python tutorial - Programming interview questions python - learn python - python programming
python interview questions :31
Linting Python: what is good?
- I tried the first example and PyLint 0.18.1 gave me the warning:
W: 1:spam: Dangerous default value [] as argument
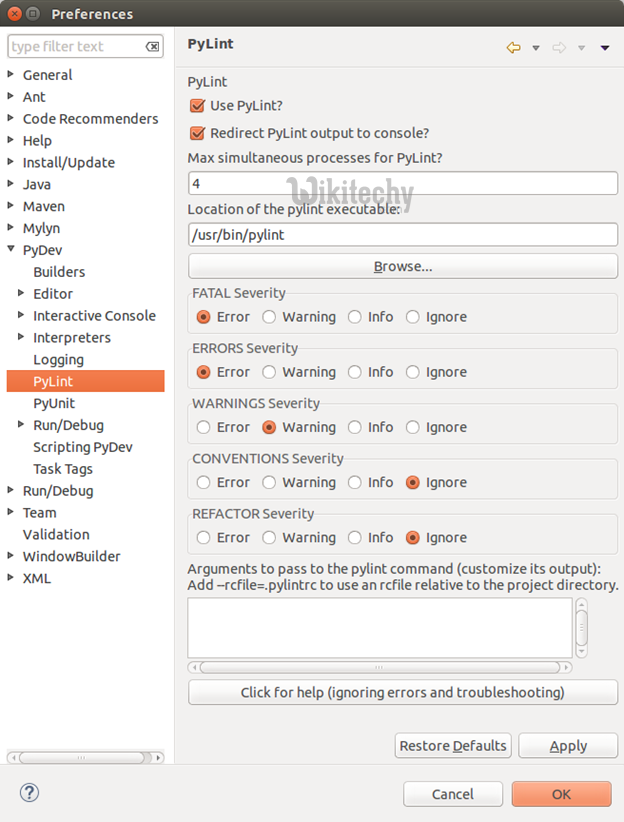
Learn python - python tutorial - lint - python examples - python programs
python interview questions :32
Python what is '*' used for ?
- This is called the "splat" operator. For more information on it, see the Python documentation on it.
- What it's basically doing is this:
- print(reversed(binary)[0], reversed(binary)[1], ..., sep='')
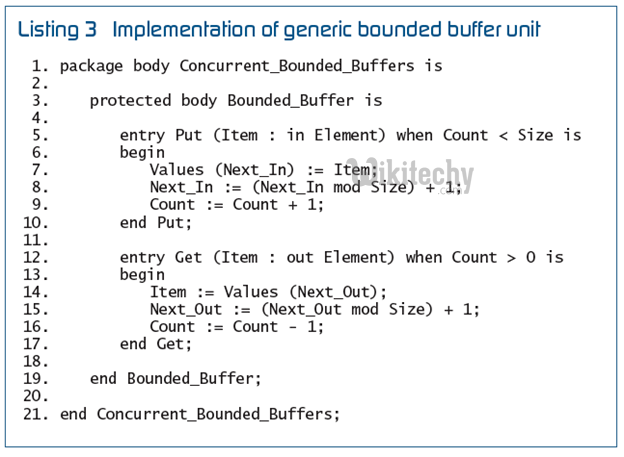
Learn python - python tutorial - generic - python examples - python programs
- Essentially, it uses the array elements as arguments instead of passing the array as a single argument itself.
>>> lst = [1, 2, 3]
>>> print(lst) # equivalent to `print([1, 2, 3])'
<b>[1, 2, 3] </b>
>>> print(*lst) # equivalent to `print(1, 2, 3)'
<b>1 2 3</b>
>>> print(reversed(lst))
<list_reverseiterator object at 0x7f4863ae4a20>
>>> print(*reversed(lst))
<b>3 2 1</b>
click below button to copy the code. By Python tutorial team
python interview questions :33
Explain Inheritance in Python with an example.
- Inheritance allows One class to gain all the members (say attributes and methods) of another class. Inheritance provides code reusability, makes it easier to create and maintain an application. They are different types of inheritance supported by Python.
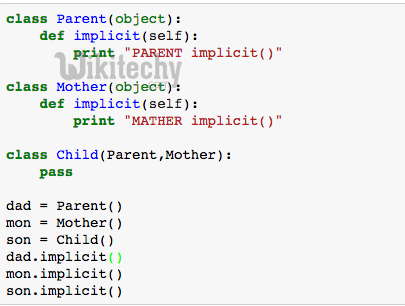
Learn python - python tutorial - inheritance - python examples - python programs
- They are: single, multi-level, hierarchical and multiple inheritance. The class from which we are inheriting is called super-class and the class that is inherited is called a derived / child class.
- Single Inheritance - where a derived class acquires the members of a single super class.
- multi-level inheritance - a derived class d1 in inherited from base class base1, and d2 is inherited from base2.
- hierarchical inheritance - from one base class you can inherit any number of child classes
- multiple inheritance - a derived class is inherited from more than one base class.
class ParentClass:
v1 = "from ParentClass - v1"
v2 = "from ParentClass - v2"class ChildClass(ParentClass):
passc = ChildClass() print(c.v1) print(c.v2)
click below button to copy the code. By Python tutorial team
python interview questions :34
What is multithreading? Give an example.
