python tutorial - Python interview questions - learn python - python programming
python interview questions :101
Print in terminal with colors using Python?
- This somewhat depends on what platform you are on. The most common way to do this is by printing ANSI escape sequences. For a simple example, here's some python code from the blender build scripts:
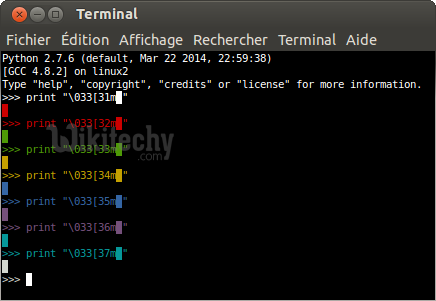
Learn python - python tutorial - colors - python examples - python programs
class bcolors:
HEADER = '\033[95m'
OKBLUE = '\033[94m'
OKGREEN = '\033[92m'
WARNING = '\033[93m'
FAIL = '\033[91m'
ENDC = '\033[0m'
BOLD = '\033[1m'
UNDERLINE = '\033[4m'
click below button to copy the code. By Python tutorial team
python interview questions :102
How to convert string to lowercase in Python?
- Is there any way to convert an entire user inputted string from uppercase, or even part uppercase to lowercase.
- E.g. Kilometers --> kilometers
- This doesn't work for non-English words in UTF-8. In this case decode('utf-8') can help:
>>> s='Километр'
>>> print s.lower()
Километр
>>> print s.decode('utf-8').lower()
километр
click below button to copy the code. By Python tutorial team
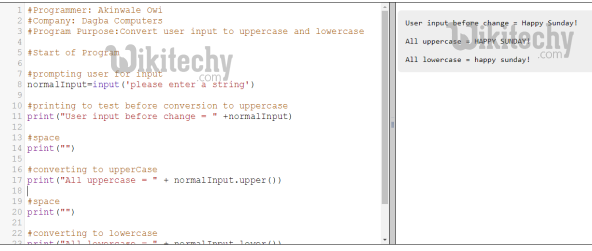
Learn python - python tutorial - lowercase - python examples - python programs
- It will then automatically convert the thing they typed into lowercase.
raw_input('Type Something').lower()
click below button to copy the code. By Python tutorial team
Note: raw_input
was renamed to input
in Python 3.x and above
python interview questions :103
How can I remove (chomp) a newline in Python?
- Python's
rstrip()
method strips all kinds of trailing whitespace by default, not just one newline as Perl does withchomp.
>>> 'test string \n \r\n\n\r \n\n'.rstrip()
'test string'
click below button to copy the code. By Python tutorial team
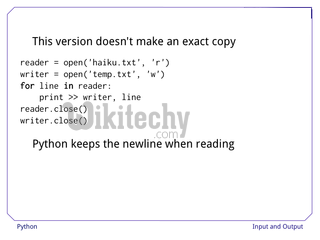
Learn python - python tutorial - chomp - python examples - python programs
- To strip only newlines:
>>> 'test string \n \r\n\n\r \n\n'.rstrip('\n')
'test string \n \r\n\n\r '
click below button to copy the code. By Python tutorial team
- There are also the methods
lstrip()
andstrip():
>>> s = " \n\r\n \n abc def \n\r\n \n "
>>> s.strip()
'abc def'
>>> s.lstrip()
'abc def \n\r\n \n '
>>> s.rstrip()
' \n\r\n \n abc def'
click below button to copy the code. By Python tutorial team
python interview questions :104
- The
index()
function only returns the first occurrence, whileenumerate()
returns all occurrences. - As a list comprehension:
[i for i, j in enumerate(['foo', 'bar', 'baz']) if j == 'bar']
click below button to copy the code. By Python tutorial team
- This is more efficient for larger lists than using
enumerate():
$ python -m timeit -s "from itertools import izip as zip, count" "[i for i, j in zip(count(), ['foo', 'bar', 'baz']*500) if j == 'bar']"
10000 loops, best of 3: 174 usec per loop
$ python -m timeit "[i for i, j in enumerate(['foo', 'bar', 'baz']*500) if j == 'bar']"
10000 loops, best of 3: 196 usec per loop
click below button to copy the code. By Python tutorial team
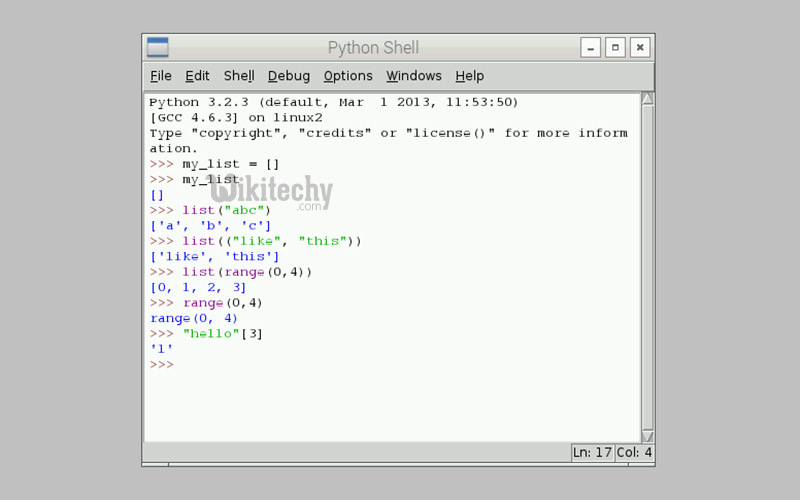
Learn python - python tutorial - index - python examples - python programs
python interview questions :105
Python method to check if a string is a float ?
