Android tutorial - toast in android - android app development - android studio - android development tutorial
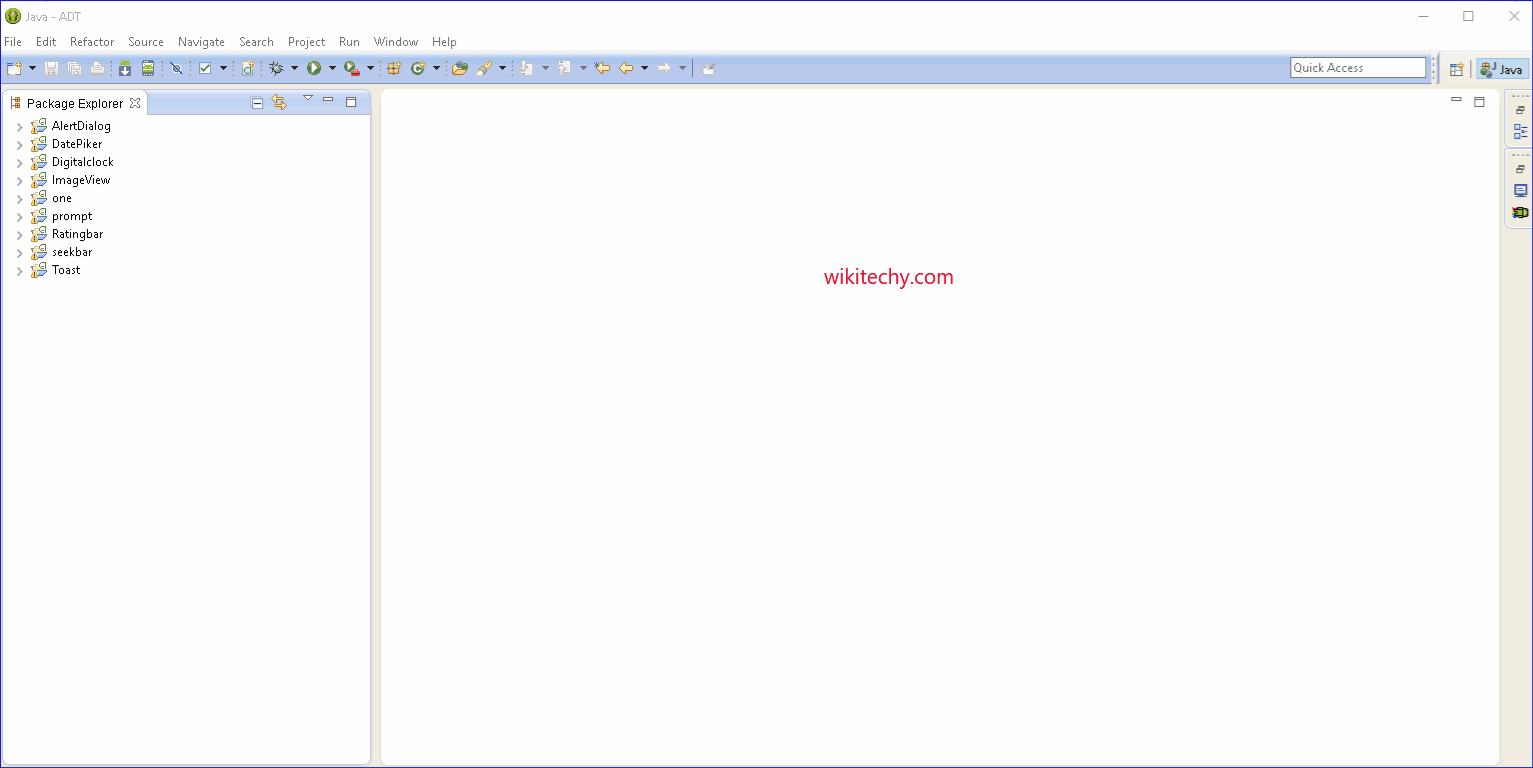
Learn android - android tutorial - Toast in android - android examples - android programs
What is Android Toast?
- A toast provides simple feedback about an operation in a small popup. It only fills the amount of space required for the message and the current activity remains visible and interactive.
- For example, navigating away from an email before you send it triggers a "Draft saved" toast to let you know that you can continue editing later.
- Toasts automatically disappear after a timeout.
- Toast is a solution for android developer when required to notify user about an operation without expecting any user input.
- This provides a small popup that displays for a small period and fades out automatically after timeout. Sometimes developers use this for debugging.
- In Android, Toast is a notification message that pop up, display a certain amount of time, and automatically fades in and out, most people just use it for debugging purpose.
