python tutorial - Python scripting interview questions and answers - learn python - python programming
python interview questions :221
How to implement Quicksort?
- Quicksort is the fastest known comparison-based sorting algorithm.
- It requires O(n log n) steps on average while works in place, which means minimal extra memory required. Quicksort is a recursive algorithm.
def qsort(a,left_index,right_index):
if left_index > right_index:
return
left = left_index
right = right_index
# pivot elem
pivot = a[(left+right)/2]
# partition
while left <= right:
while(a[left] < pivot): left += 1
while(a[right] > pivot): right -= 1
if(left <= right):
a[left], a[right] = a[right], a[left]
left += 1
right -= 1
print(a);
# recursion
qsort(a,left_index,right)
qsort(a,left,right_index)
a = range(10)
random.shuffle(a)
print a
qsort(a,0,len(a)-1);
click below button to copy the code. By Python tutorial team
Output:
[5, 7, 8, 6, 9, 0, 2, 3, 1, 4]
[5, 7, 8, 6, 4, 0, 2, 3, 1, 9]
[5, 7, 8, 6, 4, 0, 2, 3, 1, 9]
[1, 7, 8, 6, 4, 0, 2, 3, 5, 9]
[1, 3, 8, 6, 4, 0, 2, 7, 5, 9]
[1, 3, 2, 6, 4, 0, 8, 7, 5, 9]
[1, 3, 2, 0, 4, 6, 8, 7, 5, 9]
[1, 3, 2, 0, 4, 6, 8, 7, 5, 9]
[1, 0, 2, 3, 4, 6, 8, 7, 5, 9]
[1, 0, 2, 3, 4, 6, 8, 7, 5, 9]
[0, 1, 2, 3, 4, 6, 8, 7, 5, 9]
[0, 1, 2, 3, 4, 6, 8, 7, 5, 9]
[0, 1, 2, 3, 4, 6, 8, 7, 5, 9]
[0, 1, 2, 3, 4, 6, 8, 7, 5, 9]
[0, 1, 2, 3, 4, 6, 8, 7, 5, 9]
[0, 1, 2, 3, 4, 6, 5, 7, 8, 9]
[0, 1, 2, 3, 4, 6, 5, 7, 8, 9]
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
python interview questions :222
How to use Regular expression ?
Write a code to check the validity of password input. Then following are the criteria for valid password:
- At least 1 letter between [a-z]
- At least 1 number between [0-9]
- At least 1 letter between [A-Z]
- At least 1 character from [$#@]
- Minimum length of password: 6
- Maximum length of password: 12
Here are the list of passwords:
password = ["c#12Z","7@yE*","Zd12$a1","all4@9"]
click below button to copy the code. By Python tutorial team
So, the right output should be like this:
{'Zd12$a1': True, 'all4@9': False, 'c#12Z': False, '7@yE*': False}
click below button to copy the code. By Python tutorial team
python interview questions :223
Which statement of Python is used whenever a statement is required syntactically but the program needs no action?
- Pass - is no-operation / action statement in Python
- If we want to load a module or open a file, and even if the requested module/file does not exist, we want to continue with other tasks. In such a scenario, use try-except block with pass statement in the except block.
try:import mymodulemyfile = open(“C:\myfile.csv”)except:pass
click below button to copy the code. By Python tutorial team
python interview questions :224
What is Web Scraping? How do you achieve it in Python?
- Web Scrapping is a way of extracting the large amounts of information which is available on the web sites and saving it onto the local machine or onto the database tables.
- In order to scrap the web:load the web page which is interesting to you. To load the web page, use “requests” module.
- parse HTML from the web page to find the interesting information.Python has few modules for scraping the web. They are urllib2, scrapy, pyquery, BeautifulSoap, etc.
python interview questions :225
What Is Python? What Are The Benefits Of Using Python?
Python is one of the most successful interpreted languages. When you write a Python script, it doesn’t need to get compiled before execution. Few other interpreted languages are PHP and Javascript.
