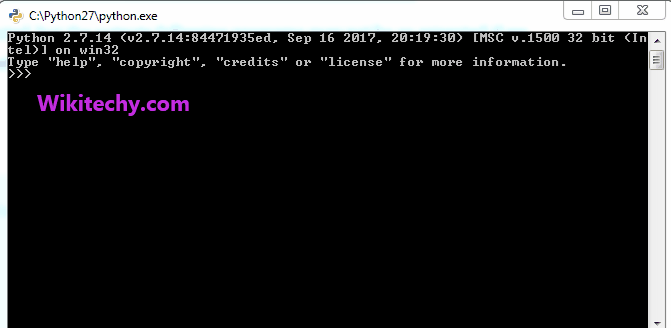
Learn Python - Python tutorial - python operator - Python examples - Python programs
Most of the languages including C, C++, Java and Python provide the boolean type that can be either set to False or True.
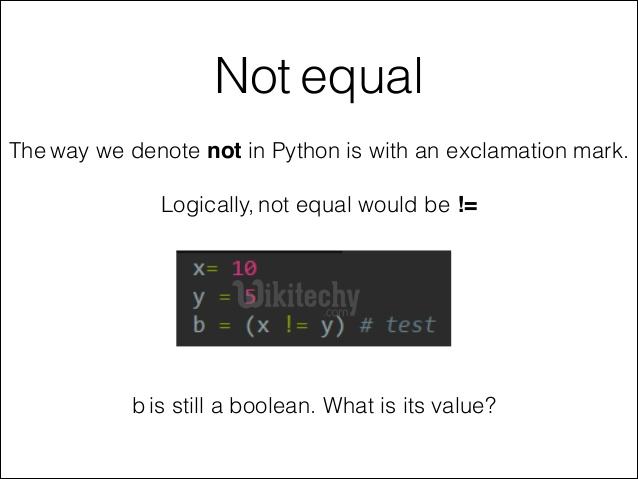
Consider below programs that use Logical Not (or !) operator on boolean.
python - Sample - python code :
# A Python program that uses Logical Not or ! on boolean
a = not True
b = not False
print a
print b
# Output: False
# True
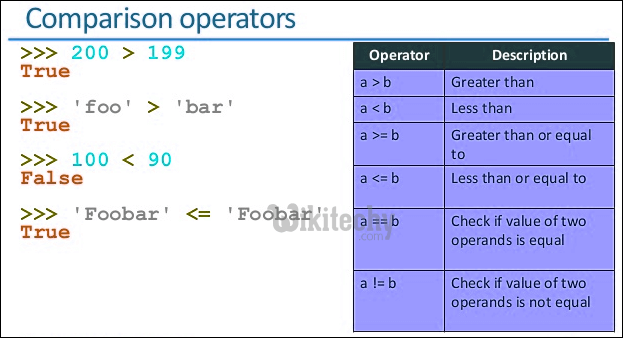
The outputs of above programs are as expected, but the outputs following programs may not be as expected if we have not used Bitwise Not (or ~) operator before.
python - Sample - python code :
# A Python program that uses Bitwise Not or ~ on boolean
a = True
b = False
print ~a
print ~b
python programming - Output :
-2
-1
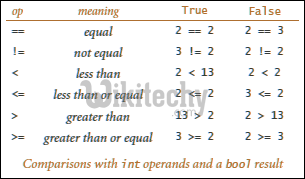
Reason: The bitwise not operator ~ returns the complement of a number i.e., it switches each 1 to 0 and each 0 to 1. Booleans True and False have values 1 and 0 respectively.
˜being the bitwise not operator,
- The expression “˜True” returns bitwise inverse of 1.
- The expression “˜False” returns bitwise inverse of 0.
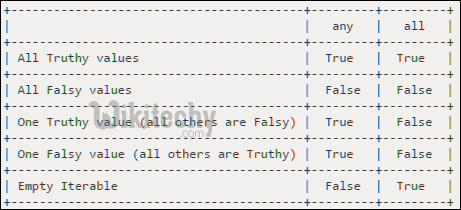
Java doesn’t allow ~ operator to be applied on boolean values. For example, the below program produces compiler error.
python - Sample - python code :
// A Java program that uses Bitwise Not or ~ on boolean
import java.io.*;
class GFG
{
public static void main (String[] args)
{
boolean a = true, b = false;
System.out.println(~a);
System.out.println(~b);
}
}
python programming - Output :
6: error: bad operand type boolean for unary operator '~'
System.out.println(~a);
^
7: error: bad operand type boolean for unary operator '~'
System.out.println(~b);
^
2 errors
Conclusion:
“Logical not or !” is meant for boolean values and “bitwise not or ~” is for integers. Languages like C/C++ and python do auto promotion of boolean to integer type when an integer operator is applied. But Java doesn’t do it.