- A longer and more accurate name for this function could be:
isConvertibleToFloat(value)
def isfloat(value):
try:
float(value)
return True
except:
return False
click below button to copy the code. By Python tutorial team
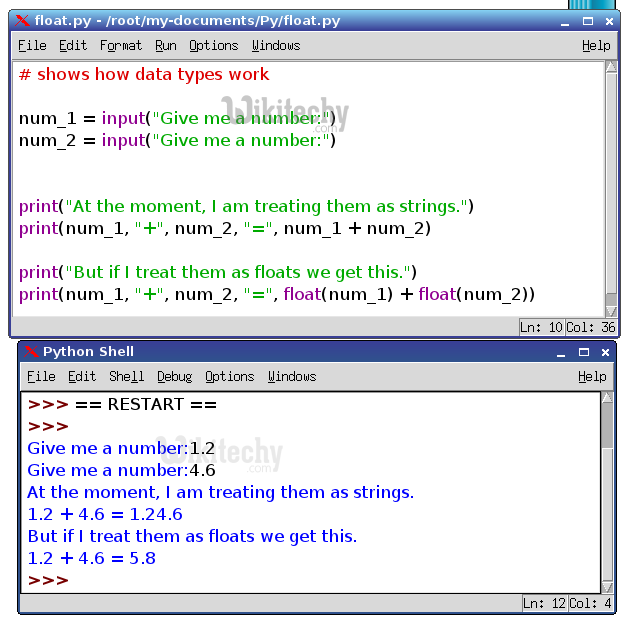
Learn python - python tutorial - float - python examples - python programs
python interview questions :106
Hidden features of Python [closed] ?
- Try to limit answers to Python core.
- One feature per answer.
- Give an example and short description of the feature, not just a link to documentation.
- Label the feature using a title as the first line.
python interview questions :107
Get the python regex parse tree to debug your regex ?
- Regular expressions are a great feature of python, but debugging them can be a pain, and it's all too easy to get a regex wrong.
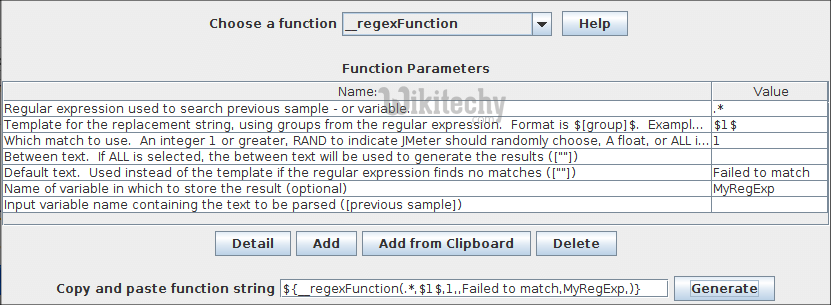
Learn python - python tutorial - hidden - python examples - python programs
- Fortunately, python can print the regex parse tree, by passing the undocumented, experimental, hidden flag
re.DEBUG
(actually, 128) tore.compile.
