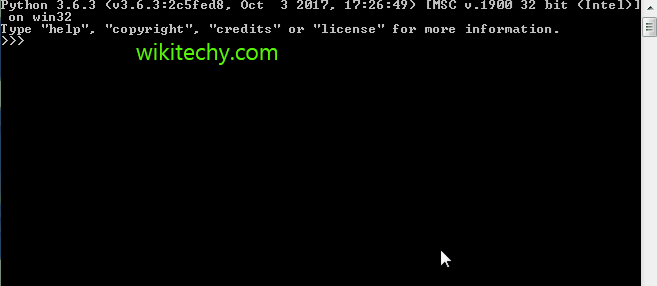
Learn Python - Python tutorial - python oops - Python examples - Python programs
What is an object?
- Everything in Python is an object, and everything can have attributes and methods.
- All functions have a built-in attribute __doc__, which returns the docstring defined in the function's source code.
- For example, sys module is an object which has an attribute called path, and so forth.
- Different programming languages define object in different ways. In some, it means that all objects must have attributes and methods; in others, it means that all objects are subclassable.
- In Python, the definition is looser. Some objects have neither attributes nor methods, but they could. Not all objects are subclassable.
- But everything is an object in the sense that it can be assigned to a variable or passed as an argument to a function
- You may have heard the term first-class object in other programming contexts.
- In Python, functions are first-class objects. You can pass a function as an argument to another function.
- Modules are first-class objects. You can pass an entire module as an argument to a function.
- Classes are first-class objects, and individual instances of a class are also first-class objects.
- This is important, so I'm going to repeat it in case you missed it the first few times: everything in Python is an object.
- Strings are objects. Lists are objects. Functions are objects. Classes are objects. Class instances are objects. Even modules are objects.
- This section on object is from "Dive into Python 3" by Mark Pilgrim.
Below is a simple Python program that creates a class with single method.
python - Sample - python code :
# A simple example class
class Test:
# A sample method
def fun(self):
print("Hello")
# Driver code
obj = Test()
obj.fun()
Output:
Hello
As we can see above, we create a new class using the class statement and the name of the class. This is followed by an indented block of statements which form the body of the class. In this case, we have defined a single method in the class.
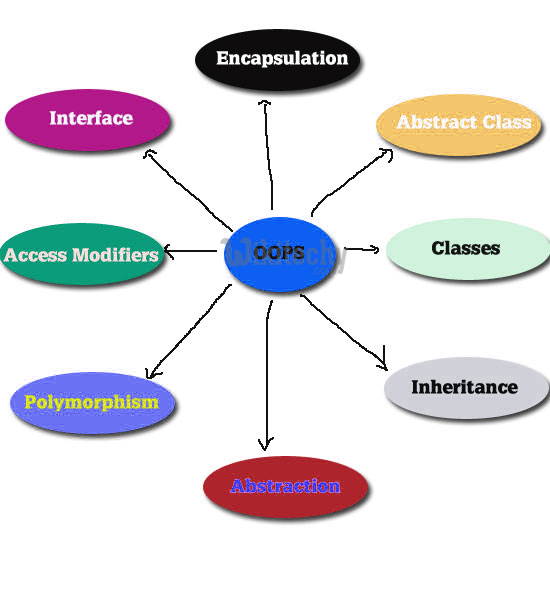
Next, we create an object/instance of this class using the name of the class followed by a pair of parentheses.
The self
- Class methods must have an extra first parameter in method definition. We do not give a value for this parameter when we call the method, Python provides it
- If we have a method which takes no arguments, then we still have to have one argument – the self. See fun() in above simple example.
- This is similar to this pointer in C++ and this reference in Java.
When we call a method of this object as myobject.method(arg1, arg2), this is automatically converted by Python into MyClass.method(myobject, arg1, arg2) – this is all the special self is about.
The __init__ method
The __init__ method is similar to constructors in C++ and Java. It is run as soon as an object of a class is instantiated. The method is useful to do any initialization you want to do with your object.
python - Sample - python code :
# A Sample class with init method
class Person:
# init method or constructor
def __init__(self, name):
self.name = name
# Sample Method
def say_hi(self):
print('Hello, my name is', self.name)
p = Person('Shwetanshu')
p.say_hi()
Output:
Hello, my name is Shwetanshu
Here, we define the __init__ method as taking a parameter name (along with the usual self). .
Class and Instance Variables (Or attributes)
In Python, instance variables are variables whose value is assigned inside a constructor or method with self.
Class variables are variables whose value is assigned in class.
python - Sample - python code :
# Python program to show that the variables with a value
# assigned in class declaration, are class variables and
# variables inside methods and constructors are instance
# variables.
# Class for Computer Science Student
class CSStudent:
# Class Variable
stream = 'cse'
# The init method or constructor
def __init__(self, roll):
# Instance Variable
self.roll = roll
# Objects of CSStudent class
a = CSStudent(101)
b = CSStudent(102)
print(a.stream) # prints "cse"
print(b.stream) # prints "cse"
print(a.roll) # prints 101
# Class variables can be accessed using class
# name also
print(CSStudent.stream) # prints "cse"
python tutorial - Output :
Noida, UP
How to create an empty class?
We can create an empty class using pass statement in Python.
python - Sample - python code :
# Python program to show that we can create
# instance variables inside methods
# Class for Computer Science Student
class CSStudent:
# Class Variable
stream = 'cse'
# The init method or constructor
def __init__(self, roll):
# Instance Variable
self.roll = roll
# Adds an instance variable
def setAddress(self, address):
self.address = address
# Retrieves instance variable
def getAddress(self):
return self.address
# Driver Code
a = CSStudent(101)
a.setAddress("Noida, UP")
print(a.getAddress())