python tutorial - Running Python Programs - (Os, Sys, Import) - learn python - python programming
Interactive Running
- Python interpreter executes the code entered on each line immediately, when the Enter key is pressed.
- For example, if we type print statement at the >>> prompt, the output is echoed back right away.
>>>
>>> print 'interactive running'
interactive running
>>>
click below button to copy the code. By Python tutorial team
- The interactive prompt runs code and echoes results as we go, however, it doesn't save our code in a file.
- Still, the interactive prompt is a good place to do experiment and testing program files on the fly.
- The interactive interpreter is an ideal place to test code we've written in files.
- We can import our module files interactively and run tests on the tools they define by typing calls at the interactive prompt.
Interactive Run with os.getcwd()
- For example, the following tests a function in a precoded module that ships with Python in its standard library.
- It prints the name of the directory we're currently working in. But we can do the same if we have our own:
>>>
>>> import os
>>> os.getcwd()
'C:\\TEST'
>>>
click below button to copy the code. By Python tutorial team
- The os module contains variety of functions to get information on.
- In some cases, to manipulate local directories, files, processes, and environment variables.
- Python does its best to offer a unified API across all supported operating systems so our programs can run on any computer with as little platform-specific code as possible.
- The current working directory is an invisible property that Python holds in memory at all times.
- There is always a current working directory, whether we're in the Python Shell, running our own Python script from the command line, or whatever.
>>> os.chdir(SUBDIR)
>>> print(os.getcwd())
C:\TEST\SUBDIR
>>>
click below button to copy the code. By Python tutorial team
- We can a Linux-style pathname (forward slashes, no drive letter) even though we're on Windows.
- This is one of the places where Python tries to paper over the differences between operating systems.
>>> os.chdir('/TEST')
>>> print(os.getcwd())
C:\TEST
click below button to copy the code. By Python tutorial team
- We uses the os.getcwd() function to get the current working directory.
- When er run the graphical Python Shell, the current working directory starts as the directory where the Python Shell executable is. On Windows, this depends on where we installed Python; the default directory is c:\Python32.
- If we run the Python Shell from the command line, the current working directory starts as the directory we were in when we ran python3.
Tips for Using Interactive Prompt
- There are a few tips for the beginners when using interactive prompt:
- Python commands only
- print statements are required in files
- Don't indent at the interactive prompt
- Compound (multiline) statement
- We can only type Python code, not system commands.
- Though there are ways to run system commands (e.g., with os.system), but they are not as direct as typing the command itself.
>>>
>>> os.system
<built-in function system>
>>>
click below button to copy the code. By Python tutorial team
Print statements are required in files
- We must use print to see our output when we write a file.
Don't indent at the interactive prompt
- Any blank space to the left of our code is considered as nested statement. A leading space generates an error message.
Compound (multiline) statement
- Be sure to terminate multiline compound statements like for loop at the interactive prompt with a blank line.
- We must press the Enter key twice to terminate the whole multiline statement and then make it run.
- For example:
>>>
>>> for x in 'python':
... print(x)
File "<stdin>", line 2
print(x)
^
IndentationError: expected an indented block
>>>
click below button to copy the code. By Python tutorial team
- We needed an indent before print, so let's do it again.
>>>
>>> for x in 'python':
... print(x)
...
p
y
t
h
o
n
>>>
click below button to copy the code. By Python tutorial team
- We needed an indent before the print statement and two enter keys.
- Note that we do not need the blank line after compound statement in a script file.
- It is required only at the interactive prompt. In a file, blank lines are simply ignored.
System Command Lines and Files
- The programs we type into the interactive prompt go away as soon as the Python interpreter executes them.
- Because the code we type interactively is never stored in a file, we can't run it again.
- To save programs, we need to write our code in files which are called modules.
- Once we have a code, we can run it by system command lines, by file icon clicks, and by options in the IDLE user interface.
A Script File
- Here is a script file named script1.py:
# Python script
import sys #loading a library module
import math
print(sys.platform)
print(math.sqrt(1001))
x = "blah ";
print x*3;
click below button to copy the code. By Python tutorial team
C:\workspace>
C:\workspace>python script1.py
win32
31.6385840391
blah blah blah
C:\workspace>
click below button to copy the code. By Python tutorial team
- Here is a brief description of the script:
- Imports a Python module - to fetch the name of the platform and to get sqrt() from math functions.
- Runs three print function calls.
- Uses a variable x, created when it's assigned, to hold a string object.
- The sys.platform is just a string that identifies the computer. It is in a standard Python module, sys, which we must import to load.
- The name of the module could be just stript1 without .py suffix. But files of code we want to import into should end with a .py. Because we may want to import the script file later, it's recommended to use .py suffix.
- We used shell command lines to start Python programs.
- So, we can use all the usual shell syntax.
- For example, we can route the output of a Python to a file to save it:
C:\workspace>python script1.py > saved_srcipt1
click below button to copy the code. By Python tutorial team
- The file saved_script1 will have the same output as its content:
win32
31.6385840391
blah blah blah
click below button to copy the code. By Python tutorial team
Running Script File from Icons
- On Windows, running the script by clicking on a file icon has a problem.
- We cannot see the result because it disappears as soon as it pops up.
- Actually, the script exits after printing the result.
- But there is a say to work around this. If we want to our script's output to stick around for a while, we can simply put a call to the built-in input function at the bottom of the script as shown below:
# Python script
import sys #loading a library module
import math
print(sys.platform)
print(math.sqrt(1001))
x = "blah ";
print x*3;
input()
click below button to copy the code. By Python tutorial team
- The input reads the next line of standard input. It waits if there is no input available.
- So, the net effect will be to pause the script and keeping the output window remained open until we press the Enter key.
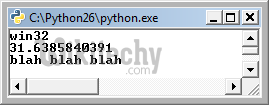
Learn Python - Python tutorial - python running - Python examples - Python programs
- Putting the input in our script should be used only when all the following conditions have been met:
- It is usually only required for Windows.
- The script prints text and exits.
- Running the script from icon.
- If we use the input trick, we may not get to see Python error messages. If our script generates an error, the error message text is written to the pop-up console window.
- Then it immediately disappears. Adding an input call to our file will not help this time because our script will likely abort long before it reaches this call.
The import Search Path
- Python looks in several places when we try to import a module. Actually, it looks in all the directories defined in sys.path.
>>> import sys
>>> sys.path
['',
'/usr/lib/python3.2',
...
'/usr/lib/python3.2/dist-packages',
'/usr/local/lib/python3.2/dist-packages']
click below button to copy the code. By Python tutorial team
- Importing the sys module makes all of its functions and attributes available.
- The sys.path is a list of directory names that constitute the current search path.
- Python will look through these directories, in this order, for a .py file whose name matches what we're trying to import.
- Actually, it is more complicated than that, because not all modules are stored as .py files.
- Some are built-in modules; they are actually baked right into Python itself.