Benefits Of Python Programming.
- Python is a dynamic-typed language, this means that you don’t need to mention the date type of variables during their declaration. It allows to set variables like var1=101 and var2 =” You are an engineer.” without any error.
- Python supports object orientated programming as you can define classes along with the composition and inheritance. It doesn’t use access specifiers like public or private).
- Functions in Python are like first-class objects. It suggests you can assign them to variables, return from other methods and pass as arguments.
- Developing using Python is quick but running it is often slower than compiled languages. Luckily, Python enables to include the “C” language extensions so you can optimize your scripts.
- Python has several usages like web-based applications, test automation, data modeling, big data analytics and much more. Alternatively, you can utilize it as “glue” layer to work with other languages.
python interview questions :226
What Is The Output Of The Following Python Code Fragment?
def extendList(val, list=[]):
list.append(val)
return list
list1 = extendList(10)
list2 = extendList(123,[])
list3 = extendList('a')
print "list1 = %s" % list1
print "list2 = %s" % list2
print "list3 = %s" % list3
click below button to copy the code. By Python tutorial team
The result of the above Python code snippet is:
list1 = [10, 'a']
list2 = [123]
list3 = [10, 'a']
- You may erroneously expect list1 to be equal to [10] and list3 to be equal to [‘a’], thinking that the list argument will initialize to its default value of [] every time there is a call to the extendList.
- However, the flow is like that a new list gets created once after the function is defined. And the same list is used whenever someone calls the extendList method without a list argument. It works like this because the calculation of expressions (in default arguments) occurs at the time of function definition, not during its invocation.
python interview questions :227
How Do You Debug A Program In Python? Is It Possible To Step Through Python Code?
- Yes, we can use the Python debugger (<pdb>) to debug any Python program. And if we start a program using <pdb>, then it let us even step through the code.
python interview questions :228
List Down Some Of The PDB Commands For Debugging Python Programs?
Here are a few PDB commands to start debugging Python code.
- Add breakpoint - <b>
- Resume execution - <c>
- Step by step debugging - <s>
- Move to next line - <n>
- List source code - <l>
- Print an expression - <p>
python interview questions :229
How can you copy objects in Python?
The functions used to copy objects in Python are-
- Copy.copy () for shallow copy
- Copy.deepcopy () for deep copy
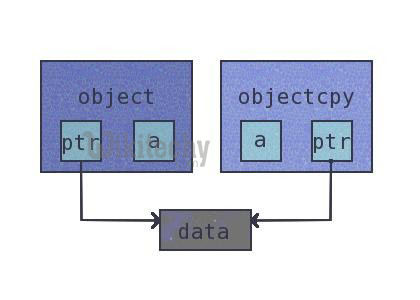
Learn python - python tutorial - python-object-copy - python examples - python programs
- However, it is not possible to copy all objects in Python using these functions. For instance, dictionaries have a separate copy method whereas sequences in Python have to be copied by ‘Slicing’.
python interview questions :240
What is the difference between tuples and lists in Python?
- Tuples can be used as keys for dictionaries i.e. they can be hashed. Lists are mutable whereas tuples are immutable - they cannot be changed.
- Tuples should be used when the order of elements in a sequence matters. For example, set of actions that need to be executed in sequence, geographic locations or list of points on a specific route.
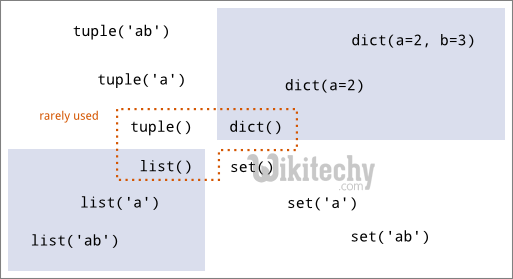
Learn python - python tutorial - python-tuples - python examples - python programs