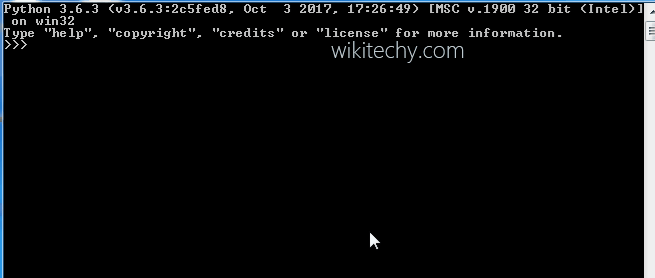
Learn Python - Python tutorial - python reverse looping - Python examples - Python programs
Python supports various looping techniques by certain inbuilt functions, in various sequential containers. These methods are primarily verya useful in competitive programming and also in various project which require a specific technique with loops maintaining the overall structure of code.
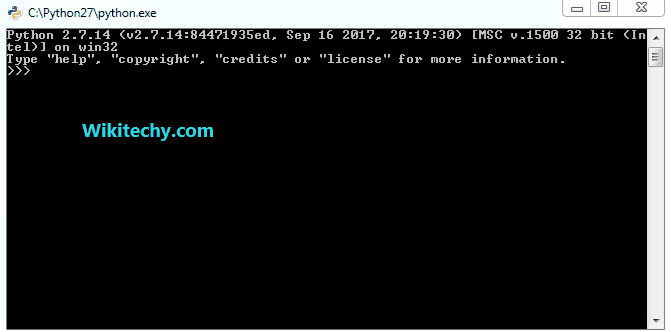
Learn Python - Python tutorial - python loops - Python examples - Python programs
Where they are used ?
Different looping techniques are primarily useful in the places where we don’t need to actually manipulate the structure and ordering of overall container, rather only print the elements for a single use instance, no inplace change occurs in the container. This can also be used in instances to save time.
Different looping techniques using Python data structures are:
- Using enumerate(): enumerate() is used to loop through the containers printing the index number along with the value present in that particular index.
- Using zip(): zip() is used to combine 2 similar containers(list-list or dict-dict) printing the values sequentially. The loop exists only till the smaller container ends. The detailed explanation of zip() and enumerate() can be found here.
- Using iteritem(): iteritems() is used to loop through the dictionary printing the dictionary key-value pair sequentially.
- Using items(): items() performs the similar task on dictionary as iteritems() but have certain disadvantages when compared with iteritems().
- It is very time consuming. Calling it on large dictionaries consumes quite a lot of time.
- It takes a lot of memory. Sometimes takes double the memory when called on dictionary.
python - Sample - python code :
# Python code to demonstrate working of iteritems(),items()
d = { "wikitechy" : "worlds best", "e-learning" : "website" }
# using iteritems to print the dictionary key-value pair
print ("The key value pair using iteritems is : ")
for i,j in d.iteritems():
print i,j
# using items to print the dictionary key-value pair
print ("The key value pair using items is : ")
for i,j in d.items():
print i,j
python tutorial - Output :
The key value pair using iteritems is : wikitechy worlds best e-learning website The key value pair using items is : wikitechy worlds best e-learning website
- Using sorted(): sorted() is used to print the container is sorted order. It doesn’t sort the container, but just prints the container in sorted order for 1 instance. Use of set() can be combined to remove duplicate occurrences.
python - Sample - python code :
# Python code to demonstrate working of sorted()
# initializing list
lis = [ 1 , 3, 5, 6, 2, 1, 3 ]
# using sorted() to print the list in sorted order
print ("The list in sorted order is : ")
for i in sorted(lis) :
print (i,end=" ")
print ("\r")
# using sorted() and set() to print the list in sorted order
# use of set() removes duplicates.
print ("The list in sorted order (without duplicates) is : ")
for i in sorted(set(lis)) :
print (i,end=" ")
python tutorial - Output :
The list in sorted order is : 1 1 2 3 3 5 6 The list in sorted order (without duplicates) is : 1 2 3 5 6
- Using reversed(): reversed() is used to print the values of container in the descending order as declared.
python - Sample - python code :
# Python code to demonstrate working of reversed()
# initializing list
lis = [ 1 , 3, 5, 6, 2, 1, 3 ]
# using revered() to print the list in reversed order
print ("The list in reversed order is : ")
for i in reversed(lis) :
print (i,end=" ")
python tutorial - Output :
The list in reversed order is : 3 1 2 6 5 3 1
Advantage of using above techniques over for, while loop
- These techniques are quick to use and reduces coding effort. for, while loops needs the entire structure of container to be changed.
- These Looping techniques do not require any structural changes to container. They have keywords which present the exact purpose of usage. Whereas, no pre-predictions or guesses can be made in for, while loop i.e not easily understandable the purpose at a glance.
- Looping technique make the code more concise than using for, while loopings.