Running your First Code in Python
Python programs are not compiled, rather they are interpreted. Now, let us move to writing a python code and running it. Please make sure that python is installed on the system you are working. If it is not installed, download it from here. We will be using python 2.7.
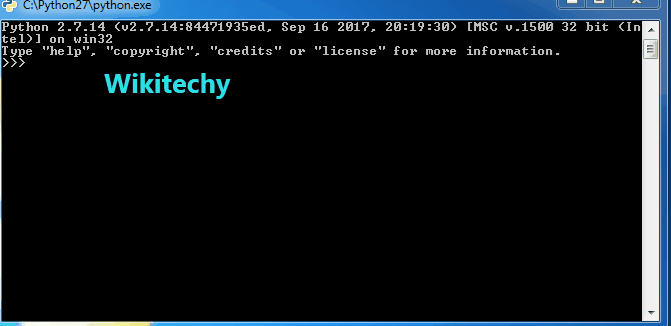
Learn Python - Python tutorial - python sum - Python examples - Python programs
Making a Python file:
Python files are stored with the extension “.py”. Open text editor and save a file with the name “hello.py”. Open it and write the following code:
python - Sample - python code :
print "Hello World"
# Notice that NO semi-colon is to be used
click below button to copy the code. By Python tutorial team
Reading the file contents:
Linux System – Move to the directory from terminal where the created file (hello.py) is stored by using the ‘cd’ command, and then type the following in the terminal :
python - Sample - python code :
python hello.py
click below button to copy the code. By Python tutorial team
Windows system – Open command prompt and move to the directory where the file is stored by using the ‘cd’ command and then run the file by writing the file name as command.
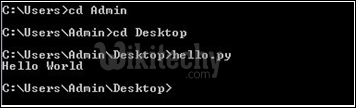
Variables in Python
Variables need not be declared first in python. They can be used directly. Variables in python are case sensitive as most of the other programming languages.
Example:
python - Sample - python code :
a = 3
A = 4
print a
print A
click below button to copy the code. By Python tutorial team
python tutorial - Output :
3
4
Expressions in Python
Arithmetic operations in python can be performed by using arithmetic operators and some of the in-built functions.
python - Sample - python code :
a = 2
b = 3
c = a + b
print c
d = a * b
print d
click below button to copy the code. By Python tutorial team
python tutorial - Output :
5
6
Conditions in Python
Conditional output in python can be obtained by using if-else and elif (else if) statements.
python - Sample - python code :
a = 3
b = 9
if b % a == 0 :
print "b is divisible by a"
elif b + 1 == 10:
print "Increment in b produces 10"
else:
print "You are in else statement"
click below button to copy the code. By Python tutorial team
python tutorial - Output :
b is divisible by a
Functions in Python
A function in python is declared by the keyword ‘def’ before the name of the function. The return type of the function need not be specified explicitly in python. The function can be invoked by writing the function name followed by the parameter list in the brackets.
python - Sample - python code :
# Function for checking the divisibility
# Notice the indentation after function declaration
# and if and else statements
def checkDivisibility(a, b):
if a % b == 0 :
print "a is divisible by b"
else:
print "a is not divisible by b"
#Driver program to test the above function
checkDivisibility(4, 2)
click below button to copy the code. By Python tutorial team
python tutorial - Output :
a is divisible by b
So, python is a very simplified and less cumbersome language to code in. This easiness of python is itself promoting its wide use.