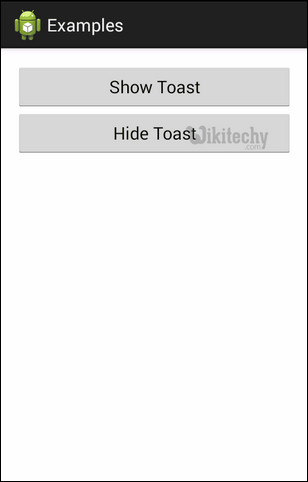
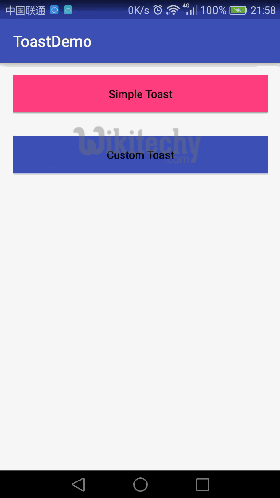
Code snippets to create a Toast message :
//display in short period of time
Toast.makeText(getApplicationContext(), "msg msg", Toast.LENGTH_SHORT).show();
//display in long period of time
Toast.makeText(getApplicationContext(), "msg msg", Toast.LENGTH_LONG).show();
click below button to copy the code from android tutorial team
In this tutorial, we will show you two Toast examples :
- Normal Toast view.
- Custom Toast view.
1. Normal Toast View
- Simple Toast example.
File : res/layout/main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<Button
android:id="@+id/buttonToast"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Show Toast" />
</LinearLayout>
click below button to copy the code from android tutorial team
File : MainActivity.java
package com.wikitechy.android;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends Activity {
private Button button;
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
button = (Button) findViewById(R.id.buttonToast);
button.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
Toast.makeText(getApplicationContext(),
"Button is clicked", Toast.LENGTH_LONG).show();
}
});
}
}
click below button to copy the code from android tutorial team
- See Demo - android emulator - android tutorial, when a button is clicked, display a normal Toast message.
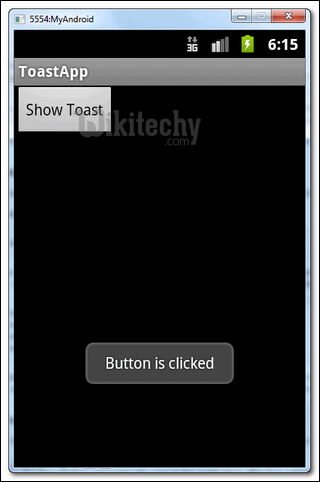
2. Custom Toast View
- Enchance above example by customize the original Toastview.
File : res/layout/custom_toast.xml - This is the custom Toast view.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/custom_toast_layout_id"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:background="#FFF"
android:orientation="horizontal"
android:padding="5dp" >
<ImageView
android:id="@+id/image"
android:layout_width="wrap_content"
android:layout_height="fill_parent"
android:layout_marginRight="5dp" />
<TextView
android:id="@+id/text"
android:layout_width="wrap_content"
android:layout_height="fill_parent"
android:textColor="#000" />
</LinearLayout>
click below button to copy the code from android tutorial team
File : MainActivity.java - Read comment, to get above custom view and attach to Toast.
package com.wikitechy.android;
import android.app.Activity;
import android.os.Bundle;
import android.view.Gravity;
import android.view.LayoutInflater;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
public class MainActivity extends Activity {
private Button button;
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
button = (Button) findViewById(R.id.buttonToast);
button.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
// get your custom_toast.xml ayout
LayoutInflater inflater = getLayoutInflater();
View layout = inflater.inflate(R.layout.custom_toast,
(ViewGroup) findViewById(R.id.custom_toast_layout_id));
// set a dummy image
ImageView image = (ImageView) layout.findViewById(R.id.image);
image.setImageResource(R.drawable.ic_launcher);
// set a message
TextView text = (TextView) layout.findViewById(R.id.text);
text.setText("Button is clicked!");
// Toast...
Toast toast = new Toast(getApplicationContext());
toast.setGravity(Gravity.CENTER_VERTICAL, 0, 0);
toast.setDuration(Toast.LENGTH_LONG);
toast.setView(layout);
toast.show();
}
});
}
}
click below button to copy the code from android tutorial team
- See Demo - android emulator - android tutorial, when a button is clicked, display the custom Toast message.
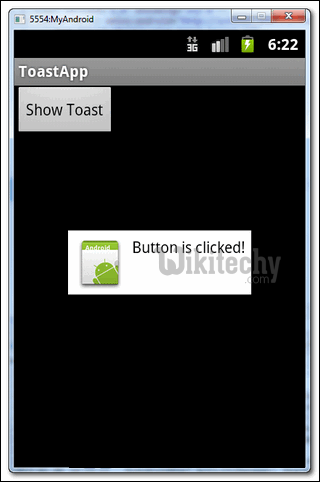