A Tuple is a collection of Python objects separated by commas. In someways a tuple is similar to a list in terms of indexing, nested objects and repetition but a tuple is immutable unlike lists which are mutable.
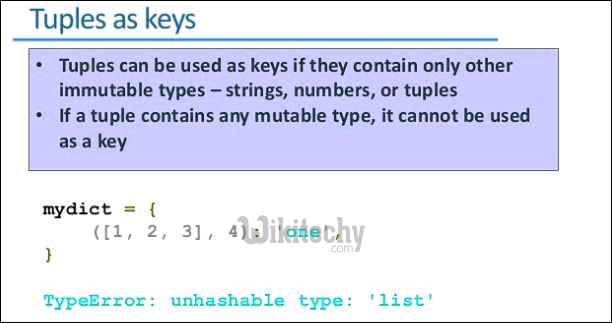
Creating Tuples
# An empty tuple
empty_tuple = ()
print (empty_tuple)
python tutorial - Output :
()
python - Sample - python code :
# Creating non-empty tuples
# One way of creation
tup = 'python', 'wikitechy'
print(tup)
# Another for doing the same
tup = ('python', 'wikitechy')
print(tup)
python tutorial - Output :
('python', 'wikitechy')
('python', 'wikitechy')
Note: In case your generating a tuple with a single element, make sure to add a comma after the element.
Concatenation of Tuples
# Code for concatenating 2 tuples
tuple1 = (0, 1, 2, 3)
tuple2 = ('python', 'wikitechy')
# Concatenating above two
print(tuple1 + tuple2)
Output:
(0, 1, 2, 3, 'python', 'wikitechy')
Nesting of Tuples
# Code for creating nested tuples
tuple1 = (0, 1, 2, 3)
tuple2 = ('python', 'wikitechy')
tuple3 = (tuple1, tuple2)
print(tuple3)
python tutorial - Output :
((0, 1, 2, 3), ('python', 'wikitechy'))
Repetition in Tuples
# Code to create a tuple with repetition
tuple3 = ('python',)*3
print(tuple3)
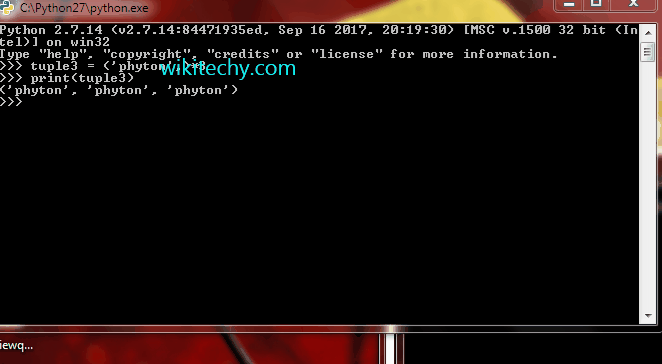
Learn Python - Python tutorial - python repetition tuples - Python examples - Python programs
python tutorial - Output :
('python', 'python', 'python')
Try the above without a comma and check. You will get tuple3 as a string ‘pythonpythonpython’.
Immutable Tuples
#code to test that tuples are immutable
tuple1 = (0, 1, 2, 3)
tuple1[0] = 4
print(tuple1)
python tutorial - Output :
Traceback (most recent call last):
File "e0eaddff843a8695575daec34506f126.py", line 3, in
tuple1[0]=4
TypeError: 'tuple' object does not support item assignment
Slicing in Tuples
# code to test slicing
tuple1 = (0 ,1, 2, 3)
print(tuple1[1:])
print(tuple1[::-1])
print(tuple1[2:4])
python tutorial - Output :
(1, 2, 3)
(3, 2, 1, 0)
(2, 3)
Deleting a Tuple
tuple3 = ( 0, 1)
del tuple3
print(tuple3)
Error:
Traceback (most recent call last):
File "d92694727db1dc9118a5250bf04dafbd.py", line 6, in <module>
print(tuple3)
NameError: name 'tuple3' is not defined
python tutorial - Output :
(0, 1)
Finding Length of a Tuple
# Code for printing the length of a tuple
tuple2 = ('python', 'wikitechy')
print(len(tuple2))
python tutorial - Output :
2
Converting list to a Tuple
# Code for converting a list and a string into a tuple
list1 = [0, 1, 2]
print(tuple(list1))
print(tuple('python')) # string 'python'
python tutorial - Output :
(0, 1, 2)
('p', 'y', 't', 'h', 'o', 'n')
Takes a single parameter which may be a list,string,set or even a dictionary( only keys are taken as elements) and converts them to a tuple.
Tuples in a loop
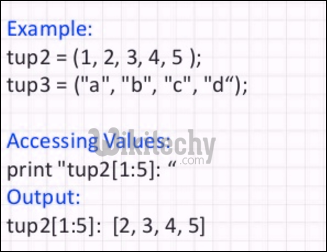
python - Sample - python code :
#python code for creating tuples in a loop
tup = ('wikitechy',)
n = 5 #Number of time loop runs
for i in range(int(n)):
tup = (tup,)
print(tup)
python tutorial - Output :
(('wikitechy',),)
((('wikitechy',),),)
(((('wikitechy',),),),)
((((('wikitechy',),),),),)
(((((('wikitechy',),),),),),)
Using cmp(), max() , min()
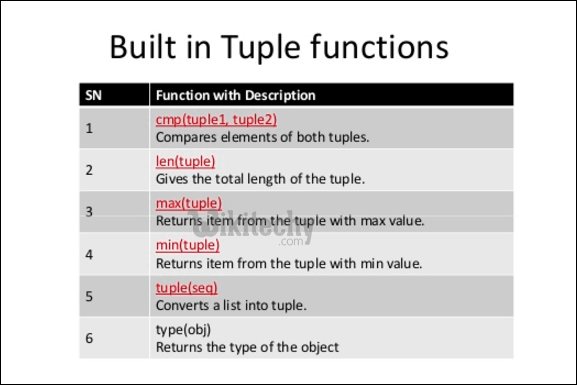
python - Sample - python code :
# A python program to demonstrate the use of
# cmp(), max(), min()
tuple1 = ('python', 'wikitechy')
tuple2 = ('coder', 1)
if (cmp(tuple1, tuple2) != 0):
# cmp() returns 0 if matched, 1 when not tuple1
# is longer and -1 when tuple1 is shoter
print('Not the same')
else:
print('Same')
print ('Maximum element in tuples 1,2: ' +
str(max(tuple1)) + ',' +
str(max(tuple2)))
print ('Minimum element in tuples 1,2: ' +
str(min(tuple1)) + ',' + str(min(tuple2)))
python tutorial - Output :
Not the same
Maximum element in tuples 1,2: python,coder
Minimum element in tuples 1,2: wikitechy,1
Note: max() and min() checks the based on ASCII values. If there are two strings in a tuple, then the first different character in the strings are checked.