>>> re.compile("^\[font(?:=(?P<size>[-+][0-9]{1,2}))?\](.*?)[/font]",
re.DEBUG)
at at_beginning
literal 91
literal 102
literal 111
literal 110
literal 116
max_repeat 0 1
subpattern None
literal 61
subpattern 1
in
literal 45
literal 43
max_repeat 1 2
in
range (48, 57)
literal 93
subpattern 2
min_repeat 0 65535
any None
in
literal 47
literal 102
literal 111
literal 110
literal 116
click below button to copy the code. By Python tutorial team
python interview questions :108
What is iter() can take a callable argument?
- For instance:
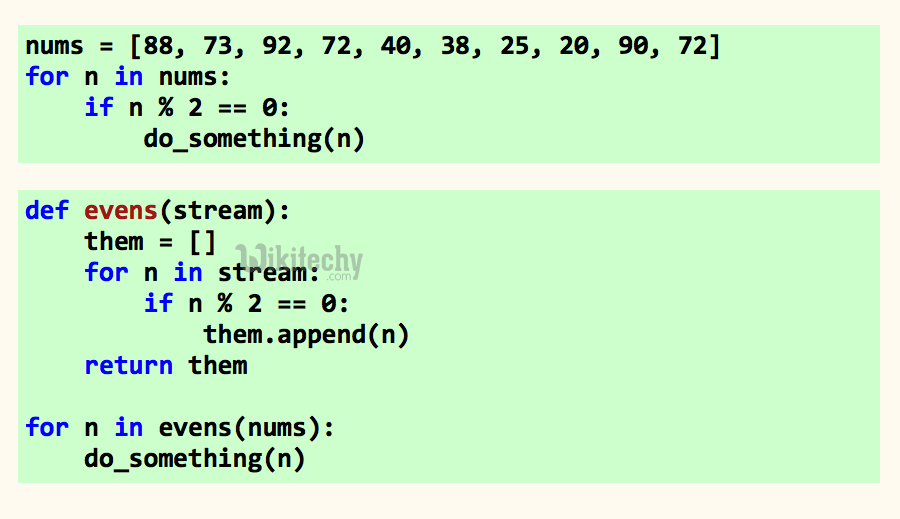
Learn python - python tutorial - iter - python examples - python programs
def seek_next_line(f):
for c in iter(lambda: f.read(1),'\n'):
pass
click below button to copy the code. By Python tutorial team
- The iter
(callable, until_value)
function repeatedly calls callable and yields its resultuntil until_value
is returned.
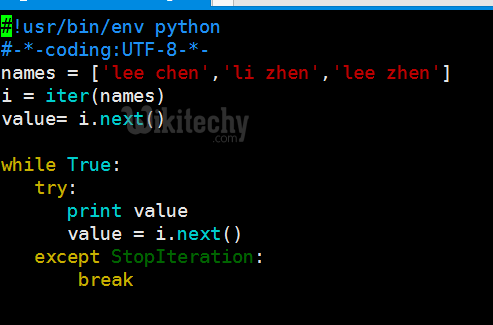
Learn python - python tutorial - iter-execution - python examples - python programs
python interview questions :109
What is the difference between @staticmethod and @classmethod in Python?
Classmethods :
- The static method does not take the instance as an argument.
- It is very similar to a module level function.
- However, a module level function must live in the module and be specially imported to other places where it is used.
a.class_foo(1)
# executing class_foo(<class '__main__.A'>,1)
click below button to copy the code. By Python tutorial team
- You can also call
class_foo
using the class. In fact, if you define something to be a classmethod, it is probably because you intend to call it from the class rather than from a class instance.A.foo(1)
would have raised a TypeError, butA.class_foo(1)
works just fine
A.class_foo(1)
# executing class_foo(<class '__main__.A'>,1)
click below button to copy the code. By Python tutorial team
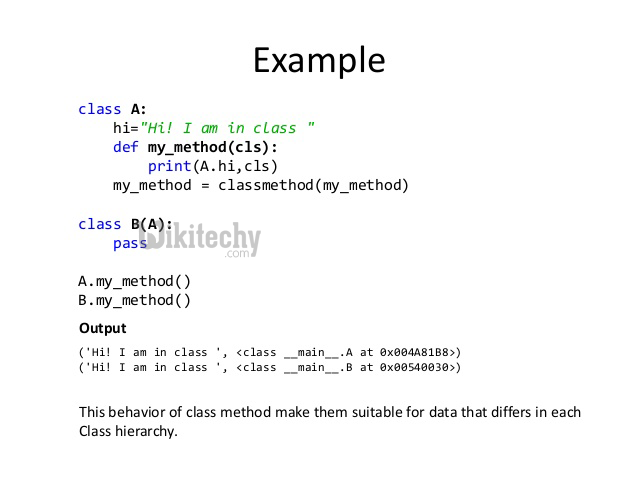
Learn python - python tutorial - static-method - python examples - python programs
- Staticmethods :
- A class method is a similar to an instance method in that it takes an implicit first argument, but instead of taking the instance, it takes the class.
