1. find(“string”, beg, end) :- This function is used to find the position of the substring within a string.It takes 3 arguments, substring , starting index( by default 0) and ending index( by default string length).
- It returns “-1 ” if string is not found in given range.
- It returns first occurrence of string if found.
2. rfind(“string”, beg, end) :- This function has the similar working as find(), but it returns the position of the last occurrence of string.
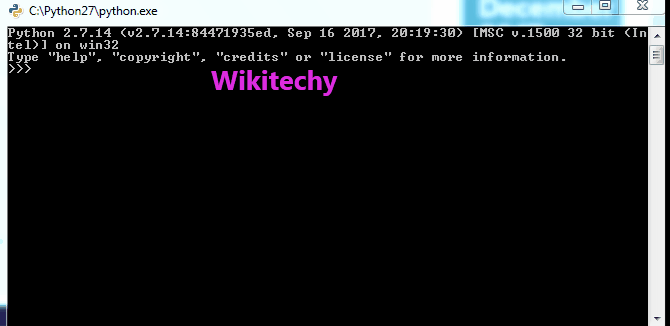
Learn Python - Python tutorial - python find - Python examples - Python programs
python - Sample - python code :
# Python code to demonstrate working of
# find() and rfind()
str = "wikitechy is a website"
str2 = "wikitechy"
# using find() to find first occurrence of str2
# returns 15
print ("The first occurrence of str2 is at : ", end="")
print (str.find( str2, 4) )
# using rfind() to find last occurrence of str2
# returns 9
print ("The last occurrence of str2 is at : ", end="")
print ( str.rfind( str2, 4) )
click below button to copy the code. By Python tutorial team
python tutorial - Output :
The first occurrence of str2 is at : 15
The last occurrence of str2 is at : 9
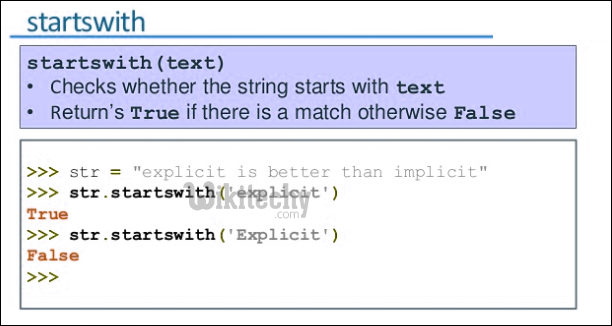
3. startswith(“string”, beg, end) :- The purpose of this function is to return true if the function begins with mentioned string(prefix) else return false.
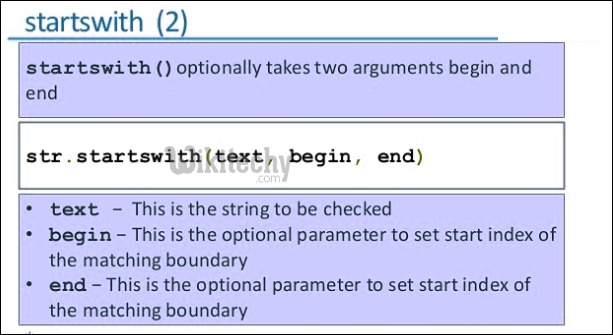
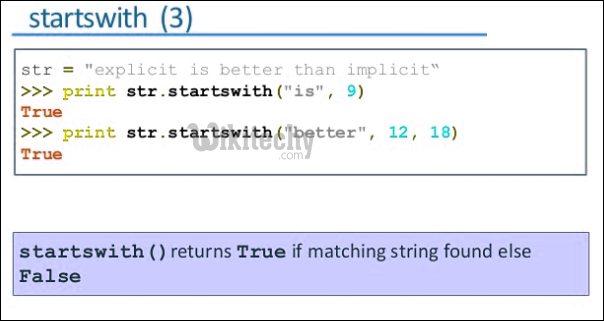
4. endswith(“string”, beg, end) :- The purpose of this function is to return true if the function ends with mentioned string(suffix) else return false.
