1. setitem(ob, pos, val) :- This function is used to assign the value at a particular position in the container.
Operation – ob[pos] = val
2. delitem(ob, pos) :- This function is used to delete the value at a particular position in the container.
Operation – del ob[pos]
3. getitem(ob, pos) :- This function is used to access the value at a particular position in the container.
Operation – ob[pos]
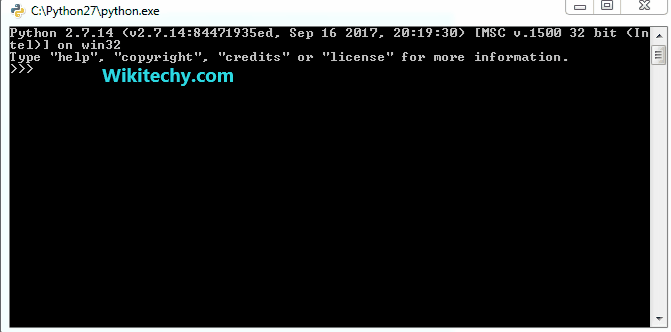
Learn Python - Python tutorial - python operator function - Python examples - Python programs
python - Sample - python code :
# Python code to demonstrate working of
# setitem(), delitem() and getitem()
# importing operator module
import operator
# Initializing list
li = [1, 5, 6, 7, 8]
# printing original list
print ("The original list is : ",end="")
for i in range(0,len(li)):
print (li[i],end=" ")
print ("\r")
# using setitem() to assign 3 at 4th position
operator.setitem(li,3,3)
# printing modified list after setitem()
print ("The modified list after setitem() is : ",end="")
for i in range(0,len(li)):
print (li[i],end=" ")
print ("\r")
# using delitem() to delete value at 2nd index
operator.delitem(li,1)
# printing modified list after delitem()
print ("The modified list after delitem() is : ",end="")
for i in range(0,len(li)):
print (li[i],end=" ")
print ("\r")
# using getitem() to access 4th element
print ("The 4th element of list is : ",end="")
print (operator.getitem(li,3))
python tutorial - Output :
The original list is : 1 5 6 7 8 The modified list after setitem() is : 1 5 6 3 8 The modified list after delitem() is : 1 6 3 8 The 4th element of list is : 8
4. setitem(ob, slice(a,b), vals) :- This function is used to set the values in a particular range in the container.
Operation – obj[a:b] = vals
5. delitem(ob, slice(a,b)) :- This function is used to delete the values from a particular range in the container.
Operation – del obj[a:b]
6. getitem(ob, slice(a,b)) :- This function is used to access the values in a particular range in the container.
Operation – obj[a:b]
python - Sample - python code :
# Python code to demonstrate working of
# setitem(), delitem() and getitem()
# importing operator module
import operator
# Initializing list
li = [1, 5, 6, 7, 8]
# printing original list
print ("The original list is : ",end="")
for i in range(0,len(li)):
print (li[i],end=" ")
print ("\r")
# using setitem() to assign 2,3,4 at 2nd,3rd and 4th index
operator.setitem(li,slice(1,4),[2,3,4])
# printing modified list after setitem()
print ("The modified list after setitem() is : ",end="")
for i in range(0,len(li)):
print (li[i],end=" ")
print ("\r")
# using delitem() to delete value at 3rd and 4th index
operator.delitem(li,slice(2,4))
# printing modified list after delitem()
print ("The modified list after delitem() is : ",end="")
for i in range(0,len(li)):
print (li[i],end=" ")
print ("\r")
# using getitem() to access 1st and 2nd element
print ("The 1st and 2nd element of list is : ",end="")
print (operator.getitem(li,slice(0,2)))
python tutorial - Output :
The original list is : 1 5 6 7 8 The modified list after setitem() is : 1 2 3 4 8 The modified list after delitem() is : 1 2 8 The 1st and 2nd element of list is : [1, 2]
7. concat(ob1,obj2) :- This function is used to concatenate two containers.
Operation – obj1 + obj2
8. contains(ob1,obj2) :- This function is used to check if obj2 in present in obj1.
Operation – obj2 in obj1
python - Sample - python code :
# Python code to demonstrate working of
# concat() and contains()
# importing operator module
import operator
# Initializing string 1
s1 = "wiki"
# Initializing string 2
s2 = "techy"
# using concat() to concatenate two strings
print ("The concatenated string is : ",end="")
print (operator.concat(s1,s2))
# using contains() to check if s1 contains s2
if (operator.contains(s1,s2)):
print ("wiki contains techy")
else : print ("wiki does not contain techy")
python tutorial - Output :
The concatenated string is : wikitechy wiki contains techy
9. and_(a,b) :- This function is used to compute bitwise and of the mentioned arguments.
Operation – a & b
10. or_(a,b) :- This function is used to compute bitwise or of the mentioned arguments.
Operation – a | b
11. xor(a,b) :- This function is used to compute bitwise xor of the mentioned arguments.
Operation – a ^ b
12. invert(a) :- This function is used to compute bitwise inversion of the mentioned argument.
Operation – ~ a
python - Sample - python code :
# Python code to demonstrate working of
# and_(), or_(), xor(), invert()
# importing operator module
import operator
# Initializing a and b
a = 1
b = 0
# using and_() to display bitwise and operation
print ("The bitwise and of a and b is : ",end="")
print (operator.and_(a,b))
# using or_() to display bitwise or operation
print ("The bitwise or of a and b is : ",end="")
print (operator.or_(a,b))
# using xor() to display bitwise exclusive or operation
print ("The bitwise xor of a and b is : ",end="")
print (operator.xor(a,b))
# using invert() to invert value of a
operator.invert(a)
# printing modified value
print ("The inverted value of a is : ",end="")
print (a)
python tutorial - Output :
The bitwise and of a and b is : 0 The bitwise or of a and b is : 1 The bitwise xor of a and b is : 1 The inverted value of a is : 1