- Frequently these are used as alternative constructors for better semantic usage and it will support inheritance.
- Neither
self
(the object instance) norcls
(the class) is implicitly passed as the first argument.
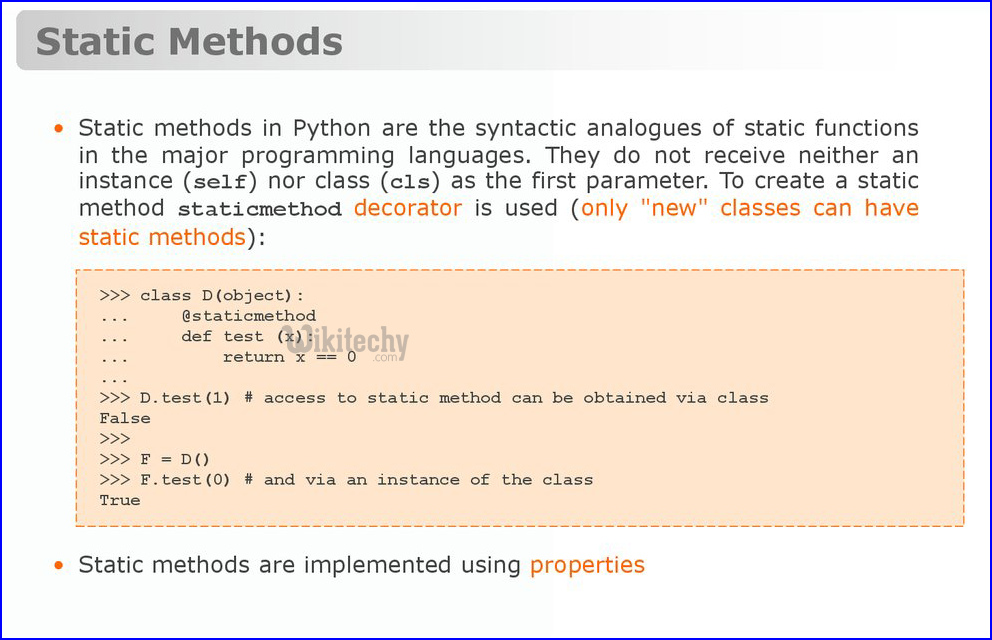
Learn python - python tutorial - static-method-implementation - python examples - python programs
- They behave like plain functions except that you can call them from an instance or the class:
a.static_foo(1)
# executing static_foo(1)
A.static_foo('hi')
# executing static_foo(hi)
click below button to copy the code. By Python tutorial team
- Staticmethods are used to group functions which have some logical connection with a class to the class
python interview questions :110
How can I make a time delay in Python?
- In a single thread I suggest the sleep function:
>>> from time import sleep
>>> sleep(4)
click below button to copy the code. By Python tutorial team
- This actually suspends the processing of the thread in which it is called by the operating system, allowing other threads and processes to execute while it sleeps.
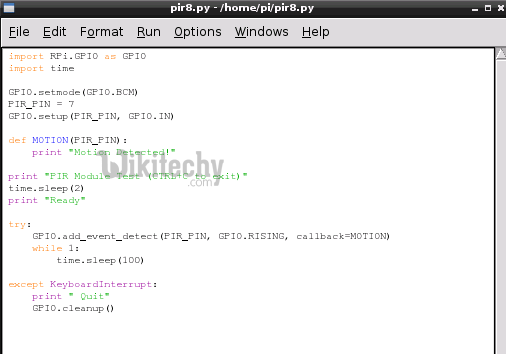
Learn python - python tutorial - time-delay - python examples - python programs
- Use it for that purpose, or simply to delay a function from executing. For example:
>>> def party_time():
... print('hooray!')
...
>>> sleep(3); party_time()
hooray!
click below button to copy the code. By Python tutorial team
"hooray!" printed 3 seconds after I hit Enter.