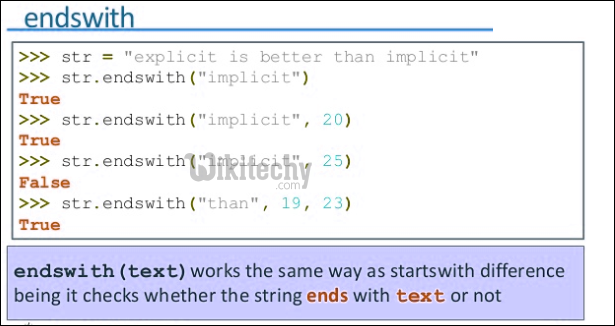
python - Sample - python code :
# Python code to demonstrate working of
# startswith() and endswith()
str = "wikitechy is a website"
str1 = "wikitechy"
# using startswith() to find if str starts with str1
if str.startswith(str1):
print ("str begins with str1")
else : print ("str does not begin with str1")
# using endswith() to find if str ends with str1
if str.startswith(str1):
print ("str ends with str1")
else : print ("str does not end with str1")
click below button to copy the code. By Python tutorial team
python tutorial - Output :
str begins with str1
str ends with str1
5. islower(“string”) :- This function returns true if all the letters in the string are
6. isupper(“string”) :- This function returns true if all the letters in the string are upper cased, otherwise false.
python - Sample - python code :
# Python code to demonstrate working of
# isupper() and islower()
str = "Wikitechy"
str1 = "wikitechy"
# checking if all characters in str are upper cased
if str.isupper() :
print ("All characters in str are upper cased")
else : print ("All characters in str are not upper cased")
# checking if all characters in str1 are lower cased
if str1.islower() :
print ("All characters in str1 are lower cased")
else : print ("All characters in str1 are not lower cased")
click below button to copy the code. By Python tutorial team
python tutorial - Output :
All characters in str are not upper cased
All characters in str1 are lower cased
7. lower() :- This function returns the new string with all the letters converted into its lower case. lower cased, otherwise false.
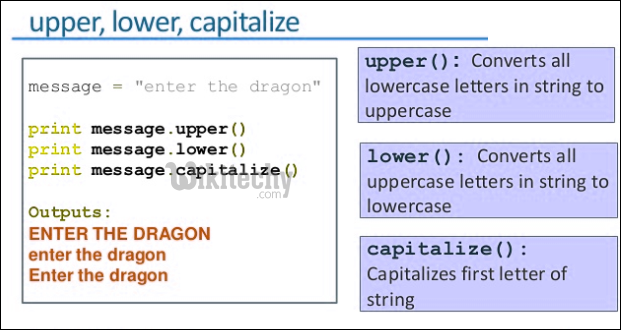
8. upper() :- This function returns the new string with all the letters converted into its upper case.
9. swapcase() :- This function is used to swap the cases of string i.e upper case is converted to lower case and vice versa.
10. title() :- This function converts the string to its title case i.e the first letter of every word of string is upper cased and else all are lower cased.
python - Sample - python code :
# Python code to demonstrate working of
# upper(), lower(), swapcase() and title()
str = "WikiTechy is A Worlds Best Learning WebSite"
# Coverting string into its lower case
str1 = str.lower();
print (" The lower case converted string is : " + str1)
# Coverting string into its upper case
str2 = str.upper();
print (" The upper case converted string is : " + str2)
# Coverting string into its swapped case
str3 = str.swapcase();
print (" The swap case converted string is : " + str3)
# Coverting string into its title case
str4 = str.title();
print (" The title case converted string is : " + str4)
click below button to copy the code. By Python tutorial team
python tutorial - Output :
The lower case converted string is : wikitechy is a worlds best learning website
The upper case converted string is : WIKITECHY IS A WORLDS BEST LEARNING WEBSITE
The swap case converted string is : wIKItECHY IS A wORLDS bEST lEARNING wEBsITE
The title case converted string is : Wikitechy Is A Worlds Best Learning Website