python tutorial - Python Program To find Area Of Circle - learn python - python programming
Area Of a Circle
- The area of a circle is number of square units inside the circle. Standard formula to calculate the area of a circle is: A=πr².
- How to write python program to find area of circle using radius, circumstance and diameter
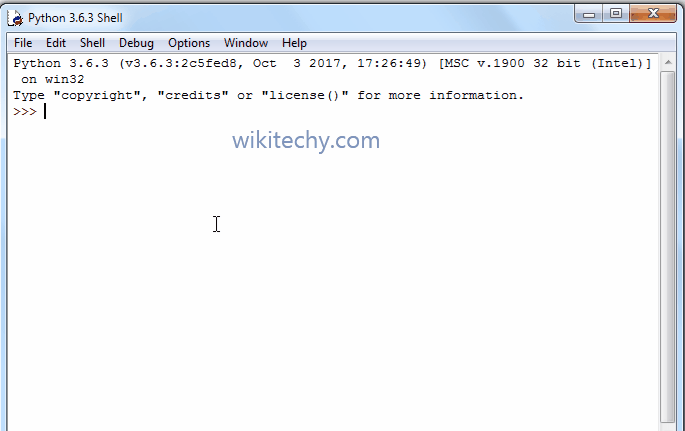
Learn Python - Python tutorial - Python Program To find Area Of Circle - Python examples - Python programs
Python Program to find Area Of Circle using Radius
- If we know the radius then we can calculate the area of a circle using formula: A=πr² (Here A is the area of the circle and r is radius).
Sample Code
# Python Program to find Area Of Circle using Radius
PI = 3.14
radius = float(input(' Please Enter the radius of a circle: '))
area = PI * radius * radius
circumference = 2 * PI * radius
print(" Area Of a Circle = %.2f" %area)
print(" Circumference Of a Circle = %.2f" %circumference)
click below button to copy the code. By Python tutorial team
Analysis:
- We defined pi as global variable and assigned value as 3.14.
- This program allows user to enter the value of a radius and then it will calculate the area of circle as per the formula.
Output
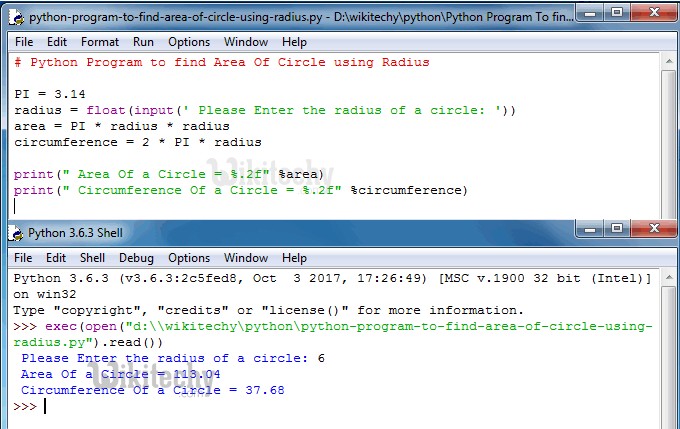
Learn Python - Python tutorial - Python Program to find Area Of Circle using Radius - Python examples - Python programs
Python Program to find Area Of Circle using Circumference
- Distance around the circle is called as circumference. If you know the circumference then we can calculate the area of a circle using formula: A= C²⁄ 4π (Here C is circumference)
Sample Code
# Python Program to find Area Of Circle using Circumference
import math
circumference = float(input(' Please Enter the Circumference of a circle: '))
area = (circumference * circumference)/(4 * math.pi)
print(" Area Of a Circle = %.2f" %area)
click below button to copy the code. By Python tutorial team
Analysis:
- First, We imported the math library and this supports us to use all the mathematical functions in Python programming.
- In this example we can call the PI value using math.pi
import math
click below button to copy the code. By Python tutorial team
- Next line of code allows user to enter the value of a circumference.
circumference = float(input(' Please Enter the Circumference of a circle: '))
click below button to copy the code. By Python tutorial team
- Using the circumference, this program will calculate the area of circle as per the formula: A= C²⁄ 4π
Output
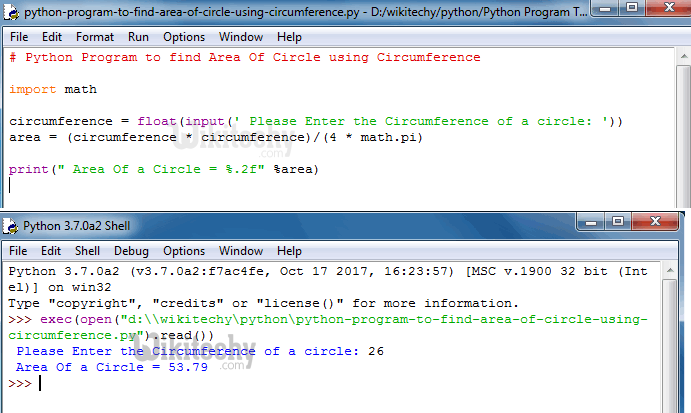
Learn Python - Python tutorial - Python Program to find Area Of Circle using Circumference - Python examples - Python programs
Python Program to Calculate Area Of a Circle using Diameter
- Distance across the circle passing through the center is called as diameter.
- If we know the diameter then we can calculate the area of a circle using formula: A=π/4*D² (D is the diameter)
Sample Code
# Python Program to find Area Of Circle using Diameter
import math
diameter = float(input(' Please Enter the Diameter of a circle: '))
area1 = (math.pi/4) * (diameter * diameter)
# diameter = 2 * radius
# radius = diameter/2
radius = diameter / 2
area2 = math.pi * radius * radius
print(" Area of Circle using direct formula = %.2f" %area1);
print(" Area of Circle Using Method 2 = %.2f" %area2)
click below button to copy the code. By Python tutorial team
Analysis:
- This program allows user to enter the value of a diameter and then it will calculate the area of circle as per the formula we shown above.
We also mentioned other approach.
diameter = 2 * radius
radius = diameter/2
area = π * radius * radius
Output
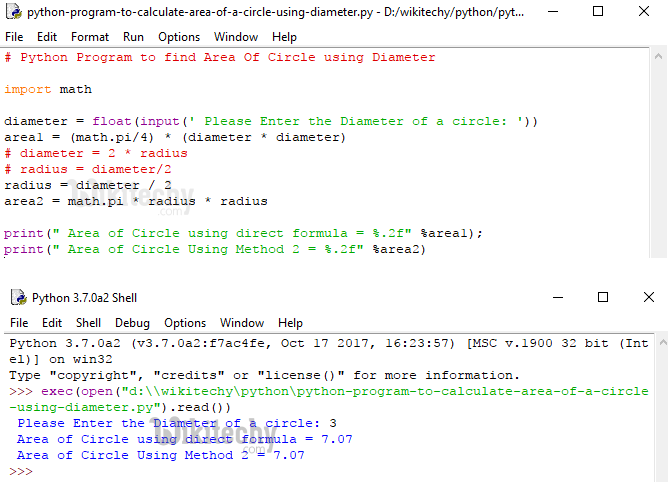
Learn Python - Python tutorial - Python Program to Calculate Area Of a Circle using Diameter - Python examples - Python programs