar (or time field) appears.
- The date picker provides several advantages, including:
- allowing the user to enter a date by merely clicking on a date in the pop-up calendar as opposed to having to take their hand off the mouse to type in a date.
- validation of dates by restricting date ranges, e.g. only after today and for two weeks later, or only for dates in the past.
- a date range can be entered such that for a set of "from-to" date fields,
- if the "from" field is filled, the "to" field cannot be set to a date before the "from" field, or if the "to" field is filled,
- the "from" field cannot be set to a later date than the "to" field.
- Only legal dates can be entered, e.g. February 29, 2100 can't be entered, nor could June 31.
- Date format confusion is eliminated, e.g. is 7/4/10 July 4, 2010, April 7, 2010, or April 10, 2007?
- Android DatePicker is a widget to select date.
- This control allows you to select date by day, month and year. Like DatePicker, android also provides TimePicker to select time.
- The android.widget.DatePicker is the subclass of FrameLayout class.
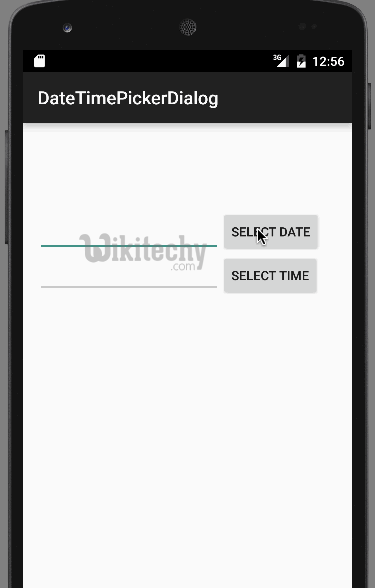
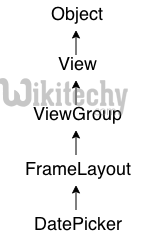
Android DatePicker Example
1. DatePicker
- 1. DatePicker
- Open “res/layout/main.xml” file, add date picker, label and button for Demo - android emulator - android tutorialnstration.
- File: res/layout/main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<Button
android:id="@+id/btnChangeDate"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Change Date" />
<TextView
android:id="@+id/lblDate"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Current Date (M-D-YYYY): "
android:textAppearance="?android:attr/textAppearanceLarge" />
<TextView
android:id="@+id/tvDate"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text=""
android:textAppearance="?android:attr/textAppearanceLarge" />
<DatePicker
android:id="@+id/dpResult"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
click below button to copy the code from android tutorial team
2. Code Code
- File: MyAndroidAppActivity.java
package com.wikitechy.android;
import java.util.Calendar;
import android.app.Activity;
import android.app.DatePickerDialog;
import android.app.Dialog;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.DatePicker;
import android.widget.TextView;
public class MyAndroidAppActivity extends Activity {
private TextView tvDisplayDate;
private DatePicker dpResult;
private Button btnChangeDate;
private int year;
private int month;
private int day;
static final int DATE_DIALOG_ID = 999;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
setCurrentDateOnView();
addListenerOnButton();
}
// display current date
public void setCurrentDateOnView() {
tvDisplayDate = (TextView) findViewById(R.id.tvDate);
dpResult = (DatePicker) findViewById(R.id.dpResult);
final Calendar c = Calendar.getInstance();
year = c.get(Calendar.YEAR);
month = c.get(Calendar.MONTH);
day = c.get(Calendar.DAY_OF_MONTH);
// set current date into textview
tvDisplayDate.setText(new StringBuilder()
// Month is 0 based, just add 1
.append(month + 1).append("-").append(day).append("-")
.append(year).append(" "));
// set current date into datepicker
dpResult.init(year, month, day, null);
}
public void addListenerOnButton() {
btnChangeDate = (Button) findViewById(R.id.btnChangeDate);
btnChangeDate.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
showDialog(DATE_DIALOG_ID);
}
});
}
@Override
protected Dialog onCreateDialog(int id) {
switch (id) {
case DATE_DIALOG_ID:
// set date picker as current date
return new DatePickerDialog(this, datePickerListener,
year, month,day);
}
return null;
}
private DatePickerDialog.OnDateSetListener datePickerListener
= new DatePickerDialog.OnDateSetListener() {
// when dialog box is closed, below method will be called.
public void onDateSet(DatePicker view, int selectedYear,
int selectedMonth, int selectedDay) {
year = selectedYear;
month = selectedMonth;
day = selectedDay;
// set selected date into textview
tvDisplayDate.setText(new StringBuilder().append(month + 1)
.append("-").append(day).append("-").append(year)
.append(" "));
// set selected date into datepicker also
dpResult.init(year, month, day, null);
}
};
}
click below button to copy the code from android tutorial team
3. Demo - android emulator - android tutorial
- Run the application.
- Result, “date picker” and “textview” are set to current date.
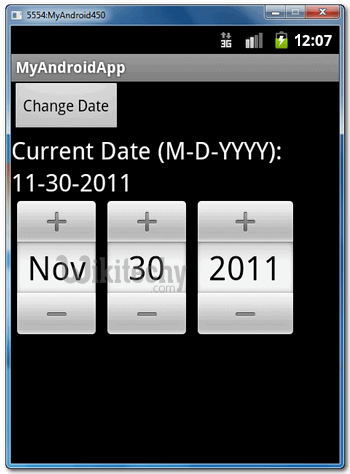
- Click on the “Change Date” button, it will prompt a date picker component in a dialog box via DatePickerDialog.
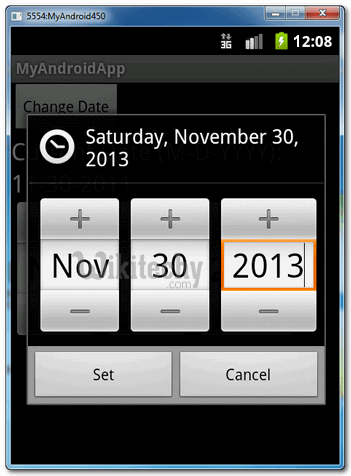
- Both “date picker” and “textview” are updated with selected date.
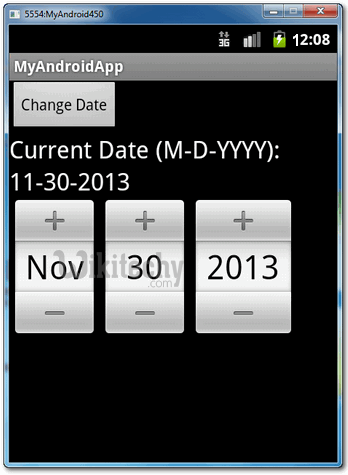