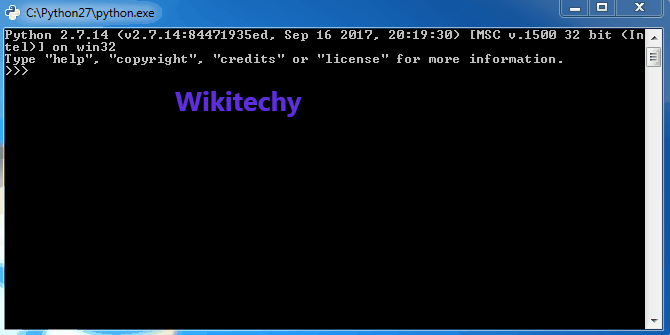
Learn Python - Python tutorial - python matrix - Python examples - Python programs
Transpose of a matrix is a task we all can perform very easily in python (Using a nested loop). But there are some interesting ways to do the same in a single line.
In Python, we can implement a matrix as nested list (list inside a list). Each element is treated as a row of the matrix. For example m = [[1, 2], [4, 5], [3, 6]] represents a matrix of 3 rows and 2 columns.
First element of the list – m[0] and element in first row, first column – m[0][0].
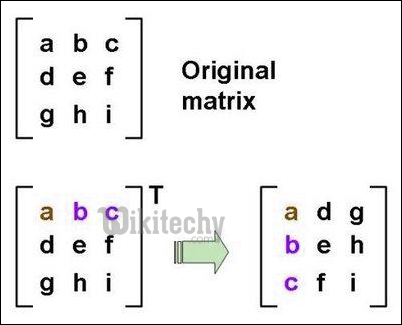
- Using Nested List Comprehension: Nested list comprehension are used to iterate through each element in the matrix.In the given example ,we iterate through each element of matrix (m) in column major manner and assign the result to rez matrix which is the transpose of m.
python - Sample - python code :
m = [[1,2],[3,4],[5,6]]
for row in m :
print(row)
rez = [[m[j][i] for j in range(len(m))] for i in range(len(m[0]))]
print("\n")
for row in rez:
print(row)
click below button to copy the code. By Python tutorial team
python tutorial - Output :
[1, 2]
[3, 4]
[5, 6]
[1, 3, 5]
[2, 4, 6]
- Using zip: Zip returns an iterator of tuples, where the i-th tuple contains the i-th element from each of the argument sequences or iterables. In this example we unzip our array using * and then zip it to get the transpose.
python - Sample - python code :
matrix=[(1,2,3),(4,5,6),(7,8,9),(10,11,12)]
for row in matrix:
print(row)
print("\n")
t_matrix = zip(*matrix)
for row in t_matrix:
print row
click below button to copy the code. By Python tutorial team
python tutorial - Output :
(1, 2, 3)
(4, 5, 6)
(7, 8, 9)
(10, 11, 12)
(1, 4, 7, 10)
(2, 5, 8, 11)
(3, 6, 9, 12)
Note :- If you want your result in the form [[1,4,7,10][2,5,8,11][3,6,9,12]] , you can use t_matrix=map(list, zip(*matrix)).
- Using numpy: NumPy is a general-purpose array-processing package designed to efficiently manipulate large multi-dimensional arrays. The transpose method returns a transposed view of the passed multi-dimensional matrix.
python - Sample - python code :
# You need to install numpy in order to import it
# Numpy transpose returns similar result when
# applied on 1D matrix
import numpy
matrix=[[1,2,3],[4,5,6]]
print(matrix)
print("\n")
print(numpy.transpose(matrix))