- It means running several different programs at the same time concurrently by invoking multiple threads. Multiple threads within a process refer the data space with main thread and they can communicate with each other to share information more easily.
- Threads are light-weight processes and have less memory overhead.
- Threads can be used just for quick task like calculating results and also running other processes in the background while the main program is running.
python interview questions :35
How instance variables are different from class variables?
Instance variables:
- The variables in an object that have values that are local to that object.
- Two objects of the same class maintain distinct values for their variables. These variables are accessed with “object-name.instancevariable-name”.
class variables:
- These are the variables of class. All the objects of the same class will share value of “Class variables. They are accessed with their class name alone as “class- name.classvariable-name”.
- If you change the value of a class variable in one object, its new value is visible among all other objects of the same class. In the Java world, a variable that is declared as static is a class variable.
python interview questions :36
Explain different ways to trigger / raise exceptions in your python script ?
- The following are the two possible ways by which you can trigger an exception in your Python script. They are:
raise - it is used to manually raise an exception general-form: raise exception-name (“message to be conveyed”).
>>> voting_age = 15
>>> if voting_age < 18: raise ValueError(“voting age should be atleast 18 and above”) output: ValueError: voting age should be atleast 18 and above 2. assert statement assert statements are used to tell your program to test that condition attached to assert keyword, and trigger an exception whenever the condition becomes false. Eg: >>> a = -10
>>> assert a > 0 #to raise an exception whenever a is a negative number
click below button to copy the code. By Python tutorial team
output:
AssertionError
Another way of raising and exception can be done by making a programming mistake, but that’s not
usually a good way of triggering an exception.
python interview questions :37
How is Inheritance and Overriding methods are related?
- If class A is a sub class of class B, then everything in B is accessible in /by class A. In addition, class A can define methods that are unavailable in B, and also it is able to override methods in B.
- For Instance, If class B and class A both contain a method called func(), then func() in class B can override func() in class A.
- Similarly, a method of class A can call another method defined in A that can invoke a method of B that overrides it.
python interview questions :38
Which methods of Python are used to determine the type of instance and inheritance?
Python has 2 built-in functions that work with inheritance:
- isinstance() - this method checks the type of instance.
- for eg: isinstance(myObj, int) - returns True only when “myObj. class ” is “int”.
- issubclass() - this method checks class inheritance
- for eg: issubclass(bool, int) - returns True because “bool” is a subclass of “int”.
- issubclass(unicode, str) - returns False because “unicode” is not a subclass of “str”.
python interview questions :39
In the case of Multiple inheritance, if a child class C is derived from two base classes say A and B as: class C(A, B): which parent class's method will be invoked by the interpreter whenever object of class C calls a method func() that is existing in both the parent classes say A and B and does not exist in class C as
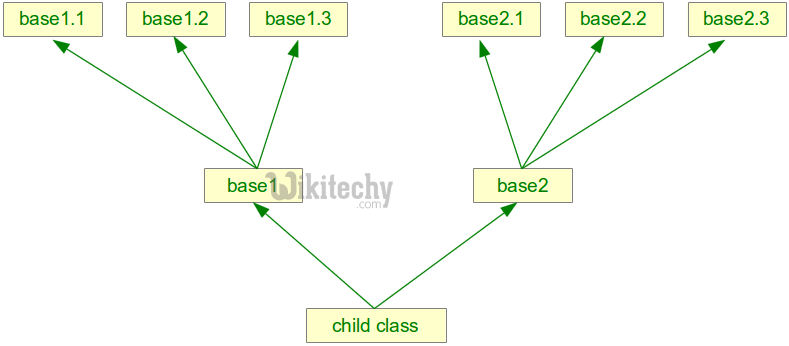
Learn python - python tutorial - multiple-inheritance - python examples - python programs
- since class C does not contain the definition of the method func(), they Python searches for the func() in parent classes. Since the search is performed in a left-to-right fashion, Python executes the method func() present in class A and not the func() method in B.
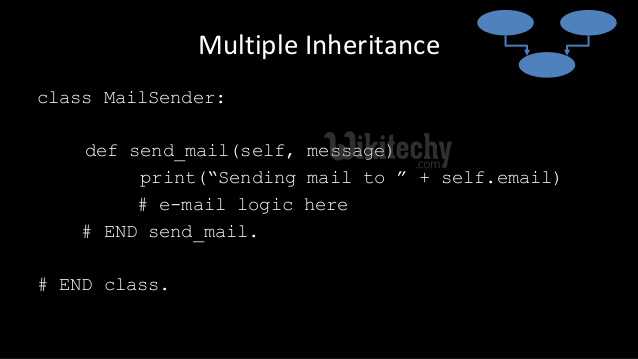
Learn python - python tutorial - multiple-inheritance-one - python examples - python programs
python interview questions :40
Does Python supports interfaces like in Java? Discuss.
- Python does not provide interfaces like in Java. Abstract Base Class (ABC) and its feature are provided by the Python’s “abc” module.
- Abstract Base Class is a mechanism for specifying what methods must be implemented by its implementation subclasses.
- The use of ABC’c provides a sort of “understanding” about methods and their expected behaviour. This module was made available from Python 2.7 version onwards.
- The .pyc file is having byte code which is platform independent and can be executed on any operating system that supports .pyc format.

Learn python - python tutorial - interface - python examples - python programs